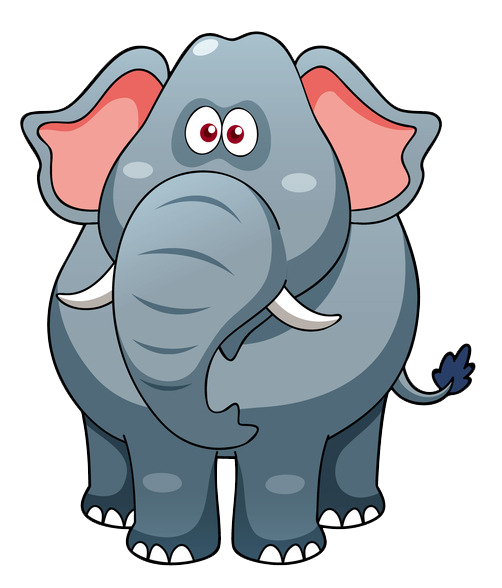
Correction status:qualified
Teacher's comments:常用的数据少了一个 undefined
...
<script>
console.log(100);
console.log(typeof(100));
console.log(typeof(100.1));
console.log(typeof('100'));
console.log(typeof(true));
let a;
console.log(typeof(a));
// null返回的是object不是null
console.log(typeof(null));
// 变量由值类型决定,而且可以变化
let n = 123;
console.log(typeof(n));
n = '123'
console.log(typeof(n));
</script>
数组的每个成员可以是普通类型,也可以是引用。
...
<script>
...
const arr = ['电脑','2','5000']
console.table(arr);
// 分别打印数组成员
console.log(arr[0]);
console.log(arr[1]);
console.log(arr[2]);
// 测试数组长度
console.log(arr.length);
// 判断数组的类型,对数组、函数、对象的引用都返回object。
console.log(typeof(arr));
// 准确判断数组的方法,返回true则表示是数组。
console.log(Array.isArray(arr));
...
</script>
...
<script>
...
obj = {
name:'电脑',
num: 2,
price: 5000,
'contact email': 'mail@mail.com',
total:function(){
return this.name+'的价格是'+this.price;
},
};
console.log(obj.name);
console.log(obj.price);
// 对于不规范的命名要使用[]引用
console.log(obj['contact email']);
// 对象中函数的调用
console.log(obj.total());
...
</script>
既是数据类型也是对象
...
// 数据类型:函数
console.log(typeof(function(){}));
// 函数也是对象
console.log(function(){} instanceof Object);
...
将函数当成数据类型的优点
优点1 当成函数的参数:回调
优点2 当成函数返回值:闭包
...
function f1(){}
// 把函数当成参数
function f2(f){
console.log(typeof f);
}
f2(f1);
// 把函数当成返回值:闭包
function f3(){
return function(){
return 'hello world';
};
};
//注意三种打印的不同
console.log(f3);
console.log(f3());
console.log(f3()());
...
...
<script>
...
// 单分支
if (true) console.log('这是单分支');
// 双分支
if(true){
console.log('这是多分支的true');
}else{
console.log('这是多分支的false');
}
// 双分支的简化写法
console.log( true ?'条件为true':'条件为false');
// 多分支
let condition;
if(condition == true){
console.log('条件为true');
}else if(condition == false){
console.log('条件为false');
}else{
console.log('不知道啥条件');
}
// switch多分支
switch(condition){
case true:
console.log('条件为true');
break;
case false:
console.log('条件为false');
break;
default:
console.log('不知道啥条件');
}
...
</script>
...
循环三要素:
1、索引初始化
2、循环条件
3、更新循环条件
例,数组遍历
...
<script>
...
const colors = ['red','green','blue'];
// 索引初始化
let i = 0;
// 数组长度
let length = colors.length;
// 入口判断循环条件
while(i < length){
console.log(colors[i]);
// 更新循环条件
i++;
}
// 出口判断循环条件,最少执行一次。
i = 0;
do{
console.log(colors[i]);
i++;
}while(i < colors.length)
// for循环
for(i = 0; i < colors.length; i++){
console.log(colors[i]);
}
// for-of方式打印数组成员
for(let item of colors.values()){
console.log('成员:'+item);
}
// for-of方式打印数组键值
for(let item of colors.keys()){
console.log('键值:'+item);
}
// 键值都打印
for(let item of colors.entries()){
console.log('键值+成员:'+item);
}
// for-in遍历数组
for(let key in colors){
console.log(colors[key]);
}
...
</script>
...
例,对象遍历
对象不能用for-of,用for-in。
...
<script>
...// for-in遍历对象
const obj = {
a:1,
b:2,
f:function (){},
}
for(let key in obj){
console.log(obj[key]);
}
...
</script>
...