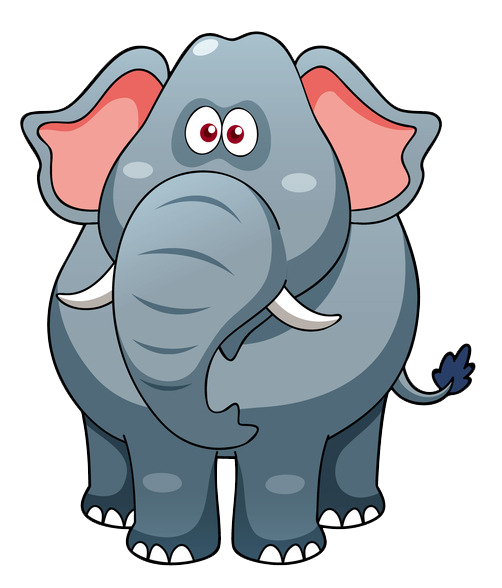
Correction status:qualified
Teacher's comments:
代码如下:
<script>
//1. 纯数组:Araay:
let arr = ['red','yellow','blue'];
console.log(arr);
// Array(3) [ "red", "yellow", "blue" ]
//
// 0: "red"
//
// 1: "yellow"
//
// 2: "blue"
//
// length: 3
// <prototype>: Array [] //这是一个纯数组
// 2. 类数组:就是一个对象字面量, 结构类似数组:
const obj ={1:'red',2:'yellow',3:'blue'};
console.log(obj);
console.log(obj[1]);
// Object { 1: "red", 2: "yellow", 3: "blue" }
//
// 1: "red"
//
// 2: "yellow"
//
// 3: "blue"
//
// <prototype>: Object { … } 这个是类数组
// 类数组的使用:1.遍历函数参数
// 2.类数组和纯数组的访问方式一样,类数组可以转为纯数组
console.log('-----------------------');
// 将OBJ转为纯数组:可以用:Array.from():
obj1 = {1:'猪老师',2:'马老师',3:'牛老师'}
let objArr = Array.from(obj1);
console.log(objArr);
</script>
效果图展示:
代码如下:
<script>
// DOM元素的API有2个:1.获取一组元素:querySelectorAll()
// 2.获取单个元素:qureySeletcor()
// 使用场景:DOM遍历
// 获取一组元素:
const items = document.querySelectorAll('.list .item');
// console.log(items); 可以拿到全部的li
// 用箭头函数简写:
items.forEach(bg =>(bg.style.backgroundColor='yellow'));
// items.forEach(function bg(v){
// v.style.backgroundColor='blue';
// });
// 获取一个元素:
const item = document.querySelector('.list .item:last-of-type');
// console.log(item);获取到了最后一个li
console.log(item);
item.style.backgroundColor = 'cyan';
// item.forEach(bg =>(bg.style.backgroundColor='red'));
</script>
效果图展示:
代码如下:
<script>
const ipt = document.querySelectorAll('.login input');
console.log(ipt); //获取到了表单里面的全部input元素
ipt.forEach(cl=>(cl.style.color='red')); // 把所有input元素的文本颜色改为红色
const psw = document.querySelector('.login input[ type="password"]');
// 获取密码的input 输入框
console.log(psw);
// 获取密码值:
let password = psw.value;
console.log(password);
// 下面是优雅的获取方式: document.forms
console.log(document.forms.login);
console.log(document.forms.login.username);
console.log(document.forms.login.username.value);
let user = document.forms.login.username.value;
console.log(user);
function us(user){
return user = 'admin888';
}
console.log(us());
// 转为箭头函数:
us = user =>(user='admin888');
console.log(us());
</script>
效果图展示:
代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>DOM元素 API</title>
</head>
<body>
<ul class="list">
<li class="item">item1</li>
<li class="item">item2</li>
<li class="item">item3</li>
<li class="item">item4</li>
<li class="item">item5</li>
</ul>
<script>
// dom元素的遍历:1.全局类型:window
// 2.文档类型:docunment 当前HTML
// 3.元素类型:element
// 4.文本类型:text
let all= window.innerHeight;
// console.log(all);
let items = document.querySelector('.list');
// 查询ul有多少个节点,这个把换行也算到里面的:
console.log(items.childNodes);
console.log(items.children); //获取的是一个类数组:
// 将类数组转为纯数组:
// console.log(Array.from(items.children));
console.log([...items.children]);
[...items.children].forEach(li =>(li.style.color='red'));
let item = [...items.children];
console.log(item[0]);
item[0].style.color= 'green';
item[3].style.color = 'blue';
// 第一个兄弟:firstElementChild
items.firstElementChild.style.backgroundColor = 'yellow';
// 当前兄弟的下一个兄弟:firstElementChild.nextElementSibling;
items.firstElementChild.nextElementSibling.style.backgroundColor = 'green';
// 最后一个兄弟:lastElementChild
items.lastElementChild.style.backgroundColor = '#eee';
// 当前兄弟的前一个兄弟:
items.lastElementChild.previousElementSibling.style.backgroundColor = 'pink';
// 当前兄弟的父类:
items.lastElementChild.parentElement.style.border='1px solid #000';
// 修改最后一个兄弟的文本:文本类型:text
// items.lastElementChild.textContent = 'php.cn';
items.lastElementChild.textContent = 'php.cn';
</script>
</body>
</html>
效果图展示: