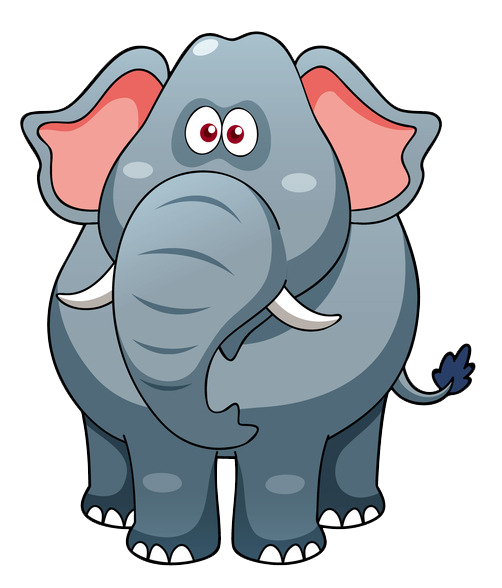
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>轮播图</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.silder {
width: 400px;
margin: 10px auto;
}
.images img {
width: 100%;
display: none;
}
.images img.active {
display: block;
}
.btns {
display: flex;
place-content: center;
}
.btns span {
width: 10px;
height: 10px;
border-radius: 50%;
background-color: gray;
margin: -20px 5px 5px;
}
.btns span.active {
background-color: yellow;
}
</style>
</head>
<body>
<div class="silder">
<div class="images"></div>
<div class="btns"></div>
</div>
<script>
// 3. 在页面初始化时,自动加载以上内容,事件是 load
window.addEventListener("load", fun, false);
let imageArr = ["banner-1.jpg", "banner-2.jpg", "banner-3.jpg", "banner-4.jpg"];
const images = document.querySelector(".silder .images");
const btns = document.querySelector(".btns");
function fun() {
imageArr.forEach(function (item, index) {
if (index == 0) {
// 1. 将所有图片放在一个数组中, 并动态添加到页面中
images.insertAdjacentHTML("beforeend", `<a href="#"><img src="./images/${item}" alt=" " class="active" data-index=${index}></a>`);
// 2. 根据图片数量,动态创建按钮组,并动态添加到页面中
btns.insertAdjacentHTML("beforeend", `<span class="active" data-index="${index}"></span>`);
} else {
// 1. 将所有图片放在一个数组中, 并动态添加到页面中
images.insertAdjacentHTML("beforeend", `<a href="#"><img src="./images/${item}" alt=" " data-index=${index}></a>`);
// 2. 根据图片数量,动态创建按钮组,并动态添加到页面中
btns.insertAdjacentHTML("beforeend", `<span data-index="${index}"></span>`);
}
});
// console.log(document.body);
// console.log(imageArr);
const imgs = document.querySelectorAll(".silder .images img");
const btns2 = document.querySelectorAll(".silder .btns span");
btns.addEventListener("click", fun2, false);
// console.log(imgs, btns2);
function fun2() {
// console.log(event.target);
btns2.forEach(function (item) {
item.classList.remove("active");
});
// console.log(event.target);
// console.log(event.currentTarget);
imgs.forEach((item) => item.classList.remove("active"));
event.target.classList.add("active");
// console.log(event.target.dataset.index);
// console.log(imgs);
imgs.forEach((item) => {
// console.log(item.dataset.index);
if (item.dataset.index === event.target.dataset.index) {
item.classList.add("active");
}
});
}
// 4. [选做]: 为轮播图添加翻页按钮,提供翻页功能,提示:参考定时播放
setInterval(
function (arr) {
// console.log(arr);
let index = arr.shift();
// btns2[index].dispatchEvent(new Event("click"));
btns2[index].click();
arr.push(index);
// console.log(arr);
},
3000,
Object.keys(btns2)
);
}
</script>
<script>
// 6. 任选5个课程上没讲过的数组方法,实例演示
let arr1 = [3, 4, 1, 5];
let arr2 = [9, 3, 4, 1];
console.log("concat() 连接两个或多个数组:" + arr1.concat(arr2));
const obj = arr1.entries();
console.log("entries()返回一个新的 Array Iterator 对象:" + obj.next().value);
//arr.fill(value[, start[, end]]),会修改原数组
console.log(arr1.fill(9));
console.log(arr1.fill(8, 2));
console.log(arr1.fill(7, 1, 3));
//arr.includes(valueToFind[, fromIndex])
console.log(arr2.includes(4));
console.log(arr2.includes(3, 2));
//arr.reverse() 返回颠倒后的数组
console.log(arr2.reverse());
//arr.keys 返回数组的键组成的Array Iterator对象。
const iterator = arr2.keys();
for (const key of iterator) {
console.log(key);
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>// 5. 仿写php中文网编程词典选项卡</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body > div {
width: 400px;
margin-top: 20px;
/* display: grid; */
/* place-content: center; */
}
.list {
display: grid;
grid-template-columns: repeat(3, 100px);
grid-auto-rows: 40px;
place-content: space-evenly;
place-items: center;
font-size: large;
margin: auto;
}
.list .btn {
background-color: green;
width: 80px;
height: 30px;
text-align: center;
border-radius: 10px;
}
.list .active {
background-color: purple;
cursor: pointer;
}
.list .active:hover,
.content .item:hover {
cursor: pointer;
}
.content {
width: 350px;
height: 300px;
position: fixed;
top: 70px;
left: 25px;
}
.content .item {
width: 350px;
height: 300px;
text-align: center;
display: none;
}
.item.active {
display: block;
}
.content div:first-of-type {
background-color: red;
}
.content div:nth-of-type(2) {
background-color: yellow;
}
.content div:last-of-type {
background-color: orange;
}
</style>
</head>
<body>
<div>
<div class="list">
<div class="btn active" data-index="1">按钮1</div>
<div class="btn" data-index="2">按钮2</div>
<div class="btn" data-index="3">按钮3</div>
</div>
<div class="content">
<div class="item active" data-index="1">内容1</div>
<div class="item" data-index="2">内容2</div>
<div class="item" data-index="3">内容3</div>
</div>
</div>
<script>
// const list = document.querySelector(".list");
const lists = document.querySelectorAll(".list .btn");
// console.log(lists);
const contents = document.querySelectorAll(".content .item");
// console.log(contents);
lists.forEach((item, index) => item.addEventListener("click", fun, false));
function fun() {
lists.forEach((item, index) => item.classList.remove("active"));
event.currentTarget.classList.add("active");
contents.forEach((item, index) => item.classList.remove("active"));
contents.forEach((item, index) => {
if (item.dataset.index == event.currentTarget.dataset.index) {
item.classList.add("active");
}
});
}
</script>
</body>
</html>