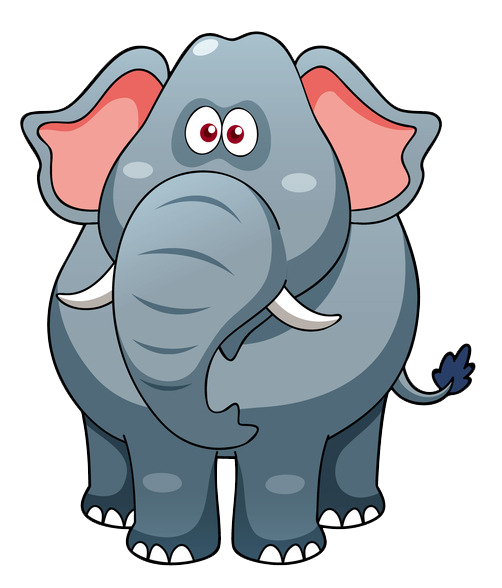
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
table {
width: 20em;
height: 10em;
text-align: center;
border-collapse: collapse;
margin: 1em;
}
table caption {
font-size: 1.2em;
padding: 1em;
margin-bottom: 2em;
border-bottom: 1px solid;
}
tbody tr th:first-of-type {
background: linear-gradient(to left, lightcyan, #fff);
border-right: 1px solid;
}
body tbody tr:not(:last-of-type) > * {
border-bottom: 1px solid;
}
</style>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<!-- es6 -->
<!-- <p> -->
<!-- 事件属性 -->
<!-- <a href="https://www.php.cn" onclick="showUrl(this)">show URL : </a>
<span class="url"></span>
</p> -->
<!-- <script>
// es6 原生方法
function showUrl(ele) {
event.preventDefault();
console.log(ele.nextElementSibling);
console.log(event.currentTarget.nextElementSibling);
// event.currentTarget.nextElementSibling.textContent = event.currentTarget.href;
ele.nextElementSibling.textContent = ele.href;
}
</script> -->
<!-- Vue -->
<!-- <div id="app"> -->
<!-- 事件属性 -->
<!-- <a href="https://www.php.cn" v-on:click="showUrl($event)">show URL : </a> -->
<!-- <span v-text="url"></span> -->
<!-- <span v-text="'url'"></span> -->
<!-- </div> -->
<!-- 循环渲染 -->
<!-- <div id="app">
<ul>
<li v-text="cities[0]"></li>
<li v-text="cities[1]"></li>
<li v-text="cities[2]"></li>
<li v-text="cities[3]"></li>
</ul>
<ul>
<li v-for="city of cities" v-bind:key="index">{{city}}</li>
</ul>
<ul>
<li v-text="user.name"></li>
<li v-text="user.age"></li>
</ul>
<ul>
<li v-for="(user,index) of users" v-bind:key="index">第{{++index}}-{{user.name}}:{{user.age}}岁</li>
</ul>
</div> -->
<!-- 条件渲染 -->
<!-- <div id="app">
<p v-if="flag">{{content}}</p>
<button v-on:click="flag = !flag" v-text="flag ? '隐藏':'显示'"></button>
<p v-if="distance >1000">我在{{cities[0]}}</p>
<p v-else-if="distance>800 & distance <=1000">我在{{cities[1]}}</p>
<p v-else-if="distance >500 & distance <=800">我在{{cities[2]}}</p>
<p v-else-if="distance <=500">我在{{cities[3]}}</p>
</div> -->
<!-- Vue留言版 -->
<!-- <div id="app"> -->
<!-- <input type="text" placeholder="请输入你的留言" v-on:keydown="talk($event)" /> -->
<!-- enter:键盘修饰符,代表回车 .enter -->
<!-- <input type="text" placeholder="请输入你的留言" v-on:keydown.enter="talk($event)" />
<ul>
<li v-for="msg of message" :key="index">{{msg}}</li>
</ul>
</div> -->
<!-- 计算属性,侦听器属性 -->
<div id="app">
<table>
<caption>
商品结算
</caption>
<tbody>
<tr>
<th>ID</th>
<td>HA110</td>
</tr>
<tr>
<th>品名</th>
<td>伊利纯牛奶</td>
</tr>
<tr>
<th>单价</th>
<td>100</td>
</tr>
<tr>
<th>数量</th>
<td><input type="number" v-model="num" style="width: 5em" /></td>
</tr>
<tr>
<th>金额</th>
<!-- <td>{{num * price}}</td> -->
<td>{{amount}}</td>
</tr>
</tbody>
</table>
<p style="font-size: 1.2em">
实付金额: {{realAmount}}, 优惠了 :
<span style="color: red">{{difAmount}}</span>
</p>
</div>
<script>
const app = Vue.createApp({
data() {
return {
url: null,
cities: ["北京", "上海", "杭州", "厦门"],
user: {
name: "小鸡",
age: 18,
},
users: [
{
name: "小马",
age: 19,
},
{
name: "小猪",
age: 20,
},
{
name: "小牛",
age: 30,
},
],
content: "你找到我了,恭喜你",
flag: false,
distance: 600,
message: [],
price: 100,
num: 0,
discount: 1,
};
},
methods: {
showUrl(ev) {
ev.preventDefault();
this.url = ev.currentTarget.href;
},
talk(ev) {
console.log(ev);
// if (ev.key === "Enter") {
// this.message.unshift(ev.currentTarget.value);
// ev.currentTarget.value = null;
// }
this.message.unshift(ev.currentTarget.value);
ev.currentTarget.value = null;
},
},
//计算属性(访问器属性)
computed: {
amount: {
get() {
return this.price * this.num;
},
},
},
//侦听属性
watch: {
amount(current, origin) {
console.log(current, origin);
switch (true) {
case current >= 1000 && current < 2000:
this.discount = 0.9;
break;
case current >= 2000 && current < 3000:
this.discount = 0.8;
break;
case current >= 3000 && current < 5000:
this.discount = 0.6;
break;
}
this.realAmount = this.amount * this.discount;
this.difAmount = this.amount - this.realAmount;
},
},
mounted() {
this.num = 1;
},
}).mount("#app");
</script>
</body>
</html>