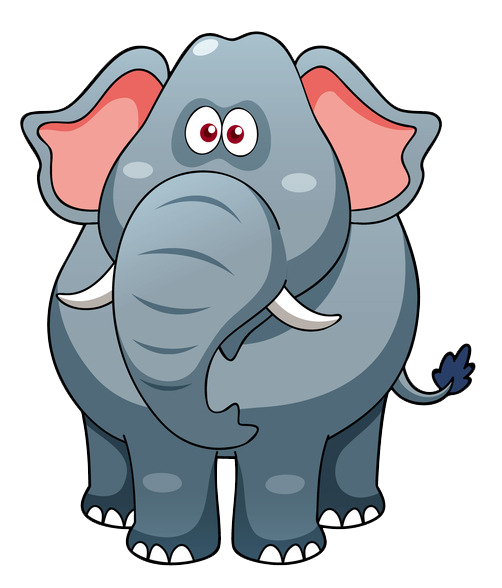
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>事件绑定</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<!-- es6 -->
<p>
<!-- <a href="https://www.baidu.com" onclick = "showURL( )">URL地址跳转 </a> -->
<!-- 事件属性 -->
<a href="https://www.php.cn" onclick="showUrl()">显示地址一 : </a>
<span class="url"> </span>
</p>
<!-- vue -->
<div class="app">
<p >
<!-- <a href="https://www.baidu.com" onclick = "showURL( )">URL地址跳转 </a>
-->
<!-- 事件属性 v-on @ -->
<a href="https://www.php.cn" @click="showUrl1($event)">显示地址二 : </a>
<span class="url1">{{url1}} </span>
</p>
</div>
<!-- 简化 -->
<div class="app">
<p onclick=" console.log( '你好呀');">
<!-- <a href="https://www.baidu.com" onclick = "showURL( )">URL地址跳转 </a>
-->
<!-- 事件属性 v-on @ -->
<a href="https://www.php.cn" @click="showUrl2($event)">显示地址三 : </a>
<span class="url2">{{url2}} </span>
</p>
</div>
<script>
// 为事件属性绑定 事件方法
function showUrl(){
// 禁用跳转行为
event.preventDefault();
console.log( EventTarget);
console.log(event.target);
console.log(event.target.href);
console.log(event.currentTarget);
console.log(event.currentTarget.href);
console.log('=======================');
// 获取下一个兄弟节点event.nextElementSibling.textConent
console.log(event.target.nextElementSibling);
// 下一个兄弟节点内容修改
// event.target.nextElementSibling.textContent = event.target.href;
console.log('+++++++++++++++++++++++++++++');
}
</script>
<!-- // 为事件属性绑定 事件方法 VUE -->
<script>
Vue.createApp({
// 实例数据
data() {
return {
// 插值变量
url1: null,
};
},
// 事件方法
methods: {
showUrl1( ev){
ev.preventDefault();
console.log(ev);
console.log(ev.target.href);
console.log(ev.currentTarget.href);
this.url1 = ev.currentTarget.href;
},
},
}).mount('.app');
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>事件绑定</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div class="app">
<p onclick=" console.log( '你好呀');">
<!-- <a href="https://www.baidu.com" onclick = "showURL( )">URL地址跳转 </a>
-->
<!-- 事件属性 v-on @ -->
<a href="https://www.php.cn" @click.prevent.stop="showUrl2($event)">显示地址三 : </a>
<span class="url2">{{url2}} </span>
</p>
</div>
<!-- // 为事件属性绑定 事件方法 VUE -->
<script>
Vue.createApp({
// 实例数据
data() {
return {
// 插值变量
url2: null,
};
},
// 事件方法
methods: {
showUrl2( ev){
// 其中 ev.preventDefault();和 ev.stopPropagation(); 可以简化 用修饰符简化, 如 @click.prevent.stop="showUrl2($event)"
// 去掉时间点击跳转行为
// ev.preventDefault();
// 去掉冒泡行为
// ev.stopPropagation();
console.log(ev);
console.log(ev.target.href);
console.log(ev.currentTarget.href);
this.url2 = ev.currentTarget.href;
},
},
}).mount('.app');
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>列表渲染(数组、关联数组)</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div class="app">
<!-- 数组 array -->
<p>{{cities}}</p>
<p>{{cities[1]}}</p>
<ul>
<li>{{cities[1]}}</li>
<li>{{cities[2]}}</li>
</ul>
<!-- fot of 循环数组
for(let item of array) {
}
-->
<!-- v-for :指令循环输出 -->
<ul>
<li v-for="city of cities">{{city}}</li>
</ul>
<ul>
<!-- index 是键 key ,可以激发diff算法 city是值value :key 等于 v-bind:key -->
<!-- 注意 of前后空格 -->
<li v-for="(city,index) of cities" :key="index">{{index}}:{{city}}</li>
</ul>
console.log('=====');
<p>{{cities}}</p>
<hr>
<!-- v-for 对象数组 -->
<ul>
<!-- prop属性 -->
<li v-for="(user,prop,index) of users" :key="index">{{index}}:{{user}}</li>
<li v-for="(user,prop,index) of users" :key="index">{{user.name}},{{user.email}}</li>
</ul>
<hr>
<ul>
<!-- 对象 -->
<li >{{user.name}}:{{user.email}}</li>
</ul>
</div>
<script>
const app = Vue.createApp({
data() {
return {
// 数组 array
cities:['1','2','3','4','5'],
// 对象 object 关联数组
user:{
name:'小狗',
email:'123@qq.com',
},
// 对象数组 array of object
users :[
{
name:'小猫',
email:'123@qq.com',
},
{
name:'小牛',
email:'123@qq.com',
},
],
}
},
}, ).mount('.app');
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>条件渲染v-if</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div class="app">
<p>{{message}}</p>
<p v-if = 'true'>{{message}},ture显示</p>
<p v-if = 'false'>{{message}},false不显示</p>
<p v-if = 'flag'>{{message}}</p>
<!-- 开关控制 -->
<!-- <button>隐藏</button> -->
<hr>
<button v-text = 'flag?"隐藏":"显示"'></button>
<br>
<button v-text = 'flag1?"隐藏":"显示"'></button>
<hr>
<p v-if = 'flag'>{{message}}</p>
<button @click = 'flag=!flag' v-text = 'flag1?"隐藏":"显示"'></button>
<hr>
<!-- if -else if else if else -->
<!-- <p v-if ='point'>{{grade[4]}}</p> -->
console.log('=======');<br>
<p v-if ='point>100 && point< 1000'>{{grade[0]}}</p>
<!-- console.log('=======');<br> 条件判断中间不能加其他的标签 -->
<p v-else-if='point>=2000 && point< 3000'>{{grade[1]}}</p>
<p v-else-if="point>=3000 && point< 4000">{{grade[2]}}</p>
<p v-else-if="point>=5000 && point< 6000">{{grade[3]}}</p>
<!-- <p v--else-if="point>=4000 && point< 5000">{{grade[3]}}</p> -->
<p v-else>{{grade[4]}}</p>
</div>
<script>
const app = Vue.createApp({
data() {
return {
message:'今天好热',
// true 显示 false 不显示 style = display: none
flag :true,
flag1 :false,
grade:['普通','银卡','金卡','白金','非会员'],
point:5100,
};
},
}).mount('.app');
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<body>
<div class="app">
<!-- <input type="text" @keydown = 'submit($event)'> -->
<!-- enter 键盘修饰符 回车-->
<input type="text" @keydown.enter = 'submit($event)'>
<ul>
<!-- :key 为了增加渲染的效率 -->
<li v-for="(item ,index) of list" :key="index">{{item}} </li>
</ul>
</div>
<script>
Vue.createApp({
data() {
return {
// 留言列表
list:[],
};
},
methods: {
submit(ev) {
console.log(ev);
if (ev.key === 'Enter') {
// this.list.unshift(ev.currentTarget.value);
// ev.currentTarget.value = null;
}
this.list.unshift(ev.currentTarget.value);
ev.currentTarget.value = null;
},
},
// methods: {
// submit:(ev){
// console.log(ev);
// if (ev.key =='Enter'){
// this.List.unshift(ev.currentTarget.value);
// }
// }
// },
}).mount('.app');
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>计算属性,侦听器属性</title>
<script src="https://unpkg.com/vue@next"></script>
</head>
<style>
table {
width: 20em;
height: 10em;
text-align: center;
border-collapse: collapse;
margin: 1em;
}
table caption {
font-size: 1.2em;
padding: 1em;
margin-bottom: 2em;
border-bottom: 1px solid;
}
tbody tr th:first-of-type {
background: linear-gradient(to left, lightcyan, #fff);
border-right: 1px solid;
}
body tbody tr:not(:last-of-type) > * {
border-bottom: 1px solid;
}
</style>
</head>
<body>
<div class="app">
<table>
<caption>
商品结算
</caption>
<tbody>
<tr>
<th>ID</th>
<td>HA110</td>
</tr>
<tr>
<th>品名</th>
<td>伊利纯牛奶</td>
</tr>
<tr>
<th>单价</th>
<td>100</td>
</tr>
<tr>
<th>数量</th>
<!-- v- modle 双向绑定 -->
<td><input type="number" v-model="num" style="width: 5em" /></td>
</tr>
<tr>
<th>金额</th>
<!-- <td>{{num * price}}</td> -->
<td>{{amount}}</td>
</tr>
</tbody>
</table>
<p style="font-size: 1.2em">
实付金额: {{realAmount}}
<br>
优惠了 :
<span style="color: red">{{difAmount}}</span>
</p>
</div>
<script>
const app = Vue.createApp({
data() {
return {
// 单价
price: 100,
// 数量
num: 0,
// 折扣
discount: 1,
// 实际金额
// realAmount:100,
// 优惠金额
difAmount:0,
};
},
// 计算器属性
computed:{
// 计算属性金额 = 单价乘以数量
// get amount(){
// },
amount:{
get(){
return this.price * this.num;
},
set (value){
},
},
},
// 侦听器属性
watch:{
// 访问器属性实现。看着是属性,实际是方法
// 被侦听的 属性其实一个方法 有两个参数组装,第一是 新值当前值 第二个值是原来的值,以前的旧值
amount( current,origin){
// console.log('当前'+ current ,'旧值'+origin);
// 根据当前金额确定打折
switch (true) {
// 1000-2000: 9折
case current >= 1000 && current < 2000:
this.discount = 0.9;
break;
// 2000 -> 3000 : 8折
case current >= 2000 && current < 3000:
this.discount = 0.8;
break;
// 3000 -> 4000 : 7折
case current >= 3000 && current < 4000:
this.discount = 0.7;
break;
// 4000 -> 5000 : 6折
case current >= 4000 && current < 5000:
this.discount = 0.6;
break;
// 5000 : 5折
case current >= 5000:
this.discount = 0.5;
}
// 实付金额 =原始金额*折扣率
// this.realAmount = this.amount * this.discount;
// 优惠金额(差价) =原始金额 -实付金额
// this.difAmount = this.amount - this.realAmount ;
// 实付金额 = 原始金额 * 折扣率
this.realAmount = this.amount * this.discount;
console.log(this.realAmount);
// 优惠金额(差价) = 原始金额 - 实付金额
this.difAmount = this.amount - this.realAmount;
},
},
// 实例的声明周期,当实例与挂载点绑定成功时,自动执行
mounted(){
this.num =1;
}
// 挂载
}).mount('.app');
</script>
</body>
</html>