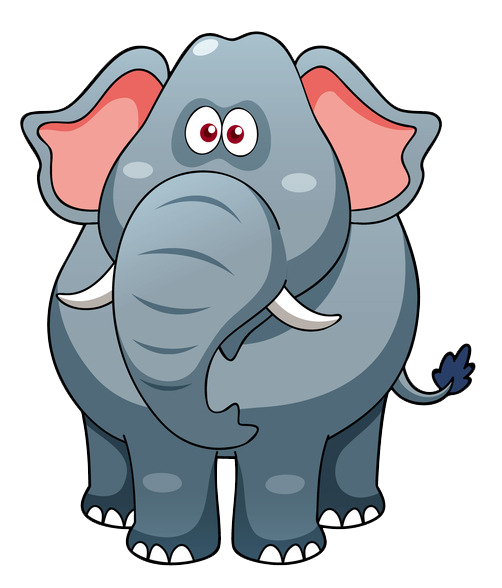
Correction status:qualified
Teacher's comments:
关键字extends
<?php
namespace _0815;
/**
* 学生类
*/
class Student
{
//公共属性 类中、类外、子类皆可以使用
public int $age;
//受保护的 类中、子类中可以使用
protected string $name;
//私有属性 只能在类中使用
private int $id = 101;
// 构造方法
public function __construct($name)
{
$this->name = $name;
}
//自定义方法 受保护的 只能在子类中使用扩展
protected function getInfo(): string
{
return '父类私有属性=>id: ' . $this->id . ',<br>父类公共属性=> 姓名: ' . $this->name;
}
}
/**
* 小学生类 继承 学生类
* 对学生类进行扩展
*/
class PrimarySchool extends Student
{
// 属性扩展
private string $lesson;
private int $score;
// 构造方法扩展
public function __construct($name, $lesson, $score)
{
// parent: 引用父类的构造方法
parent::__construct($name);
$this->lesson = $lesson;
$this->score = $score;
}
// 子类中对自定义方法扩展
// 若是父类的关键字是protected,那么子类关键字只能时public和protected
// 若是父类的关键字是public,那么子类关键字只能是public
public function getInfo(): string
{
return parent::getInfo() . ', <br> 子类扩展=> 课程: ' . $this->lesson . ', 成绩: ' . $this->score;
}
}
$primarySchool = new PrimarySchool('张国', '数学', 90);
echo $primarySchool->getInfo();
关键字abstract
<?php
namespace _0815;
/**
*
* 声明抽象类:abstract
* 只要是抽象类,其父类中的属性和方法不能直接访问,必须通过子类扩展后访问
* 如果类中有抽象方法。则这个类必须声明为抽象类
*/
abstract class Demo1
{
public string $name;
// 如果某个方法没有具体实现(只声明未实现),应该声明成抽象方法
abstract public static function getInfo($name);
}
/**
* 若父类时抽象类,子类扩展时必须将父类的抽象方法实现,否则报错
*/
class Demo2 extends Demo1
{
// 实现父类抽象类的方法
public static function getInfo($name)
{
return 'Hello, ' . $name;
}
}
// 输出结果 Hello,张三
echo Demo2::getInfo('张三');
关键字final
只要声明为final
的类,是不可以继承扩展的
final class Demo3
{
//代码块
}
关键字interface
接口命名规则:小写的i
开头加类名,如:iUser
iStu
关键字implements
实现接口
<?php
namespace _0815;
//接口
interface iUserInfo
{
//常量
public const NATION = 'China';
//方法 没有具体实现
public function getInfo();
public function m2();
}
// 接口实现类
class UserInfo implements iUserInfo
{
public string $name = '张三';
//必须实现接口类中的所有方法
public function getInfo():string
{
return 'Hello, ' . $this->name;
}
public function m2()
{
}
}
$userInfo = new UserInfo();
// 输出结果 Hello,张三
echo $userInfo->getInfo();
全局成员包含
函数
常量
类/接口
特点:全局可使用,禁止重复声明
命名空间的作用
是为了解决全局变量命名冲突的问题
类似同一个目录不允许同名文件存在,但是可以通过新建目录的方式来解决声明方式
关键字:namespace 命名空间名称
跨空间成员的访问方式
<?php
//命名空间:_0815_one
namespace _0815_one;
function getInfo(): string
{
return __METHOD__ . '<br>';
}
// 1. 当前路径: 非限定名称, 访问当前空间内的方法: getInfo()
echo '当前路径: ' . getInfo();
// 跨空间请求
// 2. 相对路径: 限定名称, 跨空间访问:two\Index::getInfo()
echo '跨空间访问-相对路径: ' . two\Index::getInfo();
// 3. 绝对路径: 完全限定名称, 跨空间访问: \_0815_one\two\Index::getInfo()
echo '跨空间访问-绝对路径: ' . \_0815_one\two\Index::getInfo();
// 4. 别名访问
use \_0815_one\two\Index as indexInfo;
echo '跨空间访问-别名访问: ' . indexInfo::getInfo();
//命名空间:_0815_one\two
namespace _0815_one\two;
class Index
{
public static function getInfo(): string
{
return __METHOD__ . '<br>';
}
}
echo Index::getInfo();