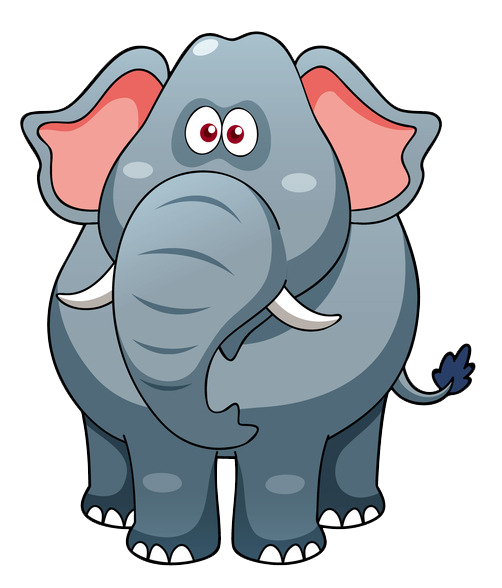
Correction status:qualified
Teacher's comments:
namespace pdo_edu;
use PDO;
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// 匿名参数: ?
$sql = 'INSERT `staff` SET `name`= ?,`sex`= ?;';
$stmt = $db->prepare($sql);
// 索引数组
$data = ['陈梦', 1];
// 执行sql
$stmt->execute($data);
// 验证: 打印sql预处理命令
echo '新增成功, id = ' . $db->lastInsertId() . '<br>';
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// 命名参数: ":name"
$sql = 'INSERT `staff` SET `name`= :name,`sex`= :sex;'
$stmt = $db->prepare($sql);
// 关联数组
$data = ['name'=>'孙颖莎', 'sex'=>1];
// 执行sql
$stmt->execute($data);
// 验证: 打印sql预处理命令
echo '新增成功, id = ' . $db->lastInsertId() . '<br>';
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// CURD: INSERT
$sql = 'INSERT `staff` SET `name`= ?,`sex`= ?;';
$stmt = $db->prepare($sql);
// 引用绑定: 动态绑定,绑定的不是数据本身,而它的地址/引用/别
名
$stmt->bindParam(1, $name, PDO::PARAM_STR);
$stmt->bindParam(2, $sex, PDO::PARAM_INT);
list($name, $sex, $email)=['王曼昱', 1];
// 执行sql
$stmt->execute();
echo '新增成功, id = ' . $db->lastInsertId() . '<br>';
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// CURD: INSERT
$sql = 'INSERT `staff` SET `name`= ?,`sex`= ?;';
$stmt = $db->prepare($sql);
// 引用绑定
$stmt->bindParam(1, $name, PDO::PARAM_STR);
$stmt->bindParam(2, $sex, PDO::PARAM_INT);
// 二维数组
$data = [
['樊振东',0],
['马龙',0],
['徐昕',0],
];
foreach ($data as list($name, $sex)) {
// 执行sql
$stmt->execute();
echo '新增成功, id = ' . $db->lastInsertId() .
'<br>';
}
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// CURD: INSERT
$sql = 'INSERT `staff` SET `name`= ?,`sex`= ?;';
$stmt = $db->prepare($sql);
$data = ['刘翔', 0];
// 执行sql
if ($stmt->execute($data)) {
if ($stmt->rowCount() > 0) {
// success
echo '新增成功, id = ' . $db->lastInsertId() .
'<br>';
} else {
// fail
echo '新增失败';
print_r($stmt->errorInfo());
}
} else {
// false
echo 'sql执行失败';
print_r($stmt->errorInfo());
}
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// CURD: UPDATE
$sql = <<< SQL
UPDATE `staff`
SET `name`= ?,`sex`= ?,
WHERE `id` = ? ;
SQL;
if (false === stripos($sql, 'where')) {
exit('禁止无条件更新');
}
$stmt = $db->prepare($sql);
$data = ['苏炳添', 0, 10];
// 执行sql
if ($stmt->execute($data)) {
if ($stmt->rowCount() > 0) {
echo '成功的更新了 '.$stmt->rowCount() . '条记录
~~';
} else {
echo '没有记录被更新';
print_r($stmt->errorInfo());
}
} else {
echo 'sql执行失败';
print_r($stmt->errorInfo());
}
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// CURD: DELETE
$sql = 'DELETE FROM `staff` WHERE `id` = ?;';
if (false === stripos($sql, 'where')) {
exit('禁止无条件删除');
}
$stmt = $db->prepare($sql);
// 执行sql
if ($stmt->execute([9])) {
if ($stmt->rowCount() > 0) {
echo '成功的删除了 '.$stmt->rowCount() . ' 条记录
~~';
} else {
echo '没有记录被删除';
print_r($stmt->errorInfo());
}
} else {
echo 'sql执行失败';
print_r($stmt->errorInfo());
// $stmt->debugDumpParams();
}
namespace pdo_edu;
use PDO;
// 连接
$db = new PDO('mysql:dbname=phpedu', 'root', 'root');
// CURD: SELECT
$sql = 'SELECT `id`,`name` FROM `staff`LIMIT ?';
$stmt = $db->prepare($sql);
$stmt->bindValue(1, 3, PDO::PARAM_INT);
// 执行sql
if ($stmt->execute()) {
while ($staff = $stmt->fetch(PDO::FETCH_ASSOC))
{
printf('<pre>%s</pre>', print_r($staff, true));
}
} else {
echo 'sql执行失败';
print_r($stmt->errorInfo());
}