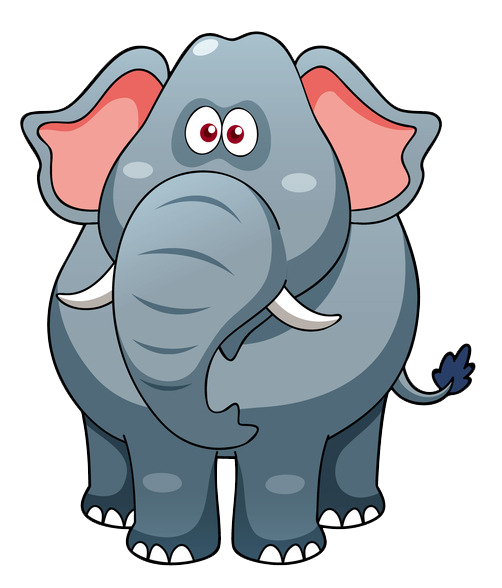
Correction status:qualified
Teacher's comments:
JOIN
: 等同于 JOIN(默认的JOIN类型),如果表中有至少一个匹配,则返回行LEFT JOIN
: 即使右表中没有匹配,也从左表返回所有的行RIGHT JOIN
: 即使左表中没有匹配,也从右表返回所有的行
// 匹配所有满足条件的数据
$ret = Db::table('user u')->join('order o', 'u.id=o.uid')->select();
print_r($ret);
// 以左表作为主表,查询右表中满足条件的数据 不满足则返回的字段的值为null
$ret = Db::table('user u')->leftjoin('order o', 'u.id=o.uid')->select();
print_r($ret);
// 以右表作为主表,查询左表中满足条件的数据 不满足则返回的字段的值为null
$ret = Db::table('user u')->rightjoin('order o', 'u.id=o.uid')->select();
print_r($ret);
database.php
中配置了数据库连接信息:config/database.php 文件里设置了表前缀prefix
,模型类的命名规则是除去表前缀的数据表名称,采用驼峰法命名,并且首字母大写;反之模型的命名规则不去表前缀,同样采用驼峰法命名,并且首字母大写,且要去掉下划线app
目录下新建model
目录,并在model
目录下建立User.php
文件,User
对应数据库中的user
表User.php
<?php
namespace app\model;
use think\Model;
class User extends Model
{
// 方法
}
model目录下User.php
模型
<?php
// 命名空间与当前模型路径保持一致
namespace app\model;
// 引入think框架中的Model
use think\Model;
// 模型名称与文件名称保持一致
class User extends Model
{
// 设置当前模型的数据库连接
// 若查询其他数据库可做更改,
// 因为User模型下默认表名为user表,所以要注意该库中是否有user表 若无则需更改表名称
protected $connection = 'test';
// 设置表名称
// User模型下默认表名为user表 若查询其他表可在此更改
protected $name = 'order';
// 改变主键的名称
protected $pk = 'id';
// 设置废弃字段 数组
// 废弃数据表中'phone'和'last_time'字段 ,在进行查询的时候不返回这两个字段
protected $disuse = [
'phone',
'last_time'
];
// 设置模型对应数据表字段及类型 数组
// 数据类型转换
protected $type = [
'id' => 'int',
'password' => 'string',
'address' => 'string'
];
// 查询所有数据,此方法在controller控制器啊中实例化调用
public function lists()
{
// 查询user表中的所有数据
// 这里的User对应数据库中的user表 且User就是模型的名称
return User::select()->toArray();
}
// 根据主键查询数据且数据表中主键名称必须为‘id’
// 若是数据表中的主键名称不是id,可以通过$pk来设置
public function findId($id)
{
// 根据主键id查询数据
return User::find($id);
}
// 获取器 对接收的参数进行处理
// 格式:get + 数据表中某一字段 + Attr
// 接收一个参数$v 当前字段’status‘的值
public function getStatusAttr($v)
{
return $v ? '关闭' : '开启';
}
// 获取器 将查询的性别sex初始化
public function getSexAttr($v)
{
return $v ? '男' : '女';
}
// 修改器 在数据添加到数据库之前对数据进行处理
// 在create方法时触发
// 格式: set + 数据表中某一字段 + Attr
// 接收两个参数,$v:当前字段的值,$all:所有字段的值
public function setPasswordAttr($v, $all)
{
//打印所有数据
print_r($all);
// 对psssword字段的值进行加密处理
return md5($v);
}
// 添加数据
public function insertOne($data)
{
//添加数据 并将添加数据的结果返回
return User::create($data);
}
}
controller目录下user.php
关键代码
// 实例化模型
$users = new User();
// 得到user表的所有数据
$ret = $users->lists();
print_r($ret);
// 根据id得到数据
$ret = $users->findId(1);
print_r($ret);
// 数据
$data = [
'name' => '小米粒',
'password' => 123456,
'phone'=>'12345678789',
'sex' => 0,
'email' => 'xiaomili@php.cn',
'address'=>'贵阳市观山湖区',
'status' => 0,
'add_time' => 1661737536
];
// 添加数据
$ret = $users->insertOne($data);
print_r($ret);