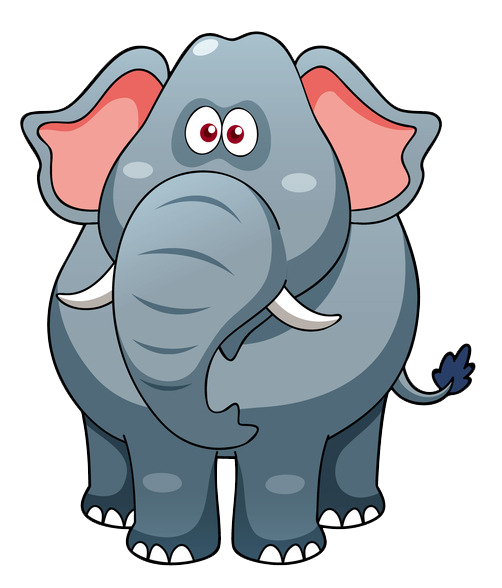
Correction status:qualified
Teacher's comments:
上传文件设置两个属性
<?php
printf('<pre>%s</pre>',print_r($_FILES,true));
if(isset($_FILES['my_pic'])){
//原始文件名
$name = $_FILES['my_pic']['name'];
// 临时文件名
$tmpName = $_FILES['my_pic']['tmp_name'];
// 错误代码
$error = $_FILES['my_pic']['error'];
if($error > 0){
$tips = '<span style="color:red">上传失败!</span><br>';
switch($error){
case 1:
$tips .= '超过php.ini允许上传的大小!';
break;
case 2:
$tips .= '超过表单中MAX_FILE_SIZE允许上传的大小!';
break;
case 3:
$tips .= '文件只有部分被上传!';
break;
case 4:
$tips .= '没有文件被上传!';
break;
case 6:
$tips .= '找不到临时目录!';
break;
case 7:
$tips .= '文件写入失败,请检查目录权限!';
break;
}
echo "<p>$tips</p>";
}else{
// echo '上传成功!';
// 判断上传方式是否合法?
if(is_uploaded_file($tmpName)){
// 文件类型白名单
$allow = ['jpg', 'jpeg', 'png', 'gif'];
// 获取扩展名
$ext = pathinfo($name)['extension'];
if(in_array($ext, $allow)){
// 类型合法,设置指定目录
$path = 'uploads/';
// 重命名,防止重名。
$dest = $path.md5($tmpName).'.'.$ext;
// 移动到指定目录
if(move_uploaded_file($tmpName, $dest)){
echo '上传成功!';
echo "<img src='$dest' width='50'>";
}else{
echo '上传失败!';
}
}else{
echo '<p>不允许的类型!</p>';
}
}else{
echo '<p>非法方式上传!</p>';
}
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="" method="POST" enctype="multipart/form-data">
<fieldset>
<legend>单文件上传</legend>
<!-- name="my_pic":给服务器段语言用的变量,PHP可以用$FILE来获取。 -->
<!-- <input type="hidden" name="MAX_FILE_SIZE" value="8"> -->
<input type="file" name="my_pic">
<button>上传</button>
</fieldset>
</form>
</body>
</html>
方式1
<?php
printf('<pre>%s</pre>',print_r($_FILES,true));
foreach($_FILES as $file){
if($file['error'] === 0){
$dest = 'uploads/'. $file['name'];
move_uploaded_file($file['tmp_name'], $dest);
echo "<img src='$dest' width='150'>";
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="" method="POST" enctype="multipart/form-data">
<fieldset>
<legend>多文件上传-1:逐个上传</legend>
<input type="file" name="my_pic1">
<input type="file" name="my_pic2">
<input type="file" name="my_pic3">
<button>上传</button>
</fieldset>
</form>
</body>
</html>
方式2
<?php
printf('<pre>%s</pre>',print_r($_FILES,true));
if(isset($_FILES['my_pic'])){
foreach($_FILES['my_pic']['error'] as $key=>$error){
// php5.3以后支持UPLOAD_ERR_OK常量
if($error === UPLOAD_ERR_OK){
// 临时文件名
$tmpName = $_FILES['my_pic']['tmp_name'][$key];
// 原始文件名
$name = $_FILES['my_pic']['name'][$key];
$dest = 'uploads/'. $name;
move_uploaded_file($tmpName, $dest);
echo "<img src='$dest' width='150'>";
}
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="" method="POST" enctype="multipart/form-data">
<fieldset>
<legend>多文件上传-2</legend>
<input type="file" name="my_pic[]">
<input type="file" name="my_pic[]">
<input type="file" name="my_pic[]">
<button>上传</button>
</fieldset>
</form>
</body>
</html>
<?php
printf('<pre>%s</pre>',print_r($_FILES,true));
if(isset($_FILES['my_pic'])){
foreach($_FILES['my_pic']['error'] as $key=>$error){
// php5.3以后支持UPLOAD_ERR_OK常量
if($error === UPLOAD_ERR_OK){
// 临时文件名
$tmpName = $_FILES['my_pic']['tmp_name'][$key];
// 原始文件名
$name = $_FILES['my_pic']['name'][$key];
$dest = 'uploads/'. $name;
move_uploaded_file($tmpName, $dest);
echo "<img src='$dest' width='150'>";
}
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="" method="POST" enctype="multipart/form-data">
<fieldset>
<legend>批量上传</legend>
<!-- 与逐个上传方式2相比,只保留一行下列代码,最后还要增加multiple属性。 -->
<input type="file" name="my_pic[]" multiple>
<button>上传</button>
</fieldset>
</form>
</body>
</html>