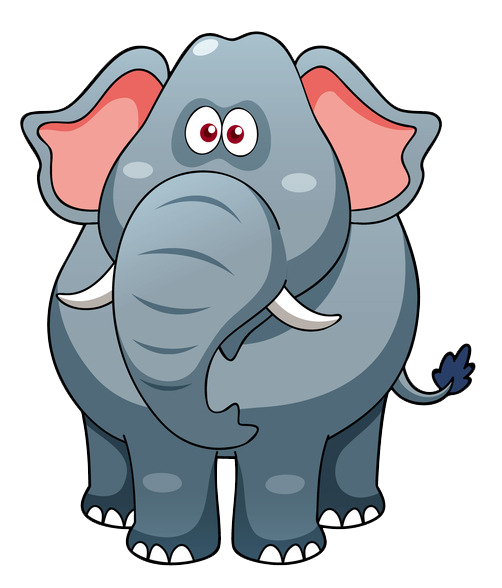
Correction status:qualified
Teacher's comments:完成的很好, 没什么问题, 继续加油
HTML
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>仿淘宝移动端轮播图</title>
<link rel="stylesheet" href="static/css/slideshow.css" />
</head>
<body>
<!-- 轮播图容器 -->
<div class="slideshow">
<!-- 图片容器 -->
<div class="imgs"></div>
<!-- 按钮容器 -->
<div class="btns"></div>
</div>
<!-- 模块化开发,添加type="module" -->
<script type="module">
//获取图片容器
const imgs = document.querySelector(".imgs");
//获取按钮容器
const btns = document.querySelector(".btns");
//导入模块
import {
createImgs,
createBtns,
switchImg,
timePlay,
} from "./static/js/slideshow.js";
//页面加载完成后的动作window.onload
window.onload = function () {
//执行模块的createImgs(创建图片组)
createImgs(imgs);
//执行模块的createBtns
createBtns(imgs, btns);
//创建按钮事件,点击按钮执行的动作(显示对应图片)
//将类数组转为真数组然后用forEach循环遍历
//forEach的参数是一个函数,函数中有三个参数分别是值(必选),索引,数组本身
/*[...btns.children].forEach(function (btn) {
btn.onclick = function () {
switchImg(this, imgs);
};
});*/
Array.from(btns.children).forEach(function (btn) {
btn.onclick = function () {
//被点击时执行模块的switchImg
switchImg(this, imgs);
};
});
//添加定时器,实现图片轮播
//按钮数组
const btnArr = [...btns.children];
//按钮索引
const btnKeys = Object.keys(btns.children);
//console.log(btnKeys);
//定时器setInterval()
setInterval(play, 2000, btnArr, btnKeys);
function play(btnArr, btnKeys) {
//执行模块的timePlay
timePlay(btnArr, btnKeys);
}
};
</script>
</body>
</html>
JavaScript
// 轮播图模块
//图片组数据
const imgArr = [
{
key: 1,
src: "static/images/item1.jpeg",
url: "http://php.cn",
},
{
key: 2,
src: "static/images/item2.jpeg",
url: "http://php.cn",
},
{
key: 3,
src: "static/images/item3.jpeg",
url: "http://php.cn",
},
];
//创建图片组
function createImgs(imgs) {
//图片资源较大,用文档片段来做
const frag = new DocumentFragment();
//for循环获取imgArr全部图片资源
for (let i = 0; i < imgArr.length; i++) {
// 创建图片元素
const img = new Image();
//添加img的src
img.src = imgArr[i].src;
//添加自定义属性data-key
img.dataset.key = imgArr[i].key;
//第一张图片添加class='active'
if (i === 0) img.classList.add("active");
//添加图片点击事件
img.onclick = function () {
location.href = imgArr[i].url;
};
//img.onclick = () => (location.href = imgArr[i].url);
//将图片添加到临时文档片段frag
frag.append(img);
}
//将文档片段添加到图片容器imgs
imgs.append(frag);
}
//创建按钮组,按钮数量根据图片数量得来
function createBtns(imgs, btns) {
//获取图片容器中的图片数量childElementCount
const length = imgs.childElementCount;
for (let i = 0; i < length; i++) {
//创建按钮元素span
const btn = document.createElement("span");
//添加自定义元素data-key
btn.dataset.key = imgs.children[i].dataset.key;
//第一个按钮添加class='active'
if (i === 0) btn.classList.add("active");
//将按钮元素添加到按钮容器btns
btns.append(btn);
}
}
//按钮点击事件
function switchImg(btn, imgs) {
//按钮被点击时去除默认的激活状态class='active'
//由于激活状态的按钮并不是固定的,所以进行遍历去除
Array.from(btn.parentNode.children).forEach(function (btn) {
btn.classList.remove("active");
});
/*[...btn.parentNode.children].forEach(function(btn){
btn.classList.remove('active');
});*/
/*[...btn.parentElement.children].forEach(function(btn){
btn.classList.remove('active');
});*/
[...imgs.children].forEach(function (img) {
img.classList.remove("active");
});
//修改当前按钮处于激活状态,添加class='active'
btn.classList.add("active");
//查找索引与当前按钮索引一致的图片元素
const currImg = [...imgs.children].find(function (img) {
return img.dataset.key == btn.dataset.key;
});
//修改图片元素处于激活状态
currImg.classList.add("active");
}
//定时播放
//将按钮索引从第一个开始 取出、进行点击事件、添加到尾部实现首尾相连循环播放
// 0,1,2
// 1,2,0
// 2,0,1
// 0,1,2
function timePlay(btnArr, btnKeys) {
//shift()删除数组中的第一个元素并返回该值
let key = btnKeys.shift();
//dispatchEvent()对取出值按钮进行点击事件
btnArr[key].dispatchEvent(new Event("click"));
//push()将该取出值添加到数组尾部
btnKeys.push(key);
}
//导出
export { createImgs, createBtns, switchImg, timePlay };
CSS
/* 添加背景色 */
body {
background-color: #eee;
}
/* 轮播图容器 */
.slideshow {
width: 240px;
height: 360px;
}
/* 图片容器 */
.slideshow .imgs {
/* inherit父元素的值 */
width: inherit;
height: inherit;
}
/* 图片适应 */
.slideshow img {
width: 100%;
height: 100%;
/* border-radius设置圆角 */
border-radius: 10px;
/* 图片默认状态-隐藏 */
display: none;
}
/* 图片激活状态 */
.slideshow img.active {
display: block;
}
/* 鼠标悬停图片时小手样式 */
.slideshow img:hover {
cursor: pointer;
}
/* 按钮容器 */
.slideshow .btns {
/* flex布局 */
display: flex;
/* 居中 */
place-content: center;
/* transform 属性允许你旋转,缩放,倾斜或平移给定元素。 */
transform: translateY(-40px);
}
.slideshow .btns > span {
/* rgba(,,,,)颜色和透明度 */
background-color: rgba(233, 233, 233, 0.5);
height: 16px;
width: 16px;
/* 按钮圆形 */
border-radius: 50%;
/* 按钮间距 */
margin: 5px;
}
.slideshow .btns > span.active {
background-color: orangered;
}
.slideshow .btns > span:hover {
cursor: pointer;
}
效果图