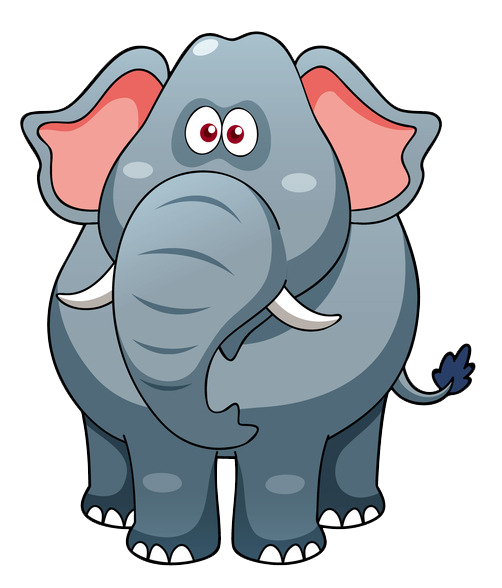
Correction status:qualified
Teacher's comments:总结的很好, 继续加油
选项卡效果图如下:
基于JS的选项卡的实现,基本上由JS的模块实现。
html搭建好基本框架,内容有JS动态填充。
<div class="box">
/* 选项卡选项*/
<div class="menu"></div>
/*选项卡内容*/
<div class="content"></div>
</div>
样式中最主要的时.hidden 和.active。用来切换内容的显示状态:显示或者不显示。
<style>
/* 目录选中状态时的样式 */
.box .menu .active {
display:block;
background-color: green;
}
/* 内容选中状态时的样式 */
.box .content .active{
background-color: cornsilk;
}
/* 隐藏样式 */
.hidden{
display: none;
}
/* 目录,flex布局是为了盒子里的内容横排 */
.menu{
display: flex;
}
/* 目录里的按钮为圆角样式 */
.menu button{
border-radius: 10px;
margin-left: 5px;
}
.box .content ul{
list-style: none;
margin-left: -30px;
}
.box .content ul li:nth-of-type(2n){
background-color: rgb(224, 220, 228);
}
</style>
<!-- 导入模块时,script的type必须是module -->
<script type="module">
// 导入模块,注意,用了别名,所以后续引用模块中的到处项时,必须使用.的形式
import * as tab from "./content.js"; //注意模块的路径和别名的使用
// 获取选项标题
const menu = document.querySelector('.menu');
// 获取每个选项下对应的内容
const content = document.querySelector('.content');
// 加载选项标题和选项内容
window.onload = ()=>tab.createTab(menu,content);
// 点击选项卡目录时,改变选中卡的背景色并切换选项卡内容,第二个参数是为了简化模块中的代码
menu.onclick = (ev)=> tab.changeItems(menu,ev.target);
</script>
js模块包括动态建立DOM元素所需要的数据和函数。主要有选项卡的栏目及其对应的内容
// 选项卡目录
const menu =[
{cid:1,content:"menu_1"},
{cid:2,content:"menu_2"},
{cid:3,content:"menu_3"}
];
// 项目卡内容
// 在visual studio code编辑器里,摁住alt后,鼠标左键单击多行中的某片段,可以更改多个地方。
const neirong = [
{key:1,cid:1,ncontent:[{url:"https://www.jacgoo.com",content:"试验测试网络1"},{url:"https://www.jacgoo.com",content:"环宇测试网1"},{url:"https://www.php.cn",content:"php中文网1"}]},
{key:2,cid:2,ncontent:[{url:"https://www.jacgoo.com",content:"试验测试网络2"},{url:"https://www.jacgoo.com",content:"环宇测试网2"},{url:"https://www.php.cn",content:"php中文网2"}]},
{key:3,cid:3,ncontent:[{url:"https://www.jacgoo.com",content:"试验测试网络3"},{url:"https://www.jacgoo.com",content:"环宇测试网3"},{url:"https://www.php.cn",content:"php中文网3"}]}
]
function createTab(type,card){
// 创建项目目录
for(let i = 0 ; i < menu.length;i++){
const btn = document.createElement('button');
// 添加按钮的文本内容
btn.textContent = menu[i].content;
// 添加按钮的data-key属性
btn.dataset.key = menu[i].cid;
// 将第一个按钮家加上active样式
if(i === 0 ) btn.classList.add('active');
type.append(btn);
}
//创建内容
//1.创建ul
for(let j = 0 ;j < neirong.length;j++){
const ul = document.createElement("ul");
//为ul添加data-key属性,便于改变目录时改变内容
ul.dataset.key = neirong[j].cid;
// 创建每个ul下的li和a
for(let k = 0 ;k < neirong[j].ncontent.length;k++){
const li = document.createElement('li');
const a = document.createElement('a');
a.href = neirong[j].ncontent[k].url;
a.textContent = neirong[j].ncontent[k].content;
li.append(a);
ul.append(li);
}
ul.classList.add(j === 0?'active':'hidden');
card.append(ul);
}
}
// 点击目录里的选项时,改变活动目录的背景色,同时改变内容
function changeItems(type,ev){
// 获取所点目录的key值
const key = ev.dataset.key;
console.log(type);
// 去掉目录里所有的active类
[...type.children].forEach(btn => btn.classList.remove('active'));
// 为目录里的key值等于常亮key的button加上active类
[...type.children].find(btn => btn.dataset.key === key).classList.add('active');
// 找到目录选项卡的近邻兄弟元素content
const con = type.nextElementSibling;
console.log(con.children);
// 去掉内容上的active类;
[...con.children].forEach(nr => nr.classList.replace('active','hidden'));
[...con.children].find(nr => nr.dataset.key === key).classList.replace('hidden','active');
}
export {createTab,changeItems};