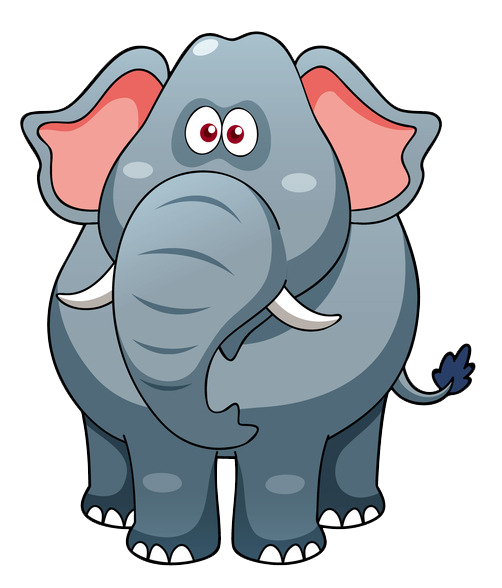
Correction status:qualified
Teacher's comments:
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>fetch的异步和同步以及Mock.js使用</title>
<style type="text/css">
*{box-sizing:border-box;margin:0;padding:0;}
body{background-color:#f2f2f2;font-size:16px;}
h1,h2,h3,h4{margin-bottom:15px;}
input[type=checkbox]+label,input[type=radio]+label{margin-right:10px;margin-left:5px;height:36px;line-height:36px;}
button{padding:0 18px;height:36px;border:1px solid transparent;border-radius:2px;background-color:#03a9f4;color:#fff;text-align:center;white-space:nowrap;font-size:14px;line-height:36px;cursor:pointer;}
button:hover{opacity:.8;}
pre{display:block;overflow:auto;margin:15px 0;padding:15px;border-radius:6px;background-color:#f5f5f5;color:#333;word-wrap:break-word;font-size:13px;line-height:1.4;word-break:break-all;}
#app{margin:30px auto;padding:20px;max-width:970px;background-color:#fff;}
.mock{position:relative;}
.mock>.refresh{position:absolute;right:0;bottom:0;border:none;border-radius:0 0 6px 0;background-color:#03a9f4;color:#fff;cursor:pointer;}
</style>
</head>
<body>
<div id="app">
<div class="mock">
<h2>Mock.js 演示</h2>
<pre></pre>
<button class="refresh" onclick="mockTest(this)">刷新</button>
</div>
<div class="fetch">
<h2>fetch API演示</h2>
<div class="btn-group">
<button onclick="fetchTest(this, 'GET')">发送GET请求</button>
<button onclick="fetchTest(this, 'POST')">异步发送POST请求</button>
<button onclick="fetch2Test(this, 'PUT')">同步发送PUT请求</button>
<button onclick="fetch2Test(this, 'PATCH')">同步发送PATCH请求</button>
<button onclick="fetch2Test(this, 'DELETE')">同步发送DELETE请求</button>
</div>
<pre></pre>
</div>
</div>
<script src="https://cdn.staticfile.org/Mock.js/1.0.0/mock-min.js" type="text/javascript" charset="utf-8">
</script>
<script type="text/javascript" charset="utf-8">
document.querySelector('.mock>.refresh').click();
// mock测试
function mockTest(ele) {
let mock = {
"id": Mock.mock('@integer(100000, 999999)'),
"name": Mock.mock('@cname'),
"ip": Mock.mock('@ip'),
"region": Mock.mock('@region'),
"province": Mock.mock('@province'),
"city": Mock.mock('@city')
}
ele.parentNode.querySelector('pre').innerHTML = JSON.stringify(mock, null, 2)
}
// 异步方式
function fetchTest(ele, method = 'GET') {
let obj = getMockData(method);
fetch(obj.url, obj.option).then(response => response.json()).then(json => {
ele.parentNode.parentNode.querySelector('pre').innerHTML = JSON.stringify(json, null, 2)
});
}
// 同步方式
async function fetch2Test(ele, method = 'GET') {
let obj = getMockData(method);
// 1. 等待结果再进行下一步操作,返回响应对象
const response = await fetch(obj.url, obj.option);
// 2. 将响应结果,转为json, json()
const result = await response.json();
ele.parentNode.parentNode.querySelector('pre').innerHTML = JSON.stringify(result, null, 2)
}
// 获取测试数据
function getMockData(method){
let url = '';
let option = {};
option.method = method;
option.headers = {
'Content-type': 'application/json; charset=UTF-8',
}
// GET方法没有 body参数, 其他请求必须要有body
switch (method) {
case 'GET':
url = 'https://jsonplaceholder.typicode.com/todos/' + Mock.mock('@integer(1, 200)');
break;
case 'POST':
url = 'https://jsonplaceholder.typicode.com/posts/';
option.body = JSON.stringify({
userId: Mock.mock('@integer(100000, 999999)'),
title: Mock.mock('@ctitle'),
body: Mock.mock('@cparagraph'),
})
break;
case 'PUT':
url = 'https://jsonplaceholder.typicode.com/posts/' + Mock.mock('@integer(1, 100)');
option.body = JSON.stringify({
userId: Mock.mock('@integer(100000, 999999)'),
title: Mock.mock('@ctitle'),
body: Mock.mock('@cparagraph'),
})
break;
case 'PATCH':
url = 'https://jsonplaceholder.typicode.com/posts/' + Mock.mock('@integer(1, 100)');
option.body = JSON.stringify({
title: Mock.mock('@ctitle'),
})
break;
case 'DELETE':
url = 'https://jsonplaceholder.typicode.com/posts/' + Mock.mock('@integer(1, 100)');
break;
}
return {url: url, option: option}
}
</script>
</body>
</html>