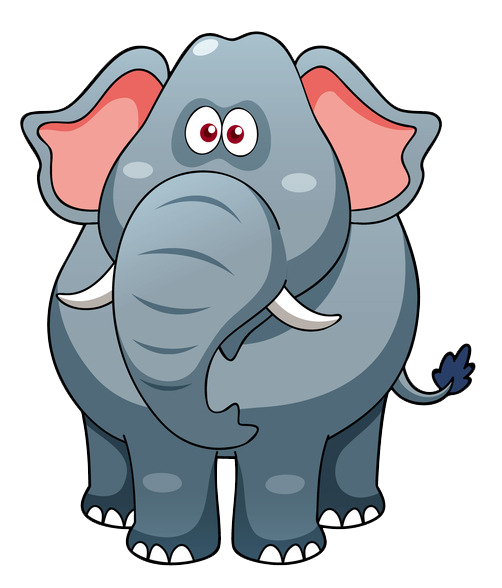
Correction status:qualified
Teacher's comments:完成的不错, 继续加油
1.实例演示购物车
<style>
.cart {
width: 460px;
display: grid;
gap: 10px;
}
table {
border-collapse: collapse;
text-align: center;
}
table caption {
font-size: 20px;
margin-bottom: 10px;
}
table input[type="number"] {
width: 40px;
}
table th,
table td {
border-bottom: thin solid #888;
padding: 5px;
}
table thead {
border-top: thin solid #888;
background-color: lightcyan;
}
table tfoot {
background-color: lightcyan;
}
table tbody tr:nth-child(odd):hover {
background-color: #eee;
cursor: pointer;
}
table tr td:first-child {
padding-left: 20px;
}
table tr td:last-child {
padding-right: 20px;
}
.cart .pay {
display: grid;
grid: 1fr / auto-flow;
place-content: end;
gap: 10px;
}
</style>
</head>
<body>
<div class="cart">
<table>
<caption>
我的购物车
</caption>
<thead>
<tr>
<td><input type="checkbox" name="" class="check-all" checked /></td>
<td>编号</td>
<td>品名</td>
<td>单位</td>
<td>单价</td>
<td>数量</td>
<td>金额(元)</td>
</tr>
</thead>
</table>
</div>
<script type="module">
// (一) 商品信息
const items = [
{ id: 286, name: "酸奶", units: "箱", price: 50, num: 1 },
{ id: 870, name: "苹果", units: "千克", price: 10, num: 1 },
{ id: 633, name: "外套", units: "件", price: 300, num: 1 },
{ id: 153, name: "皮鞋", units: "双", price: 400, num: 1 },
{ id: 109, name: "手机", units: "台", price: 5000, num: 1 },
];
// (二)导入购物车模块
import Cart from "./modules/cart.js";
//(三)实例化购物车类
const cart = new Cart(items);
// (四) 获取购物车(表格)
const table = document.querySelector("table");
// (五) 将商品渲染到购物车元素中 tbody
// 1 创建 tbody: 商品容器
const tbody = table.createTBody();
// 2. 创建tbody的内容,商品列表
items.forEach(function (item, key) {
// 1. 创建商品模板字符串
const tr = `
<tr>
<td><input type="checkbox" name="" class="check" checked /></td>
<td>${item.id}</td>
<td>${item.name}</td>
<td>${item.units}</td>
<td>${item.price}</td>
<td>
<input type="number" name="" value="${item.num}"
</td>
<td class="money">${cart.money[key]}</td>
</tr>
`;
// 2. 将内容填充到tbody
tbody.insertAdjacentHTML("beforeend", tr);
// tbody.innerHTML = tr;
});
// (六) 将相关统计数据(总数量,总金额),填充到tfoot中
const tfoot = table.createTFoot();
// 创建tfoot内容
let tr = `
<tr>
<td colspan="5">总计:</td>
<td class="total">${cart.total}</td>
<td class="total-money">${cart.totalMoney}</td>
</tr>
`;
tfoot.insertAdjacentHTML("beforeend", tr);
// (七) 更新数量,实时计算出结果并显示出来
// 1. 拿到所有的数量控件
const nums = table.querySelectorAll("input[type=number]");
// 2. 为每一个数量控件添加事件监听: input
nums.forEach(function (num, key) {
num.oninput = function () {
// 1. 计算总数量
items[key].num = num.value * 1;
cart.total = cart.getTotal(items);
// 2. 计算每个商品金额
cart.money[key] = num.value * 1 * items[key].price;
// 3. 计算商品总金额
cart.totalMoney = cart.money.reduce(function (acc, cur) {
return acc + cur;
});
// 4. 将数据渲染到指定元素上
table.querySelector(".total").textContent = cart.total;
table.querySelectorAll(".money")[key].textContent = cart.money[key];
table.querySelector(".total-money").textContent = cart.totalMoney;
};
});
// 满减促销活动表
const promotion = [
{ key: 1, money: 500, reduce: 50 },
{ key: 2, money: 800, reduce: 60 },
{ key: 3, money: 1000, reduce: 100 },
{ key: 4, money: 2000, reduce: 300 },
{ key: 5, money: 5000, reduce: 500 },
];
</script>
</body>
</html>
// 默认导出
// 购物车模块
export default class {
// 构造器
constructor(items) {
// 1. 商品总数量
this.total = this.getTotal(items);
// 2. 每个商品金额(数组)
this.money = this.getMoney(items);
// 3. 商品总金额
this.totalMoney = this.getTotalMoney();
}
// (一) 计算商品总数量
getTotal(items) {
// 1. 数量数组: 每个商品的数量num字段组成的数组
let numArr = items.map(function (item) {
return item.num;
});
// 2. 计算总数量
return numArr.reduce(function (acc, cur) {
return acc + cur;
});
}
// (二) 计算每个商品的金额
getMoney(items) {
// 金额 = 数量 * 单价
return items.map(function (item) {
return item.num * item.price;
});
}
// (三) 计算商品总金额
getTotalMoney() {
return this.money.reduce(function (acc, cur) {
return acc + cur;
});
}
}