


C# programming to obtain client computer hardware and system information function code case analysis
This article mainly introduces the function of C#Programming to obtain the client computer hardware and system information, which can realize the client system CPU, hard disk, motherboard and other hardware information and client Friends in need can refer to the operation skills of operating system, IP, MAC and other information.
This article describes the function of C# programming to obtain client computer hardware and system information. Share it with everyone for your reference, the details are as follows:
C# is used here to obtain the client computer hardware and system information, including CPU, hard disk, IP, MAC address, operating system, etc.
1. The project references the System.Management library.
2. Create the HardwareHandler.cs class file
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Management; namespace MyStudy.Utility { /// <summary> /// 计算机硬件处理类 /// </summary> public class HardwareHandler { public enum WMIPath { // 硬件 Win32_Processor, // CPU 处理器 Win32_PhysicalMemory, // 物理内存条 Win32_Keyboard, // 键盘 Win32_PointingDevice, // 点输入设备,包括鼠标。 Win32_FloppyDrive, // 软盘驱动器 Win32_DiskDrive, // 硬盘驱动器 Win32_CDROMDrive, // 光盘驱动器 Win32_BaseBoard, // 主板 Win32_BIOS, // BIOS 芯片 Win32_ParallelPort, // 并口 Win32_SerialPort, // 串口 Win32_SerialPortConfiguration, // 串口配置 Win32_SoundDevice, // 多媒体设置,一般指声卡。 Win32_SystemSlot, // 主板插槽 (ISA & PCI & AGP) Win32_USBController, // USB 控制器 Win32_NetworkAdapter, // 网络适配器 Win32_NetworkAdapterConfiguration, // 网络适配器设置 Win32_Printer, // 打印机 Win32_PrinterConfiguration, // 打印机设置 Win32_PrintJob, // 打印机任务 Win32_TCPIPPrinterPort, // 打印机端口 Win32_POTSModem, // MODEM Win32_POTSModemToSerialPort, // MODEM 端口 Win32_DesktopMonitor, // 显示器 Win32_DisplayConfiguration, // 显卡 Win32_DisplayControllerConfiguration, // 显卡设置 Win32_VideoController, // 显卡细节。 Win32_VideoSettings, // 显卡支持的显示模式。 // 操作系统 Win32_TimeZone, // 时区 Win32_SystemDriver, // 驱动程序 Win32_DiskPartition, // 磁盘分区 Win32_LogicalDisk, // 逻辑磁盘 Win32_LogicalDiskToPartition, // 逻辑磁盘所在分区及始末位置。 Win32_LogicalMemoryConfiguration, // 逻辑内存配置 Win32_PageFile, // 系统页文件信息 Win32_PageFileSetting, // 页文件设置 Win32_BootConfiguration, // 系统启动配置 Win32_ComputerSystem, // 计算机信息简要 Win32_OperatingSystem, // 操作系统信息 Win32_StartupCommand, // 系统自动启动程序 Win32_Service, // 系统安装的服务 Win32_Group, // 系统管理组 Win32_GroupUser, // 系统组帐号 Win32_UserAccount, // 用户帐号 Win32_Process, // 系统进程 Win32_Thread, // 系统线程 Win32_Share, // 共享 Win32_NetworkClient, // 已安装的网络客户端 Win32_NetworkProtocol, // 已安装的网络协议 } /// <summary> /// Cpu信息 /// </summary> /// <returns></returns> public void CpuInfo() { try { ManagementClass mc = new ManagementClass(WMIPath.Win32_Processor.ToString()); ManagementObjectCollection moc = mc.GetInstances(); foreach (ManagementObject mo in moc) { Console.WriteLine("CPU编号:" + mo.Properties["ProcessorId"].Value); Console.WriteLine("CPU型号:" + mo.Properties["Name"].Value); Console.WriteLine("CPU状态:" + mo.Properties["Status"].Value); Console.WriteLine("主机名称:" + mo.Properties["SystemName"].Value); } } catch { Console.WriteLine("Erroe"); } } /// <summary> /// 主板信息 /// </summary> public void MainBoardInfo() { try { ManagementClass mc = new ManagementClass(WMIPath.Win32_BaseBoard.ToString()); ManagementObjectCollection moc = mc.GetInstances(); foreach (ManagementObject mo in moc) { Console.WriteLine("主板ID:" + mo.Properties["SerialNumber"].Value); Console.WriteLine("制造商:" + mo.Properties["Manufacturer"].Value); Console.WriteLine("型号:" + mo.Properties["Product"].Value); Console.WriteLine("版本:" + mo.Properties["Version"].Value); } } catch { Console.WriteLine("Erroe"); } } /// <summary> /// 硬盘信息 /// </summary> public void DiskDriveInfo() { try { ManagementClass mc = new ManagementClass(WMIPath.Win32_DiskDrive.ToString()); ManagementObjectCollection moc = mc.GetInstances(); foreach (ManagementObject mo in moc) { Console.WriteLine("硬盘SN:" + mo.Properties["SerialNumber"].Value); Console.WriteLine("型号:" + mo.Properties["Model"].Value); Console.WriteLine("大小:" + Convert.ToDouble(mo.Properties["Size"].Value) / (1024 * 1024 * 1024)); } } catch { Console.WriteLine("Erroe"); } } /// <summary> /// 网络连接信息 /// </summary> public void NetworkInfo() { try { ManagementClass mc = new ManagementClass(WMIPath.Win32_NetworkAdapterConfiguration.ToString()); ManagementObjectCollection moc = mc.GetInstances(); foreach (ManagementObject mo in moc) { Console.WriteLine("MAC地址:" + mo.Properties["MACAddress"].Value); Console.WriteLine("IP地址:" + mo.Properties["IPAddress"].Value); } } catch { Console.WriteLine("Erroe"); } } /// <summary> /// 操作系统信息 /// </summary> public void OsInfo() { try { ManagementClass mc = new ManagementClass(WMIPath.Win32_OperatingSystem.ToString()); ManagementObjectCollection moc = mc.GetInstances(); foreach (ManagementObject mo in moc) { Console.WriteLine("操作系统:" + mo.Properties["Name"].Value); Console.WriteLine("版本:" + mo.Properties["Version"].Value); Console.WriteLine("系统目录:" + mo.Properties["SystemDirectory"].Value); } } catch { Console.WriteLine("Erroe"); } } } }
The above is the detailed content of C# programming to obtain client computer hardware and system information function code case analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










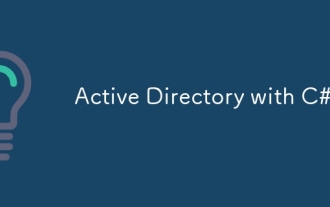
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
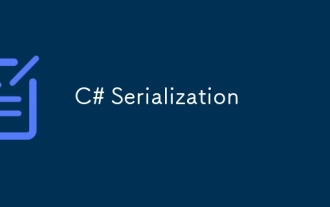
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
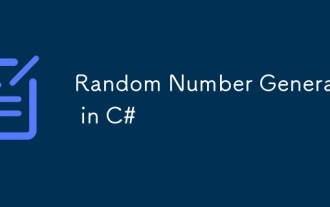
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
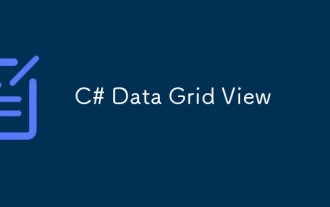
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
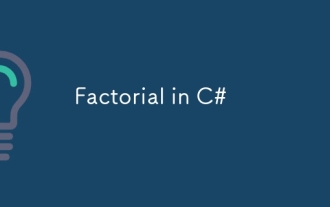
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
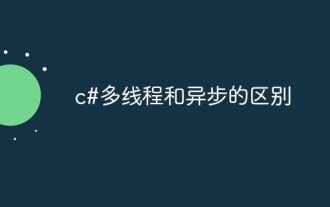
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
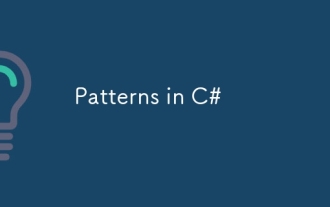
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
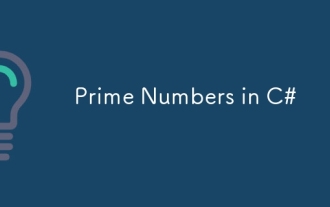
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
