
-
All
-
web3.0
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Backend Development
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Web Front-end
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Database
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Operation and Maintenance
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Development Tools
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
PHP Framework
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Common Problem
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Other
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Tech
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
CMS Tutorial
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Java
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
System Tutorial
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Computer Tutorials
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Hardware Tutorial
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Mobile Tutorial
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Software Tutorial
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
-
Mobile Game Tutorial
-
PHP Tutorial
-
Python Tutorial
-
Golang
-
XML/RSS Tutorial
-
C#.Net Tutorial
-
C++
-
RabbitMQ
-
ruby language
-
rust language
-
Flask framework
-
Django framework
-
Tomcat server
-
Spring framework
-
Spring Boot
-
restful
-
node.js
-
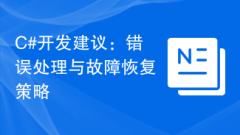
C# Development Advice: Error Handling and Failure Recovery Strategies
C# development suggestions: Error handling and fault recovery strategies In the C# development process, correctly handling errors and formulating effective fault recovery strategies are important aspects to ensure the robustness and stability of the program. Although we strive to write high-quality code, errors and glitches are inevitable. Therefore, this article will introduce several suggestions on error handling and failure recovery strategies to help developers better handle abnormal situations and ensure system stability and reliability. Exception handling In C#, an exception is an error condition that may occur during program running. by capturing
Nov 22, 2023 am 08:16 AM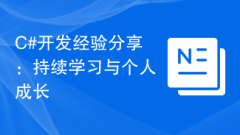
C# development experience sharing: continuous learning and personal growth
With the development of the times and the advancement of technology, the software development industry is growing day by day, and various programming languages are emerging in endlessly. As a C# developer, I would like to share some of my experiences and insights in C# development, hoping to be helpful to other developers. First of all, as a C# developer, continuous learning is very important. The C# language itself is constantly evolving, and Microsoft continues to update and improve the features and functions of the language. Therefore, we developers must always maintain a learning attitude, follow the latest technology development trends, and master the latest C# language features.
Nov 22, 2023 am 08:13 AM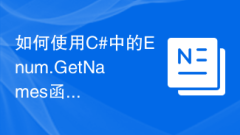
How to get all defined names in an enum type using Enum.GetNames function in C#
In C#, the enumeration type is a very useful data type that allows us to define some constants to represent certain states or options. Once the enum type is defined, you can use the Enum.GetNames function to get all defined names. This article details how to use this function and provides specific code examples. What is the Enum.GetNames function The Enum.GetNames function is a static method that returns an array of strings of the specified enumeration type, where each string represents the
Nov 18, 2023 pm 03:44 PM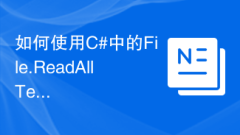
How to read text file contents using File.ReadAllText function in C#
How to use the File.ReadAllText function in C# to read the contents of a text file. In C# programming, we often need to read the contents of a text file. File.ReadAllText is a very convenient function that can help us quickly read the entire contents of a text file. This article will introduce how to use the File.ReadAllText function and provide specific code examples. First, we need to introduce the System.IO namespace in order to use the related methods of the File class.
Nov 18, 2023 pm 03:23 PM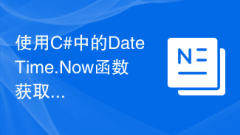
Get the current time using DateTime.Now function in C#
Use the DateTime.Now function in C# to get the current time. In C# programming, we often need to get the current time. The DateTime.Now function is provided in C# to obtain the current system time. Below I will introduce to you how to use the DateTime.Now function and give specific code examples. The DateTime.Now function returns a DateTime object representing the current date and time. Its usage is very simple, just call the function. Below is one
Nov 18, 2023 pm 03:14 PM
Use the DateTime.AddDays function in C# to add a specified number of days to a date
Use the DateTime.AddDays function in C# to add a specified number of days to a date. In C# programming, we often encounter situations where we need to add and subtract dates. The DateTime class in C# provides many convenient methods and properties for working with dates and times, including the AddDays function, which can be used to add a specified number of days to a specified date. Here is a specific code example that demonstrates how to use the DateTime.AddDays function to add a specified number of days to a date:
Nov 18, 2023 pm 03:08 PM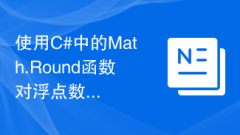
Round floating point numbers using Math.Round function in C#
Using the Math.Round function in C# to round floating-point numbers requires specific code examples. In the C# programming language, sometimes we need to round floating-point numbers. At this time, we can use the Math.Round function to achieve this function. The Math.Round function is a built-in function in C# used for mathematical calculations. Its main function is to round the specified floating point number. The following is the common format of the Math.Round function: Math.Round(doub
Nov 18, 2023 pm 02:17 PM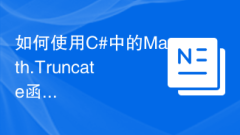
How to round down a floating point number using the Math.Truncate function in C#
How to use the Math.Truncate function in C# to round down floating point numbers requires specific code examples. In C# programming, we often encounter situations where floating point numbers need to be rounded down. Among them, rounding down is a common operation and can be implemented using the Math.Truncate function in C#. This article will introduce the usage of Math.Truncate function in detail and provide specific code examples. Math.Truncate function is a mathematical function in C#, used to convert a floating
Nov 18, 2023 pm 02:02 PM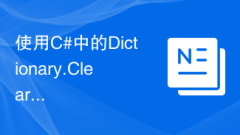
Clear all elements in a dictionary using Dictionary.Clear function in C#
Use the Dictionary.Clear function in C# to clear all elements in the dictionary. Dictionary (dictionary) is one of the commonly used data structures in C#. When it comes to data processing and storage, dictionaries provide a fast and efficient way to find, insert, and delete elements. The Dictionary class in C# is a generic class that uses key-value pairs to store data. In actual development, we often need to clear all elements in the dictionary in order to re-process the data
Nov 18, 2023 pm 01:31 PM
How to get all parts of a string that match a regular expression using the Regex.MatchCollection function in C#
How to use the Regex.MatchCollection function in C# to get all parts of a string that match the regular expression. Specific code examples are required. Regular expressions are a powerful pattern matching tool. In C#, you can use the Regex.MatchCollection function to get characters. All parts of the string that match the regular expression. This article explains how to use this function and provides specific code examples. First, we need to introduce System.Text.Reg into the code
Nov 18, 2023 pm 01:29 PM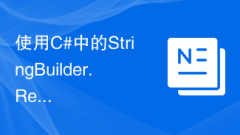
Remove a specified part of a string using the StringBuilder.Remove function in C#
Use the StringBuilder.Remove function in C# to delete the specified part of the string. In C#, the StringBuilder class is a mutable string type that allows us to modify and operate strings. The Remove function of StringBuilder provides a convenient way to delete the specified part of the string. The usage of StringBuilder.Remove function is as follows: publicStringBuilde
Nov 18, 2023 pm 01:28 PM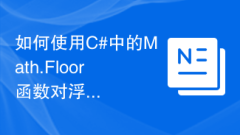
How to round down a floating point number using the Math.Floor function in C#
How to use the Math.Floor function in C# to round down floating-point numbers requires an overview of specific code examples: In C#, the Math.Floor function can round down floating-point numbers. This article will introduce the use of the Math.Floor function and provide specific code examples to help readers better understand the function and usage of the function. How to use the Math.Floor function: The Math.Floor function is used to round down a floating point number and return a value less than or equal to the specified floating point number.
Nov 18, 2023 pm 01:26 PM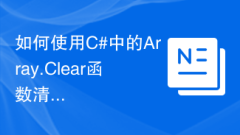
How to clear an array using the Array.Clear function in C#
How to use the Array.Clear function in C# to clear an array requires specific code examples. In C#, we often need to clear arrays for reuse. C# provides the Array.Clear function to clear the array. This article will introduce how to use the Array.Clear function and provide specific code examples. The Array.Clear function is a static method that clears the contents of the array to default values. This function contains three parameters: the array to be cleared, the starting index and the
Nov 18, 2023 pm 01:11 PM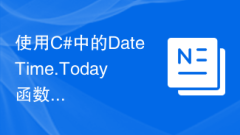
Get today's date using DateTime.Today function in C#
Getting today's date using DateTime.Today function in C# requires specific code examples C# is an object-oriented programming language that provides many built-in classes and methods to handle dates and times. Among them, the DateTime class has some very useful methods, such as the Today property, which can be used to obtain today's date. Here is a sample code that demonstrates how to get today's date using the DateTime.Today function in C#: usingSystem;
Nov 18, 2023 pm 12:41 PM
Hot tools Tags

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

vc9-vc14 (32+64 bit) runtime library collection (link below)
Download the collection of runtime libraries required for phpStudy installation

VC9 32-bit
VC9 32-bit phpstudy integrated installation environment runtime library

PHP programmer toolbox full version
Programmer Toolbox v1.0 PHP Integrated Environment

VC11 32-bit
VC11 32-bit phpstudy integrated installation environment runtime library

SublimeText3 Chinese version
Chinese version, very easy to use
