JAVA操作Hbase基础例子
package com.cma.hbase.test; import java.io.BufferedInputStream; import java.io.BufferedReader; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException
package com.cma.hbase.test;import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang.StringUtils;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.KeyValue;
import org.apache.hadoop.hbase.MasterNotRunningException;
import org.apache.hadoop.hbase.ZooKeeperConnectionException;
import org.apache.hadoop.hbase.client.Delete;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import org.apache.hadoop.hbase.client.HTable;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.util.Bytes;
import com.cma.hbase.Constants;
import com.cma.hbase.entity.DataModel;
import com.cma.hbase.tools.HbaseUtils;
public class HbaseTest {
?? ?public static void main(String[] args) {
?? ??? ?创建一张表
?? ??? ?createTable("hello_baby",new String[]{"code","ws","wd","t","ps","rh","vis","r"});
?? ??? ?写入一条数据
?? ??? ?writeRecord("hello_baby","row1","code","","code");
?? ??? ?writeRecord("hello_baby","row1","ws","","ws");
?? ??? ?writeRecord("hello_baby","row1","wd","","wd");
?? ??? ?writeRecord("hello_baby","row1","t","","t");
?? ??? ?writeRecord("hello_baby","row1","ps","","ps");
?? ??? ?writeRecord("hello_baby","row1","rh","","rh");
?? ??? ?writeRecord("hello_baby","row1","vis","","vis");
?? ??? ?writeRecord("hello_baby","row1","r","","r");
?? ??? ?写入一组数据
?? ??? ?writeRecordList("hello_baby");
?? ??? ?//查询出一条数据
?? ??? ?getRecord("hello_baby","row1");
?? ??? ?删除一行
?? ??? ?deleteRecord("hello_baby","row1");
?? ??? ?删除一张表
?? ??? ?dropTable("hello_baby");
?? ??? ?清空一张表
?? ??? ?clearTable("hello_baby");
?? ?}
?? ?/**
?? ? * 清空一张表
?? ? * @param string
?? ? */
?? ?private static void clearTable(String tableName) {
?? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ?
?? ??? ?try {
?? ??? ??? ?HBaseAdmin admin = new HBaseAdmin(cfg);
?? ??? ??? ?
?? ??? ?} catch (MasterNotRunningException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?} catch (ZooKeeperConnectionException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}finally{
?? ??? ??? ?System.out.println("tableName: "+tableName+" drop over!");
?? ??? ?}
?? ?}
?? ?/**
?? ? * 写入一组数据
?? ? * @param tableName
?? ? */
?? ?private static void writeRecordList(String tableName) {
?? ??? ?Long start = System.currentTimeMillis();
?? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ?try {
?? ??? ??? ?HTable htable = new HTable(cfg,tableName);
?? ??? ??? ?List
?? ??? ??? ?System.out.println(puts.size());
?? ??? ??? ?htable.put(puts);
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}finally{
?? ??? ??? ?System.out.println("tableName:"+tableName+" write over!");
?? ??? ?}
?? ??? ?Long end = System.currentTimeMillis();
?? ??? ?System.out.println("cost time: "+(end -start));
?? ?}
?? ?private static List
?? ??? ?List
?? ??? ?List
?? ??? ?try {
?? ??? ??? ?List
?? ??? ??? ?for(int i = 1 ;i ?? ??? ??? ??? ?putRecord = getPutsByLine(lines.get(i));
?? ??? ??? ??? ?putList.addAll(putRecord);
?? ??? ??? ?}
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}
?? ??? ?return putList;
?? ?}
?? ?/**
?? ? * 获得一组Put
?? ? * @param line
?? ? * @return
?? ? */
?? ?private static List
?? ??? ?List
?? ??? ?Put put = null;
?? ??? ?
?? ??? ?if(StringUtils.isNotBlank(line)){
?? ??? ??? ?String[] columns = line.split(",");
?? ??? ??? ?String[] families = Constants.FAMILIES;
?? ??? ??? ?String rowKey = "201307310800"+columns[0];
?? ??? ??? ?for(int i = 0;i ?? ??? ??? ??? ?String family = families[i];
?? ??? ??? ??? ?String qualifier = "";
?? ??? ??? ??? ?String value = columns[i];
?? ??? ??? ??? ?put = getPut(rowKey,family,qualifier,value);
?? ??? ??? ??? ?puts.add(put);
?? ??? ??? ?}
?? ??? ?}
?? ??? ?return puts;
?? ?}
?? ?/**
?? ? * 组装一个Put
?? ? * @param rowKey
?? ? * @param family
?? ? * @param qualifier
?? ? * @param value
?? ? * @return
?? ? */
?? ?private static Put getPut(String rowKey, String family, String qualifier,
?? ??? ??? ?String value) {
?? ??? ?Put put = new Put(Bytes.toBytes(rowKey));
?? ??? ?put.add(Bytes.toBytes(family), Bytes.toBytes(qualifier), Bytes.toBytes(value));
?? ??? ?return put;
?? ?}
?? ?/**
?? ? * 查询出一条数据
?? ? * @param tableName
?? ? * @param rowKey
?? ? */
?? ?private static void getRecord(String tableName, String rowKey) {
?? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ?try {
?? ??? ??? ?HTable htable = new HTable(cfg,tableName);
?? ??? ??? ?Get get = new Get(Bytes.toBytes(rowKey));
?? ??? ??? ?Result rs = htable.get(get);
?? ??? ??? ?
?? ??? ??? ?for(KeyValue kv : rs.raw()){
?? ??? ??? ??? ?System.out.print(new String(kv.getRow())+" *** ");
?? ??? ??? ??? ?System.out.print(new String(kv.getFamily())+" *** ");
?? ??? ??? ??? ?System.out.print(new String(kv.getQualifier())+" *** ");
?? ??? ??? ??? ?System.out.print(new String(kv.getValue()));
?? ??? ??? ??? ?System.out.println();
?? ??? ??? ?}
?? ??? ??? ?
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}
?? ?}
?? ?/**
?? ? * 删除一张表
?? ? * @param tableName
?? ? */
?? ?private static void dropTable(String tableName) {
?? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ?
?? ??? ?try {
?? ??? ??? ?HBaseAdmin admin = new HBaseAdmin(cfg);
?? ??? ??? ?admin.disableTable(tableName);
?? ??? ??? ?admin.deleteTable(tableName);
?? ??? ?} catch (MasterNotRunningException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?} catch (ZooKeeperConnectionException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}finally{
?? ??? ??? ?System.out.println("tableName: "+tableName+" drop over!");
?? ??? ?}
?? ?}
?? ?/**
?? ? * 删除一行
?? ? * @param tableName
?? ? * @param rowKey
?? ? */
?? ?private static void deleteRecord(String tableName, String rowKey) {
?? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ?
?? ??? ?try {
?? ??? ??? ?HTable htable = new HTable(cfg,tableName);
?? ??? ??? ?Delete del = new Delete(Bytes.toBytes(rowKey));
?? ??? ??? ?htable.delete(del);
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}finally{
?? ??? ??? ?System.out.println("rowKey: "+rowKey+" delete over!");
?? ??? ?}
?? ?}
?? ?/**
?? ? * 存储一列
?? ? * @param tableName
?? ? * @param rowKey
?? ? * @param family
?? ? * @param qualifier
?? ? * @param value
?? ? */
?? ?private static void writeRecord(String tableName,String rowKey,String family,String qualifier,String value) {
?? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ?try {
?? ??? ??? ?HTable htable = new HTable(cfg,tableName);
?? ??? ??? ?Put put = new Put(Bytes.toBytes(rowKey));
?? ??? ??? ?put.add(Bytes.toBytes(family), Bytes.toBytes(qualifier), Bytes.toBytes(value));
?? ??? ??? ?htable.put(put);
?? ??? ??? ?
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}finally{
?? ??? ??? ?System.out.println("family: "+family+" put over!");
?? ??? ?}
?? ?}
?? ?/**
?? ? * 创建一张表
?? ? * @param tableName
?? ? * @param families
?? ? */
?? ?private static void createTable(String tableName,String[] families) {
?? ??? ?HBaseAdmin admin = null;
?? ??? ?try {
?? ??? ??? ?Configuration cfg = HbaseUtils.getCfg();
?? ??? ??? ?System.out.println(cfg);
?? ??? ??? ?admin = new HBaseAdmin(cfg);
?? ??? ??? ?if(admin.tableExists(tableName)){
?? ??? ??? ??? ?System.out.println("表:"+tableName+" 已经存在!");
?? ??? ??? ?}else{
?? ??? ??? ??? ?HTableDescriptor tableDesc = new HTableDescriptor(tableName);
?? ??? ??? ??? ?for(String column : families){
?? ??? ??? ??? ??? ?tableDesc.addFamily(new HColumnDescriptor(column));
?? ??? ??? ??? ?}
?? ??? ??? ??? ?admin.createTable(tableDesc);
?? ??? ??? ?}
?? ??? ?} catch (MasterNotRunningException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?} catch (ZooKeeperConnectionException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?} catch (IOException e) {
?? ??? ??? ?e.printStackTrace();
?? ??? ?}finally{
?? ??? ??? ?System.out.println("over!");
?? ??? ?}
?? ?}
?? ?
}
作者:lovemelovemycode 发表于2013-8-25 20:35:24 原文链接
阅读:97 评论:0 查看评论
原文地址:JAVA操作Hbase基础例子, 感谢原作者分享。

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


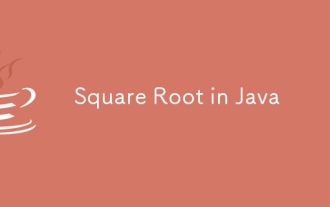
Leitfaden zur Quadratwurzel in Java. Hier diskutieren wir anhand eines Beispiels und seiner Code-Implementierung, wie Quadratwurzel in Java funktioniert.
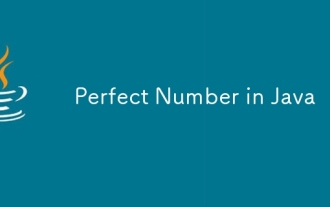
Leitfaden zur perfekten Zahl in Java. Hier besprechen wir die Definition, Wie prüft man die perfekte Zahl in Java?, Beispiele mit Code-Implementierung.
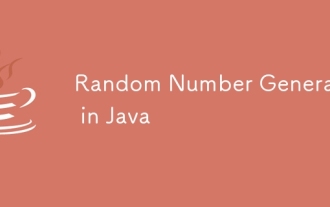
Leitfaden zum Zufallszahlengenerator in Java. Hier besprechen wir Funktionen in Java anhand von Beispielen und zwei verschiedene Generatoren anhand ihrer Beispiele.
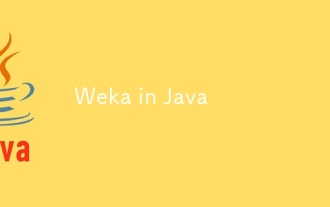
Leitfaden für Weka in Java. Hier besprechen wir die Einführung, die Verwendung von Weka Java, die Art der Plattform und die Vorteile anhand von Beispielen.
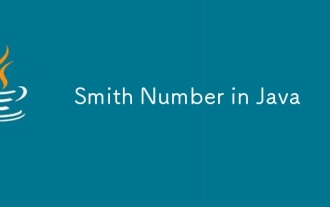
Leitfaden zur Smith-Zahl in Java. Hier besprechen wir die Definition: Wie überprüft man die Smith-Nummer in Java? Beispiel mit Code-Implementierung.

In diesem Artikel haben wir die am häufigsten gestellten Fragen zu Java Spring-Interviews mit ihren detaillierten Antworten zusammengestellt. Damit Sie das Interview knacken können.
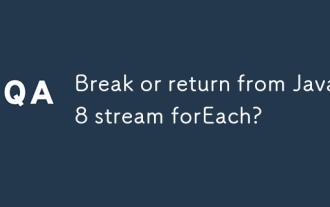
Java 8 führt die Stream -API ein und bietet eine leistungsstarke und ausdrucksstarke Möglichkeit, Datensammlungen zu verarbeiten. Eine häufige Frage bei der Verwendung von Stream lautet jedoch: Wie kann man von einem Foreach -Betrieb brechen oder zurückkehren? Herkömmliche Schleifen ermöglichen eine frühzeitige Unterbrechung oder Rückkehr, aber die Stream's foreach -Methode unterstützt diese Methode nicht direkt. In diesem Artikel werden die Gründe erläutert und alternative Methoden zur Implementierung vorzeitiger Beendigung in Strahlverarbeitungssystemen erforscht. Weitere Lektüre: Java Stream API -Verbesserungen Stream foreach verstehen Die Foreach -Methode ist ein Terminalbetrieb, der einen Vorgang für jedes Element im Stream ausführt. Seine Designabsicht ist
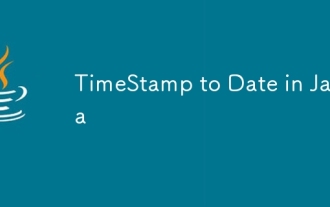
Anleitung zum TimeStamp to Date in Java. Hier diskutieren wir auch die Einführung und wie man Zeitstempel in Java in ein Datum konvertiert, zusammen mit Beispielen.
