


Python. Automatisieren Sie die Erstellung von Backups der MySQL-Datenbank.
Dieses Skript automatisiert die Erstellung von Backups von MySQL-Datenbanken, deren Wiederherstellung und die Verwaltung der Datenbank- und Benutzererstellung auf dem Ziel-MySQL-Server.
import subprocess import datetime import sys import os def check_and_create_database(host, port, username, password, database): # Command to check if the database exists check_database_command = f"mysql -sN --host={host} --port={port} --user={username} --password={password} -e \"SELECT EXISTS(SELECT 1 FROM INFORMATION_SCHEMA.SCHEMATA WHERE SCHEMA_NAME = '{database}')\" 2>/dev/null" # Execute the command output = subprocess.check_output(check_database_command, shell=True) # If the output contains b'1', the database exists if b'1' in output: subprocess.run(check_database_command, shell=True, check=True) print(f"Database '{database}' already exists.") sys.exit(1) else: # If the command fails, the database does not exist print(f"Database '{database}' does not exist. Creating...") # Command to create the database create_database_command = f"mysql --host={host} --port={port} --user={username} --password={password} -e 'CREATE DATABASE {database}' 2>/dev/null" subprocess.run(create_database_command, shell=True) def check_and_create_user(host, port, username, password, database, new_username, new_password): # Command to check if the user exists check_user_command = f"mysql -sN --host={host} --port={port} --user={username} --password={password} -e \"SELECT EXISTS(SELECT 1 FROM mysql.user WHERE user = '{new_username}')\" 2>/dev/null" # Execute the command output = subprocess.check_output(check_user_command, shell=True) # If the output contains b'1', the user exists if b'1' in output: print(f"User '{new_username}' already exists.") sys.exit(1) else: # The user does not exist, create it print(f"User '{new_username}' does not exist. Creating...") # Command to create the user and grant privileges create_user_command = f"mysql --host={host} --port={port} --user={username} --password={password} -e \"CREATE USER '{new_username}'@'%' IDENTIFIED BY '{new_password}'; GRANT ALL PRIVILEGES ON {database}.* TO '{new_username}'@'%'; FLUSH PRIVILEGES;\" 2>/dev/null" subprocess.run(create_user_command, shell=True) def backup_mysql_database(host, port, username, password, database, backup_path): # Check if the backup directory exists if not os.path.exists(backup_path): print(f"Error: Backup directory '{backup_path}' does not exist.") sys.exit(1) # Create a filename for the backup with the current date and time timestamp = datetime.datetime.now().strftime("%Y-%m-%d_%H-%M-%S") backup_file = f"{backup_path}/{database}_{timestamp}.sql" # Command to create a database backup using mysqldump dump_command = f"mysqldump --no-tablespaces --host={host} --port={port} --user={username} --password={password} {database} > {backup_file} 2>/dev/null" # Execute the mysqldump command subprocess.run(dump_command, shell=True) return backup_file def restore_mysql_database(host, port, username, password, database, backup_file): # Command to restore a database from a backup using mysql restore_command = f"mysql --host={host} --port={port} --user={username} --password={password} {database} < {backup_file} 2>/dev/null" # Execute the mysql command subprocess.run(restore_command, shell=True) def main(): # Connection parameters to the source MySQL database source_host = "127.0.0.1" source_port = "3309" source_username = "my_user" source_password = "my_password" source_database = "my_database" # Connection parameters to the target MySQL database target_host = "127.0.0.1" target_port = "3309" new_username = "new_username" new_password = "new_password" target_database = "my_database_two" target_username = "root" target_password = "root_password" # Path to save the backup locally backup_path = "my_dbs_dumps" # Check if source_database is different from target_database if source_database == target_database: print("Error: Source database should be different from target database.") sys.exit(1) # Check and create the target database if it does not exist check_and_create_database(target_host, target_port, target_username, target_password, target_database) # Check and create the target user if it does not exist check_and_create_user(target_host, target_port, target_username, target_password, target_database, new_username, new_password) # Create a backup of the MySQL database backup_file = backup_mysql_database(source_host, source_port, source_username, source_password, source_database, backup_path) print(f"Database backup created: {backup_file}") # Restore the database on the target server from the backup restore_mysql_database(target_host, target_port, target_username, target_password, target_database, backup_file) print("Database backup restored on the target server.") if __name__ == "__main__": main()
check_and_create_database:
Diese Funktion prüft, ob eine Datenbank auf einem MySQL-Server vorhanden ist. Wenn die Datenbank nicht vorhanden ist, wird sie erstellt. Zur Überprüfung oder Erstellung werden Parameter wie Host, Port, Benutzername, Passwort und Datenbankname benötigt.
check_and_create_user:
Wie die Datenbankfunktion prüft diese Funktion, ob ein Benutzer auf dem MySQL-Server existiert. Wenn der Benutzer nicht vorhanden ist, wird er erstellt und Berechtigungen für eine bestimmte Datenbank gewährt. Es werden auch Parameter wie Host, Port, Benutzername, Passwort, der Name der Datenbank, der neue Benutzername und das neue Passwort benötigt.
backup_mysql_database:
Diese Funktion führt eine Sicherung einer MySQL-Datenbank mit mysqldump durch. Es werden Parameter wie Host, Port, Benutzername, Passwort, Datenbankname und der Pfad zum Speichern der Sicherungsdatei benötigt.
restore_mysql_database:
Diese Funktion stellt eine MySQL-Datenbank aus einer Sicherungsdatei wieder her. Es werden Parameter wie Host, Port, Benutzername, Passwort, Datenbankname und der Pfad zur Sicherungsdatei benötigt.
main:
Dies ist die Hauptfunktion des Skripts. Es richtet Parameter für die Quell- und Ziel-MySQL-Datenbanken ein, einschließlich Verbindungsdetails, Datenbanknamen und Sicherungspfade. Anschließend führt es Prüfungen durch, um sicherzustellen, dass die Quell- und Zieldatenbanken unterschiedlich sind, erstellt die Zieldatenbank und den Benutzer, falls diese nicht vorhanden sind, erstellt eine Sicherung der Quelldatenbank und stellt schließlich die Sicherung in der Zieldatenbank wieder her.
Darüber hinaus verwendet das Skript das Unterprozessmodul, um Shell-Befehle für MySQL-Operationen (mysql, mysqldump) auszuführen und führt Fehlerbehandlung und Ausgabeumleitung (2>/dev/null) durch, um unnötige Ausgaben zu unterdrücken.
Wenn Sie mit MySQL-Datenbanken arbeiten und eine Automatisierung erstellen möchten, hilft Ihnen dieser Code.
Dieser Code stellt eine gute Ausgangsvorlage zum Erstellen von Automatisierungsskripten für die Verwaltung von MySQL-Datenbanken dar.
dmi@dmi-laptop:~/my_python$ python3 mysql_backup_restore.py Database 'my_database_two' does not exist. Creating... User 'new_username' does not exist. Creating... Database backup created: my_dbs_dumps/my_database_2024-05-13_20-05-24.sql Database backup restored on the target server. dmi@dmi-laptop:~/my_python$
ask_dima@yahoo.com
Das obige ist der detaillierte Inhalt vonPython. Automatisieren Sie die Erstellung von Backups der MySQL-Datenbank.. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen
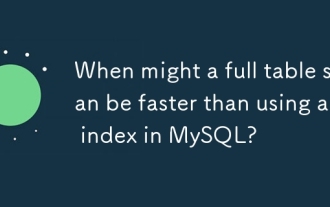
Die volle Tabellenscannung kann in MySQL schneller sein als die Verwendung von Indizes. Zu den spezifischen Fällen gehören: 1) das Datenvolumen ist gering; 2) Wenn die Abfrage eine große Datenmenge zurückgibt; 3) wenn die Indexspalte nicht sehr selektiv ist; 4) Wenn die komplexe Abfrage. Durch Analyse von Abfrageplänen, Optimierung von Indizes, Vermeidung von Überindex und regelmäßiger Wartung von Tabellen können Sie in praktischen Anwendungen die besten Auswahlmöglichkeiten treffen.
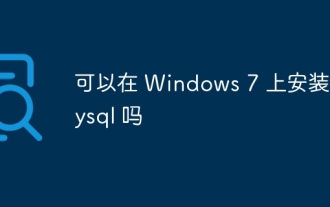
Ja, MySQL kann unter Windows 7 installiert werden, und obwohl Microsoft Windows 7 nicht mehr unterstützt hat, ist MySQL dennoch kompatibel damit. Während des Installationsprozesses sollten jedoch folgende Punkte festgestellt werden: Laden Sie das MySQL -Installationsprogramm für Windows herunter. Wählen Sie die entsprechende Version von MySQL (Community oder Enterprise) aus. Wählen Sie während des Installationsprozesses das entsprechende Installationsverzeichnis und das Zeichen fest. Stellen Sie das Stammbenutzerkennwort ein und behalten Sie es ordnungsgemäß. Stellen Sie zum Testen eine Verbindung zur Datenbank her. Beachten Sie die Kompatibilitäts- und Sicherheitsprobleme unter Windows 7, und es wird empfohlen, auf ein unterstütztes Betriebssystem zu aktualisieren.
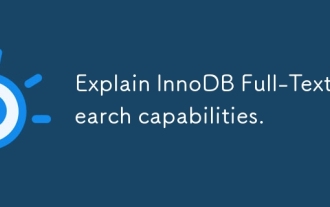
Die Volltext-Suchfunktionen von InnoDB sind sehr leistungsfähig, was die Effizienz der Datenbankabfrage und die Fähigkeit, große Mengen von Textdaten zu verarbeiten, erheblich verbessern kann. 1) InnoDB implementiert die Volltext-Suche durch invertierte Indexierung und unterstützt grundlegende und erweiterte Suchabfragen. 2) Verwenden Sie die Übereinstimmung und gegen Schlüsselwörter, um den Booleschen Modus und die Phrasesuche zu unterstützen. 3) Die Optimierungsmethoden umfassen die Verwendung der Word -Segmentierungstechnologie, die regelmäßige Wiederaufbauung von Indizes und die Anpassung der Cache -Größe, um die Leistung und Genauigkeit zu verbessern.
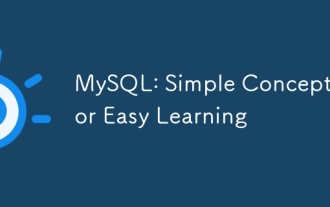
MySQL ist ein Open Source Relational Database Management System. 1) Datenbank und Tabellen erstellen: Verwenden Sie die Befehle erstellte und creatEtable. 2) Grundlegende Vorgänge: Einfügen, aktualisieren, löschen und auswählen. 3) Fortgeschrittene Operationen: Join-, Unterabfrage- und Transaktionsverarbeitung. 4) Debugging -Fähigkeiten: Syntax, Datentyp und Berechtigungen überprüfen. 5) Optimierungsvorschläge: Verwenden Sie Indizes, vermeiden Sie ausgewählt* und verwenden Sie Transaktionen.
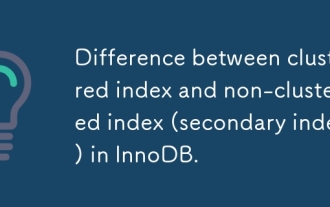
Der Unterschied zwischen Clustered Index und nicht klusterer Index ist: 1. Clustered Index speichert Datenzeilen in der Indexstruktur, die für die Abfrage nach Primärschlüssel und Reichweite geeignet ist. 2. Der nicht klusterierte Index speichert Indexschlüsselwerte und -zeiger auf Datenzeilen und ist für nicht-primäre Schlüsselspaltenabfragen geeignet.
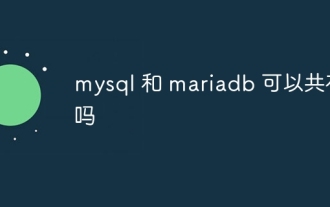
MySQL und Mariadb können koexistieren, müssen jedoch mit Vorsicht konfiguriert werden. Der Schlüssel besteht darin, jeder Datenbank verschiedene Portnummern und Datenverzeichnisse zuzuordnen und Parameter wie Speicherzuweisung und Cache -Größe anzupassen. Verbindungspooling, Anwendungskonfiguration und Versionsunterschiede müssen ebenfalls berücksichtigt und sorgfältig getestet und geplant werden, um Fallstricke zu vermeiden. Das gleichzeitige Ausführen von zwei Datenbanken kann in Situationen, in denen die Ressourcen begrenzt sind, zu Leistungsproblemen führen.
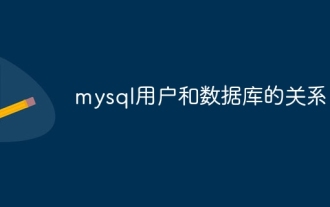
In der MySQL -Datenbank wird die Beziehung zwischen dem Benutzer und der Datenbank durch Berechtigungen und Tabellen definiert. Der Benutzer verfügt über einen Benutzernamen und ein Passwort, um auf die Datenbank zuzugreifen. Die Berechtigungen werden über den Zuschussbefehl erteilt, während die Tabelle durch den Befehl create table erstellt wird. Um eine Beziehung zwischen einem Benutzer und einer Datenbank herzustellen, müssen Sie eine Datenbank erstellen, einen Benutzer erstellen und dann Berechtigungen erfüllen.
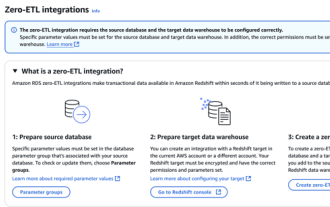
Vereinfachung der Datenintegration: AmazonRDSMYSQL und Redshifts Null ETL-Integration Die effiziente Datenintegration steht im Mittelpunkt einer datengesteuerten Organisation. Herkömmliche ETL-Prozesse (Extrakt, Konvertierung, Last) sind komplex und zeitaufwändig, insbesondere bei der Integration von Datenbanken (wie AmazonRDSMysQL) in Data Warehouses (wie Rotverschiebung). AWS bietet jedoch keine ETL-Integrationslösungen, die diese Situation vollständig verändert haben und eine vereinfachte Lösung für die Datenmigration von RDSMysQL zu Rotverschiebung bietet. Dieser Artikel wird in die Integration von RDSMYSQL Null ETL mit RedShift eintauchen und erklärt, wie es funktioniert und welche Vorteile es Dateningenieuren und Entwicklern bringt.
