TreeMap wird mit der Abstract-Klasse verwendet, um die Map- und NavigableMap-Schnittstelle in Java bereitzustellen. Die Karte wird nach der natürlichen Reihenfolge ihrer Schlüssel oder durch einen vorgefertigten Komparator sortiert, der vom Builder abhängt. Dies ist eine einfache Möglichkeit, die Schlüssel-Wert-Paare zu sortieren und zu speichern. Die von der Baumkarte beibehaltene Speicherreihenfolge muss die gleiche sein wie bei jeder anderen klassifizierten Karte, unabhängig von den spezifischen Komparatoren.
Starten Sie Ihren kostenlosen Softwareentwicklungskurs
Webentwicklung, Programmiersprachen, Softwaretests und andere
Im Folgenden sind einige der Eigenschaften von TreeMap aufgeführt.
- Es besteht aus einzigartigen Elementen.
- Es darf keinen Nullschlüssel haben.
- Kann mehrmals Nullwerte haben.
- Nicht synchronisiert.
Erklärung:
public class TreeMap<Key, Value> extends AbstractMap<Key, Value>implements NavigableMap<Key, Value>, Cloneable, Serializable
Nach dem Login kopieren
Hier sind Schlüssel und Wert die Art der Schlüssel bzw. zugeordneten Werte in dieser Karte.
Konstrukteure von TreeMap
Im Folgenden sind die Konstrukteure von TreeMap aufgeführt.
-
TreeMap(): Eine neue und leere Baumkarte wird durch natürliche Reihenfolge der Schlüssel erstellt.
-
TreeMap( Comparator super Key> comparator): Eine neue und leere Baumkarte wird erstellt, indem die im Komparator angegebenen Schlüssel bestellt werden.
-
TreeMap( Map erweitert Key,? Ext Value> m): Eine neue Baumkarte wird mit den Zuordnungen in Karte m und natürlicher Reihenfolge der Schlüssel erstellt.
-
TreeMap( SortedMap<Key,? erweitert Value> m): Eine neue Baumkarte wird mit den Zuordnungen in Karte m und der Reihenfolge der im Komparator angegebenen Schlüssel erstellt.
Methoden von TreeMap
TreeMap bietet eine große Sammlung von Methoden, die bei der Ausführung verschiedener Funktionen helfen. Sie sind:
-
clear(): All the mapping in the map will be removed.
-
clone(): A shallow copy will be returned for the TreeMap instance.
-
containsKey(Objectk): If there is mapping available for the specified key, true will be returned.
-
containsValue(Objectv): If there is mapping available for one or more keys for the value v, true will be returned.
-
ceilingKey(Kkey): Least key which is larger than or equal to the specified key will be returned. If there is no key, then null will be returned.
-
ceilingEntry(Kkey): Key-value pair for the least key, which is larger than or equal to the specified key will be returned. If there is no key, then null will be returned.
-
firstKey(): First or last key in the map will be returned.
-
firstEntry(): Key-value pair for the least or first key in the map will be returned. If there is no key, then null will be returned.
-
floorKey(Kkey): The Largest key, which is less than or equal to the specified key, will be returned. If there is no key, then null will be returned.
-
floorEntry(Kkey): Key-value pair for the largest key, which is less than or equal to the specified key, will be returned. If there is no key, then null will be returned.
-
lastKey(): Highest or last key in the map will be returned.
-
lastEntry(): Key-value pair for the largest key in the map will be returned. If there is no key, then null will be returned.
-
lowerKey(Kkey): The Largest key, which is strictly smaller than the specified key, will be returned. If there is no key, then null will be returned.
-
lowerEntry(Kkey): Key-value pair for the largest key, which is strictly smaller than the specified key, will be returned. If there is no key, then null will be returned.
-
remove(Objectk): Mapping for the specified key in the map will be removed.
-
size(): Count of key-value pairs in the map will be returned.
-
higherEntry(Kkey): Key-value pair for the smallest key, which is strictly larger than the specified key, will be returned. If there is no key, then null will be returned.
-
higherKey(Kkey): The Smallest key, which is strictly higher than the specified key, will be returned. If there is no key, then null will be returned.
-
descendingMap(): Reverse order will be returned for the mappings.
-
entrySet(): Set view will be returned for the mappings.
-
get(Objectk): The value of the specified key will be returned. If the key does not contain any mapping, then null will be returned.
-
keySet(): Set view will be returned for the keys.
-
navigableKeySet(): NavigableSet view will be returned for the keys.
-
pollFirstEntry(): Key-value pair for the least or first key in the map will be removed and returned. If the map is empty, then null will be returned.
-
pollLastEntry(): Key-value pairs for the greatest key in the map will be removed and returned. If the map is empty, then null will be returned.
-
values(): Collection view will be returned for the values in the map.
Example to Implement TreeMap in Java
Now, let us see a sample program to create a treemap and add elements to it.
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
public class TreeMapExample {
public static void main(String args[]) {
// Tree Map creation
TreeMap tmap = new TreeMap();
// Add elements to the treemap tmap
tmap.put("Anna Jeshua", new Double(3459.70));
tmap.put("Annamu Jeshua", new Double(321.56));
tmap.put("Izanorah Denan", new Double(1234.87));
tmap.put("Adam Jeshua", new Double(89.35));
tmap.put("Anabeth Jeshua", new Double(-20.98));
// Retrieve the entry set of treemap
Set set = tmap.entrySet();
// Create an iterator itr
Iterator itr = set.iterator();
// Display the elements in treemap using while loop
while(itr.hasNext()) {
Map.Entry mp = (Map.Entry)itr.next();
System.out.print(" Key : " + mp.getKey() + ";");
System.out.println(" Value : "+ mp.getValue());
}
System.out.println();
// Add 2500 to Anabeth's value
double val = ((Double)tmap.get("Anabeth Jeshua")).doubleValue();
tmap.put("Anabeth Jeshua", new Double(val + 2500));
System.out.println("Anabeth's new value: " + tmap.get("Anabeth Jeshua"));
} }
Nach dem Login kopieren
Output:
Keys and corresponding values of the TreeMap will be displayed on executing the code.
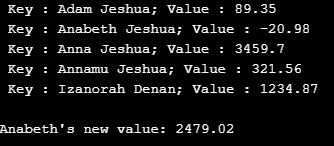
Explanation:
- Erstellen Sie zunächst eine TreeMap und fügen Sie ihr Elemente hinzu.
- Um die Elemente anzuzeigen, muss ein Iterator erstellt werden.
- Mithilfe des Iterators werden alle Schlüssel und ihre entsprechenden Werte angezeigt.
- Um 2500 zum Wert eines Schlüssels hinzuzufügen, wird auch die Methode put() verwendet.
Fazit
Java TreeMap ist eine Implementierung des Rot-Schwarz-Baums, der beim Speichern von Schlüssel-Wert-Paaren in sortierter Reihenfolge hilft. In diesem Dokument werden verschiedene Details wie Deklaration, Konstruktoren, Methoden und Beispielprogramm von Java TreeMap ausführlich besprochen.
Das obige ist der detaillierte Inhalt vonWas ist TreeMap in Java?. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!