Die SortedDictionary-Klasse in C# wird als SortedDictionary dargestellt. Diese besteht aus einer Sammlung von Schlüsseln und Werten, wobei der Schlüssel das Wort und der Wert die Definition darstellt. Diese Schlüssel-Wert-Paare werden basierend auf dem Schlüssel und diesem SortedDictionary sortiert. Die Klasse gehört zum System.Collection.Generics-Namespace und die Schlüssel in SortedDictionary sind immer eindeutig, unveränderlich und nicht null, aber der Wert kann null sein, wenn der Werttyp vom Typ und Referenztyp ist und die Einfüge- und Abrufvorgänge mit SortedDictionary schneller sind Klasse und der Abruf des Schlüsselwertpaares in der SortedDictionary-Klasse erfolgt mithilfe der KeyValuePair-Struktur.
Syntax:
SortedDictionary<TKey, TValue>variable_name = new SortedDictionary<TKey, TValue>();
Nach dem Login kopieren
Arbeitsweise der SortedDictionary-Klasse in C#
- ICollection>, IEnumerable, IReadOnlyCollection>, IEnumerable>, ICollection, IDictionary, IDictionary, IEnumerable, IReadOnlyDictionary Schnittstellen werden von der SortedDictionary-Klasse implementiert.
- Das Einfügen von Elementen und das Entfernen der Elemente können mit der SortedDictionary-Klasse schneller erfolgen.
- Die Schlüssel müssen eindeutig sein und es dürfen keine doppelten Schlüssel in der SortedDictionary-Klasse vorhanden sein.
- Die Schlüssel sind eindeutig und werden in der SortedDictionary-Klasse nicht null sein.
- Wenn der Wert vom Typ Referenz ist, darf der Wert null sein.
- Derselbe Typ von Schlüssel- und Wertpaaren kann mit der SortedDictionary-Klasse gespeichert werden.
- Das SortedDictionary in C# ist dynamischer Natur, was bedeutet, dass die Größe des SortedDictionary je nach Bedarf zunimmt.
- Die Sortierung erfolgt in absteigender Reihenfolge nach SortedDictionary-Klasse.
- Die Gesamtzahl der Schlüssel- und Wertpaare, die die SortedDictionary-Klasse aufnehmen kann, ist die Kapazität der SortedDictionary-Klasse.
Konstruktoren von C# SortedDictionary
Im Folgenden sind die Konstruktoren von C# SortedDictionary aufgeführt:
1. SortedDictionary()
Eine Instanz der SortedDictionary-Klasse wird initialisiert, die leer ist, und die Implementierung von IComparer wird standardmäßig für den Typ Schlüssel verwendet.
2. SortedDictionary(IComparer)
Eine Instanz der SortedDictionary-Klasse wird initialisiert, die leer ist, und die angegebene Implementierung von IComparer wird für den Schlüsselvergleich verwendet.
3. SortedDictionary(IDictionary)
Eine Instanz der SortedDictionary-Klasse wird initialisiert, die aus Elementen besteht, die aus dem IDictionary stammen, das als Parameter angegeben ist, und die Implementierung von ICompare wird standardmäßig für den Typ Schlüssel verwendet.
4. SortedDictionary(IDictionary, IComparer)
Eine Instanz der SortedDictionary-Klasse wird initialisiert, die aus Elementen besteht, die aus dem als Parameter angegebenen IDictionary kopiert wurden, und die angegebene Implementierung von IComparer wird für den Schlüsselvergleich verwendet.
Methoden von C# SortedDictionary
Im Folgenden sind die Methoden aufgeführt:
-
Add(TKey, TValue): An element with key and value specified as parameters is added into the SortedDictionary using Add(TKey, TValue) method.
-
Remove(Tkey): An element with key specified as parameter is removed from the SortedDictionary using Remove(TKey) method.
-
ContainsKey(TKey): The ContainsKey(TKey) method is used to determine if the key specified as parameter is present in the SortedDictionary.
-
ContainsValue(TValue): The ContainsKey(TValue) method is used to determine if the value specified as parameter is present in the SortedDictionary.
-
Clear(): The clear() method is used to clear all the objects from the SortedDictionary.
-
CopyTo(KeyValuePair[], Int32): The CopyTo(KeyValuePair[], Int32) method is used to copy the elements of the SortedDictionary to the array of KeyValuePair structures specified as the parameter with the array starting from the index specified in the parameter.
-
Equals(Object): The Equals(Object) method is used to determine if the object specified as the parameter is equal to the current object.
-
GetEnumerator(): The GetEnumerator() method is used to return an enumerator which loops through the SortedDictionary.
-
GetHashCode(): The GetHashCode() method is the hash function by default.
-
GetType(): The GetType() method returns the current instance type.
-
MemberwiseClone(): The MemberwiseClone() method is used to create a shallow copy of the current object.
-
ToString():The ToString() method is used to return a string which represents the current object.
-
TryGetValue(TKey, TValue): The TryGetValue(TKey, TValue) method is used to obtain the value associated with key specified as parameter.
Examples
Given below are the examples mentioned:
Example #1
C# program to demonstrate Add method, Remove method, ContainsKey method, ContainsValue method and TryGetValue method of Sorted Dictionary class.
Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
//a class called program is defined
public class program
{
//main method is called
public static void Main()
{
//a new sorted dictionary is created with key type int and value type string
SortedDictionary<int, string>st = new SortedDictionary<int, string>();
//Add method is used to add objects to the dictionary
st.Add(30,"India");
st.Add(10,"China");
st.Add(20,"Nepal");
st.Remove(10);
Console.WriteLine("If the key 30 is present?{0}", st.ContainsKey(30));
Console.WriteLine("If the key 20 is present? {0}", st.Contains(new KeyValuePair<int, string>(20, "Nepal")));
//new sorted dictionary of both string key and string value types is defined
SortedDictionary<string, string> st1 = new SortedDictionary<string, string>();
st1.Add("Flag","India");
Console.WriteLine("If the value India is present?{0}", st1.ContainsValue("India"));
string rest;
if(st.TryGetValue(30, out rest))
{
Console.WriteLine("The value of the specified key is {0}", rest);
}
else
{
Console.WriteLine("The specified key is not present.");
}
}
}
Nach dem Login kopieren
Output:
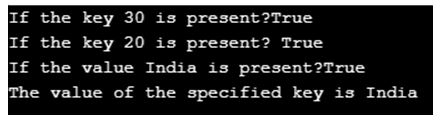
Explanation:
- In the above program, a class called program is defined. Then the main method is called. Then a new sorted dictionary is created with key type int and value type string. Then Add method is used to add objects to the sorted dictionary. Then Remove method is used to remove objects from the sorted dictionary.
- Then new sorted dictionary of both string key and string value types is defined. Then contains value method is used to determine if a certain value is present in the sorted dictionary. Then trygetvalue method is used to obtain the value of a specified key.
Example #2
C# program to demonstrate Add method and Clear method of sorted dictionary class.
Code:
using System;
using System.Collections.Generic;
//a class called check is defined
class check
{
// main method is called
public static void Main()
{
// a new sorted dictionary is created with key type string and value type string
SortedDictionary<string, string> tam = new SortedDictionary<string, string>();
// using add method in dictionary to add the objects to the dictionary
tam.Add("R", "Red");
tam.Add("G", "Green");
tam.Add("Y", "Yellow");
// a foreach loop is used to loop around every key in the dictionary and to obtain each key value
foreach(KeyValuePair<string,string>ra in tam)
{
Console.WriteLine("The key and value pairs is SortedDictionary are = {0} and {1}", ra.Key, ra.Value);
}
//using clear method to remove all the objects from sorted dictionary
tam.Clear();
foreach(KeyValuePair<string,string>tr in tam)
{
Console.WriteLine("The key and value pairs is SortedDictionary are = {0} and {1}", tr.Key, tr.Value);
}
}
}
Nach dem Login kopieren
Output:

Explanation:
- In the above program, check is the class defined. Then main method is called. Then a new sorted dictionary is created with key type string and value type string. Then we have used add method to add the objects to the sorted dictionary.
- Then a for each loop is used to loop around every key in the sorted dictionary to obtain each key value. Then clear method is used to clear the console.
Das obige ist der detaillierte Inhalt vonC# SortedDictionary. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!