Erstellen Sie ein Wordle-Spiel in HTML, CSS und JS
Erstellen Sie eine Datei mit dem Namen index.html:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Wordle Game</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <h1>Wordle Game</h1> <div id="game-board"></div> <input type="text" id="guess-input" maxlength="5" placeholder="Enter your guess"> <button id="submit-btn">Submit</button> <p id="message"></p> </div> <script src="script.js"></script> </body> </html>
Erstellen Sie eine Datei mit dem Namen „styles.css:“
body { font-family: Arial, sans-serif; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; background-color: #f0f0f0; } .container { text-align: center; } #game-board { display: grid; grid-template-columns: repeat(5, 40px); gap: 10px; margin-bottom: 20px; } .cell { width: 40px; height: 40px; display: flex; justify-content: center; align-items: center; border: 1px solid #ddd; font-size: 18px; } .correct { background-color: #6aaa64; color: white; } .present { background-color: #c9b458; color: white; } .absent { background-color: #787c7e; color: white; }
Erstellen Sie eine Datei mit dem Namen script.js:
const possibleWords = ['apple', 'brave', 'crane', 'dough', 'eagle']; // List of possible words const targetWord = possibleWords[Math.floor(Math.random() * possibleWords.length)]; // Random target word const gameBoard = document.getElementById('game-board'); const guessInput = document.getElementById('guess-input'); const submitBtn = document.getElementById('submit-btn'); const message = document.getElementById('message'); function createBoard() { for (let i = 0; i < 6; i++) { // 6 rows for 6 guesses for (let j = 0; j < 5; j++) { // 5 columns for 5 letters const cell = document.createElement('div'); cell.classList.add('cell'); gameBoard.appendChild(cell); } } } function checkGuess(guess) { const cells = gameBoard.querySelectorAll('.cell'); const targetWordArray = targetWord.split(''); const guessArray = guess.split(''); let index = 0; for (let i = 0; i < 5; i++) { if (guessArray[i] === targetWordArray[i]) { cells[index].classList.add('correct'); } else if (targetWordArray.includes(guessArray[i])) { cells[index].classList.add('present'); } else { cells[index].classList.add('absent'); } cells[index].textContent = guessArray[i]; index++; } } function handleSubmit() { const guess = guessInput.value.toLowerCase(); if (guess.length !== 5 || !possibleWords.includes(guess)) { message.textContent = 'Invalid word. Try again.'; return; } checkGuess(guess); guessInput.value = ''; // Additional game logic (e.g., end game if correct guess or number of attempts) can be added here } createBoard(); submitBtn.addEventListener('click', handleSubmit);
Sie können auch direkt klonen: https://github.com/prince-dev007/wordle-game
Lesen Sie mehr über Wordle Game
Das obige ist der detaillierte Inhalt vonErstellen Sie ein Wordle-Spiel in HTML, CSS und JS. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen










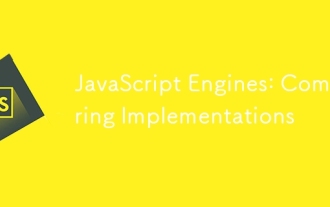
Unterschiedliche JavaScript -Motoren haben unterschiedliche Auswirkungen beim Analysieren und Ausführen von JavaScript -Code, da sich die Implementierungsprinzipien und Optimierungsstrategien jeder Engine unterscheiden. 1. Lexikalanalyse: Quellcode in die lexikalische Einheit umwandeln. 2. Grammatikanalyse: Erzeugen Sie einen abstrakten Syntaxbaum. 3. Optimierung und Kompilierung: Generieren Sie den Maschinencode über den JIT -Compiler. 4. Führen Sie aus: Führen Sie den Maschinencode aus. V8 Engine optimiert durch sofortige Kompilierung und versteckte Klasse.
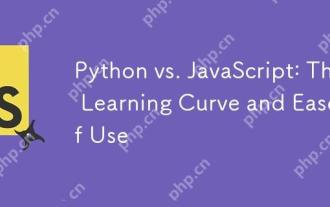
Python eignet sich besser für Anfänger mit einer reibungslosen Lernkurve und einer kurzen Syntax. JavaScript ist für die Front-End-Entwicklung mit einer steilen Lernkurve und einer flexiblen Syntax geeignet. 1. Python-Syntax ist intuitiv und für die Entwicklung von Datenwissenschaften und Back-End-Entwicklung geeignet. 2. JavaScript ist flexibel und in Front-End- und serverseitiger Programmierung weit verbreitet.
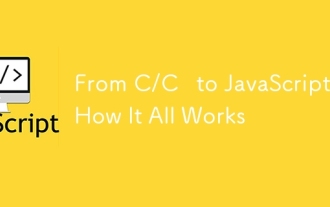
Die Verschiebung von C/C zu JavaScript erfordert die Anpassung an dynamische Typisierung, Müllsammlung und asynchrone Programmierung. 1) C/C ist eine statisch typisierte Sprache, die eine manuelle Speicherverwaltung erfordert, während JavaScript dynamisch eingegeben und die Müllsammlung automatisch verarbeitet wird. 2) C/C muss in den Maschinencode kompiliert werden, während JavaScript eine interpretierte Sprache ist. 3) JavaScript führt Konzepte wie Verschlüsse, Prototypketten und Versprechen ein, die die Flexibilität und asynchrone Programmierfunktionen verbessern.
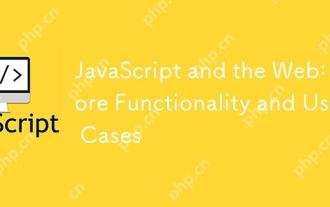
Zu den Hauptanwendungen von JavaScript in der Webentwicklung gehören die Interaktion der Clients, die Formüberprüfung und die asynchrone Kommunikation. 1) Dynamisches Inhaltsaktualisierung und Benutzerinteraktion durch DOM -Operationen; 2) Die Kundenüberprüfung erfolgt vor dem Einreichung von Daten, um die Benutzererfahrung zu verbessern. 3) Die Aktualisierung der Kommunikation mit dem Server wird durch AJAX -Technologie erreicht.
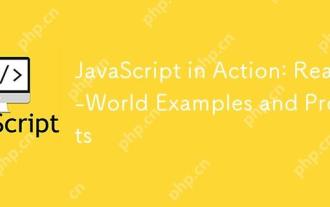
Die Anwendung von JavaScript in der realen Welt umfasst Front-End- und Back-End-Entwicklung. 1) Zeigen Sie Front-End-Anwendungen an, indem Sie eine TODO-Listanwendung erstellen, die DOM-Operationen und Ereignisverarbeitung umfasst. 2) Erstellen Sie RESTFUFFUPI über Node.js und express, um Back-End-Anwendungen zu demonstrieren.
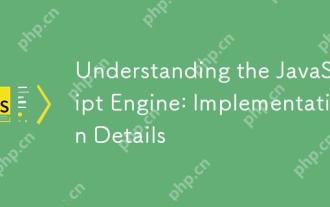
Es ist für Entwickler wichtig, zu verstehen, wie die JavaScript -Engine intern funktioniert, da sie effizientere Code schreibt und Leistungs Engpässe und Optimierungsstrategien verstehen kann. 1) Der Workflow der Engine umfasst drei Phasen: Parsen, Kompilieren und Ausführung; 2) Während des Ausführungsprozesses führt die Engine dynamische Optimierung durch, wie z. B. Inline -Cache und versteckte Klassen. 3) Zu Best Practices gehören die Vermeidung globaler Variablen, die Optimierung von Schleifen, die Verwendung von const und lass und die Vermeidung übermäßiger Verwendung von Schließungen.
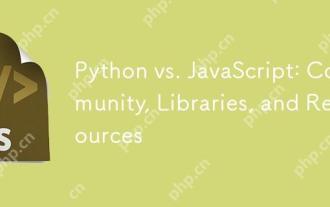
Python und JavaScript haben ihre eigenen Vor- und Nachteile in Bezug auf Gemeinschaft, Bibliotheken und Ressourcen. 1) Die Python-Community ist freundlich und für Anfänger geeignet, aber die Front-End-Entwicklungsressourcen sind nicht so reich wie JavaScript. 2) Python ist leistungsstark in Bibliotheken für Datenwissenschaft und maschinelles Lernen, während JavaScript in Bibliotheken und Front-End-Entwicklungsbibliotheken und Frameworks besser ist. 3) Beide haben reichhaltige Lernressourcen, aber Python eignet sich zum Beginn der offiziellen Dokumente, während JavaScript mit Mdnwebdocs besser ist. Die Wahl sollte auf Projektbedürfnissen und persönlichen Interessen beruhen.
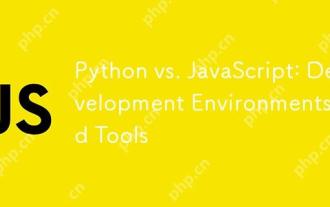
Sowohl Python als auch JavaScripts Entscheidungen in Entwicklungsumgebungen sind wichtig. 1) Die Entwicklungsumgebung von Python umfasst Pycharm, Jupyternotebook und Anaconda, die für Datenwissenschaft und schnelles Prototyping geeignet sind. 2) Die Entwicklungsumgebung von JavaScript umfasst Node.JS, VSCODE und WebPack, die für die Entwicklung von Front-End- und Back-End-Entwicklung geeignet sind. Durch die Auswahl der richtigen Tools nach den Projektbedürfnissen kann die Entwicklung der Entwicklung und die Erfolgsquote der Projekte verbessert werden.
