


Umgang mit Datums- und Zeitzonenkonvertierungen: Warum die richtige UTC-Konvertierung wichtig ist
While retrieving data for a selected date range, we noticed that our calculation was off by some margin. However, when we decreased the date by one day, the data matched exactly!
Hmmm… There might be an issue with how the date is being handled in our code. Perhaps the timezone is not being handled correctly—and yes, I was right!
When building applications that involve users from different timezones, handling dates properly can be tricky. Storing dates in UTC is a common best practice to ensure consistency, but things can get complicated when users input dates in their local timezone, especially during filtering and querying.
Developers often resort to the native JavaScript Date object for handling these conversions. However, this approach can lead to inconsistencies across environments, such as Node.js vs. browser consoles like Chrome. In this article, we’ll explore why handling date and timezone conversions properly is crucial, how Luxon can make this process easier, and why relying on the native JavaScript Date object can lead to inconsistencies.
The Problem: UTC Storage vs. Local Time Filtering
When dates are stored in UTC, they represent a global standard that eliminates the ambiguity caused by timezones. However, users typically think in terms of their local timezone. This discrepancy becomes evident when users try to filter records by date using local time inputs.
Let’s look at an example where a user’s local time inputs could lead to missed records if not handled properly.
Example Scenario: A User in the GMT-7 Timezone
Imagine a user in the GMT-7 timezone (Pacific Daylight Time). On September 5th, 2024, they create a record at 10:00 PM in their local time. Here’s what happens behind the scenes:
- September 5th, 2024, 10:00 PM GMT-7 is converted to September 6th, 2024, 05:00 AM UTC and stored in the database as such.
- The user, however, perceives that they created this record on September 5th.
The Filter Mismatch
Now, suppose the user wants to query all records created on September 5th. They input the date September 5th, 2024, expecting to retrieve their record. However, if the system compares the input date directly to the stored UTC date without adjusting for timezone differences, the user will miss the record. Why?
- The record was saved in the database as September 6th (UTC).
- The user filters for September 5th (their local time), but the system compares this against UTC, resulting in no match.
JavaScript Date Object: Inconsistencies Across Environments
The following example code demonstrates a common problem when using the native JavaScript Date object for handling date and time conversions, particularly across different environments such as Node.js and the browser (e.g., Chrome console).
Example Code:
function convertToUtcStartOfDay(isoString) { // Step 1: Parse the ISO string into a Date object let localDate = new Date(isoString); // Step 2: Set the time to the start of the day (00:00:00) in local time zone localDate.setHours(0, 0, 0, 0); // Step 3: Get the UTC time using toISOString() – it converts local time to UTC let utcStartOfDay = localDate.toISOString(); return utcStartOfDay; // This will be in UTC } // Example usage: let frontendDate = "2023-08-22T00:00:00+05:30"; // ISO string with timezone offset let startOfDayUtc = convertToUtcStartOfDay(frontendDate); console.log(startOfDayUtc); // Expected output: "2023-08-21T18:30:00.000Z"
In this example, the user inputs the date "2023-08-22T00:00:00+05:30" (from a GMT+5:30 timezone). The Date object should convert it to the start of the day in UTC, but when executed:
- In Node.js, the output is 2023-08-21T00:00:00.000Z - Wrong
- In Chrome's console, the output is 2023-08-21T18:30:00.000Z - Correct
This discrepancy can cause unpredictable results depending on where the code is executed. This behavior makes the Date object unreliable for consistent date handling across different environments.
Using Luxon for Accurate Date Handling
To solve this problem, it's important to use a library like Luxon that provides consistent behavior across environments. Luxon helps you convert the user’s local input to the proper start and end of the day in their timezone, and then convert those times to UTC for accurate database queries.
Here’s an example using Luxon to handle this:
const { DateTime } = require('luxon'); // Example user input date in ISO string with timezone information from the frontend const userInputDate = "2023-08-22T00:00:00+05:30"; // ISO string sent by frontend // Step 1: Parse the ISO string to get the user's local time const userLocalDate = DateTime.fromISO(userInputDate); // Step 2: Convert this date to start of the day and end of the day in the user's local timezone const startOfDayLocal = userLocalDate.startOf('day'); // start of the day in the user's timezone const endOfDayLocal = userLocalDate.endOf('day'); // end of the day in the user's timezone // Step 3: Convert these local start and end times to UTC const startOfDayUtc = startOfDayLocal.toUTC().toJSDate(); // start of the day in UTC const endOfDayUtc = endOfDayLocal.toUTC().toJSDate(); // end of the day in UTC // Step 4: Query the database using the UTC range db.records.find({ createdAt: { $gte: startOfDayUtc, $lte: endOfDayUtc } });
Why Luxon is Better than JavaScript Date Objects
Handling date and timezone conversions directly with the native JavaScript Date object can lead to inconsistencies like the one demonstrated above. Here are a few reasons why Luxon is a better alternative:
Cohérence entre les environnements : Luxon fournit un comportement cohérent, que le code soit exécuté dans Node.js ou dans le navigateur (par exemple, la console Chrome). Cela élimine les divergences résultant de l'utilisation de l'objet Date dans différents environnements.
Prise en charge intégrée des fuseaux horaires : Luxon facilite la conversion entre les fuseaux horaires, tandis que l'objet Date n'offre pas de prise en charge robuste pour la manipulation des fuseaux horaires.
Manipulation simple de la date : définir le début ou la fin d'une journée dans le fuseau horaire local de l'utilisateur et le convertir en UTC est une tâche courante dans les applications mondiales. Luxon simplifie ce processus grâce à son API intuitive, tandis que Date nécessite une manipulation manuelle complexe.
Conclusion
Gérer correctement les conversions de date et de fuseau horaire est crucial pour créer des applications fiables et conviviales. Si les développeurs ne tiennent pas compte des différences de fuseau horaire lors du filtrage des enregistrements, les utilisateurs risquent de manquer des données importantes, ce qui entraîne une confusion et des erreurs potentiellement critiques.
L'utilisation de Luxon au lieu de l'objet JavaScript Date natif offre une cohérence, une meilleure gestion des fuseaux horaires et une manipulation plus facile des dates. Cela permet aux développeurs de créer une expérience transparente pour les utilisateurs sur tous les fuseaux horaires, garantissant que les requêtes fonctionnent comme prévu et qu'aucun enregistrement n'est manqué lors du filtrage.
Dans les applications mondiales, une gestion précise et fiable des dates est essentielle pour offrir une expérience de haute qualité aux utilisateurs, quel que soit leur fuseau horaire.
Pensées finales
Avez-vous déjà rencontré une situation similaire dans laquelle la gestion de la date et du fuseau horaire provoquait des résultats inattendus dans votre application ? Comment l’avez-vous abordé ? J’aimerais connaître vos expériences, vos commentaires ou toute question ou préoccupation que vous pourriez avoir. N'hésitez pas à les partager dans la section commentaires ci-dessous. Si vous avez trouvé cet article utile, aimez-le et partagez-le avec d'autres personnes qui pourraient en bénéficier !
Das obige ist der detaillierte Inhalt vonUmgang mit Datums- und Zeitzonenkonvertierungen: Warum die richtige UTC-Konvertierung wichtig ist. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen










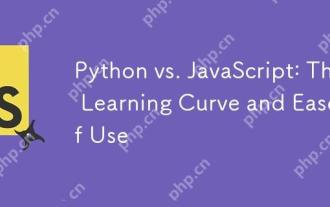
Python eignet sich besser für Anfänger mit einer reibungslosen Lernkurve und einer kurzen Syntax. JavaScript ist für die Front-End-Entwicklung mit einer steilen Lernkurve und einer flexiblen Syntax geeignet. 1. Python-Syntax ist intuitiv und für die Entwicklung von Datenwissenschaften und Back-End-Entwicklung geeignet. 2. JavaScript ist flexibel und in Front-End- und serverseitiger Programmierung weit verbreitet.
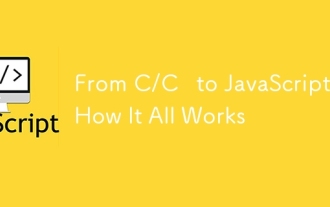
Die Verschiebung von C/C zu JavaScript erfordert die Anpassung an dynamische Typisierung, Müllsammlung und asynchrone Programmierung. 1) C/C ist eine statisch typisierte Sprache, die eine manuelle Speicherverwaltung erfordert, während JavaScript dynamisch eingegeben und die Müllsammlung automatisch verarbeitet wird. 2) C/C muss in den Maschinencode kompiliert werden, während JavaScript eine interpretierte Sprache ist. 3) JavaScript führt Konzepte wie Verschlüsse, Prototypketten und Versprechen ein, die die Flexibilität und asynchrone Programmierfunktionen verbessern.
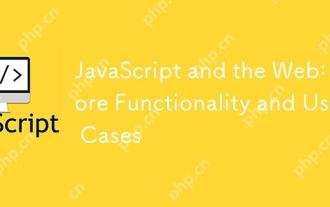
Zu den Hauptanwendungen von JavaScript in der Webentwicklung gehören die Interaktion der Clients, die Formüberprüfung und die asynchrone Kommunikation. 1) Dynamisches Inhaltsaktualisierung und Benutzerinteraktion durch DOM -Operationen; 2) Die Kundenüberprüfung erfolgt vor dem Einreichung von Daten, um die Benutzererfahrung zu verbessern. 3) Die Aktualisierung der Kommunikation mit dem Server wird durch AJAX -Technologie erreicht.
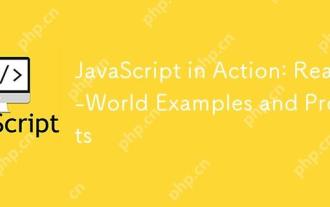
Die Anwendung von JavaScript in der realen Welt umfasst Front-End- und Back-End-Entwicklung. 1) Zeigen Sie Front-End-Anwendungen an, indem Sie eine TODO-Listanwendung erstellen, die DOM-Operationen und Ereignisverarbeitung umfasst. 2) Erstellen Sie RESTFUFFUPI über Node.js und express, um Back-End-Anwendungen zu demonstrieren.
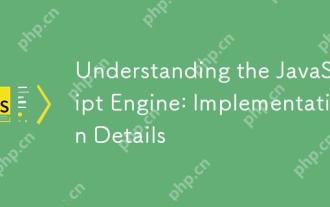
Es ist für Entwickler wichtig, zu verstehen, wie die JavaScript -Engine intern funktioniert, da sie effizientere Code schreibt und Leistungs Engpässe und Optimierungsstrategien verstehen kann. 1) Der Workflow der Engine umfasst drei Phasen: Parsen, Kompilieren und Ausführung; 2) Während des Ausführungsprozesses führt die Engine dynamische Optimierung durch, wie z. B. Inline -Cache und versteckte Klassen. 3) Zu Best Practices gehören die Vermeidung globaler Variablen, die Optimierung von Schleifen, die Verwendung von const und lass und die Vermeidung übermäßiger Verwendung von Schließungen.
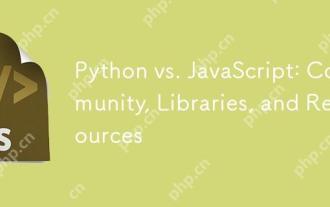
Python und JavaScript haben ihre eigenen Vor- und Nachteile in Bezug auf Gemeinschaft, Bibliotheken und Ressourcen. 1) Die Python-Community ist freundlich und für Anfänger geeignet, aber die Front-End-Entwicklungsressourcen sind nicht so reich wie JavaScript. 2) Python ist leistungsstark in Bibliotheken für Datenwissenschaft und maschinelles Lernen, während JavaScript in Bibliotheken und Front-End-Entwicklungsbibliotheken und Frameworks besser ist. 3) Beide haben reichhaltige Lernressourcen, aber Python eignet sich zum Beginn der offiziellen Dokumente, während JavaScript mit Mdnwebdocs besser ist. Die Wahl sollte auf Projektbedürfnissen und persönlichen Interessen beruhen.
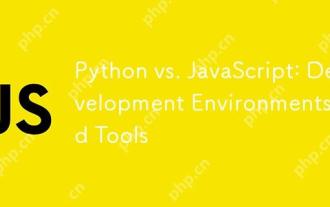
Sowohl Python als auch JavaScripts Entscheidungen in Entwicklungsumgebungen sind wichtig. 1) Die Entwicklungsumgebung von Python umfasst Pycharm, Jupyternotebook und Anaconda, die für Datenwissenschaft und schnelles Prototyping geeignet sind. 2) Die Entwicklungsumgebung von JavaScript umfasst Node.JS, VSCODE und WebPack, die für die Entwicklung von Front-End- und Back-End-Entwicklung geeignet sind. Durch die Auswahl der richtigen Tools nach den Projektbedürfnissen kann die Entwicklung der Entwicklung und die Erfolgsquote der Projekte verbessert werden.
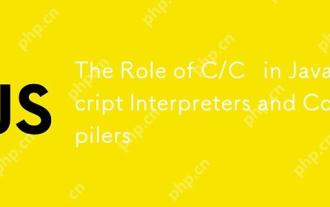
C und C spielen eine wichtige Rolle in der JavaScript -Engine, die hauptsächlich zur Implementierung von Dolmetschern und JIT -Compilern verwendet wird. 1) C wird verwendet, um JavaScript -Quellcode zu analysieren und einen abstrakten Syntaxbaum zu generieren. 2) C ist für die Generierung und Ausführung von Bytecode verantwortlich. 3) C implementiert den JIT-Compiler, optimiert und kompiliert Hot-Spot-Code zur Laufzeit und verbessert die Ausführungseffizienz von JavaScript erheblich.
