


Wie werden sowohl Formular- als auch JSON-Daten in einem einzigen FastAPI-Endpunkt verarbeitet?
Wie erstelle ich einen FastAPI-Endpunkt, der entweder ein Formular oder einen JSON-Text akzeptieren kann?
In FastAPI können Sie einen Endpunkt erstellen, der entweder ein Formular oder einen JSON-Text akzeptieren kann mit unterschiedlichen Methoden. Hier sind einige Optionen:
Option 1: Verwendung einer Abhängigkeitsfunktion
Diese Option beinhaltet das Erstellen einer Abhängigkeitsfunktion, die den Wert des Content-Type-Anfrageheaders überprüft und den Text mithilfe der Methoden von Starlette analysiert , entsprechend.
<code class="python">from fastapi import FastAPI, Depends, Request from starlette.datastructures import FormData app = FastAPI() async def get_body(request: Request): content_type = request.headers.get('Content-Type') if content_type is None: raise HTTPException(status_code=400, detail='No Content-Type provided!') elif content_type == 'application/json': return await request.json() elif (content_type == 'application/x-www-form-urlencoded' or content_type.startswith('multipart/form-data')): try: return await request.form() except Exception: raise HTTPException(status_code=400, detail='Invalid Form data') else: raise HTTPException(status_code=400, detail='Content-Type not supported!') @app.post('/') def main(body = Depends(get_body)): if isinstance(body, dict): # if JSON data received return body elif isinstance(body, FormData): # if Form/File data received msg = body.get('msg') items = body.getlist('items') return msg</code>
Option 2: Separate Endpunkte definieren
Eine andere Option besteht darin, einen einzelnen Endpunkt zu haben und Ihre Datei(en) und/oder Formulardatenparameter als optional zu definieren. Wenn an einen der Parameter Werte übergeben werden, bedeutet dies, dass die Anfrage entweder application/x-www-form-urlencoded oder multipart/form-data war. Andernfalls handelte es sich wahrscheinlich um eine JSON-Anfrage.
<code class="python">from fastapi import FastAPI, UploadFile, File, Form from typing import Optional, List app = FastAPI() @app.post('/') async def submit(items: Optional[List[str]] = Form(None), files: Optional[List[UploadFile]] = File(None)): # if File(s) and/or form-data were received if items or files: filenames = None if files: filenames = [f.filename for f in files] return {'File(s)/form-data': {'items': items, 'filenames': filenames}} else: # check if JSON data were received data = await request.json() return {'JSON': data}</code>
Option 3: Verwendung einer Middleware
Sie können auch eine Middleware verwenden, um die eingehende Anfrage zu überprüfen und sie entweder an /submitJSON oder / umzuleiten. „submitForm“-Endpunkt, abhängig vom Inhaltstyp der Anfrage.
<code class="python">from fastapi import FastAPI, Request from fastapi.responses import JSONResponse app = FastAPI() @app.middleware("http") async def some_middleware(request: Request, call_next): if request.url.path == '/': content_type = request.headers.get('Content-Type') if content_type is None: return JSONResponse( content={'detail': 'No Content-Type provided!'}, status_code=400) elif content_type == 'application/json': request.scope['path'] = '/submitJSON' elif (content_type == 'application/x-www-form-urlencoded' or content_type.startswith('multipart/form-data')): request.scope['path'] = '/submitForm' else: return JSONResponse( content={'detail': 'Content-Type not supported!'}, status_code=400) return await call_next(request) @app.post('/') def main(): return @app.post('/submitJSON') def submit_json(item: Item): return item @app.post('/submitForm') def submit_form(msg: str = Form(...), items: List[str] = Form(...), files: Optional[List[UploadFile]] = File(None)): return msg</code>
Testen der Optionen
Sie können die oben genannten Optionen mit der Anforderungsbibliothek von Python testen:
<code class="python">import requests url = 'http://127.0.0.1:8000/' files = [('files', open('a.txt', 'rb')), ('files', open('b.txt', 'rb'))] payload ={'items': ['foo', 'bar'], 'msg': 'Hello!'} # Send Form data and files r = requests.post(url, data=payload, files=files) print(r.text) # Send Form data only r = requests.post(url, data=payload) print(r.text) # Send JSON data r = requests.post(url, json=payload) print(r.text)</code>
Das obige ist der detaillierte Inhalt vonWie werden sowohl Formular- als auch JSON-Daten in einem einzigen FastAPI-Endpunkt verarbeitet?. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen










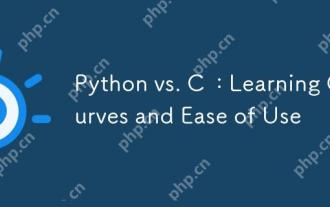
Python ist leichter zu lernen und zu verwenden, während C leistungsfähiger, aber komplexer ist. 1. Python -Syntax ist prägnant und für Anfänger geeignet. Durch die dynamische Tippen und die automatische Speicherverwaltung können Sie die Verwendung einfach zu verwenden, kann jedoch zur Laufzeitfehler führen. 2.C bietet Steuerung und erweiterte Funktionen auf niedrigem Niveau, geeignet für Hochleistungsanwendungen, hat jedoch einen hohen Lernschwellenwert und erfordert manuellem Speicher und Typensicherheitsmanagement.
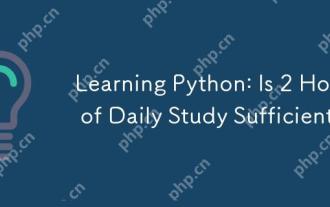
Ist es genug, um Python für zwei Stunden am Tag zu lernen? Es hängt von Ihren Zielen und Lernmethoden ab. 1) Entwickeln Sie einen klaren Lernplan, 2) Wählen Sie geeignete Lernressourcen und -methoden aus, 3) praktizieren und prüfen und konsolidieren Sie praktische Praxis und Überprüfung und konsolidieren Sie und Sie können die Grundkenntnisse und die erweiterten Funktionen von Python während dieser Zeit nach und nach beherrschen.
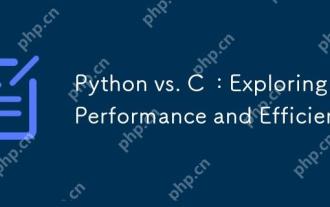
Python ist in der Entwicklungseffizienz besser als C, aber C ist in der Ausführungsleistung höher. 1. Pythons prägnante Syntax und reiche Bibliotheken verbessern die Entwicklungseffizienz. 2. Die Kompilierungsmerkmale von Compilation und die Hardwarekontrolle verbessern die Ausführungsleistung. Bei einer Auswahl müssen Sie die Entwicklungsgeschwindigkeit und die Ausführungseffizienz basierend auf den Projektanforderungen abwägen.
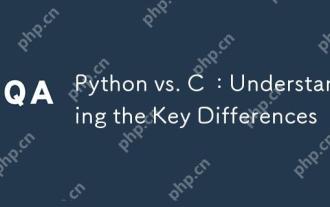
Python und C haben jeweils ihre eigenen Vorteile, und die Wahl sollte auf Projektanforderungen beruhen. 1) Python ist aufgrund seiner prägnanten Syntax und der dynamischen Typisierung für die schnelle Entwicklung und Datenverarbeitung geeignet. 2) C ist aufgrund seiner statischen Tipp- und manuellen Speicherverwaltung für hohe Leistung und Systemprogrammierung geeignet.
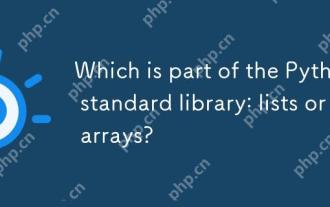
PythonlistsarePartThestandardlibrary, whilearraysarenot.listarebuilt-in, vielseitig und UNDUSEDFORSPORINGECollections, während dieArrayRay-thearrayModulei und loses und loses und losesaluseduetolimitedFunctionality.
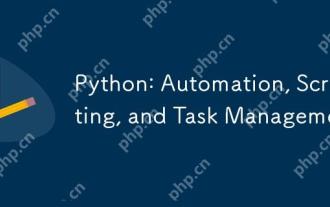
Python zeichnet sich in Automatisierung, Skript und Aufgabenverwaltung aus. 1) Automatisierung: Die Sicherungssicherung wird durch Standardbibliotheken wie OS und Shutil realisiert. 2) Skriptschreiben: Verwenden Sie die PSUTIL -Bibliothek, um die Systemressourcen zu überwachen. 3) Aufgabenverwaltung: Verwenden Sie die Zeitplanbibliothek, um Aufgaben zu planen. Die Benutzerfreundlichkeit von Python und die Unterstützung der reichhaltigen Bibliothek machen es zum bevorzugten Werkzeug in diesen Bereichen.
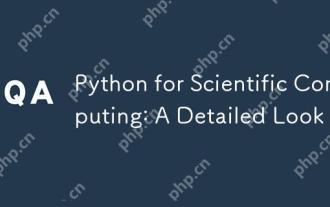
Zu den Anwendungen von Python im wissenschaftlichen Computer gehören Datenanalyse, maschinelles Lernen, numerische Simulation und Visualisierung. 1.Numpy bietet effiziente mehrdimensionale Arrays und mathematische Funktionen. 2. Scipy erweitert die Numpy -Funktionalität und bietet Optimierungs- und lineare Algebra -Tools. 3.. Pandas wird zur Datenverarbeitung und -analyse verwendet. 4.Matplotlib wird verwendet, um verschiedene Grafiken und visuelle Ergebnisse zu erzeugen.
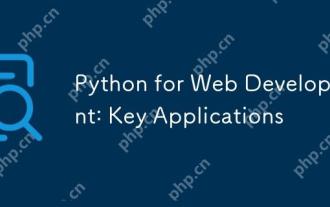
Zu den wichtigsten Anwendungen von Python in der Webentwicklung gehören die Verwendung von Django- und Flask -Frameworks, API -Entwicklung, Datenanalyse und Visualisierung, maschinelles Lernen und KI sowie Leistungsoptimierung. 1. Django und Flask Framework: Django eignet sich für die schnelle Entwicklung komplexer Anwendungen, und Flask eignet sich für kleine oder hochmobile Projekte. 2. API -Entwicklung: Verwenden Sie Flask oder Djangorestframework, um RESTFUFFUPI zu erstellen. 3. Datenanalyse und Visualisierung: Verwenden Sie Python, um Daten zu verarbeiten und über die Webschnittstelle anzuzeigen. 4. Maschinelles Lernen und KI: Python wird verwendet, um intelligente Webanwendungen zu erstellen. 5. Leistungsoptimierung: optimiert durch asynchrones Programmieren, Caching und Code
