Versprechen/A und Async warten auf Sie – JavaScript-Herausforderungen
Sie finden den gesamten Code in diesem Beitrag im Repo Github.
Async-Programmierung Promises/A und Async warten auf entsprechende Herausforderungen
Implementieren Sie Promises/A mit Promise.finally()
class MyPromise { constructor(executor) { this.state = 'pending'; this.value = undefined; this.reason = undefined; this.onFulfilledCallbacks = []; this.onRejectedCallbacks = []; const resolve = (value) => { if (this.state === 'pending') { this.state = 'fulfilled'; this.value = value; this.onFulfilledCallbacks.forEach((callbackFn) => callbackFn(this.value)); } } const reject = (reason) => { if (this.state === 'pending') { this.state = 'rejected'; this.reason = reason; this.onRejectedCallbacks.forEach((callbackFn) => callbackFn(this.reason)); } } try { executor(resolve, reject); } catch (err) { reject(err); } } handlePromiseResult(result, resolve, reject) { if (result instanceof MyPromise) { result.then(resolve, reject); } else { resolve(result); } } then(onFulfilled, onRejected) { onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : (value) => value; onRejected = typeof onRejected === 'function' ? onRejected : (reason) => { throw reason; }; // return a promise return new MyPromise((resolve, reject) => { const fulfilledHandler = () => { queueMicrotask(() => { try { const result = onFulfilled(this.value); this.handlePromiseResult(result, resolve, reject); } catch (err) { reject(err); } }); }; const rejectedHandler = () => { queueMicrotask(() => { try { const result = onRejected(this.reason); this.handlePromiseResult(result, resolve, reject); } catch (err) { reject(err); } }); }; if (this.state === 'fulfilled') { fulfilledHandler(); } else if (this.state === 'rejected') { rejectedHandler(); } else { this.onFulfilledCallbacks.push(fulfilledHandler); this.onRejectedCallbacks.push(rejectedHandler); } }); } catch(onRejected) { return this.then(null, onRejected); } finally(onFinally) { if (typeof onFinally !== 'function') { return this.then(); } return this.then( (value) => MyPromise.resolve((onFinally()).then(() => value)), (reason) => MyPromise.resolve(onFinally()).then(() => { throw reason }) ); } static resolve(value) { return new MyPromise((resolve) => resolve(value)); } static reject(reason) { return new MyPromise((_, reject) => reject(reason)); } } // Usage example const promise = MyPromise.resolve(1); promise.then((value) => { console.log(value); }) .then(() => { throw new Error('Error'); }) .catch((err) => { console.log(`Error catched: ${err}`); });
Implementieren Sie asynchrones Warten mit Generator
function asyncGenerator(generatorFunc) { return function (...args) { const generator = generatorFunc(...args); return new Promise((resolve, reject) => { function handle(iteratorResult) { if (iteratorResult.done) { resolve(iteratorResult.value); return; } Promise.resolve(iteratorResult.value) .then( (value) => handle(generator.next(value)), (err) => handle(generator.throw(err)), ); } try { handle(generator.next()); } catch (err) { reject(err); } }); } } // Usage example function* fetchData() { const data1 = yield fetch('https://jsonplaceholder.typicode.com/posts/1').then(res => res.json()); console.log(data1); const data2 = yield fetch('https://jsonplaceholder.typicode.com/posts/2').then(res => res.json()); console.log(data2); } // Create an async version of the generator function const asyncFetchData = asyncGenerator(fetchData); // Call the async function asyncFetchData() .then(() => console.log('All data fetched!')) .catch(err => console.error('Error:', err));
Referenz
- 67. Erstellen Sie Ihr eigenes Versprechen – BFE.dev
- 123. Implementieren Sie Promise.prototype.finally() - BFE.dev
- Versprochen – MDN
- asynchrone Funktion – MDN
- Versprechen/A
Das obige ist der detaillierte Inhalt vonVersprechen/A und Async warten auf Sie – JavaScript-Herausforderungen. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen










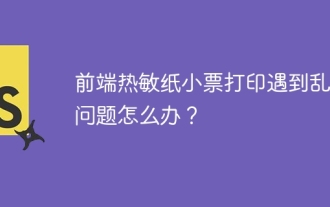
Häufig gestellte Fragen und Lösungen für das Ticket-Ticket-Ticket-Ticket in Front-End im Front-End-Entwicklungsdruck ist der Ticketdruck eine häufige Voraussetzung. Viele Entwickler implementieren jedoch ...
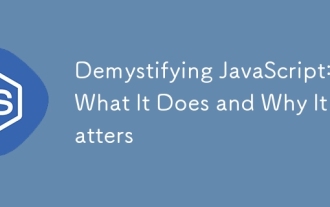
JavaScript ist der Eckpfeiler der modernen Webentwicklung. Zu den Hauptfunktionen gehören eine ereignisorientierte Programmierung, die Erzeugung der dynamischen Inhalte und die asynchrone Programmierung. 1) Ereignisgesteuerte Programmierung ermöglicht es Webseiten, sich dynamisch entsprechend den Benutzeroperationen zu ändern. 2) Die dynamische Inhaltsgenerierung ermöglicht die Anpassung der Seiteninhalte gemäß den Bedingungen. 3) Asynchrone Programmierung stellt sicher, dass die Benutzeroberfläche nicht blockiert ist. JavaScript wird häufig in der Webinteraktion, der einseitigen Anwendung und der serverseitigen Entwicklung verwendet, wodurch die Flexibilität der Benutzererfahrung und die plattformübergreifende Entwicklung erheblich verbessert wird.
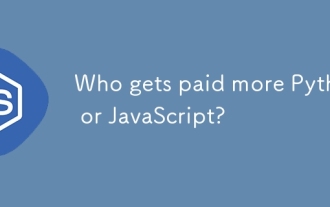
Es gibt kein absolutes Gehalt für Python- und JavaScript -Entwickler, je nach Fähigkeiten und Branchenbedürfnissen. 1. Python kann mehr in Datenwissenschaft und maschinellem Lernen bezahlt werden. 2. JavaScript hat eine große Nachfrage in der Entwicklung von Front-End- und Full-Stack-Entwicklung, und sein Gehalt ist auch beträchtlich. 3. Einflussfaktoren umfassen Erfahrung, geografische Standort, Unternehmensgröße und spezifische Fähigkeiten.
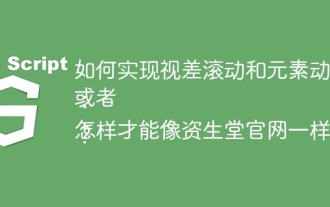
Diskussion über die Realisierung von Parallaxe -Scrolling- und Elementanimationseffekten in diesem Artikel wird untersuchen, wie die offizielle Website der Shiseeido -Website (https://www.shiseeido.co.jp/sb/wonderland/) ähnlich ist ...
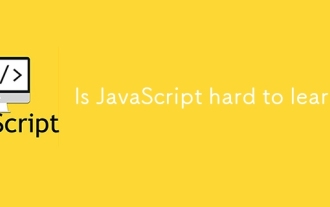
JavaScript zu lernen ist nicht schwierig, aber es ist schwierig. 1) Verstehen Sie grundlegende Konzepte wie Variablen, Datentypen, Funktionen usw. 2) Beherrschen Sie die asynchrone Programmierung und implementieren Sie sie durch Ereignisschleifen. 3) Verwenden Sie DOM -Operationen und versprechen Sie, asynchrone Anfragen zu bearbeiten. 4) Vermeiden Sie häufige Fehler und verwenden Sie Debugging -Techniken. 5) Die Leistung optimieren und Best Practices befolgen.
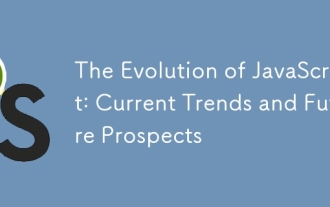
Zu den neuesten Trends im JavaScript gehören der Aufstieg von Typenkripten, die Popularität moderner Frameworks und Bibliotheken und die Anwendung der WebAssembly. Zukunftsaussichten umfassen leistungsfähigere Typsysteme, die Entwicklung des serverseitigen JavaScript, die Erweiterung der künstlichen Intelligenz und des maschinellen Lernens sowie das Potenzial von IoT und Edge Computing.
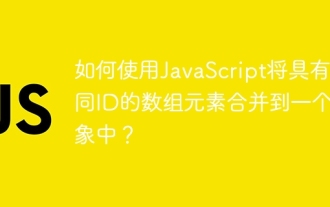
Wie fusioniere ich Array -Elemente mit derselben ID in ein Objekt in JavaScript? Bei der Verarbeitung von Daten begegnen wir häufig die Notwendigkeit, dieselbe ID zu haben ...
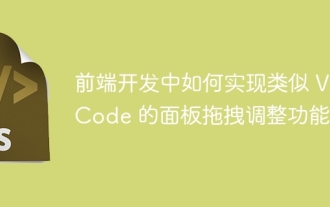
Erforschen Sie die Implementierung der Funktion des Bedien- und Drop-Einstellungsfunktion der Panel ähnlich wie VSCODE im Front-End. In der Front-End-Entwicklung wird VSCODE ähnlich wie VSCODE implementiert ...
