Wie lade ich Dateien über cURL in PHP hoch?
Nov 07, 2024 pm 01:42 PMDateien über cURL in PHP hochladen
Problem
Verarbeitung von Datei-Uploads aus einem Formular-POST in PHP und das Senden mit cURL kann eine Herausforderung sein. Das HTML-Formular verwendet die Kodierung multipart/form-data, aber das genaue Format zum Hochladen von Dateien in einer cURL-Anfrage bleibt unklar.
Lösung
So laden Sie Dateien mit cURL hoch Führen Sie in PHP die folgenden Schritte aus:
Erstellen der cURL-Anfrage
<?php // Define the file to be uploaded $fileKey = 'image'; $tmpFile = $_FILES[$fileKey]['tmp_name']; $fileName = $_FILES[$fileKey]['name']; // Initialize cURL $ch = curl_init(); // Set cURL options curl_setopt_array($ch, [ CURLOPT_URL => 'https://your-api-endpoint.com', // Replace with your API endpoint CURLOPT_USERPWD => 'username:password', // Replace with your API credentials CURLOPT_UPLOAD => true, CURLOPT_INFILE => fopen($tmpFile, 'r'), // Open the file for reading CURLOPT_INFILESIZE => filesize($tmpFile), ]); **Sending the Request** // Execute the cURL request $response = curl_exec($ch); if (curl_errno($ch)) { echo 'Error sending file: ' . curl_error($ch); } else { // Handle the response echo 'File uploaded successfully.'; } // Close the cURL connection curl_close($ch); ?>
Empfangen der Datei
Für den Empfang Skript (curl_receiver.php) können Sie den folgenden Code verwenden:
<?php // Get the file data $file = fopen('php://input', 'rb'); // Save the file to a temporary location $tempFile = tempnam(sys_get_temp_dir(), 'file'); file_put_contents($tempFile, $file); // Do something with the file // ... // Clean up fclose($file); unlink($tempFile); ?>
Mit diesen Schritten können Sie mithilfe von cURL erfolgreich Dateien aus einem Formular-POST in PHP hochladen.
Das obige ist der detaillierte Inhalt vonWie lade ich Dateien über cURL in PHP hoch?. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heißer Artikel

Hot-Tools-Tags

Heißer Artikel

Heiße Artikel -Tags

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen
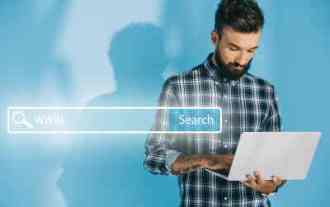
11 beste PHP -URL -Shortener -Skripte (kostenlos und Premium)
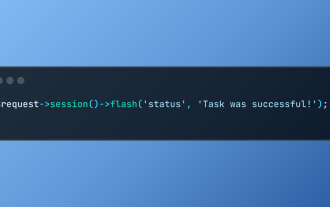
Arbeiten mit Flash -Sitzungsdaten in Laravel
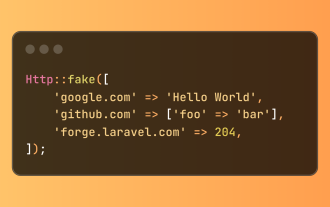
Vereinfachte HTTP -Reaktion verspottet in Laravel -Tests
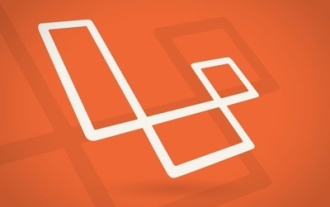
Erstellen Sie eine React -App mit einem Laravel -Back -Ende: Teil 2, reagieren
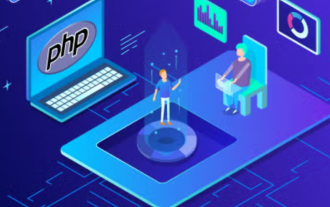
Curl in PHP: So verwenden Sie die PHP -Curl -Erweiterung in REST -APIs
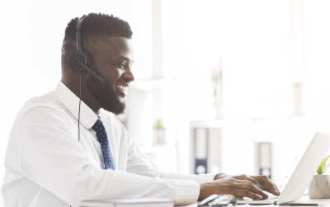
12 Beste PHP -Chat -Skripte auf Codecanyon
