JavaScript-Datumsobjekt-Spickzettel
Das Date-Objekt in JavaScript wird zum Arbeiten mit Datums- und Uhrzeitangaben verwendet. Es bietet Methoden zum Erstellen, Bearbeiten und Formatieren von Datums- und Uhrzeitwerten.
Termine erstellen
Sie können ein Datumsobjekt auf verschiedene Arten erstellen:
- Aktuelles Datum und aktuelle Uhrzeit:
const now = new Date(); console.log(now); // Current date and time
- Spezifisches Datum:
const specificDate = new Date(2024, 10, 21); // Year, Month (0-based), Day console.log(specificDate); // Thu Nov 21 2024
- Aus einer Zeichenfolge:
const fromString = new Date("2024-11-21T10:00:00"); console.log(fromString); // Thu Nov 21 2024 10:00:00 GMT
- Aus Zeitstempeln (Millisekunden seit der Unix-Epoche):
const fromTimestamp = new Date(1732231200000); console.log(fromTimestamp); // Thu Nov 21 2024 10:00:00 GMT
Gemeinsame Methoden
Datum und Uhrzeit abrufen
Method | Description | Example |
---|---|---|
getFullYear() | Returns the year | date.getFullYear() -> 2024 |
getMonth() | Returns the month (0-11) | date.getMonth() -> 10 (November) |
getDate() | Returns the day of the month (1-31) | date.getDate() -> 21 |
getDay() | Returns the weekday (0-6, Sun=0) | date.getDay() -> 4 (Thursday) |
getHours() | Returns the hour (0-23) | date.getHours() -> 10 |
getMinutes() | Returns the minutes (0-59) | date.getMinutes() -> 0 |
getSeconds() | Returns the seconds (0-59) | date.getSeconds() -> 0 |
getTime() | Returns timestamp in milliseconds | date.getTime() -> 1732231200000 |
Beschreibung
Method | Description | Example |
---|---|---|
setFullYear(year) | Sets the year | date.setFullYear(2025) |
setMonth(month) | Sets the month (0-11) | date.setMonth(0) -> January |
setDate(day) | Sets the day of the month | date.setDate(1) -> First day of the month |
setHours(hour) | Sets the hour (0-23) | date.setHours(12) |
setMinutes(minutes) | Sets the minutes (0-59) | date.setMinutes(30) |
setSeconds(seconds) | Sets the seconds (0-59) | date.setSeconds(45) |
Datumsangaben formatieren
Method | Description | Example |
---|---|---|
toDateString() | Returns date as a human-readable string | date.toDateString() -> "Thu Nov 21 2024" |
toISOString() | Returns date in ISO format | date.toISOString() -> "2024-11-21T10:00:00.000Z" |
toLocaleDateString() | Returns date in localized format | date.toLocaleDateString() -> "11/21/2024" |
toLocaleTimeString() | Returns time in localized format | date.toLocaleTimeString() -> "10:00:00 AM" |
Beschreibung
- Häufige Anwendungsfälle
const now = new Date(); console.log(now); // Current date and time
- Tage zwischen zwei Daten berechnen
- :
const specificDate = new Date(2024, 10, 21); // Year, Month (0-based), Day console.log(specificDate); // Thu Nov 21 2024
- Countdown-Timer
- :
const fromString = new Date("2024-11-21T10:00:00"); console.log(fromString); // Thu Nov 21 2024 10:00:00 GMT
- Aktuelles Datum formatieren
- :
const fromTimestamp = new Date(1732231200000); console.log(fromTimestamp); // Thu Nov 21 2024 10:00:00 GMT
- Finden Sie den Wochentag
- :
const startDate = new Date("2024-11-01"); const endDate = new Date("2024-11-21"); const diffInTime = endDate - startDate; // Difference in milliseconds const diffInDays = diffInTime / (1000 * 60 * 60 * 24); // Convert to days console.log(diffInDays); // 20
- Überprüfen Sie das Schaltjahr
- :
const targetDate = new Date("2024-12-31T23:59:59"); setInterval(() => { const now = new Date(); const timeLeft = targetDate - now; // Milliseconds left const days = Math.floor(timeLeft / (1000 * 60 * 60 * 24)); const hours = Math.floor((timeLeft / (1000 * 60 * 60)) % 24); const minutes = Math.floor((timeLeft / (1000 * 60)) % 60); const seconds = Math.floor((timeLeft / 1000) % 60); console.log(`${days}d ${hours}h ${minutes}m ${seconds}s`); }, 1000);
Tage hinzufügen/subtrahieren
:
- Profi-Tipps
const now = new Date(); const formatted = `${now.getFullYear()}-${now.getMonth() + 1}-${now.getDate()}`; console.log(formatted); // "2024-11-21"
- Date.now()
- , um den aktuellen Zeitstempel direkt abzurufen, ohne ein Date-Objekt zu erstellen:
Achten Sie auf Zeitzonen
, wenn Sie mit Datumsangaben in verschiedenen Regionen arbeiten. Verwenden Sie Bibliotheken wie Moment.js - oder
Day.js für eine erweiterte Handhabung.
0-indiziert sind
Das obige ist der detaillierte Inhalt vonJavaScript-Datumsobjekt-Spickzettel. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen
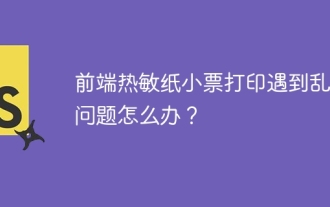
Häufig gestellte Fragen und Lösungen für das Ticket-Ticket-Ticket-Ticket in Front-End im Front-End-Entwicklungsdruck ist der Ticketdruck eine häufige Voraussetzung. Viele Entwickler implementieren jedoch ...
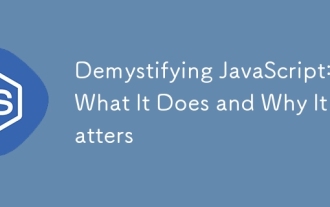
JavaScript ist der Eckpfeiler der modernen Webentwicklung. Zu den Hauptfunktionen gehören eine ereignisorientierte Programmierung, die Erzeugung der dynamischen Inhalte und die asynchrone Programmierung. 1) Ereignisgesteuerte Programmierung ermöglicht es Webseiten, sich dynamisch entsprechend den Benutzeroperationen zu ändern. 2) Die dynamische Inhaltsgenerierung ermöglicht die Anpassung der Seiteninhalte gemäß den Bedingungen. 3) Asynchrone Programmierung stellt sicher, dass die Benutzeroberfläche nicht blockiert ist. JavaScript wird häufig in der Webinteraktion, der einseitigen Anwendung und der serverseitigen Entwicklung verwendet, wodurch die Flexibilität der Benutzererfahrung und die plattformübergreifende Entwicklung erheblich verbessert wird.
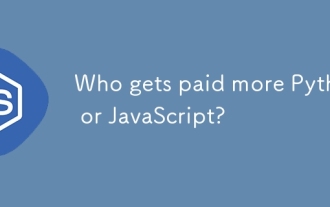
Es gibt kein absolutes Gehalt für Python- und JavaScript -Entwickler, je nach Fähigkeiten und Branchenbedürfnissen. 1. Python kann mehr in Datenwissenschaft und maschinellem Lernen bezahlt werden. 2. JavaScript hat eine große Nachfrage in der Entwicklung von Front-End- und Full-Stack-Entwicklung, und sein Gehalt ist auch beträchtlich. 3. Einflussfaktoren umfassen Erfahrung, geografische Standort, Unternehmensgröße und spezifische Fähigkeiten.
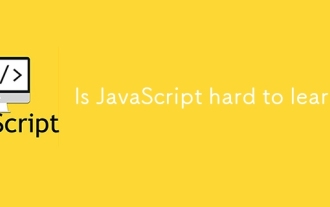
JavaScript zu lernen ist nicht schwierig, aber es ist schwierig. 1) Verstehen Sie grundlegende Konzepte wie Variablen, Datentypen, Funktionen usw. 2) Beherrschen Sie die asynchrone Programmierung und implementieren Sie sie durch Ereignisschleifen. 3) Verwenden Sie DOM -Operationen und versprechen Sie, asynchrone Anfragen zu bearbeiten. 4) Vermeiden Sie häufige Fehler und verwenden Sie Debugging -Techniken. 5) Die Leistung optimieren und Best Practices befolgen.
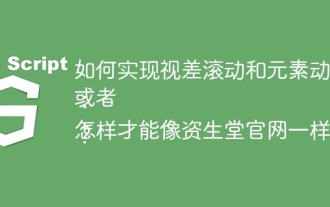
Diskussion über die Realisierung von Parallaxe -Scrolling- und Elementanimationseffekten in diesem Artikel wird untersuchen, wie die offizielle Website der Shiseeido -Website (https://www.shiseeido.co.jp/sb/wonderland/) ähnlich ist ...
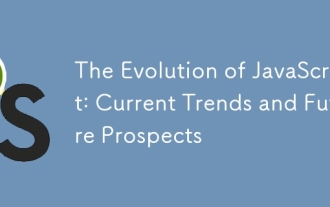
Zu den neuesten Trends im JavaScript gehören der Aufstieg von Typenkripten, die Popularität moderner Frameworks und Bibliotheken und die Anwendung der WebAssembly. Zukunftsaussichten umfassen leistungsfähigere Typsysteme, die Entwicklung des serverseitigen JavaScript, die Erweiterung der künstlichen Intelligenz und des maschinellen Lernens sowie das Potenzial von IoT und Edge Computing.
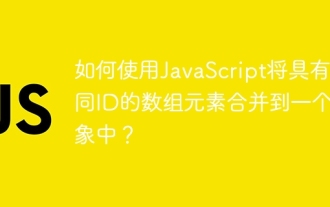
Wie fusioniere ich Array -Elemente mit derselben ID in ein Objekt in JavaScript? Bei der Verarbeitung von Daten begegnen wir häufig die Notwendigkeit, dieselbe ID zu haben ...
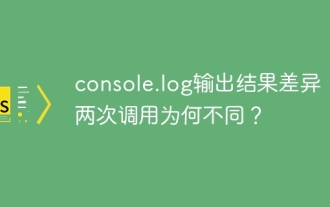
Eingehende Diskussion der Ursachen des Unterschieds in der Konsole.log-Ausgabe. In diesem Artikel wird die Unterschiede in den Ausgabeergebnissen der Konsolenfunktion in einem Code analysiert und die Gründe dafür erläutert. � ...
