


Wie können „wait()' und „notify()' verwendet werden, um eine Blockierungswarteschlange in Java zu implementieren?
Verwenden von wait() und notify() zum Implementieren einer Blockierungswarteschlange
Einführung
In der Multithread-Programmierung sind es wait() und notify() Wird für die Thread-Synchronisation verwendet. In diesem Artikel wird erklärt, wie Sie mit wait() und notify() eine Blockierungswarteschlange implementieren, eine Datenstruktur, die es Threads ermöglicht, zu blockieren, bis ein Element verfügbar ist oder Platz frei wird.
Implementieren einer Blockierungswarteschlange mit wait( ) und notify()
Bedingungen für die Sperrung:
- put() Methode: Blockiert, bis freier Platz in der Warteschlange ist.
- take()-Methode: Blockiert, bis ein Element in der Warteschlange verfügbar ist.
Java-Code:
public class BlockingQueue<T> { private Queue<T> queue = new LinkedList<>(); private int capacity; public BlockingQueue(int capacity) { this.capacity = capacity; } public synchronized void put(T element) throws InterruptedException { while (queue.size() == capacity) { wait(); } queue.add(element); notify(); // notifyAll() for multiple producer/consumer threads } public synchronized T take() throws InterruptedException { while (queue.isEmpty()) { wait(); } T item = queue.remove(); notify(); // notifyAll() for multiple producer/consumer threads return item; } }
Überlegungen bei der Verwendung von wait() und notify()
- Synchronisierter Code: Rufen Sie wait() und notify() innerhalb einer synchronisierten Methode oder eines synchronisierten Blocks auf.
- While-Schleifen: Verwenden Sie while-Schleifen anstelle von if-Anweisungen, um Bedingungen aufgrund falscher Aktivierungen zu überprüfen.
Java 1.5 Parallelitätsbibliothek
Java 1.5 führte eine Parallelitätsbibliothek ein, die Abstraktionen auf höherer Ebene bereitstellt:
Modifizierte Blockierungswarteschlangenimplementierung:
public class BlockingQueue<T> { private Queue<T> queue = new LinkedList<>(); private int capacity; private Lock lock = new ReentrantLock(); private Condition notFull = lock.newCondition(); private Condition notEmpty = lock.newCondition(); public BlockingQueue(int capacity) { this.capacity = capacity; } public void put(T element) throws InterruptedException { lock.lock(); try { while (queue.size() == capacity) { notFull.await(); } queue.add(element); notEmpty.signal(); } finally { lock.unlock(); } } public T take() throws InterruptedException { lock.lock(); try { while (queue.isEmpty()) { notEmpty.await(); } T item = queue.remove(); notFull.signal(); return item; } finally { lock.unlock(); } } }
Das obige ist der detaillierte Inhalt vonWie können „wait()' und „notify()' verwendet werden, um eine Blockierungswarteschlange in Java zu implementieren?. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen










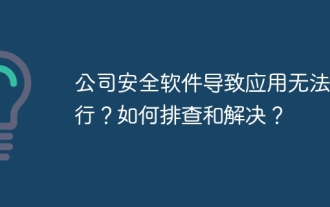
Fehlerbehebung und Lösungen für die Sicherheitssoftware des Unternehmens, die dazu führt, dass einige Anwendungen nicht ordnungsgemäß funktionieren. Viele Unternehmen werden Sicherheitssoftware bereitstellen, um die interne Netzwerksicherheit zu gewährleisten. ...
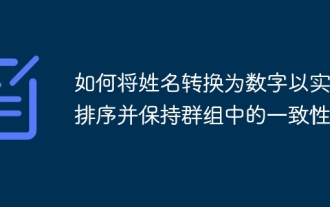
Lösungen zum Umwandeln von Namen in Zahlen zur Implementierung der Sortierung in vielen Anwendungsszenarien müssen Benutzer möglicherweise in Gruppen sortieren, insbesondere in einem ...
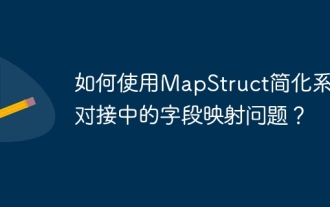
Die Verarbeitung von Feldzuordnungen im Systemdocken stößt häufig auf ein schwieriges Problem bei der Durchführung von Systemdocken: So kartieren Sie die Schnittstellenfelder des Systems und ...
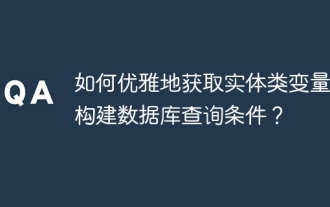
Bei Verwendung von MyBatis-Plus oder anderen ORM-Frameworks für Datenbankvorgänge müssen häufig Abfragebedingungen basierend auf dem Attributnamen der Entitätsklasse erstellt werden. Wenn Sie jedes Mal manuell ...
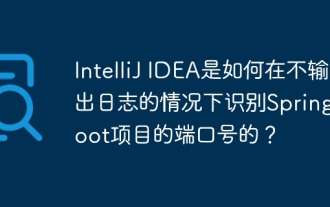
Beginnen Sie den Frühling mit der Intellijideaultimate -Version ...
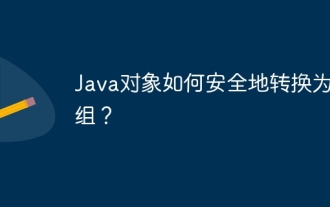
Konvertierung von Java-Objekten und -Arrays: Eingehende Diskussion der Risiken und korrekten Methoden zur Konvertierung des Guss-Typs Viele Java-Anfänger werden auf die Umwandlung eines Objekts in ein Array stoßen ...
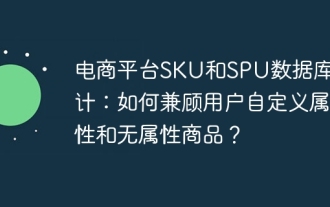
Detaillierte Erläuterung des Designs von SKU- und SPU-Tabellen auf E-Commerce-Plattformen In diesem Artikel werden die Datenbankdesignprobleme von SKU und SPU in E-Commerce-Plattformen erörtert, insbesondere wie man mit benutzerdefinierten Verkäufen umgeht ...
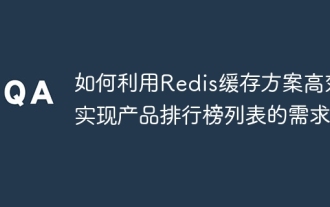
Wie erkennt die Redis -Caching -Lösung die Anforderungen der Produktranking -Liste? Während des Entwicklungsprozesses müssen wir uns häufig mit den Anforderungen der Ranglisten befassen, z. B. das Anzeigen eines ...
