简易 Javascript 调试包 Debug包_javascript技巧
来看一个简易的 Javascript 调试包:jscript.debug.js,包含两个函数,第一个用来遍历对象的各个属性;第二个是一个通用的 Debug 函数(其实 说‘对象'比较‘精确些',呵呵),用来规定各种错误级别及其各种提示、错误信息的格式化显示,还是《Javascript 实战》上面的经典例子,先看源码:
/**
* jscript.debug package
* This package contains utility functions for helping debug JavaScript.
*
*/
/*命名空间*/
if (typeof jscript == 'undefined') {
jscript = function() { }
}
jscript.debug = function() { }
/**
* This simple function is one of the handiest: pass it an object, and it
* will pop an alert() listing all the properties of the object and their
* values.(这个函数用来遍历对象的属性及其相应的值,并显示出来)
*
* @param inObj The object to display properties of.
*/
jscript.debug.enumProps = function(inObj) {
var props = "";
var i;
for (i in inObj) {
props += i + " = " + inObj[i] + "\n";
}
alert(props);
} // End enumProps().
/**
* This is a very simple logger that sends all log messages to a specified
* DIV.(这是一个简单的 debug 日志记录系统)
*/
jscript.debug.DivLogger = function() {
/**
* The following are faux constants that define the various levels a log
* instance can be set to output.(下面的常量用来定义错误级别)
*/
this.LEVEL_TRACE = 1;
this.LEVEL_DEBUG = 2;
this.LEVEL_INFO = 3;
this.LEVEL_WARN = 4;
this.LEVEL_ERROR = 5;
this.LEVEL_FATAL = 6;
/**
* These are the font colors for each logging level.(定义各种错误的显示颜色)
*/
this.LEVEL_TRACE_COLOR = "a0a000";
this.LEVEL_DEBUG_COLOR = "64c864";
this.LEVEL_INFO_COLOR = "000000";
this.LEVEL_WARN_COLOR = "0000ff";
this.LEVEL_ERROR_COLOR = "ff8c00";
this.LEVEL_FATAL_COLOR = "ff0000";
/**
* logLevel determines the minimum message level the instance will show.(需要显示的最小错误级别,默认为 3)
*/
this.logLevel = 3;
/**
* targetDIV is the DIV object to output to.
*/
this.targetDiv = null;
/**
* This function is used to set the minimum level a log instance will show.
*(在这里定义需要显示的最小错误级别)
* @param inLevel One of the level constants. Any message at this level
* or a higher level will be displayed, others will not.
*/
this.setLevel = function(inLevel) {
this.logLevel = inLevel;
} // End setLevel().
/**
* This function is used to set the target DIV that all messages are
* written to. Note that when you call this, the DIV's existing contents
* are cleared out.(设置信息显示的 DIV,调用此函数的时候,原有的信息将被清除)
*
* @param inTargetDiv The DIV object that all messages are written to.
*/
this.setTargetDiv = function(inTargetDiv) {
this.targetDiv = inTargetDiv;
this.targetDiv.innerHTML = "";
} // End setTargetDiv().
/**
* This function is called to determine if a particular message meets or
* exceeds the current level of the log instance and should therefore be
* logged.(此函数用来判定现有的错误级别是否应该被显示)
*
* @param inLevel The level of the message being checked.
*/
this.shouldBeLogged = function(inLevel) {
if (inLevel >= this.logLevel) {
return true;
} else {
return false;
}
} // End shouldBeLogged().
/**
* This function logs messages at TRACE level.
*(格式化显示 TRACE 的错误级别信息,往依此类推)
* @param inMessage The message to log.
*/
this.trace = function(inMessage) {
if (this.shouldBeLogged(this.LEVEL_TRACE) && this.targetDiv) {
this.targetDiv.innerHTML +=
"
"[TRACE] " + inMessage + "
}
} // End trace().
/**
* This function logs messages at DEBUG level.
*
* @param inMessage The message to log.
*/
this.debug = function(inMessage) {
if (this.shouldBeLogged(this.LEVEL_DEBUG) && this.targetDiv) {
this.targetDiv.innerHTML +=
"
"[DEBUG] " + inMessage + "
}
} // End debug().
/**
* This function logs messages at INFO level.
*
* @param inMessage The message to log.
*/
this.info = function(inMessage) {
if (this.shouldBeLogged(this.LEVEL_INFO) && this.targetDiv) {
this.targetDiv.innerHTML +=
"
"[INFO] " + inMessage + "
}
} // End info().
/**
* This function logs messages at WARN level.
*
* @param inMessage The message to log.
*/
this.warn = function(inMessage) {
if (this.shouldBeLogged(this.LEVEL_WARN) && this.targetDiv) {
this.targetDiv.innerHTML +=
"
"[WARN] " + inMessage + "
}
} // End warn().
/**
* This function logs messages at ERROR level.
*
* @param inMessage The message to log.
*/
this.error = function(inMessage) {
if (this.shouldBeLogged(this.LEVEL_ERROR) && this.targetDiv) {
this.targetDiv.innerHTML +=
"
"[ERROR] " + inMessage + "
}
} // End error().
/**
* This function logs messages at FATAL level.
*
* @param inMessage The message to log.
*/
this.fatal = function(inMessage) {
if (this.shouldBeLogged(this.LEVEL_FATAL) && this.targetDiv) {
this.targetDiv.innerHTML +=
"
"[FATAL] " + inMessage + "
}
} // End fatal().
} // End DivLogger().
源码看完后,我们来测试一下这个“小包”,来看下面的测试源码:
上面的测试代码里面的 <script> 段进行了 debug 的实例化,设置了显示信息的 DIV,而且设置了显示信息的最小级别为:LEVEL_DEBUG: <BR>var log = new jscript.debug.DivLogger(); <BR>log.setTargetDiv(document.getElementById("divLog")); <BR>log.setLevel(log.LEVEL_DEBUG); <BR>来看看效果如何呢: <BR><div class="htmlarea"><TEXTAREA id="runcode52315"> <script type="text/javascript">// <![CDATA[ if (typeof jscript == 'undefined') { jscript = function() { } } jscript.debug = function() { } jscript.debug.enumProps = function(inObj) { var props = ""; var i; for (i in inObj) { props += i + " = " + inObj[i] + "\n"; } alert(props); } // End enumProps(). jscript.debug.DivLogger = function() { this.LEVEL_TRACE = 1; this.LEVEL_DEBUG = 2; this.LEVEL_INFO = 3; this.LEVEL_WARN = 4; this.LEVEL_ERROR = 5; this.LEVEL_FATAL = 6; this.LEVEL_TRACE_COLOR = "a0a000"; this.LEVEL_DEBUG_COLOR = "64c864"; this.LEVEL_INFO_COLOR = "000000"; this.LEVEL_WARN_COLOR = "0000ff"; this.LEVEL_ERROR_COLOR = "ff8c00"; this.LEVEL_FATAL_COLOR = "ff0000"; this.logLevel = 3; this.targetDiv = null; this.setLevel = function(inLevel) { this.logLevel = inLevel; } // End setLevel(). this.setTargetDiv = function(inTargetDiv) { this.targetDiv = inTargetDiv; this.targetDiv.innerHTML = ""; } // End setTargetDiv(). this.shouldBeLogged = function(inLevel) { if (inLevel >= this.logLevel) { return true; } else { return false; } } // End shouldBeLogged(). this.trace = function(inMessage) { if (this.shouldBeLogged(this.LEVEL_TRACE) && this.targetDiv) { this.targetDiv.innerHTML += "<div style='color:#" + this.LEVEL_TRACE_COLOR + ";'>" + "[TRACE] " + inMessage + ""; } } // End trace(). this.debug = function(inMessage) { if (this.shouldBeLogged(this.LEVEL_DEBUG) && this.targetDiv) { this.targetDiv.innerHTML += "<div style='color:#" + this.LEVEL_DEBUG_COLOR + ";'>" + "[DEBUG] " + inMessage + ""; } } // End debug(). this.info = function(inMessage) { if (this.shouldBeLogged(this.LEVEL_INFO) && this.targetDiv) { this.targetDiv.innerHTML += "<div style='color:#" + this.LEVEL_INFO_COLOR + ";'>" + "[INFO] " + inMessage + ""; } } // End info(). this.warn = function(inMessage) { if (this.shouldBeLogged(this.LEVEL_WARN) && this.targetDiv) { this.targetDiv.innerHTML += "<div style='color:#" + this.LEVEL_WARN_COLOR + ";'>" + "[WARN] " + inMessage + ""; } } // End warn(). this.error = function(inMessage) { if (this.shouldBeLogged(this.LEVEL_ERROR) && this.targetDiv) { this.targetDiv.innerHTML += "<div style='color:#" + this.LEVEL_ERROR_COLOR + ";'>" + "[ERROR] " + inMessage + ""; } } // End error(). this.fatal = function(inMessage) { if (this.shouldBeLogged(this.LEVEL_FATAL) && this.targetDiv) { this.targetDiv.innerHTML += "<div style='color:#" + this.LEVEL_FATAL_COLOR + ";'>" + "[FATAL] " + inMessage + ""; } } // End fatal(). } // End DivLogger(). // ]]></script>
[Ctrl+A 全选 注:如需引入外部Js需刷新才能执行]
在点击“enumProps()-Shows all ……”(第一个 link )的时候浏览器弹出的框如下图所示(Opera),详细地列出了你所点击的 a 标签对象的所有属性及值:

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen
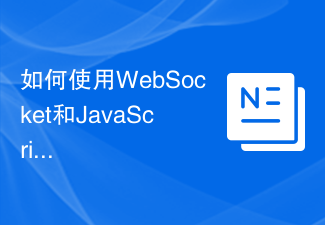
So implementieren Sie mit WebSocket und JavaScript ein Online-Spracherkennungssystem. Einführung: Mit der kontinuierlichen Weiterentwicklung der Technologie ist die Spracherkennungstechnologie zu einem wichtigen Bestandteil des Bereichs der künstlichen Intelligenz geworden. Das auf WebSocket und JavaScript basierende Online-Spracherkennungssystem zeichnet sich durch geringe Latenz, Echtzeit und plattformübergreifende Eigenschaften aus und hat sich zu einer weit verbreiteten Lösung entwickelt. In diesem Artikel wird erläutert, wie Sie mit WebSocket und JavaScript ein Online-Spracherkennungssystem implementieren.
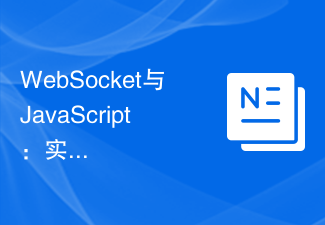
WebSocket und JavaScript: Schlüsseltechnologien zur Realisierung von Echtzeit-Überwachungssystemen Einführung: Mit der rasanten Entwicklung der Internet-Technologie wurden Echtzeit-Überwachungssysteme in verschiedenen Bereichen weit verbreitet eingesetzt. Eine der Schlüsseltechnologien zur Erzielung einer Echtzeitüberwachung ist die Kombination von WebSocket und JavaScript. In diesem Artikel wird die Anwendung von WebSocket und JavaScript in Echtzeitüberwachungssystemen vorgestellt, Codebeispiele gegeben und deren Implementierungsprinzipien ausführlich erläutert. 1. WebSocket-Technologie
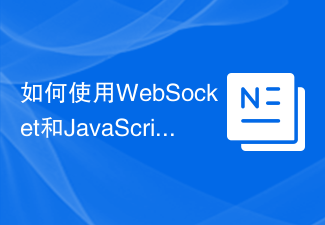
So implementieren Sie ein Online-Reservierungssystem mit WebSocket und JavaScript. Im heutigen digitalen Zeitalter müssen immer mehr Unternehmen und Dienste Online-Reservierungsfunktionen bereitstellen. Es ist von entscheidender Bedeutung, ein effizientes Online-Reservierungssystem in Echtzeit zu implementieren. In diesem Artikel wird erläutert, wie Sie mit WebSocket und JavaScript ein Online-Reservierungssystem implementieren, und es werden spezifische Codebeispiele bereitgestellt. 1. Was ist WebSocket? WebSocket ist eine Vollduplex-Methode für eine einzelne TCP-Verbindung.
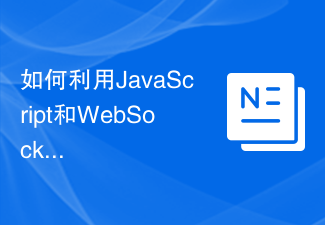
Einführung in die Verwendung von JavaScript und WebSocket zur Implementierung eines Online-Bestellsystems in Echtzeit: Mit der Popularität des Internets und dem Fortschritt der Technologie haben immer mehr Restaurants damit begonnen, Online-Bestelldienste anzubieten. Um ein Echtzeit-Online-Bestellsystem zu implementieren, können wir JavaScript und WebSocket-Technologie verwenden. WebSocket ist ein Vollduplex-Kommunikationsprotokoll, das auf dem TCP-Protokoll basiert und eine bidirektionale Kommunikation zwischen Client und Server in Echtzeit realisieren kann. Im Echtzeit-Online-Bestellsystem, wenn der Benutzer Gerichte auswählt und eine Bestellung aufgibt
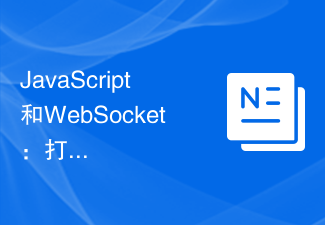
JavaScript und WebSocket: Aufbau eines effizienten Echtzeit-Wettervorhersagesystems Einführung: Heutzutage ist die Genauigkeit von Wettervorhersagen für das tägliche Leben und die Entscheidungsfindung von großer Bedeutung. Mit der Weiterentwicklung der Technologie können wir genauere und zuverlässigere Wettervorhersagen liefern, indem wir Wetterdaten in Echtzeit erhalten. In diesem Artikel erfahren Sie, wie Sie mit JavaScript und WebSocket-Technologie ein effizientes Echtzeit-Wettervorhersagesystem aufbauen. In diesem Artikel wird der Implementierungsprozess anhand spezifischer Codebeispiele demonstriert. Wir
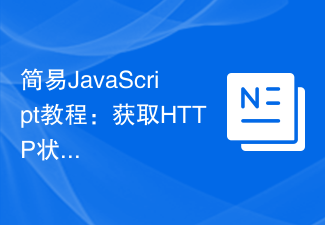
JavaScript-Tutorial: So erhalten Sie HTTP-Statuscode. Es sind spezifische Codebeispiele erforderlich. Vorwort: Bei der Webentwicklung ist häufig die Dateninteraktion mit dem Server erforderlich. Bei der Kommunikation mit dem Server müssen wir häufig den zurückgegebenen HTTP-Statuscode abrufen, um festzustellen, ob der Vorgang erfolgreich ist, und die entsprechende Verarbeitung basierend auf verschiedenen Statuscodes durchführen. In diesem Artikel erfahren Sie, wie Sie mit JavaScript HTTP-Statuscodes abrufen und einige praktische Codebeispiele bereitstellen. Verwenden von XMLHttpRequest

Verwendung: In JavaScript wird die Methode insertBefore() verwendet, um einen neuen Knoten in den DOM-Baum einzufügen. Diese Methode erfordert zwei Parameter: den neuen Knoten, der eingefügt werden soll, und den Referenzknoten (d. h. den Knoten, an dem der neue Knoten eingefügt wird).
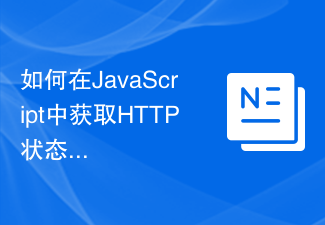
Einführung in die Methode zum Abrufen des HTTP-Statuscodes in JavaScript: Bei der Front-End-Entwicklung müssen wir uns häufig mit der Interaktion mit der Back-End-Schnittstelle befassen, und der HTTP-Statuscode ist ein sehr wichtiger Teil davon. Das Verstehen und Abrufen von HTTP-Statuscodes hilft uns, die von der Schnittstelle zurückgegebenen Daten besser zu verarbeiten. In diesem Artikel wird erläutert, wie Sie mithilfe von JavaScript HTTP-Statuscodes erhalten, und es werden spezifische Codebeispiele bereitgestellt. 1. Was ist ein HTTP-Statuscode? HTTP-Statuscode bedeutet, dass der Dienst den Dienst anfordert, wenn er eine Anfrage an den Server initiiert
