PHP的PDO数据库操作类
Jul 25, 2016 am 08:42 AM
一个简单的PDO类封装。。仅供学习交流 PdoDb 数据库类
使用的时候
|
PHP, PDO
Erklärung dieser Website
Der Inhalt dieses Artikels wird freiwillig von Internetnutzern beigesteuert und das Urheberrecht liegt beim ursprünglichen Autor. Diese Website übernimmt keine entsprechende rechtliche Verantwortung. Wenn Sie Inhalte finden, bei denen der Verdacht eines Plagiats oder einer Rechtsverletzung besteht, wenden Sie sich bitte an admin@php.cn

Heißer Artikel
Wie lange dauert es, um Split Fiction zu schlagen?
3 Wochen vor
By DDD
Repo: Wie man Teamkollegen wiederbelebt
3 Wochen vor
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Abenteuer: Wie man riesige Samen bekommt
3 Wochen vor
By 尊渡假赌尊渡假赌尊渡假赌
Zwei -Punkte -Museum: Alle Exponate und wo man sie finden kann
3 Wochen vor
By 尊渡假赌尊渡假赌尊渡假赌

Hot-Tools-Tags

Heißer Artikel
Wie lange dauert es, um Split Fiction zu schlagen?
3 Wochen vor
By DDD
Repo: Wie man Teamkollegen wiederbelebt
3 Wochen vor
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island Abenteuer: Wie man riesige Samen bekommt
3 Wochen vor
By 尊渡假赌尊渡假赌尊渡假赌
Zwei -Punkte -Museum: Alle Exponate und wo man sie finden kann
3 Wochen vor
By 尊渡假赌尊渡假赌尊渡假赌

Heiße Artikel -Tags

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen
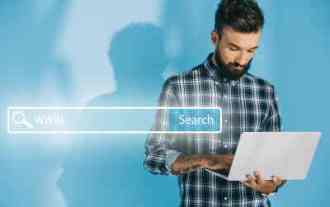
11 beste PHP -URL -Shortener -Skripte (kostenlos und Premium)
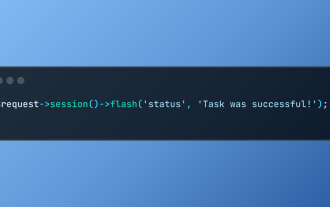
Arbeiten mit Flash -Sitzungsdaten in Laravel
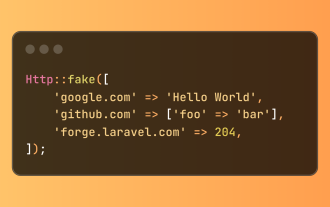
Vereinfachte HTTP -Reaktion verspottet in Laravel -Tests
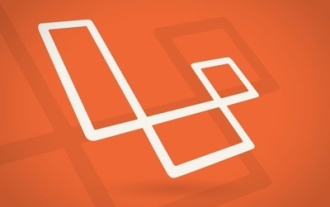
Erstellen Sie eine React -App mit einem Laravel -Back -Ende: Teil 2, reagieren
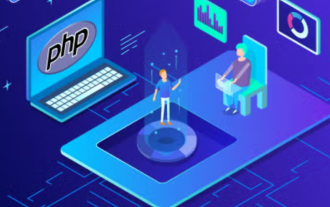
Curl in PHP: So verwenden Sie die PHP -Curl -Erweiterung in REST -APIs
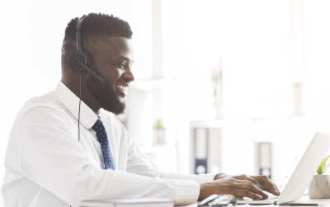
12 Beste PHP -Chat -Skripte auf Codecanyon
