MySQL的备份
本来想着用SHOW CREATE TABLE + ergodic 来做备份的但是发现如果Table 有 comment 而且还是乱码的话,会导致SHOW CREATE TABLE 出来的脚本缺少一个符号。所以有了这个版本。
|

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


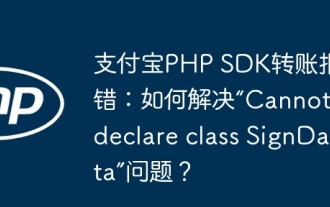
Alipay PHP ...
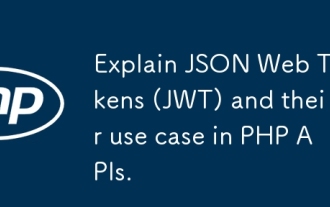
JWT ist ein offener Standard, der auf JSON basiert und zur sicheren Übertragung von Informationen zwischen Parteien verwendet wird, hauptsächlich für die Identitätsauthentifizierung und den Informationsaustausch. 1. JWT besteht aus drei Teilen: Header, Nutzlast und Signatur. 2. Das Arbeitsprinzip von JWT enthält drei Schritte: Generierung von JWT, Überprüfung von JWT und Parsingnayload. 3. Bei Verwendung von JWT zur Authentifizierung in PHP kann JWT generiert und überprüft werden, und die Funktionen und Berechtigungsinformationen der Benutzer können in die erweiterte Verwendung aufgenommen werden. 4. Häufige Fehler sind Signaturüberprüfungsfehler, Token -Ablauf und übergroße Nutzlast. Zu Debugging -Fähigkeiten gehört die Verwendung von Debugging -Tools und Protokollierung. 5. Leistungsoptimierung und Best Practices umfassen die Verwendung geeigneter Signaturalgorithmen, das Einstellen von Gültigkeitsperioden angemessen.
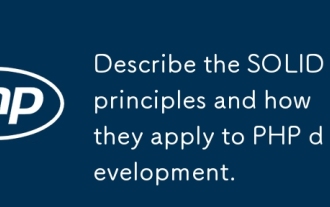
Die Anwendung des soliden Prinzips in der PHP -Entwicklung umfasst: 1. Prinzip der Einzelverantwortung (SRP): Jede Klasse ist nur für eine Funktion verantwortlich. 2. Open and Close Principle (OCP): Änderungen werden eher durch Erweiterung als durch Modifikation erreicht. 3.. Lischs Substitutionsprinzip (LSP): Unterklassen können Basisklassen ersetzen, ohne die Programmgenauigkeit zu beeinträchtigen. 4. Schnittstellen-Isolationsprinzip (ISP): Verwenden Sie feinkörnige Schnittstellen, um Abhängigkeiten und nicht verwendete Methoden zu vermeiden. 5. Abhängigkeitsinversionsprinzip (DIP): Hoch- und niedrige Module beruhen auf der Abstraktion und werden durch Abhängigkeitsinjektion implementiert.
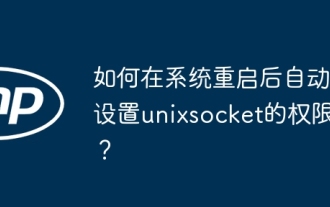
So setzen Sie die Berechtigungen von Unixsocket automatisch nach dem Neustart des Systems. Jedes Mal, wenn das System neu startet, müssen wir den folgenden Befehl ausführen, um die Berechtigungen von Unixsocket: sudo ...
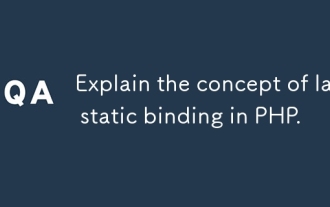
In Artikel wird die in PHP 5.3 eingeführte LSB -Bindung (LSB) erörtert, die die Laufzeitauflösung der statischen Methode ermöglicht, um eine flexiblere Vererbung zu erfordern. Die praktischen Anwendungen und potenziellen Perfo von LSB
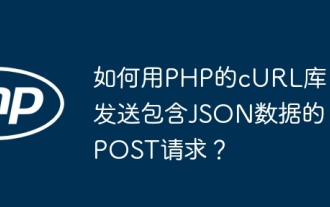
Senden von JSON -Daten mithilfe der Curl -Bibliothek von PHP in der PHP -Entwicklung müssen häufig mit externen APIs interagieren. Eine der gängigen Möglichkeiten besteht darin, die Curl Library zu verwenden, um Post � ...
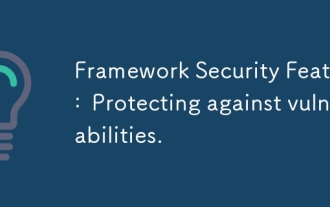
In Artikel werden wichtige Sicherheitsfunktionen in Frameworks erörtert, um vor Schwachstellen zu schützen, einschließlich Eingabevalidierung, Authentifizierung und regelmäßigen Aktualisierungen.
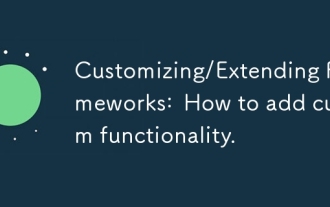
In dem Artikel werden Frameworks hinzugefügt, das sich auf das Verständnis der Architektur, das Identifizieren von Erweiterungspunkten und Best Practices für die Integration und Debuggierung hinzufügen.
