Mongodb Mapreduce-Nutzung und PHP-Beispielcode
Parameter Beschreibung: mapreduce: der zu bedienende Zielsatz map: Mapping-Funktion (erzeugt eine Folge von Schlüssel-Wert-Paaren als Parameter der Reduce-Funktion) Reduzieren: StatistikfunktionAbfrage: ZieldatensatzfilterungSortieren: Zieldatensätze sortierenLimit: Anzahl der Zieldatensätze begrenzenaus: Speicherung statistischer Ergebnisse (falls nicht angegeben) Verwenden Sie temporäre Sammlungen, die automatisch gelöscht werden, nachdem der Client die Verbindung getrennt hat) keeptemp: ob die temporäre Sammlung beibehalten werden soll finalize: Endverarbeitungsfunktion (führt aus). endgültige Sortierung nach den Ergebnissen der Reduzierungsrückgabe und Speicherung im Ergebnissatz) Umfang: Externe Variablen zum Zuordnen, Reduzieren und Finalisieren importieren Ausführlich: Detaillierte Zeitstatistiken anzeigen
Die Map-Funktion ruft das aktuelle Objekt auf, verarbeitet die Attribute des Objekts und übergibt den zu reduzierenden Wert. Die Map-Methode verwendet dies zum Betrieb das aktuelle Objekt und ruft die Methode „emit(key, value)“ mindestens einmal auf, um den zu reduzierenden Wert zu übergeben. Geben Sie Parameter an, wobei der Schlüssel von „emit“ die ID der endgültigen Daten ist.
接收一个值和数组,根据需要对数组进行合并分组等处理,reduce的key就是emit(key,value)的key,value_array是同个key对应的多个value数组。
此函数为可选函数,可在执行完map和reduce后执行,对最后的数据进行统一处理。
我们按动态类型feed_type和用户to_user进行分组统计,实现结果:
feed_type | to_user | cout |
1 | 234 | 1 |
8 | 123 | 1 |
1 | 123 | 2 |
下面是输出的结果,和预期结果一致
以上就介绍了mongodb的mapreduce用法及php示例代码,包括了方面的内容,希望对PHP教程有兴趣的朋友有所帮助。

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen
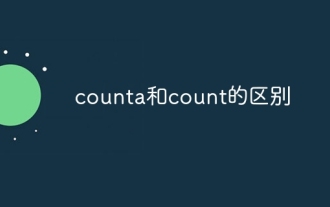
Mit der Count-Funktion wird die Anzahl der Zahlen in einem angegebenen Bereich gezählt. Sie ignoriert Text, logische Werte und Nullwerte, zählt jedoch leere Zellen. Die Count-Funktion zählt nur die Anzahl der Zellen, die tatsächliche Zahlen enthalten. Die Funktion CountA wird verwendet, um die Anzahl der nicht leeren Zellen in einem angegebenen Bereich zu zählen. Es zählt nicht nur Zellen, die tatsächliche Zahlen enthalten, sondern auch die Anzahl nicht leerer Zellen, die Text, logische Werte und Formeln enthalten.
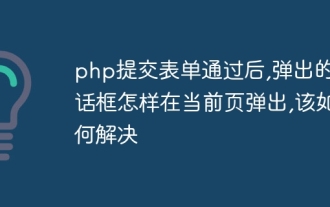
php提交表单通过后,弹出的对话框怎样在当前页弹出php提交表单通过后,弹出的对话框怎样在当前页弹出而不是在空白页弹出?想实现这样的效果:而不是空白页弹出:------解决方案--------------------如果你的验证用PHP在后端,那么就用Ajax;仅供参考:HTML code
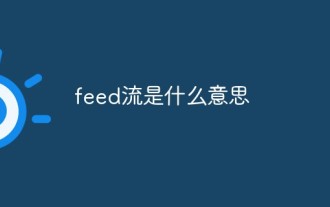
Ein Feed-Stream ist ein Informationsfluss, der den Benutzern kontinuierlich Inhalte aktualisiert und präsentiert. Ein Feed ist ein Inhaltsaggregator, der mehrere Nachrichtenquellen kombiniert, die Benutzer aktiv abonnieren, um Benutzern dabei zu helfen, kontinuierlich die neuesten Feed-Inhalte zu erhalten.
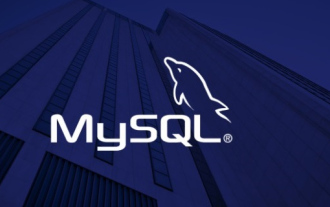
In diesem Artikel werden die Unterschiede in der Verwendung der MySQL-Funktion vorgestellt. Der Bedarf an statistischen Daten ist in unserer täglichen Entwicklung sehr einfach. Schauen wir uns als Nächstes die Unterschiede zwischen ihnen an. und ob sie einige Fallstricke haben.
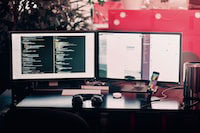
In diesem Artikel wird die Summe aller Werte im von PHP zurückgegebenen Array ausführlich erläutert. Der Herausgeber hält dies für recht praktisch, daher teile ich es Ihnen als Referenz mit und hoffe, dass Sie nach dem Lesen dieses Artikels etwas gewinnen können. PHP gibt die Summe aller Werte in einem Array zurück. In PHP gibt es mehrere Möglichkeiten, die Summe aller Werte in einem Array zu berechnen. Hier sind einige der gebräuchlichsten Techniken: 1. Funktion array_sum() Mit der Funktion array_sum() kann die Summe aller Werte in einem Array berechnet werden. Es akzeptiert ein Array als Argument und gibt ein ganzzahliges Ergebnis zurück.
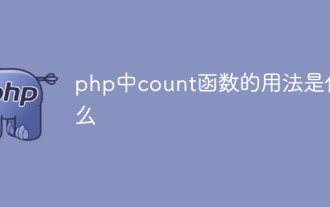
Die Verwendung der Zählfunktion in PHP lautet: [count(array,mode);], wobei der Parameter array das zu zählende Array angibt und der Parameter mode den Modus der Funktion angibt.
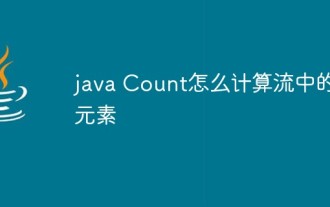
Hinweis 1. Count ist eine Terminaloperation, die die Gesamtzahl der Elemente im Stream zählen kann. Der Rückgabewert ist vom Typ long. 2. count() gibt die Anzahl der Elemente im Stream zurück. Dies ist ein Sonderfall der Induktion (eine Induktionsoperation nimmt eine Folge von Eingabeelementen und kombiniert sie durch wiederholte Anwendung der Kombinationsoperation zu einem zusammenfassenden Ergebnis). Dies ist ein Endvorgang und kann Konsequenzen und Nebenwirkungen haben. Nachdem ein Endvorgang durchgeführt wurde, gilt die Leitung als verbraucht und kann nicht wiederverwendet werden. Beispiel // Überprüfen Sie, ob eine Zeichenfolge in der Liste vorhanden ist, die mit a beginnt, und passen Sie sie an die erste an, d. h. geben Sie truebooleananyStartsWithA=stringCollection.stream().anyMatch((s) zurück

Die reproduzierte Testdatenbank lautet wie folgt: CREATETABLE`test_distinct`(`id`int(11)NOTNULLAUTO_INCREMENT,`a`varchar(50)CHARACTERSETutf8DEFAULTNULL,`b`varchar(50)CHARACTERSETutf8DEFAULTNULL,PRIMARYKEY(`id`))ENGINE= InnoDBAUTO_INCREMENT =1DEFAULTCHARSET=latin1;Die Testdaten in der Tabelle lauten wie folgt. Jetzt müssen wir die deduplizierten Spalten dieser drei Spalten zählen.
