


Der in Python geschriebene Client sendet Anweisungen an den Server, um die entsprechenden Befehle auszuführen und die Ergebnisse zurückzugeben.
Ich habe nichts zu tun, also verwende ich Python, um ein Programm für die Client- und Serverantwort zu schreiben. Das Hauptprinzip besteht darin, dass der Client über das TCP-Protokoll mit dem Server kommuniziert führt die Anweisungen aus und sendet die entsprechenden Ergebnisse an den Client zurück, und der Client druckt das Ergebnis aus. Der Code ist relativ einfach und wird nicht im Detail vorgestellt. Nur zur Unterhaltung.
Serverseitiger Code, server_tcp.py
#!/usr/bin/env python # -*- coding:utf-8 -*- # #执行客户端发送过来的命令,并把执行结果返回给客户端 import socket, traceback, subprocess host = '' port = 51888 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) s.bind((host, port)) s.listen(1) while 1: try: client_socket, client_addr = s.accept() except Exception, e: traceback.print_exc() continue try: print 'From host:', client_socket.getpeername() while 1: command = client_socket.recv(4096) if not len(command): break print client_socket.getpeername()[0] + ':' + str(command) # 执行客户端传递过来的命令 handler = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE) output = handler.stdout.readlines() if output is None: output = [] for one_line in output: client_socket.sendall(one_line) client_socket.sendall("\n") client_socket.sendall("ok") except Exception, e: traceback.print_exc() try: client_socket.close() except Exception, e: traceback.print_exc()
2. Clientseitiger Code client_tcp.py
#!/usr/bin/env python # -*- coding:utf-8 -*- # #给server端发送命令 import socket, sys, traceback host = '127.0.0.1' port = 51888 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) try: s.connect((host, port)) except Exception, e: msg = traceback.format_exc() print '连接错误:', msg input_command = raw_input('Input command:') s.send(input_command) # 利用shutdown()函数使socket双向数据传输变为单向数据传输 # 该参数表示了如何关闭socket。具体为:0表示禁止将来读;1表示禁止将来写;2表示禁止将来读和写 s.shutdown(1) print '发送完成.' print '收到内容:\n' while 1: buff = s.recv(4096) if not len(buff): break sys.stdout.write(buff)
3. Starten Sie das Skript server_tcp.py und beginnen Sie mit dem Abhören des lokalen Ports 51888; starten Sie dann client_tcp.py.
(1) Client-Inhalt:
/usr/bin/python2.7 /home/wuguowei/PycharmProjects/xplan_script/test_process/client_tcp.py Input command:ls -l 发送完成. 收到内容: 总用量 20 -rw-r--r-- 1 root root 744 2月 10 14:44 client_tcp.py -rw-r--r-- 1 root root 877 2月 10 14:18 my_sub_process.py -rw-r--r-- 1 root root 1290 2月 10 14:45 server_tcp.py -rw-r--r-- 1 root root 493 2月 10 10:43 tcpclient.py -rw-r--r-- 1 root root 1168 2月 10 11:51 tcpserver.py ok Process finished with exit code 0
(2) Serverinformationen
/usr/bin/python2.7 /home/wuguowei/PycharmProjects/xplan_script/test_process/server_tcp.py From host: ('127.0.0.1', 46993) 127.0.0.1:ls -l

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


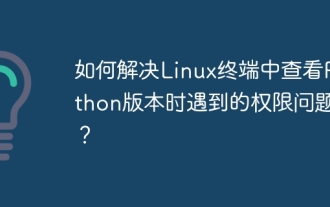
Lösung für Erlaubnisprobleme beim Betrachten der Python -Version in Linux Terminal Wenn Sie versuchen, die Python -Version in Linux Terminal anzuzeigen, geben Sie Python ein ...
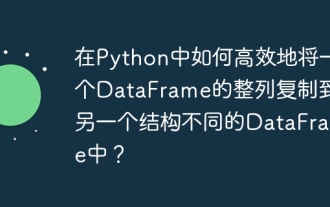
Bei der Verwendung von Pythons Pandas -Bibliothek ist das Kopieren von ganzen Spalten zwischen zwei Datenrahmen mit unterschiedlichen Strukturen ein häufiges Problem. Angenommen, wir haben zwei Daten ...
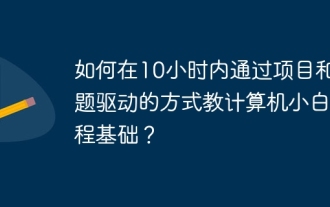
Wie lehre ich innerhalb von 10 Stunden die Grundlagen für Computer -Anfänger für Programmierungen? Wenn Sie nur 10 Stunden Zeit haben, um Computer -Anfänger zu unterrichten, was Sie mit Programmierkenntnissen unterrichten möchten, was würden Sie dann beibringen ...
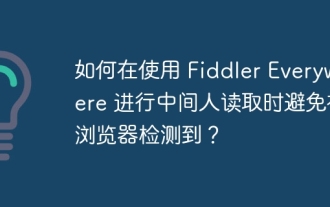
Wie kann man nicht erkannt werden, wenn Sie Fiddlereverywhere für Man-in-the-Middle-Lesungen verwenden, wenn Sie FiddLereverywhere verwenden ...
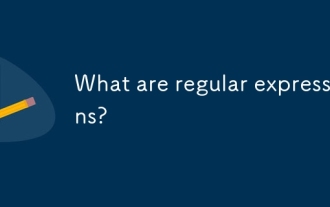
Regelmäßige Ausdrücke sind leistungsstarke Tools für Musteranpassung und Textmanipulation in der Programmierung, wodurch die Effizienz bei der Textverarbeitung in verschiedenen Anwendungen verbessert wird.
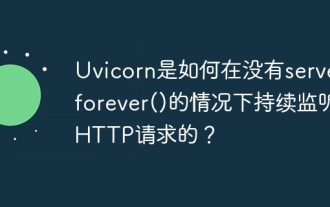
Wie hört Uvicorn kontinuierlich auf HTTP -Anfragen an? Uvicorn ist ein leichter Webserver, der auf ASGI basiert. Eine seiner Kernfunktionen ist es, auf HTTP -Anfragen zu hören und weiterzumachen ...
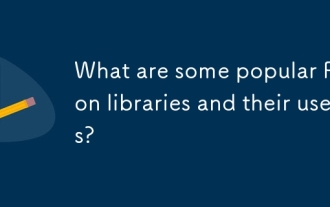
In dem Artikel werden beliebte Python-Bibliotheken wie Numpy, Pandas, Matplotlib, Scikit-Learn, TensorFlow, Django, Flask und Anfragen erörtert, die ihre Verwendung in wissenschaftlichen Computing, Datenanalyse, Visualisierung, maschinellem Lernen, Webentwicklung und h beschreiben
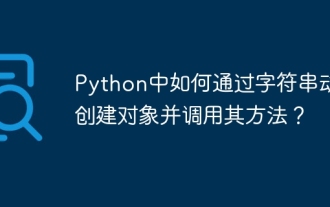
Wie erstellt in Python ein Objekt dynamisch über eine Zeichenfolge und ruft seine Methoden auf? Dies ist eine häufige Programmieranforderung, insbesondere wenn sie konfiguriert oder ausgeführt werden muss ...
