Erklärung des einfachen .NET-Factory-Musters
Einführung in das einfache Fabrikmuster:
Das einfache Fabrikmuster ist ein Erstellungsmuster, das auch als statische Fabrikmethode (Static Factory Method) bezeichnet wird, aber nicht zu den 23 GOF-Entwurfsmustern gehört. Das einfache Factory-Muster verwendet ein Factory-Objekt, um zu entscheiden, welche Produktklasseninstanz erstellt werden soll. Das einfache Factory-Pattern ist das einfachste und praktischste Pattern in der Factory-Pattern-Familie und kann als spezielle Implementierung verschiedener Factory-Patterns verstanden werden.
Strukturmusterdiagramm:
Rollenklassifizierung:
Fabrikrolle (Ersteller)
Der Kern des Einfachen Factory-Muster, das für die Implementierung der internen Logik zum Erstellen aller Instanzen verantwortlich ist. Die Methode zum Erstellen einer Produktklasse in der Factory-Klasse kann direkt von der Außenwelt aufgerufen werden, um das erforderliche Produktobjekt zu erstellen.
Abstrakte Produktrolle
Die übergeordnete Klasse aller durch das einfache Factory-Muster erstellten Objekte, die für die Beschreibung der allen Instanzen gemeinsamen öffentlichen Schnittstelle verantwortlich ist.
Die Rolle „Betonprodukt“
ist das Erstellungsziel des einfachen Factory-Musters. Alle erstellten Objekte sind Instanzen einer bestimmten Klasse, die diese Rolle spielt.
Einführung in die aktuelle Situation:
Wenn es ein Mieterverwaltungssystem gibt, sind die Mietertypen darin variabel und die Mietberechnungsformel für jeden Mietertyp ist unterschiedlich
Der Mietbetrag für Bewohner des Typs A = Anzahl der Tage * Einheitspreis + Leistung * 0,005
Der Mietbetrag für Bewohner des Typs B = Monat * (Monatspreis + Leistung * 0,001)
Analyse:
1. Geschäfte haben eine gemeinsame Berechnungsmethode. Sie verhalten sich jedoch unterschiedlich. Daher abstrahieren wir die Ladenklasse und der Code ist wie folgt >
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SimpleFactory.App.IFactroy { public interface Ishop { double Getrent(int days, double dayprice, double performance); } }
using SimpleFactory.App.IFactroy; using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SimpleFactory.App.product { //A类型的商店的创建 public class Ashop:Ishop { /// <summary> /// /// A类型商店租金额,天数*单价+绩效*0.005 /// </summary> /// <param name="days">天数</param> /// <param name="dayprice">每天单价</param> /// <param name="performance">日平均绩效</param> /// <returns></returns> public double Getrent(int days, double dayprice, double performance) { Console.WriteLine("A商店的租金算法"); return days * dayprice + performance * 0.01; } } }
using SimpleFactory.App.IFactroy; using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SimpleFactory.App.product { /// <summary> /// B类型的商店的创建 /// </summary> public class Bshop:Ishop { /// <summary> /// B类型商店的租金=月份*(每月价格+performance*0.001) /// </summary> /// <param name="month">月数</param> /// <param name="monthprice">月单价</param> /// <param name="performance">月平均绩效</param> /// <returns></returns> public double Getrent(int month, double monthprice, double performance) { Console.WriteLine("B商店的租金算法"); return month * (monthprice + performance * 0.001); } } }
using SimpleFactory.App.IFactroy; using SimpleFactory.App.product; using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SimpleFactory.App.factoryMethod { public class factorymethod { public Ishop CreateShow(string show) { switch (show.Trim().ToLower()) { case"ashop": return new Ashop(); case "bshop": return new Ashop(); default: throw new Exception("该商店不存在"); } } } }
using SimpleFactory.App.factoryMethod; using SimpleFactory.App.IFactroy; using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace SimpleFactory.App { class Program { static void Main(string[] args) { Ishop As; factorymethod afm = new factorymethod(); As = afm.CreateShow("ashop"); //a 类型的某商店 double total = As.Getrent(30, 300, 2000); //30 天/100元 日平均绩效为2000 Console.WriteLine("该A类型商店的租金为:" + total); Console.WriteLine("============="); Ishop Bs; factorymethod bfm = new factorymethod(); Bs = bfm.CreateShow("bshop"); //b 类型的某商店 total = Bs.Getrent(3, 3000, 60000); //3 月/4000元 月平均绩效为60000 Console.WriteLine("该B类型商店的租金为:" + total); Console.ReadKey(); } } }

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


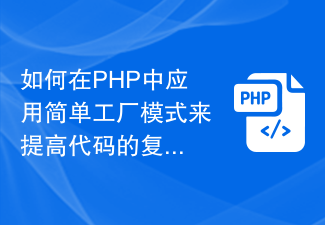
So wenden Sie das einfache Factory-Muster in PHP an, um die Wiederverwendbarkeit von Code zu verbessern. Das einfache Factory-Muster (SimpleFactoryPattern) ist ein häufig verwendetes Entwurfsmuster, das beim Erstellen von Objekten eine einheitliche Schnittstelle bieten kann, um verschiedene Objekte entsprechend unterschiedlichen Bedingungen zu erstellen. Dieser Modus kann die Kopplung von Code effektiv reduzieren und die Wartbarkeit und Wiederverwendbarkeit von Code verbessern. In PHP können wir das einfache Factory-Muster verwenden, um die Struktur und Logik des Codes zu optimieren. Das einfache Factory-Muster verstehen Das einfache Factory-Muster besteht aus drei Elementen
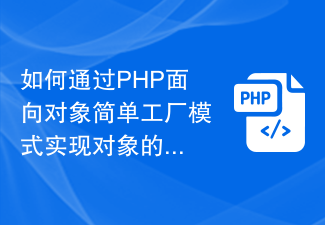
So implementieren Sie die Objektversionskontrolle und -verwaltung über das objektorientierte einfache PHP-Factory-Modell. Bei der Entwicklung großer und komplexer PHP-Projekte sind Versionskontrolle und -verwaltung sehr wichtig. Durch geeignete Entwurfsmuster können wir die Erstellung und Verwendung von Objekten besser verwalten und steuern und so die Wartbarkeit und Skalierbarkeit des Codes verbessern. In diesem Artikel wird erläutert, wie Sie das objektorientierte einfache PHP-Factory-Muster verwenden, um die Versionskontrolle und -verwaltung von Objekten zu implementieren. Das einfache Factory-Muster ist ein Entwurfsmuster zum Erstellen von Klassen, das bestimmte Objekte über eine Factory-Klasse instanziiert
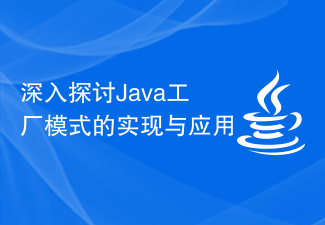
Ausführliche Erläuterung der Prinzipien und Anwendungen des Java-Factory-Musters. Das Factory-Muster ist ein häufig verwendetes Entwurfsmuster, das zum Erstellen von Objekten und zum Kapseln des Erstellungsprozesses von Objekten verwendet wird. Es gibt viele Möglichkeiten, das Factory-Muster in Java zu implementieren. Die gebräuchlichsten sind das einfache Factory-Muster, das Factory-Methodenmuster und das abstrakte Factory-Muster. In diesem Artikel werden die Prinzipien und Anwendungen dieser drei Factory-Muster ausführlich vorgestellt und entsprechende Codebeispiele gegeben. 1. Einfaches Fabrikmuster Das einfache Fabrikmuster ist das einfachste und am häufigsten verwendete Fabrikmuster. Es verwendet eine Factory-Klasse, um basierend auf den übergebenen Parametern unterschiedliche Werte zurückzugeben.
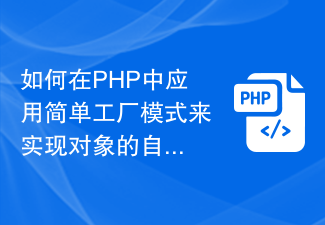
So wenden Sie das einfache Factory-Muster in PHP an, um die Objekterstellung zu automatisieren. Das einfache Factory-Muster ist ein gängiges Entwurfsmuster, das zum Erstellen von Objekten verwendet wird und den Prozess der Instanziierung von Objekten abstrahiert. In PHP kann die Anwendung des einfachen Factory-Musters dabei helfen, die Objekterstellung und die spezifische Implementierung zu entkoppeln und so den Code flexibler und wartbarer zu machen. In diesem Artikel veranschaulichen wir anhand eines Beispiels, wie das Simple Factory Pattern in PHP angewendet wird. Angenommen, wir haben ein Elektronikgeschäft, das Mobiltelefone und Fernseher verkauft. Wir müssen Fotos basierend auf der Benutzerauswahl erstellen
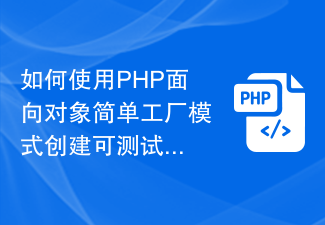
So verwenden Sie das objektorientierte einfache Factory-Muster von PHP, um testbare Objektinstanzen zu erstellen. Das einfache Factory-Muster ist ein häufig verwendetes Software-Designmuster, das uns hilft, verschiedene Objektinstanzen basierend auf unterschiedlichen Bedingungen zu erstellen. Bei der objektorientierten PHP-Programmierung kann die Kombination des einfachen Factory-Musters die Testbarkeit und Wartbarkeit des Codes verbessern. In diesem Artikel erfahren Sie, wie Sie testbare Objektinstanzen mithilfe des objektorientierten einfachen Factory-Musters in PHP erstellen. Wir werden diesen Prozess anhand eines einfachen Beispiels veranschaulichen. Definieren wir zunächst eine Schnittstelle zur Darstellung des
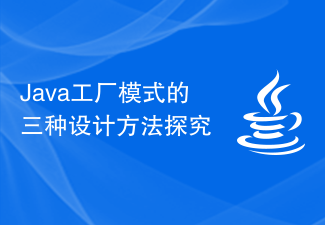
Entdecken Sie drei Designideen des Java Factory-Musters. Das Factory-Muster ist ein häufig verwendetes Entwurfsmuster zum Erstellen von Objekten ohne Angabe einer bestimmten Klasse. In Java kann das Factory-Muster auf viele Arten implementiert werden. In diesem Artikel wird die Implementierung von drei Java-Factory-Mustern basierend auf unterschiedlichen Designideen untersucht und spezifische Codebeispiele gegeben. Einfaches Factory-Muster Das einfache Factory-Muster ist das grundlegendste Factory-Muster, das Objekte über eine Factory-Klasse erstellt. Die Factory-Klasse bestimmt, welche Art von spezifischem Objekt basierend auf den Anforderungsparametern des Clients erstellt werden soll. Unten finden Sie eine Zusammenfassung
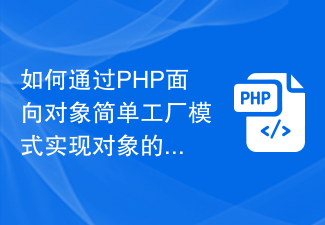
So erreichen Sie Objektpolymorphismus durch das objektorientierte einfache Fabrikmuster von PHP. Das einfache Fabrikmuster ist ein allgemeines Entwurfsmuster, das Objekte unterschiedlichen Typs über eine gemeinsame Fabrikklasse erstellen und den Objekterstellungsprozess verbergen kann. Das objektorientierte einfache PHP-Fabrikmuster kann uns dabei helfen, Objektpolymorphismus zu erreichen. Das einfache Fabrikmuster enthält drei Grundrollen: Fabrikklasse, abstrakte Klasse und konkrete Klasse. Zuerst definieren wir eine abstrakte Klasse Animal, die eine abstrakte Methode say(): abstractclas enthält
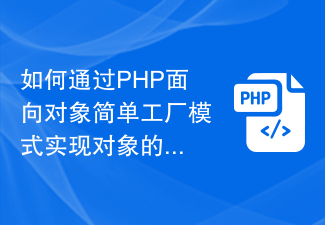
So realisieren Sie eine einheitliche Eingabe und Ausgabe von Objekten durch ein objektorientiertes einfaches PHP-Fabrikmuster. Einführung: In der PHP-Entwicklung kann die Wartbarkeit und Skalierbarkeit des Codes durch den Einsatz objektorientierter Programmierung (Object-Oriented Programming, OOP) verbessert werden. Objektorientierte Entwurfsmuster sind eine weit verbreitete Methode, die uns helfen kann, Code besser zu organisieren und zu verwalten. In diesem Artikel konzentrieren wir uns darauf, wie man mithilfe des objektorientierten einfachen PHP-Factory-Musters einen einheitlichen Zugriff auf Objekte erreicht.
