


Detaillierte Beispiele, wie jQuery Akkordeonmenüs, hierarchische Menüs, Hauptmenüs und nahtlose Bildlaufeffekte implementiert
In diesem Artikel wird hauptsächlich die grafische Beschreibung des Akkordeonmenüs, des hierarchischen Menüs, des oberen Menüs und des nahtlosen Bildlaufmenüs vorgestellt. Die Effektanzeige und die Codeimplementierung dieses Artikels werden ausführlich vorgestellt Ich hoffe, es kann allen helfen.
1. Die Darstellungen und Codes des Akkordeonmenüs sind wie folgt:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>手风琴效果制作</title> <link rel="stylesheet" href="../css/reset.css"> <style> .con{ width:908px; height:300px; margin:50px auto; overflow: hidden; position:relative; } .con .list_ul{ } .con .list_ul li{ width:805px; height:300px; position:absolute; background:#fff; } .con .list_ul li span{ width:26px; height:296px; text-align: center; color:#fff; padding-top:4px; float:left; cursor: pointer; } .con .list_ul li img{ width:777px; height:300px; float:right; } .con .list_ul li:after{ display: table; clear:both; zoom:1; content: ''; } .con .list_ul li:nth-child(1){ left:0; } .con .list_ul li:nth-child(2){ left:801px; } .con .list_ul li:nth-child(3){ left:828px; } .con .list_ul li:nth-child(4){ left:855px; } .con .list_ul li:nth-child(5){ left:882px; } .con .list_ul li:nth-child(1) span{ background: rgba(8, 201, 160, 0.49); } .con .list_ul li:nth-child(2) span{ background: rgba(120, 201, 66, 0.97); } .con .list_ul li:nth-child(3) span{ background: rgb(77, 114, 201); } .con .list_ul li:nth-child(4) span{ background: rgb(255, 179, 18); } .con .list_ul li:nth-child(5) span{ background: rgb(201, 5, 166); } </style> <script src="../js/jquery-1.12.4.min.js"></script> <script> $(function(){ $(".list_li").click(function(){ //左边 $(this).animate({left:26*$(this).index()}); //获取该li元素前面的兄弟元素,实现点击中间的部分,它前面的兄弟元素也跟着一起向左移动 $(this).prevAll().each(function(){ $(this).animate({left:26*$(this).index()}); }); //右边:获取该li元素后面的兄弟元素,实现点击中间的部分,它后面的兄弟元素也跟着一起向右移动 $(this).nextAll().each(function(){ $(this).animate({left:778+26*$(this).index()}); }); }) }) </script> </head> <body> <p class="con"> <ul class="list_ul"> <li class="list_li"> <span>风景图01</span> <img src="../images/li01.png" alt="风景图01"> </li> <li class="list_li"> <span>风景图02</span> <img src="../images/li02.png" alt="风景图02"> </li> <li class="list_li"> <span>风景图03</span> <img src="../images/li03.png" alt="风景图03"> </li> <li class="list_li"> <span>风景图04</span> <img src="../images/li04.png" alt="风景图04"> </li> <li class="list_li"> <span>风景图05</span> <img src="../images/li05.png" alt="风景图05"> </li> </ul> </p> </body> </html>
2. Die Darstellungen und Codes des Scroll-Down-Menüs (Ebene) sind wie folgt:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>用jQuery中的slideToggle制作菜单</title> <link rel="stylesheet" href="../css/reset.css"> <style> .menu{ width:200px; margin:10px auto; } .menu>li{ background: #8731dd; color:#fff; text-indent: 16px; margin-top:-1px; cursor: pointer; } .menu>li>span{ padding:10px 0; display:block; border-bottom: 1px solid #f3f3f3; } .menuactive,.menu>li>span:hover{ background:#c7254e; } .menu > li ul{ display: none; } .menu > li ul li{ text-indent:20px; background: #9a9add; border:1px solid #f3f3f3; margin-top:-1px; padding:6px 0; } .menu >li .active{ display: block; } .menu > li ul li:hover{ background:#67C962; } .menu_li ul{ margin-bottom:1px; } </style> <script src="../js/jquery-1.12.4.min.js"></script> <script> $(function () { $(".menu_li>span").click(function(){ $(this).addClass("menuactive").parent().siblings().children("span").removeClass("menuactive"); $(this).next("ul").slideToggle(); $(this).parent().siblings().children("ul").slideUp(); }) }) </script> </head> <body> <ul class="menu" id="menu"> <li class="menu_li"> <span class="menuactive">水果系列</span> <ul class="active"> <li>苹果</li> <li>梨子</li> <li>葡萄</li> <li>火龙果</li> </ul> </li> <li class="menu_li"> <span>海鲜系列</span> <ul> <li>鱼</li> <li>大虾</li> <li>螃蟹</li> <li>海带</li> </ul> </li> <li class="menu_li"> <span>果蔬系列</span> <ul> <li>茄子</li> <li>黄瓜</li> <li>豆角</li> <li>西红柿</li> </ul> </li> <li class="menu_li"> <span>速冻食品</span> <ul> <li>水饺</li> <li>冰淇淋</li> </ul> </li> <li class="menu_li"> <span>肉质系列</span> <ul> <li>猪肉</li> <li>羊肉</li> <li>牛肉</li> </ul> </li> </ul> </body> </html>
3. Sticky-Menü (wenn ein Menü den oberen Rand der Seite erreicht, stoppen Sie dort)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>置顶菜单</title> <link rel="stylesheet" href="../css/reset.css" rel="external nofollow" > <style> .header{ width:960px; height:200px; margin:0 auto; background: #ccc; } .header img{ width:100%; height:200px; } .ul_list{ width:960px; height:50px; margin:0 auto; text-align: center; display: flex; justify-content: space-between;/*实现子元素水平方向上平分*/ align-items: center;/*使子元素垂直方向上居中*/ background: #67C962; } .ul_list li{ width:160px; height:50px; line-height: 50px; border:1px solid #ccc; /*使边框合并*/ margin-right:-1px; } .ul_list li a{ color:#fff; } .ul_list li:hover{ background: #c7254e; } .ul_fixed{ position: fixed; top:0; } .menu_pos{ width:960px; height:50px; margin:0 auto; line-height: 50px; display: none; } .con p{ width:958px; height:300px; line-height: 300px; text-align: center; margin:-1px auto 0; border: 1px solid #ccc; } .footer{ height:300px; } .top{ width:38px; height:38px; border-radius: 35px; background: #000; color:#fff; font-size:13px; padding:8px; text-align: center; position: fixed; right:100px; bottom:20px; display: none; } .top:hover{ cursor: pointer; } </style> <script src="../js/jquery-1.12.4.min.js"></script> <script> $(function(){ var oLeft = ($(document).outerWidth(true)-$(".header").outerWidth())/2; var oTop = $(".top"); $(window).scroll(function(){ if($(window).scrollTop() >= $(".header").outerHeight()){ $(".ul_list").addClass("ul_fixed").css({left:oLeft}); $(".menu_pos").show(); }else{ $(".ul_list").removeClass("ul_fixed"); $(".menu_pos").hide(); } if($(window).scrollTop() >= 150){ oTop.fadeIn(); oTop.click(function(){ //第一种回到顶部的方式 //$(window).scrollTop(0); //第二种回到顶部的方式(常用) $("html,body").animate({scrollTop:0}); }) }else{ oTop.fadeOut(); } }); }) </script> </head> <body> <p class="header"> <img src="../images/li02.png" alt="banner图"> </p> <ul class="ul_list"> <li><a href="javascript:void(0);" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" >首页</a></li> <li><a href="javascript:void(0);" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" >新闻动态</a></li> <li><a href="javascript:void(0);" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" >产品展示</a></li> <li><a href="javascript:void(0);" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" >案例分析</a></li> <li><a href="javascript:void(0);" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" >关注我们</a></li> <li><a href="javascript:void(0);" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="external nofollow" >联系我们</a></li> </ul> <p class="menu_pos"></p> <p class="con"> <p class="con_header">网站主内容一</p> <p class="con_center">网站主内容二</p> <p class="con_footer">网站主内容三一</p> </p> <p class="footer"></p> <p class="top">回到顶部</p> </body> </html>
4. Das nahtlose Bildlaufeffektdiagramm und der Code lauten wie folgt:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>无缝滚动</title> <link rel="stylesheet" href="../css/reset.css"> <style> .con{ width:662px; margin:50px auto; } .con button{ width:100px; height:36px; line-height: 36px; text-align: center; border: none; margin-left:20px; } .box{ width:655px; height:135px; margin:20px auto; border:1px solid #ccc; padding-left:10px; padding-bottom:10px; position: relative; overflow: hidden; } .ul_list{ display: flex; position: absolute; left:0; top:0; padding: 10px; } .ul_list li{ width:120px; height:120px; border:1px solid #ccc; margin-left:-1px; margin-right:10px; /*margin-top:10px;*/ } .ul_list li img{ width:100%; height:100px; line-height: 100px; } </style> <script src="../js/jquery-1.12.4.min.js"></script> <script> $(function(){ var oUl = $(".ul_list"); var oLeft = $(".left"); var oRight = $(".right"); var left = 0; var delay = 2; oUl.html(oUl.html()+oUl.html()); function move(){ left-=delay; if(left<-667){ left = 0; } if(left>0){ left = -667; } oUl.css({left:left}); } var timer =setInterval(move,30); oLeft.click(function(){ delay =2; }); oRight.click(function(){ delay = -2; }); oUl.children().each(function(){ oUl.eq($(this).index()).mouseover(function(){ clearInterval(timer); }); oUl.eq($(this).index()).mouseout(function(){ timer= setInterval(function(){ left-=delay; if(left<-667){ left = 0; } if(left>0){ left = -667; } oUl.css({left:left}); },30); }) }) }) </script> </head> <body> <p class="con"> <button class="left">向左</button> <button class="right">向右</button> <p class="box clearfix"> <ul class="ul_list"> <li><img src="../images/furit_03.jpg" alt="第一张图片"></li> <li><img src="../images/goods_08.jpg" alt="第二张图片"></li> <li><img src="../images/furit_02.jpg" alt="第三张图片"></li> <li><img src="../images/goods_05.jpg" alt="第四张图片"></li> <li><img src="../images/furit_04.jpg" alt="第五张图片"></li> </ul> </p> </p> </body> </html>
Die im obigen Menü beteiligten Wissenspunkte sind wie folgt:
4. jQuery
1. slideDown() zeigt nach unten
2. slideUp() rollt nach oben
3. slideToggle() erweitert oder rollt ein Element der Reihe nach auf
5. jQuery-Kettenaufruf
nach dem jQuery-Objektmethode wird ausgeführt Um dieses JQuery-Objekt zurückzugeben, können alle jQuery-Objektmethoden zusammen geschrieben werden:
$("#p1").chlidren("ul").slideDown("fast").parent().siblings().chlidren("ul").slideUp("fase")
$("#p1").//Das Element mit der ID p1
.chlidren(" ul") // Das ul-Unterelement unter diesem Element
.slideDown("fast") // Die Höhe reicht von Null bis zur tatsächlichen Höhe, um das ul-Element
.parent() //Springe zum übergeordneten Element von ul, also zum Element mit der ID p1 .siblings() //Springe zu allen Geschwisterelementen auf derselben Ebene des p1-Elements .chlidren("ul") //Finden Sie die ul-Unterelemente unter diesen Geschwisterelementen .slideUp("fase") //Konvertieren Sie von der tatsächlichen Höhe in Null, um die zu verbergen ul-Element Verwandte Empfehlungen:
Jquery implementiert Akkordeoneffekt für Dropdown-Menüs
Instanzfreigabe jquery implementiert Multi- Ebenenmenüeffekt
jQuery-Menü, alle Auswahl, Auswahl umkehren, Instanzparsing abbrechen
Das obige ist der detaillierte Inhalt vonDetaillierte Beispiele, wie jQuery Akkordeonmenüs, hierarchische Menüs, Hauptmenüs und nahtlose Bildlaufeffekte implementiert. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


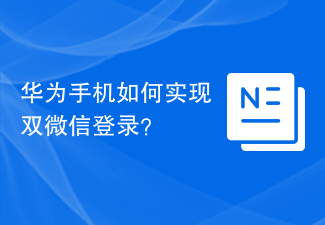
Wie implementiert man die doppelte WeChat-Anmeldung auf Huawei-Mobiltelefonen? Mit dem Aufkommen der sozialen Medien ist WeChat zu einem unverzichtbaren Kommunikationsmittel im täglichen Leben der Menschen geworden. Viele Menschen können jedoch auf ein Problem stoßen: Sie können sich gleichzeitig auf demselben Mobiltelefon bei mehreren WeChat-Konten anmelden. Für Huawei-Mobiltelefonbenutzer ist es nicht schwierig, eine doppelte WeChat-Anmeldung zu erreichen. In diesem Artikel wird erläutert, wie eine doppelte WeChat-Anmeldung auf Huawei-Mobiltelefonen erreicht wird. Erstens bietet das EMUI-System, das mit Huawei-Mobiltelefonen geliefert wird, eine sehr praktische Funktion – das doppelte Öffnen von Anwendungen. Durch die doppelte Öffnungsfunktion der Anwendung können Benutzer gleichzeitig
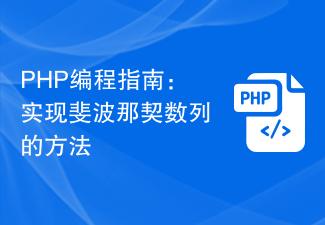
Die Programmiersprache PHP ist ein leistungsstarkes Werkzeug für die Webentwicklung, das eine Vielzahl unterschiedlicher Programmierlogiken und Algorithmen unterstützen kann. Unter diesen ist die Implementierung der Fibonacci-Folge ein häufiges und klassisches Programmierproblem. In diesem Artikel stellen wir vor, wie Sie die Fibonacci-Folge mit der Programmiersprache PHP implementieren, und fügen spezifische Codebeispiele bei. Die Fibonacci-Folge ist eine mathematische Folge, die wie folgt definiert ist: Das erste und das zweite Element der Folge sind 1, und ab dem dritten Element ist der Wert jedes Elements gleich der Summe der beiden vorherigen Elemente. Die ersten paar Elemente der Sequenz
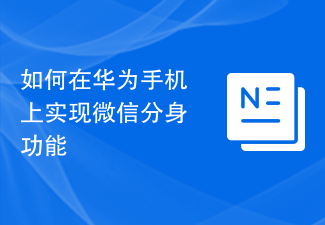
So implementieren Sie die WeChat-Klonfunktion auf Huawei-Mobiltelefonen Mit der Popularität sozialer Software und der zunehmenden Bedeutung von Datenschutz und Sicherheit rückt die WeChat-Klonfunktion allmählich in den Mittelpunkt der Aufmerksamkeit der Menschen. Die WeChat-Klonfunktion kann Benutzern helfen, sich gleichzeitig bei mehreren WeChat-Konten auf demselben Mobiltelefon anzumelden, was die Verwaltung und Nutzung erleichtert. Es ist nicht schwierig, die WeChat-Klonfunktion auf Huawei-Mobiltelefonen zu implementieren. Sie müssen lediglich die folgenden Schritte ausführen. Schritt 1: Stellen Sie sicher, dass die Version Ihres Mobiltelefonsystems und die WeChat-Version den Anforderungen entsprechen. Stellen Sie zunächst sicher, dass die Version Ihres Huawei-Mobiltelefonsystems sowie die WeChat-App auf die neueste Version aktualisiert wurden.
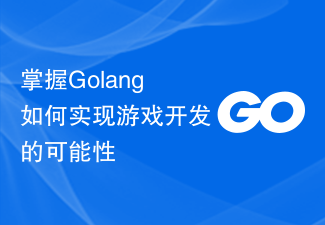
Im heutigen Bereich der Softwareentwicklung wird Golang (Go-Sprache) als effiziente, prägnante und hochgradig parallele Programmiersprache von Entwicklern zunehmend bevorzugt. Seine umfangreiche Standardbibliothek und die effizienten Parallelitätsfunktionen machen es zu einer hochkarätigen Wahl im Bereich der Spieleentwicklung. In diesem Artikel wird untersucht, wie man Golang für die Spieleentwicklung verwendet, und seine leistungsstarken Möglichkeiten anhand spezifischer Codebeispiele demonstriert. 1. Golangs Vorteile bei der Spieleentwicklung: Als statisch typisierte Sprache wird Golang beim Aufbau großer Spielsysteme verwendet.
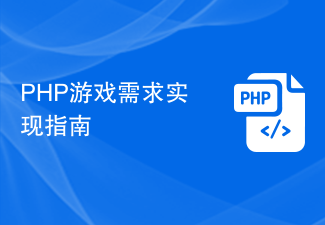
Implementierungsleitfaden für PHP-Spielanforderungen Mit der Popularität und Entwicklung des Internets erfreut sich der Markt für Webspiele immer größerer Beliebtheit. Viele Entwickler hoffen, die PHP-Sprache zur Entwicklung ihrer eigenen Webspiele nutzen zu können, und die Umsetzung der Spielanforderungen ist ein wichtiger Schritt. In diesem Artikel wird erläutert, wie Sie mithilfe der PHP-Sprache allgemeine Spielanforderungen implementieren und spezifische Codebeispiele bereitstellen. 1. Spielfiguren erstellen In Webspielen sind Spielfiguren ein sehr wichtiges Element. Wir müssen die Attribute des Spielcharakters wie Name, Level, Erfahrungswert usw. definieren und Methoden für deren Bedienung bereitstellen
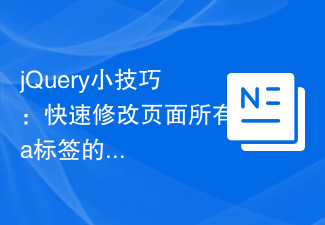
Titel: jQuery-Tipps: Ändern Sie schnell den Text aller Tags auf der Seite. In der Webentwicklung müssen wir häufig Elemente auf der Seite ändern und bedienen. Wenn Sie jQuery verwenden, müssen Sie manchmal den Textinhalt aller a-Tags auf der Seite gleichzeitig ändern, was Zeit und Energie sparen kann. Im Folgenden wird erläutert, wie Sie mit jQuery den Text aller Tags auf der Seite schnell ändern können, und es werden spezifische Codebeispiele angegeben. Zuerst müssen wir die jQuery-Bibliotheksdatei einführen und sicherstellen, dass der folgende Code in die Seite eingefügt wird: <
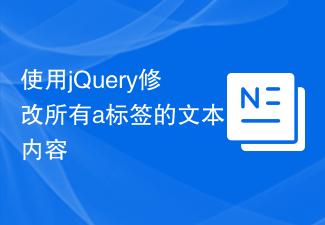
Titel: Verwenden Sie jQuery, um den Textinhalt aller Tags zu ändern. jQuery ist eine beliebte JavaScript-Bibliothek, die häufig zur Verarbeitung von DOM-Operationen verwendet wird. Bei der Webentwicklung müssen wir häufig den Textinhalt des Link-Tags (eines Tags) auf der Seite ändern. In diesem Artikel wird erläutert, wie Sie mit jQuery dieses Ziel erreichen, und es werden spezifische Codebeispiele bereitgestellt. Zuerst müssen wir die jQuery-Bibliothek in die Seite einführen. Fügen Sie den folgenden Code in die HTML-Datei ein:
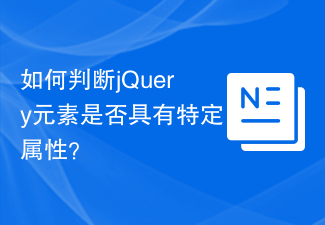
Wie kann man feststellen, ob ein jQuery-Element ein bestimmtes Attribut hat? Wenn Sie jQuery zum Betreiben von DOM-Elementen verwenden, stoßen Sie häufig auf Situationen, in denen Sie feststellen müssen, ob ein Element ein bestimmtes Attribut hat. In diesem Fall können wir diese Funktion einfach mit Hilfe der von jQuery bereitgestellten Methoden implementieren. Im Folgenden werden zwei häufig verwendete Methoden vorgestellt, um festzustellen, ob ein jQuery-Element über bestimmte Attribute verfügt, und um spezifische Codebeispiele anzuhängen. Methode 1: Verwenden Sie die Methode attr() und den Operator typeof //, um zu bestimmen, ob das Element ein bestimmtes Attribut hat
