Wellenformsortierung von Arrays mit Python
In diesem Artikel lernen wir ein Python-Programm zur Wellenformsortierung eines Arrays kennen.
Angenommen, wir haben ein unsortiertes Eingabearray. Wir werden nun das Eingabearray nach Wellenform sortieren. Wenn das Array 'arr [0..n-1]' arr [0] >= arr [1] = arr [3] = erfüllt . ...., das Array wird in eine Wellenform sortiert.
Verwendete Methoden
Hier sind die verschiedenen Methoden, mit denen diese Aufgabe erledigt wird &miinus;
Verwenden Sie die integrierte Funktion sort()
Ohne Verwendung integrierter Funktionen
Methode 1: Verwendung der integrierten Funktion sort()
Algorithmus (Schritte)
Im Folgenden sind die Algorithmen/Schritte aufgeführt, die zur Ausführung der gewünschten Aufgabe befolgt werden müssen
- Erstellen Sie eine Funktion zum Sortieren des Eingabearrays nach Wellenform und akzeptieren Sie das Eingabearray und die Arraylänge als Parameter.
- Verwenden Sie die Funktion
sort() (sortiert die Liste in aufsteigender/absteigender Reihenfolge), um das Eingabearray in aufsteigender Reihenfolge zu sortieren.
- Verwenden Sie alternativ die
for-Schleife, um bis zur Array-Länge zu durchlaufen (Schritt=2)
- Vertauschen Sie die benachbarten Elemente, d. h. das aktuelle und das nächste, mit dem Operator „,“.
- Erstellen Sie eine Variable zum Speichern des Eingabearrays.
- Verwenden Sie die Funktion
len() (die die Anzahl der Elemente im Objekt zurückgibt), um die Länge des Eingabearrays zu ermitteln.
- Rufen Sie die oben definierte Funktion
sortingInWaveform() auf, indem Sie das Eingabearray und die Länge des Arrays als Argumente übergeben
- Verwenden Sie die
for-Schleife, um alle Elemente des Arrays zu durchlaufen
- Drucken Sie das aktuelle Element eines Arrays.
Das folgende Programm sortiert das Eingabearray in Wellenform mithilfe der in Python integrierten Funktion sort() −
# creating a function to sort the array in waveform by accepting # the input array, array length as arguments def sortingInWaveform(inputArray, arrayLength): # sorting the input array in ascending order using the sort() function inputArray.sort() # travsersing till the array length alternatively(step=2) for k in range(0, arrayLength-1, 2): # swapping the adjacent elements i.e, current and it's next inputArray[k], inputArray[k+1] = inputArray[k+1], inputArray[k] # input array inputArray = [12, 45, 15, 4, 6, 70, 68, 3, 25] # getting the length of the input array arrayLength = len(inputArray) # printing the given array/list print("The Given list is:", inputArray) # calling the above defined sortingInWaveform() function by # passing input array, length of the array as arguments sortingInWaveform(inputArray, arrayLength) print("The Result Array after sorting in wave form is:") # traversing through all the elements of the array for k in range(0, arrayLength): # printing the current element of the array/list print(inputArray[k], end=" ")
Bei der Ausführung generiert das obige Programm die folgende Ausgabe &miinus;
The Given list is: [12, 45, 15, 4, 6, 70, 68, 3, 25] The Result Array after sorting in wave form is: 4 3 12 6 25 15 68 45 70
Zeitkomplexität − O(nLogn).
Hier wurde das angegebene Array mithilfe der Sortierfunktion sortiert, die normalerweise eine Zeitkomplexität von O(NlogN) aufweist.Wenn Sie einen O(nLogn)-Sortieralgorithmus wie
Merge Sort, Heap Sort usw. anwenden, beträgt die zeitliche Komplexität der oben angegebenen Methode O(nLogn).
Methode 2: Verwenden Sie nur eine SchleifeAlgorithmus (Schritte)
Im Folgenden sind die Algorithmen/Schritte aufgeführt, die zur Ausführung der gewünschten Aufgabe befolgt werden müssen
- Verwenden Sie die
- for-Schleife
, um alle geraden Indexelemente zu durchlaufen, indem Sie 0, Array-Länge und Schrittwert als Argumente übergeben
Verwenden Sie die Anweisung - if bedingt
, um zu prüfen, ob das aktuelle gerade Indexelement kleiner als das vorherige Element ist.
Tauschen Sie die Elemente, wenn die Bedingung - wahr ist.
- if-Bedingungsanweisung
, um zu überprüfen, ob das aktuelle gerade Indexelement kleiner als das nächste Element ist.
Tauschen Sie die Elemente, wenn die Bedingung - wahr ist.
- sortingInWaveform()
auf, indem Sie das Eingabearray und die Länge des Arrays als Argumente übergeben
Verwenden Sie die - for-Schleife
, um die Elemente des Arrays zu durchlaufen.
Drucken Sie das entsprechende Element des Arrays/der Liste aus.
Das folgende Programm sortiert das Eingabearray in Wellenform mit nur einer for-Schleife und ohne integrierte Funktionen −
# creating a function to sort the array in waveform by accepting # the input array, array length as arguments def sortingInWaveform(inputArray, arrayLength): # traversing through all the even index elements for p in range(0, arrayLength, 2): # checking whether the current even index element # is smaller than the previous if (p > 0 and inputArray[p] < inputArray[p-1]): # swapping the elements if the condition is true inputArray[p], inputArray[p-1] = inputArray[p-1], inputArray[p] # checking whether the current even index element # is smaller than the next element if (p < arrayLength-1 and inputArray[p] < inputArray[p+1]): # swapping the elements if the condition is true inputArray[p], inputArray[p+1] = inputArray[p+1], inputArray[p] # input array inputArray = [12, 45, 15, 4, 6, 70, 68, 3, 25] # getting the length of the input array arrayLength = len(inputArray) print("The Given list is:", inputArray) # calling the above defined sortingInWaveform() function by # passing input array, length of the array as arguments sortingInWaveform(inputArray, arrayLength) print("The Result Array after sorting in wave form is:") # traversing through all the elements of the array for k in range(0, arrayLength): # printing the current element print(inputArray[k], end=" ")
Ausgabe
Nachdem das obige Programm ausgeführt wurde, wird die folgende Ausgabe generiert -
The Given list is: [12, 45, 15, 4, 6, 70, 68, 3, 25] The Result Array after sorting in wave form is: 45 12 15 4 70 6 68 3 25
- O(n). Hier haben wir stattdessen nicht die Sortierfunktion verwendet, sondern nur die for-Schleife, um die Elemente des angegebenen Arrays zu durchlaufen, das im Durchschnitt eine Zeitkomplexität von O(N) aufweist.
Fazit
In diesem Artikel haben wir gelernt, wie man ein bestimmtes Array von Wellenformen mit zwei verschiedenen Methoden sortiert. Wir haben eine neue Logik verwendet, deren Zeitkomplexität im Vergleich zur ersten Methode um O(log N) reduziert ist. In vielen Fällen tragen solche Algorithmen dazu bei, die Zeitkomplexität zu reduzieren und effiziente Lösungen zu implementieren.
Das obige ist der detaillierte Inhalt vonWellenformsortierung von Arrays mit Python. Für weitere Informationen folgen Sie bitte anderen verwandten Artikeln auf der PHP chinesischen Website!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

AI Hentai Generator
Erstellen Sie kostenlos Ai Hentai.

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


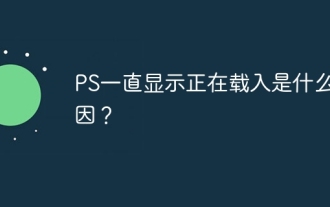
PS "Laden" Probleme werden durch Probleme mit Ressourcenzugriff oder Verarbeitungsproblemen verursacht: Die Lesegeschwindigkeit von Festplatten ist langsam oder schlecht: Verwenden Sie Crystaldiskinfo, um die Gesundheit der Festplatte zu überprüfen und die problematische Festplatte zu ersetzen. Unzureichender Speicher: Upgrade-Speicher, um die Anforderungen von PS nach hochauflösenden Bildern und komplexen Schichtverarbeitung zu erfüllen. Grafikkartentreiber sind veraltet oder beschädigt: Aktualisieren Sie die Treiber, um die Kommunikation zwischen PS und der Grafikkarte zu optimieren. Dateipfade sind zu lang oder Dateinamen haben Sonderzeichen: Verwenden Sie kurze Pfade und vermeiden Sie Sonderzeichen. Das eigene Problem von PS: Installieren oder reparieren Sie das PS -Installateur neu.
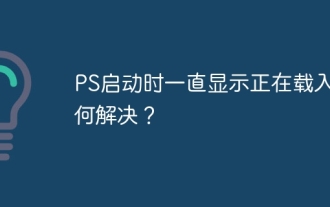
Ein PS, der beim Booten auf "Laden" steckt, kann durch verschiedene Gründe verursacht werden: Deaktivieren Sie korrupte oder widersprüchliche Plugins. Eine beschädigte Konfigurationsdatei löschen oder umbenennen. Schließen Sie unnötige Programme oder aktualisieren Sie den Speicher, um einen unzureichenden Speicher zu vermeiden. Upgrade auf ein Solid-State-Laufwerk, um die Festplatte zu beschleunigen. PS neu installieren, um beschädigte Systemdateien oder ein Installationspaketprobleme zu reparieren. Fehlerinformationen während des Startprozesses der Fehlerprotokollanalyse anzeigen.
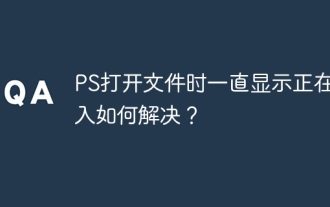
Das Laden von Stottern tritt beim Öffnen einer Datei auf PS auf. Zu den Gründen gehören: zu große oder beschädigte Datei, unzureichender Speicher, langsame Festplattengeschwindigkeit, Probleme mit dem Grafikkarten-Treiber, PS-Version oder Plug-in-Konflikte. Die Lösungen sind: Überprüfen Sie die Dateigröße und -integrität, erhöhen Sie den Speicher, aktualisieren Sie die Festplatte, aktualisieren Sie den Grafikkartentreiber, deinstallieren oder deaktivieren Sie verdächtige Plug-Ins und installieren Sie PS. Dieses Problem kann effektiv gelöst werden, indem die PS -Leistungseinstellungen allmählich überprüft und genutzt wird und gute Dateimanagementgewohnheiten entwickelt werden.
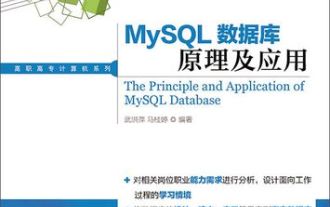
Der Artikel führt den Betrieb der MySQL -Datenbank vor. Zunächst müssen Sie einen MySQL -Client wie MySQLworkBench oder Befehlszeilen -Client installieren. 1. Verwenden Sie den Befehl mySQL-uroot-P, um eine Verbindung zum Server herzustellen und sich mit dem Stammkonto-Passwort anzumelden. 2. Verwenden Sie die Erstellung von Createdatabase, um eine Datenbank zu erstellen, und verwenden Sie eine Datenbank aus. 3.. Verwenden Sie CreateTable, um eine Tabelle zu erstellen, Felder und Datentypen zu definieren. 4. Verwenden Sie InsertInto, um Daten einzulegen, Daten abzufragen, Daten nach Aktualisierung zu aktualisieren und Daten nach Löschen zu löschen. Nur indem Sie diese Schritte beherrschen, lernen, mit gemeinsamen Problemen umzugehen und die Datenbankleistung zu optimieren, können Sie MySQL effizient verwenden.
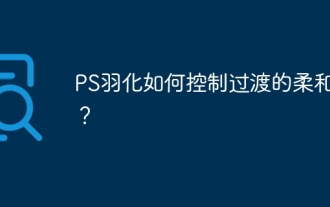
Der Schlüssel zur Federkontrolle liegt darin, seine allmähliche Natur zu verstehen. PS selbst bietet nicht die Möglichkeit, die Gradientenkurve direkt zu steuern, aber Sie können den Radius und die Gradientenweichheit flexius durch mehrere Federn, Matching -Masken und feine Selektionen anpassen, um einen natürlichen Übergangseffekt zu erzielen.
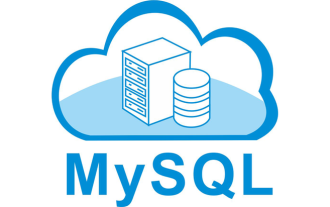
Die MySQL -Leistungsoptimierung muss von drei Aspekten beginnen: Installationskonfiguration, Indexierung und Abfrageoptimierung, Überwachung und Abstimmung. 1. Nach der Installation müssen Sie die my.cnf -Datei entsprechend der Serverkonfiguration anpassen, z. 2. Erstellen Sie einen geeigneten Index, um übermäßige Indizes zu vermeiden und Abfrageanweisungen zu optimieren, z. B. den Befehl Erklärung zur Analyse des Ausführungsplans; 3. Verwenden Sie das eigene Überwachungstool von MySQL (ShowProcessList, Showstatus), um die Datenbankgesundheit zu überwachen und die Datenbank regelmäßig zu sichern und zu organisieren. Nur durch kontinuierliche Optimierung dieser Schritte kann die Leistung der MySQL -Datenbank verbessert werden.
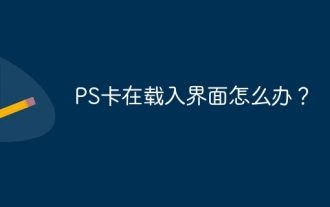
Die Ladeschnittstelle der PS-Karte kann durch die Software selbst (Dateibeschäftigung oder Plug-in-Konflikt), die Systemumgebung (ordnungsgemäße Treiber- oder Systemdateienbeschäftigung) oder Hardware (Hartscheibenbeschäftigung oder Speicherstickfehler) verursacht werden. Überprüfen Sie zunächst, ob die Computerressourcen ausreichend sind. Schließen Sie das Hintergrundprogramm und geben Sie den Speicher und die CPU -Ressourcen frei. Beheben Sie die PS-Installation oder prüfen Sie, ob Kompatibilitätsprobleme für Plug-Ins geführt werden. Aktualisieren oder Fallback die PS -Version. Überprüfen Sie den Grafikkartentreiber und aktualisieren Sie ihn und führen Sie die Systemdateiprüfung aus. Wenn Sie die oben genannten Probleme beheben, können Sie die Erkennung von Festplatten und Speichertests ausprobieren.
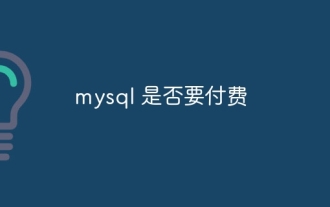
MySQL hat eine kostenlose Community -Version und eine kostenpflichtige Enterprise -Version. Die Community -Version kann kostenlos verwendet und geändert werden, die Unterstützung ist jedoch begrenzt und für Anwendungen mit geringen Stabilitätsanforderungen und starken technischen Funktionen geeignet. Die Enterprise Edition bietet umfassende kommerzielle Unterstützung für Anwendungen, die eine stabile, zuverlässige Hochleistungsdatenbank erfordern und bereit sind, Unterstützung zu bezahlen. Zu den Faktoren, die bei der Auswahl einer Version berücksichtigt werden, gehören Kritikalität, Budgetierung und technische Fähigkeiten von Anwendungen. Es gibt keine perfekte Option, nur die am besten geeignete Option, und Sie müssen die spezifische Situation sorgfältig auswählen.
