Methoden zur Klassen- und Instanzimplementierung in JavaScript
Dieser Artikel stellt hauptsächlich die Implementierungsmethoden von Klassen und Instanzen vor. Er hat einen gewissen Referenzwert. Die Details sind wie folgt 🎜>
In JavaScript gibt es kein Konzept für eine übergeordnete Klasse, eine Unterklasse oder eine Klasse und eine Instanz. Bei der Suche nach den Eigenschaften eines Objekts durchläuft JavaScript die Prototypenkette nach oben Die entsprechende Eigenschaft wird gefunden. Es gibt mehrere Möglichkeiten, die Konzepte von Klasse und Instanz zu simulieren.1. Verwenden Sie den Konstruktor direkt zum Erstellen des Objekts zur Objektinstanz.
function Animal() { this.name = "animal"; } Animal.prototype.makeSound = function() { console.log("animal sound"); } [Function] var animal1 = new Animal(); animal1.name; 'animal' animal1.makeSound(); animal sound
function Point(x, y) { this.x = x; this.y = y; } Point.prototype = { method1: function() { console.log("method1"); }, method2: function() { console.log("method2"); }, } { method1: [Function], method2: [Function] } var point1 = new Point(10, 20); point1.method1(); method1 point1.method2(); method2
2. Verwenden Sie die Methode Object.create(), um ein Objekt zu erstellen
var Animal = { name: "animal", makeSound: function() { console.log("animal sound"); }, } var animal2 = Object.create(Animal); animal2.name; 'animal' console.log(animal2.name); animal animal2.makeSound(); animal sound
3. Vom niederländischen Programmierer Gabor de Mooij vorgeschlagener minimalistischer Ansatz
var Animal = { init: function() { var animal = {}; animal.name = "animal"; animal.makeSound = function() { console.log("animal sound"); }; return animal; } }; var animal3 = Animal.init(); animal3.name; 'animal' animal3.makeSound(); animal sound
var Cat = { init: function() { var cat = Animal.init(); cat.name2 = "cat"; cat.makeSound = function() { console.log("cat sound"); }; cat.sleep = function() { console.log("cat sleep"); }; return cat; } } var cat = Cat.init(); cat.name; // 'animal' cat.name2; // 'cat' cat.makeSound(); // 类似于方法的重载 cat sound cat.sleep(); cat sleep
var Animal = { init: function() { var animal = {}; var sound = "private animal sound"; // 私有属性 animal.makeSound = function() { console.log(sound); }; return animal; } }; var animal4 = Animal.init(); Animal.sound; // undefined 私有属性只能通过对象自身的方法来读取. animal.sound; // undefined 私有属性只能通过对象自身的方法来读取 animal4.makeSound(); private animal sound
Zwischen Klassen und Instanzen können Sie einen Datenaustausch erreichen
var Animal = { sound: "common animal sound", init: function() { var animal = {}; animal.commonSound = function() { console.log(Animal.sound); }; animal.changeSound = function() { Animal.sound = "common animal sound changed"; }; return animal; } } var animal5 = Animal.init(); var animal6 = Animal.init(); Animal.sound; // 可以视为类属性 'common animal sound' animal5.sound; // 实例对象不能访问类属性 undefined animal6.sound; undefined animal5.commonSound(); common animal sound animal6.commonSound(); common animal sound animal5.changeSound(); // 修改类属性 undefined Animal.sound; 'common animal sound' animal5.commonSound(); common animal sound animal6.commonSound(); common animal sound
JavaScript-Video-Tutorial!

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


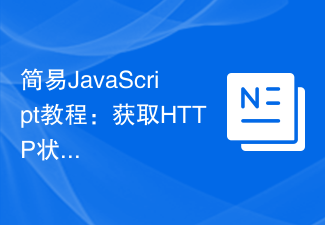
JavaScript-Tutorial: So erhalten Sie HTTP-Statuscode. Es sind spezifische Codebeispiele erforderlich. Vorwort: Bei der Webentwicklung ist häufig die Dateninteraktion mit dem Server erforderlich. Bei der Kommunikation mit dem Server müssen wir häufig den zurückgegebenen HTTP-Statuscode abrufen, um festzustellen, ob der Vorgang erfolgreich ist, und die entsprechende Verarbeitung basierend auf verschiedenen Statuscodes durchführen. In diesem Artikel erfahren Sie, wie Sie mit JavaScript HTTP-Statuscodes abrufen und einige praktische Codebeispiele bereitstellen. Verwenden von XMLHttpRequest
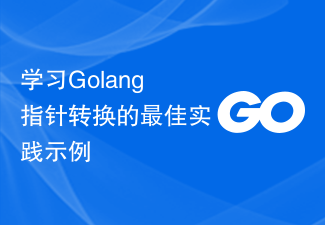
Golang ist eine leistungsstarke und effiziente Programmiersprache, mit der sich verschiedene Anwendungen und Dienste entwickeln lassen. In Golang sind Zeiger ein sehr wichtiges Konzept, das uns helfen kann, Daten flexibler und effizienter zu verwalten. Die Zeigerkonvertierung bezieht sich auf den Prozess der Zeigeroperation zwischen verschiedenen Typen. In diesem Artikel werden anhand konkreter Beispiele die Best Practices der Zeigerkonvertierung in Golang erläutert. 1. Grundkonzepte In Golang hat jede Variable eine Adresse, und die Adresse ist der Speicherort der Variablen im Speicher.
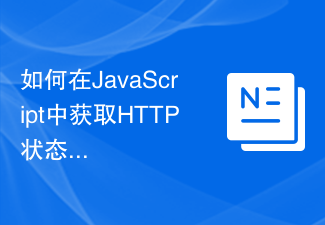
Einführung in die Methode zum Abrufen des HTTP-Statuscodes in JavaScript: Bei der Front-End-Entwicklung müssen wir uns häufig mit der Interaktion mit der Back-End-Schnittstelle befassen, und der HTTP-Statuscode ist ein sehr wichtiger Teil davon. Das Verstehen und Abrufen von HTTP-Statuscodes hilft uns, die von der Schnittstelle zurückgegebenen Daten besser zu verarbeiten. In diesem Artikel wird erläutert, wie Sie mithilfe von JavaScript HTTP-Statuscodes erhalten, und es werden spezifische Codebeispiele bereitgestellt. 1. Was ist ein HTTP-Statuscode? HTTP-Statuscode bedeutet, dass der Dienst den Dienst anfordert, wenn er eine Anfrage an den Server initiiert
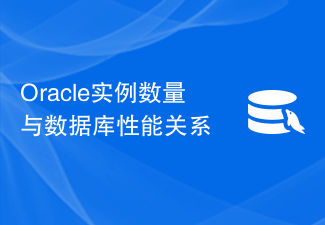
Die Beziehung zwischen der Anzahl der Oracle-Instanzen und der Datenbankleistung Oracle-Datenbank ist eines der bekanntesten relationalen Datenbankverwaltungssysteme in der Branche und wird häufig für die Datenspeicherung und -verwaltung auf Unternehmensebene verwendet. In Oracle-Datenbanken ist die Instanz ein sehr wichtiges Konzept. Instanz bezieht sich auf die laufende Umgebung der Oracle-Datenbank im Speicher. Jede Instanz verfügt über eine unabhängige Speicherstruktur und einen Hintergrundprozess, der zur Verarbeitung von Benutzeranforderungen und zur Verwaltung von Datenbankvorgängen verwendet wird. Die Anzahl der Instanzen hat einen wichtigen Einfluss auf die Leistung und Stabilität der Oracle-Datenbank.
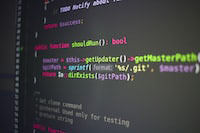
Was ist objektorientierte Programmierung? Objektorientierte Programmierung (OOP) ist ein Programmierparadigma, das reale Entitäten in Klassen abstrahiert und Objekte zur Darstellung dieser Entitäten verwendet. Klassen definieren die Eigenschaften und das Verhalten von Objekten und Objekte instanziieren Klassen. Der Hauptvorteil von OOP besteht darin, dass Code einfacher zu verstehen, zu warten und wiederzuverwenden ist. Grundkonzepte von OOP Zu den Hauptkonzepten von OOP gehören Klassen, Objekte, Eigenschaften und Methoden. Eine Klasse ist der Bauplan eines Objekts, der seine Eigenschaften und sein Verhalten definiert. Ein Objekt ist eine Instanz einer Klasse und verfügt über alle Eigenschaften und Verhaltensweisen der Klasse. Eigenschaften sind Merkmale eines Objekts, das Daten speichern kann. Methoden sind Funktionen eines Objekts, die mit den Daten des Objekts arbeiten können. Vorteile von OOP Zu den Hauptvorteilen von OOP gehören: Wiederverwendbarkeit: OOP kann den Code erweitern
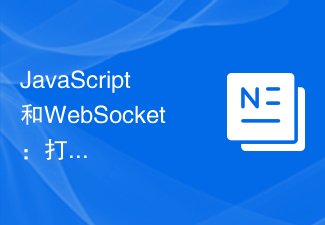
JavaScript und WebSocket: Aufbau einer effizienten Echtzeit-Suchmaschine Einführung: Mit der Entwicklung des Internets stellen Benutzer immer höhere Anforderungen an Echtzeit-Suchmaschinen. Bei der Suche mit herkömmlichen Suchmaschinen müssen Benutzer auf die Suchschaltfläche klicken, um Ergebnisse zu erhalten. Diese Methode kann den Anforderungen der Benutzer an Echtzeit-Suchergebnissen nicht gerecht werden. Daher ist die Verwendung von JavaScript und WebSocket-Technologie zur Implementierung von Echtzeitsuchmaschinen zu einem heißen Thema geworden. In diesem Artikel wird die Verwendung von JavaScript ausführlich vorgestellt
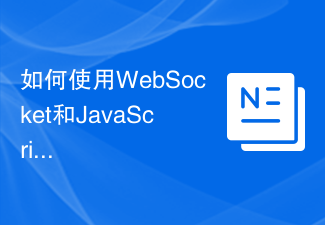
Überblick über die Verwendung von WebSocket und JavaScript zur Implementierung eines elektronischen Online-Signatursystems: Mit dem Aufkommen des digitalen Zeitalters werden elektronische Signaturen in verschiedenen Branchen häufig verwendet, um herkömmliche Papiersignaturen zu ersetzen. Als Vollduplex-Kommunikationsprotokoll kann WebSocket eine bidirektionale Datenübertragung in Echtzeit mit dem Server durchführen. In Kombination mit JavaScript kann ein elektronisches Online-Signatursystem implementiert werden. In diesem Artikel wird erläutert, wie Sie mit WebSocket und JavaScript ein einfaches Online-Programm entwickeln
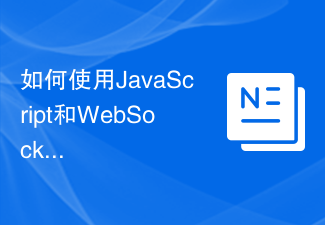
So implementieren Sie mit JavaScript und WebSocket ein Echtzeit-Online-Abstimmungssystem. Einführung: Mit der rasanten Entwicklung des Internets sind Echtzeit-Online-Abstimmungssysteme bei verschiedenen Aktivitäten und Wahlen zu einer sehr verbreiteten Form geworden. Die Verwendung von JavaScript und WebSocket-Technologie zur Implementierung eines Echtzeit-Online-Abstimmungssystems bietet die Vorteile von Flexibilität und Benutzerfreundlichkeit. In diesem Artikel wird detailliert beschrieben, wie Sie mithilfe von JavaScript und WebSocket ein einfaches Echtzeit-Online-Abstimmungssystem implementieren und den entsprechenden Code bereitstellen
