Yii框架模型类的实现以及PHP5动态语言特性的应用
Yii框架提供一个代码生成器gii, 我们一般用它来生成模型类代码。模型类是对数据(表)操作进行封装 不过在模型类中你看不到get/set属性的方法,甚至看不到和表字段关联的属性成员变量,但并不影响我们直接操作其属性,仿佛这些属性就在那里一样。 其具体实现
Yii框架提供一个代码生成器gii, 我们一般用它来生成模型类代码。模型类是对数据(表)操作进行封装
不过在模型类中你看不到get/set属性的方法,甚至看不到和表字段关联的属性成员变量,但并不影响我们直接操作其属性,仿佛这些属性就在那里一样。
其具体实现方式,正是一些设计模式和PHP5动态语言特性的一个很好的应用案例。
举个例子,如下一个用户模型类,对应的数据表为users
<?php class User extends CActiveRecord { /** * The followings are the available columns in table 'users': * @var double $Id * @var string $Username * @var string $Password * @var string $Email */ /** * Returns the static model of the specified AR class. * @return CActiveRecord the static model class */ public static function model($className='User') { return parent::model($className); } /** * @return string the associated database table name */ public function tableName() { return 'users'; } }
现在我们想读取一条user记录,首先当然得构造一个User对象
$user = new User();
可以执行一下var_dump($user),你会发现这个$user有个私有的_md属性(模型的元数据),该属性类型为CActiveRecordMetaData。
在这个_md变量中包含了数据表结构定义(Schema)。
究竟执行了什么代码,会构造出这样一个对象,并且读取了数据表结构定义,下面我们来跟踪一下:
1、和其他面向对象语言一样,在调用new创建一个对象时,首先会调用类的构造函数,如下:
/** * Constructor. * @param string $scenario scenario name. See {@link CModel::scenario} for more details about this parameter. */ public function __construct($scenario='insert') { if($scenario===null) // internally used by populateRecord() and model() return; $this->setScenario($scenario); $this->setIsNewRecord(true); $this->_attributes=$this->getMetaData()->attributeDefaults; $this->init(); ...... }
看起来模型对象元数据的构造以及数据表shema的读取和这个函数有关,继续
/** * Returns the meta-data for this AR * @return CActiveRecordMetaData the meta for this AR class. */ public function getMetaData() { if($this->_md!==null) return $this->_md; else return $this->_md=self::model(get_class($this))->_md; }
/** * Returns the static model of the specified AR class. * The model returned is a static instance of the AR class. */ public static function model($className=__CLASS__) { if(isset(self::$_models[$className])) return self::$_models[$className]; else { $model=self::$_models[$className]=new $className(null); $model->_md=new CActiveRecordMetaData($model); $model->attachBehaviors($model->behaviors()); return $model; } }
注意self::$_models是一个单例模式静态变量,你的应用所加载过的模型都被放在该对象数组中统一管理,你可以把它看作集中的模型对象管理器。看来,即使模型不再被实际使用,已经建立的模型对象也不会被释放。上述代码中创建了一个CActiveRecordMetaData对象,即前述的数据表Schema,注意User模型本身被作为其构造函数的参数被传递了进去,这里类似于应用了一种委托的模式,即模型类把获取数据表Schema的任务委托给CActiveRecordMetaData类。继续看下去,
/** * Constructor. * @param CActiveRecord $model the model instance */ public function __construct($model) { $this->_model=$model; $tableName=$model->tableName(); if(($table=$model->getDbConnection()->getSchema()->getTable($tableName))===null) throw new CDbException(Yii::t('yii','The table "{table}" for active record class "{class}" cannot be found in the database.', array('{class}'=>get_class($model),'{table}'=>$tableName))); ...... }
上述代码中getTable($tableName),这里应该是getTable('User')函数调用了CMysqlSchema的loadTable方法,最终通过SQL语句
SHOW FULL COLUMNS FROM ...
现在我们才刚刚了解到User对象中的元数据和缺省属性成员变量值是怎么来的。
接下来,才是动态语言特性相关部分,我们看看如何通过user对象,来所谓“动态”的操作数据属性的。
数据库中的users表中有Email字段,那么我们现在想给新创建的user对象的Email属性赋值,如下:
$user->Email = 'iefreer@hotmail.com';
如果是传统面向对象语言如c++/java,这里会报编译错误,因为User类没有定义Email成员变量。
而对于PHP5而言,由于语言对动态特性(魔法函数)的支持,这样的调用没有任何问题。我们看看它内部是怎么实现的。
如我之前的PHP语言动态特性文章中所言,设置对象的一个不存在的属性,会触发该对象的__set魔法函数:
/** * PHP setter magic method. * This method is overridden so that AR attributes can be accessed like properties. * @param string $name property name * @param mixed $value property value */ public function __set($name,$value) { if($this->setAttribute($name,$value)===false) { if(isset($this->getMetaData()->relations[$name])) $this->_related[$name]=$value; else parent::__set($name,$value); } }
上述代码中的setAttribute函数会把Email添加到$_attributes这个数组类型的成员变量中,也就是$_attributes充当了模型所对应的数据表属性动态管理器的功能。
再看读取user对象属性的语句:
$email = $user->Email;
类似的,该语句将触发CActiveRecord类的__get魔法函数,会返回$_attributes数组中相应属性的值。
by iefreer

Heiße KI -Werkzeuge

Undresser.AI Undress
KI-gestützte App zum Erstellen realistischer Aktfotos

AI Clothes Remover
Online-KI-Tool zum Entfernen von Kleidung aus Fotos.

Undress AI Tool
Ausziehbilder kostenlos

Clothoff.io
KI-Kleiderentferner

Video Face Swap
Tauschen Sie Gesichter in jedem Video mühelos mit unserem völlig kostenlosen KI-Gesichtstausch-Tool aus!

Heißer Artikel

Heiße Werkzeuge

Notepad++7.3.1
Einfach zu bedienender und kostenloser Code-Editor

SublimeText3 chinesische Version
Chinesische Version, sehr einfach zu bedienen

Senden Sie Studio 13.0.1
Leistungsstarke integrierte PHP-Entwicklungsumgebung

Dreamweaver CS6
Visuelle Webentwicklungstools

SublimeText3 Mac-Version
Codebearbeitungssoftware auf Gottesniveau (SublimeText3)

Heiße Themen


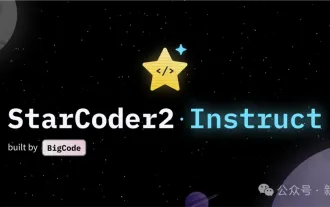
An der Spitze der Softwaretechnologie kündigte die Gruppe von UIUC Zhang Lingming zusammen mit Forschern der BigCode-Organisation kürzlich das StarCoder2-15B-Instruct-Großcodemodell an. Diese innovative Errungenschaft erzielte einen bedeutenden Durchbruch bei Codegenerierungsaufgaben, übertraf erfolgreich CodeLlama-70B-Instruct und erreichte die Spitze der Codegenerierungsleistungsliste. Die Einzigartigkeit von StarCoder2-15B-Instruct liegt in seiner reinen Selbstausrichtungsstrategie. Der gesamte Trainingsprozess ist offen, transparent und völlig autonom und kontrollierbar. Das Modell generiert über StarCoder2-15B Tausende von Anweisungen als Reaktion auf die Feinabstimmung des StarCoder-15B-Basismodells, ohne auf teure manuelle Annotationen angewiesen zu sein.

1. Einleitung In den letzten Jahren haben sich YOLOs aufgrund ihres effektiven Gleichgewichts zwischen Rechenkosten und Erkennungsleistung zum vorherrschenden Paradigma im Bereich der Echtzeit-Objekterkennung entwickelt. Forscher haben das Architekturdesign, die Optimierungsziele, Datenerweiterungsstrategien usw. von YOLO untersucht und erhebliche Fortschritte erzielt. Gleichzeitig behindert die Verwendung von Non-Maximum Suppression (NMS) bei der Nachbearbeitung die End-to-End-Bereitstellung von YOLO und wirkt sich negativ auf die Inferenzlatenz aus. In YOLOs fehlt dem Design verschiedener Komponenten eine umfassende und gründliche Prüfung, was zu erheblicher Rechenredundanz führt und die Fähigkeiten des Modells einschränkt. Es bietet eine suboptimale Effizienz und ein relativ großes Potenzial zur Leistungsverbesserung. Ziel dieser Arbeit ist es, die Leistungseffizienzgrenze von YOLO sowohl in der Nachbearbeitung als auch in der Modellarchitektur weiter zu verbessern. zu diesem Zweck
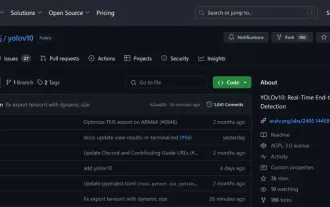
Die Benchmark-Zielerkennungssysteme der YOLO-Serie haben erneut ein großes Upgrade erhalten. Seit der Veröffentlichung von YOLOv9 im Februar dieses Jahres wurde der Staffelstab der YOLO-Reihe (YouOnlyLookOnce) in die Hände von Forschern der Tsinghua-Universität übergeben. Letztes Wochenende erregte die Nachricht vom Start von YOLOv10 die Aufmerksamkeit der KI-Community. Es gilt als bahnbrechendes Framework im Bereich Computer Vision und ist für seine End-to-End-Objekterkennungsfunktionen in Echtzeit bekannt. Es führt das Erbe der YOLO-Serie fort und bietet eine leistungsstarke Lösung, die Effizienz und Genauigkeit vereint. Papieradresse: https://arxiv.org/pdf/2405.14458 Projektadresse: https://github.com/THU-MIG/yo
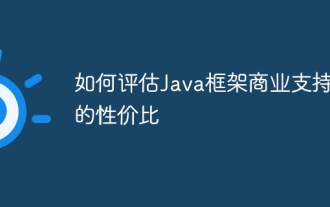
Die Bewertung des Kosten-/Leistungsverhältnisses des kommerziellen Supports für ein Java-Framework umfasst die folgenden Schritte: Bestimmen Sie das erforderliche Maß an Sicherheit und Service-Level-Agreement-Garantien (SLA). Die Erfahrung und das Fachwissen des Forschungsunterstützungsteams. Erwägen Sie zusätzliche Services wie Upgrades, Fehlerbehebung und Leistungsoptimierung. Wägen Sie die Kosten für die Geschäftsunterstützung gegen Risikominderung und Effizienzsteigerung ab.
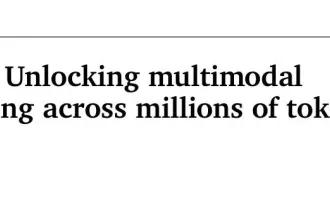
Im Februar dieses Jahres brachte Google das multimodale Großmodell Gemini 1.5 auf den Markt, das durch technische und Infrastrukturoptimierung, MoE-Architektur und andere Strategien die Leistung und Geschwindigkeit erheblich verbesserte. Mit längerem Kontext, stärkeren Argumentationsfähigkeiten und besserem Umgang mit modalübergreifenden Inhalten. Diesen Freitag hat Google DeepMind offiziell den technischen Bericht zu Gemini 1.5 veröffentlicht, der die Flash-Version und andere aktuelle Upgrades behandelt. Das Dokument ist 153 Seiten lang. Link zum technischen Bericht: https://storage.googleapis.com/deepmind-media/gemini/gemini_v1_5_report.pdf In diesem Bericht stellt Google Gemini1 vor
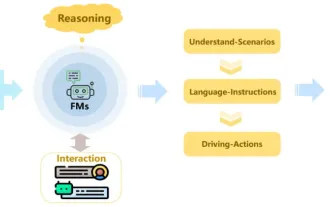
Oben geschrieben und das persönliche Verständnis des Autors: Mit der Entwicklung und den Durchbrüchen der Deep-Learning-Technologie haben kürzlich groß angelegte Grundlagenmodelle (Foundation Models) bedeutende Ergebnisse in den Bereichen natürlicher Sprachverarbeitung und Computer Vision erzielt. Große Entwicklungsperspektiven bietet auch die Anwendung von Basismodellen beim autonomen Fahren, die das Verständnis und die Argumentation von Szenarien verbessern können. Durch Vortraining mit umfangreichen Sprach- und visuellen Daten kann das Basismodell verschiedene Elemente in autonomen Fahrszenarien verstehen und interpretieren und Schlussfolgerungen ziehen, indem es Sprach- und Aktionsbefehle für die Entscheidungsfindung und Planung im Fahrbetrieb bereitstellt. Das Basismodell kann durch Datenergänzung mit einem Verständnis des Fahrszenarios ergänzt werden, um jene seltenen realisierbaren Merkmale in Long-Tail-Verteilungen bereitzustellen, die bei routinemäßigem Fahren und bei der Datenerfassung unwahrscheinlich anzutreffen sind.
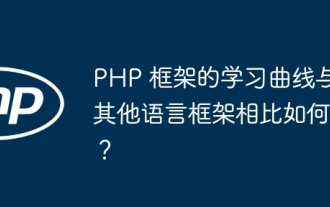
Die Lernkurve eines PHP-Frameworks hängt von Sprachkenntnissen, Framework-Komplexität, Dokumentationsqualität und Community-Unterstützung ab. Die Lernkurve von PHP-Frameworks ist im Vergleich zu Python-Frameworks höher und im Vergleich zu Ruby-Frameworks niedriger. Im Vergleich zu Java-Frameworks haben PHP-Frameworks eine moderate Lernkurve, aber eine kürzere Einstiegszeit.

Das leichte PHP-Framework verbessert die Anwendungsleistung durch geringe Größe und geringen Ressourcenverbrauch. Zu seinen Merkmalen gehören: geringe Größe, schneller Start, geringer Speicherverbrauch, verbesserte Reaktionsgeschwindigkeit und Durchsatz sowie reduzierter Ressourcenverbrauch. Praktischer Fall: SlimFramework erstellt eine REST-API, nur 500 KB, hohe Reaktionsfähigkeit und hoher Durchsatz
