


Example of using NG-BIND directive to implement one-way binding in ANGULARJS_AngularJS
After briefly introducing the AngularJS framework, this article uses an example to demonstrate the use of {{}} interpolation method and ng-bind directive.
Unlike jquery, which is just a class library that strengthens and simplifies front-end development, angularjs is a complete web front-end framework, so the learning curve is much higher.
Angularjs feels to me similar to Java's Spring framework. It is in the central container position and glues other components together. Many of its built-in components can already meet general scenarios. In special scenarios, we can expand according to the framework ideas.
Let’s start with the most basic content:
Directly output string literal value
Hello {{"World"}}
Use placeholders to output variables
Hello {{greeting}}
Use ng-bind directive to output variables
Hello
<script><br>
Function HelloController($scope) {<br>
$scope.greeting = "World";<br>
}<br>
</script>
ng-app declares an angularjs module and is limited to the scope of declaring html tags.
ng-controller declares an angularjs controller in the module. There can be multiple controllers, but the context is isolated, and the scope of the controller should be narrowed as much as possible.
{{}} is the interpolation syntax of angularjs, similar to JSP's EL expression ${}. The first output is because "World" is a literal value, and the program will output it directly; the second output is because greeting is a variable defined in the controller, so the value corresponding to the variable will also be output, which is also World; the third output The built-in ng-bind attribute directive of angularjs is used. The final result is equivalent to {{}}, but note that the directive = is followed by a string, so do not make a mistake.
The HelloController in js corresponds to the instructions on the body. The input parameter $scope is a service provided by the framework, which represents the context of the current controller. There are other similar services. The framework will automatically inject them. You will learn about them later. The method body has only one line and defines a variable on $scope, which is the variable referenced in the html code.
This article is very simple, just copy the code and it will run. Note that angular.min.js is the latest version of the 1.2 branch. The same code cannot be run with version 1.3.0. The reason is unknown. It may be related to 1.3.0 not being the final Release version.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Javascript is a very unique language. It is unique in terms of the organization of the code, the programming paradigm of the code, and the object-oriented theory. The issue of whether Javascript is an object-oriented language that has been debated for a long time has obviously been There is an answer. However, even though Javascript has been dominant for twenty years, if you want to understand popular frameworks such as jQuery, Angularjs, and even React, just watch the "Black Horse Cloud Classroom JavaScript Advanced Framework Design Video Tutorial".
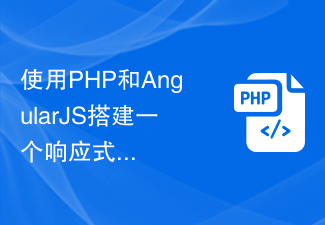
In today's information age, websites have become an important tool for people to obtain information and communicate. A responsive website can adapt to various devices and provide users with a high-quality experience, which has become a hot spot in modern website development. This article will introduce how to use PHP and AngularJS to build a responsive website to provide a high-quality user experience. Introduction to PHP PHP is an open source server-side programming language ideal for web development. PHP has many advantages, such as easy to learn, cross-platform, rich tool library, development efficiency
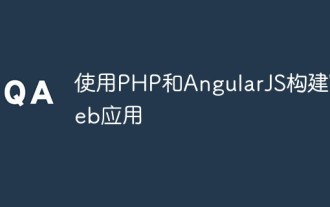
With the continuous development of the Internet, Web applications have become an important part of enterprise information construction and a necessary means of modernization work. In order to make web applications easy to develop, maintain and expand, developers need to choose a technical framework and programming language that suits their development needs. PHP and AngularJS are two very popular web development technologies. They are server-side and client-side solutions respectively. Their combined use can greatly improve the development efficiency and user experience of web applications. Advantages of PHPPHP
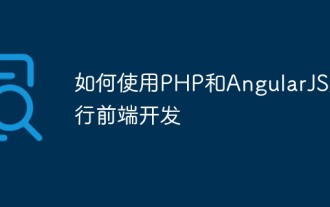
With the popularity and development of the Internet, front-end development has become more and more important. As front-end developers, we need to understand and master various development tools and technologies. Among them, PHP and AngularJS are two very useful and popular tools. In this article, we will explain how to use these two tools for front-end development. 1. Introduction to PHP PHP is a popular open source server-side scripting language. It is suitable for web development and can run on web servers and various operating systems. The advantages of PHP are simplicity, speed and convenience
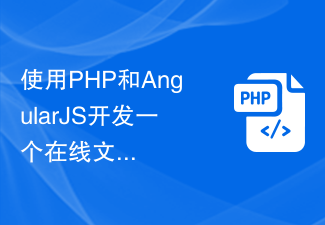
With the popularity of the Internet, more and more people are using the network to transfer and share files. However, due to various reasons, using traditional methods such as FTP for file management cannot meet the needs of modern users. Therefore, establishing an easy-to-use, efficient, and secure online file management platform has become a trend. The online file management platform introduced in this article is based on PHP and AngularJS. It can easily perform file upload, download, edit, delete and other operations, and provides a series of powerful functions, such as file sharing, search,
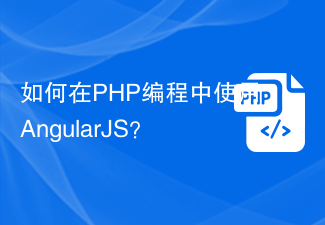
With the popularity of web applications, the front-end framework AngularJS has become increasingly popular. AngularJS is a JavaScript framework developed by Google that helps you build web applications with dynamic web application capabilities. On the other hand, for backend programming, PHP is a very popular programming language. If you are using PHP for server-side programming, then using PHP with AngularJS will bring more dynamic effects to your website.
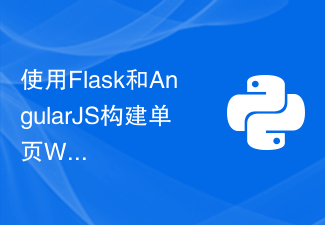
With the rapid development of Web technology, Single Page Web Application (SinglePage Application, SPA) has become an increasingly popular Web application model. Compared with traditional multi-page web applications, the biggest advantage of SPA is that the user experience is smoother, and the computing pressure on the server is also greatly reduced. In this article, we will introduce how to build a simple SPA using Flask and AngularJS. Flask is a lightweight Py
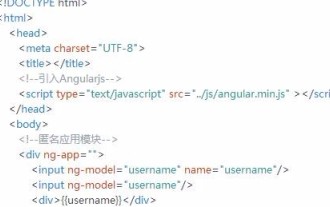
The content of this article is about the basic introduction to AngularJS. It has certain reference value. Now I share it with you. Friends in need can refer to it.
