PHP 面向对象程序设计(oop)学习笔记(一)
面向对象程序设计(OOP)是一种计算机编程架构。OOP的一条基本原则是计算机程序是由单个能够起到子程序作用的单元或对象组合而成。OOP达到了软件工程的三个主要
1、PHP中的抽象类
PHP 5 支持抽象类和抽象方法。定义为抽象的类不能被实例化。任何一个类,如果它里面至少有一个方法是被声明为抽象的,那么这个类就必须被声明为抽象的。被定义为抽象的方法只是声明了其调用方式(参数),不能定义其具体的功能实现。在类的声明中使用 abstract 修饰符可以将某个类声明为抽象的。
可以这样理解,抽象类作为一个基类,它把特定的细节留给继承者来实现。通过抽象概念,可以在开发项目中创建扩展性很好的架构。
复制代码 代码如下:
abstract class AbstractClass
{
code...
}
1.1、抽象方法
使用 abstract 关键字定义抽象方法。抽象方法只保留方法原型(方法的定义中剔除了方法体之后的签名),它包括存取级别、函数关键字、函数名称和参数。他不包含({})或者括号内部的任何代码。例如下面的代码就是一个抽象方法定义:
复制代码 代码如下:
abstract public function prototypeName($protoParam);
继承一个抽象类的时候,子类必须定义父类中的所有抽象方法;另外,这些方法的访问控制必须和父类中一样(或者更为宽松)。此外方法的调用方式必须匹配,即类型和所需参数数量必须一致。
1.2、关于抽象类
某个类只要包含至少一个抽象方法就必须声明为抽象类
声明为抽象的方法,在实现的时候必须包含相同的或者更低的访问级别。
不能使用 new 关键字创建抽象类的实例。
被声明为抽象的方法不能包含函数体。
如果将扩展的类也声明为抽象类,在扩展抽象类时,可以不用实现所有的抽象方法。(如果某个类从抽象类继承,当它没有实现基类中所声明的所有抽象方法时,它就必须也被声明为抽象的。)
1.3、使用抽象类
复制代码 代码如下:
abstract class Car
{
abstract function getMaxSpeend();
}
class Roadster extends Car
{
public $Speend;
public function SetSpeend($speend = 0)
{
$this->Speend = $speend;
}
public function getMaxSpeend()
{
return $this->Speend;
}
}
class Street
{
public $Cars ;
public $SpeendLimit ;
function __construct( $speendLimit = 200)
{
$this -> SpeendLimit = $speendLimit;
$this -> Cars = array();
}
protected function IsStreetLegal($car)
{
if ($car->getMaxSpeend() SpeendLimit)
{
return true;
}
else
{
return false;
}
}
public function AddCar($car)
{
if($this->IsStreetLegal($car))
{
echo 'The Car was allowed on the road.';
$this->Cars[] = $car;
}
else
{
echo 'The Car is too fast and was not allowed on the road.';
}
}
}
$Porsche911 = new Roadster();
$Porsche911->SetSpeend(340);
$FuWaiStreet = new Street(80);
$FuWaiStreet->AddCar($Porsche911);
/**
*
* @result
*
* The Car is too fast and was not allowed on the road.[Finished in 0.1s]
*
*/
?>
2.对象接口
使用接口(interface),,可以指定某个类必须实现哪些方法,但不需要定义这些方法的具体内容。
接口是通过 interface 关键字来定义的,就像定义一个标准的类一样,但其中定义所有的方法都是空的。
接口中定义的所有方法都必须是公有,这是接口的特性。
接口是一种类似于类的结构,可用于声明实现类所必须声明的方法。例如,接口通常用来声明API,而不用定义如何实现这个API。
大多数开发人员选择在接口名称前加上大写字母I作为前缀,以便在代码和生成的文档中将其与类区别开来。
2.1接口实现(implements)
要实现一个接口,使用 implements 操作符(继承抽象类需要使用 extends 关键字不同),类中必须实现接口中定义的所有方法,否则会报一个致命错误。类可以实现多个接口,用逗号来分隔多个接口的名称。
实现多个接口时,接口中的方法不能有重名。
接口也可以继承,通过使用 extends 操作符。
类要实现接口,必须使用和接口中所定义的方法完全一致的方式。否则会导致致命错误。
接口中也可以定义常量。接口常量和类常量的使用完全相同,但是不能被子类或子接口所覆盖。
2.2使用接口的案例
复制代码 代码如下:

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


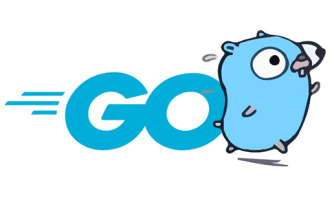
Golang has no abstract classes. Golang is not an object-oriented (OOP) language. It has no concepts of classes, inheritance, and abstract classes. However, there are structures (structs) and interfaces (interfaces) in golang, which can be indirectly implemented through the combination of struct and interface. Abstract classes in object languages.
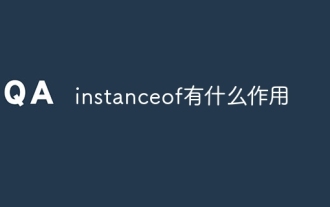
The function of instanceof is to determine whether an object is an instance of a certain class, or whether it implements a certain interface. instanceof is an operator used to check whether an object is of a specified type. Usage scenarios of instanceof operator: 1. Type checking: can be used to determine the specific type of an object, so as to perform different logic according to different types; 2. Interface judgment: can be used to determine whether an object implements an interface, so as to determine whether an object implements an interface. The definition of the interface calls the corresponding method; 3. Downward transformation, etc.

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
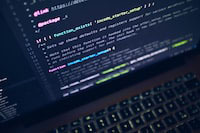
Interface Interface defines abstract methods and constants in Java. The methods in the interface are not implemented, but are provided by the class that implements the interface. The interface defines a contract that requires the implementation class to provide specified method implementations. Declare the interface: publicinterfaceExampleInterface{voiddoSomething();intgetSomething();} Abstract class An abstract class is a class that cannot be instantiated. It contains a mixture of abstract and non-abstract methods. Similar to interfaces, abstract methods in abstract classes are implemented by subclasses. However, abstract classes can also contain concrete methods, which provide default implementations. Declare abstract class: publicabstractcl
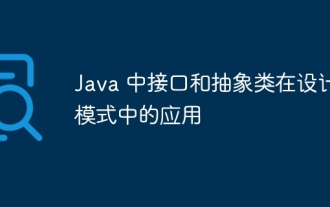
Interfaces and abstract classes are used in design patterns for decoupling and extensibility. Interfaces define method signatures, abstract classes provide partial implementation, and subclasses must implement unimplemented methods. In the strategy pattern, the interface is used to define the algorithm, and the abstract class or concrete class provides the implementation, allowing dynamic switching of algorithms. In the observer pattern, interfaces are used to define observer behavior, and abstract or concrete classes are used to subscribe and publish notifications. In the adapter pattern, interfaces are used to adapt existing classes. Abstract classes or concrete classes can implement compatible interfaces, allowing interaction with original code.
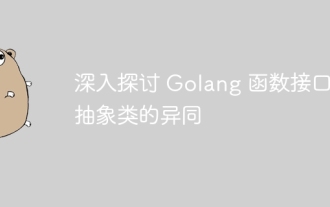
Both functional interfaces and abstract classes are used for code reusability, but they are implemented in different ways: functional interfaces through reference functions, abstract classes through inheritance. Functional interfaces cannot be instantiated, but abstract classes can. Functional interfaces must implement all declared methods, while abstract classes can only implement some methods.
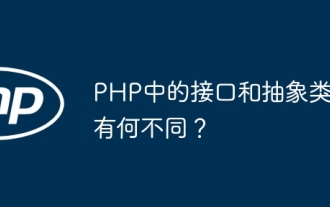
Interfaces and abstract classes are used to create extensible PHP code, and there is the following key difference between them: Interfaces enforce through implementation, while abstract classes enforce through inheritance. Interfaces cannot contain concrete methods, while abstract classes can. A class can implement multiple interfaces, but can only inherit from one abstract class. Interfaces cannot be instantiated, but abstract classes can.
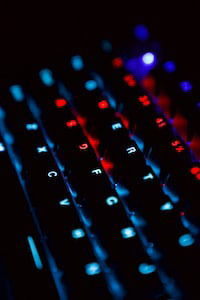
Interface: An implementationless contract interface defines a set of method signatures in Java but does not provide any concrete implementation. It acts as a contract that forces classes that implement the interface to implement its specified methods. The methods in the interface are abstract methods and have no method body. Code example: publicinterfaceAnimal{voideat();voidsleep();} Abstract class: Partially implemented blueprint An abstract class is a parent class that provides a partial implementation that can be inherited by its subclasses. Unlike interfaces, abstract classes can contain concrete implementations and abstract methods. Abstract methods are declared with the abstract keyword and must be overridden by subclasses. Code example: publicabstractcla
