


JavaScript framework design reading notes seed module_javascript skills
1. Namespace :
The namespace in js is extended using the properties of objects. For example, the user defines an A object. Under the A object, there are B attributes and C attributes. At the same time, the B attributes and C attributes are objects. Therefore, A={B:{},C:{}}, then the user can define the same methods and properties in the B object and the C object. Therefore B and C belong to different namespaces. When we call methods in objects B and C, we can call them through A.B.like() and A.C.like(). Of course A belongs to the properties in the window object.
But there is a situation, for example: boke.jsp page introduces jquery.js and prototype.js (they will add $ attribute to the window object), then a conflict occurs.
Therefore, there is noConflict() in jquery.js to handle conflicts. Execution process: The page first introduces prototype. At this time, prototype will occupy the $ attribute of window. Then when jquery is introduced, jquery will store the $ attribute of the previous window in _$, and then use the $ attribute itself. At this time, you can call the jquery method through $. When you don't need to use jquery now but want to use prototype, you can call $.noConflict(), and $ will be restored to the prototype object. At this time you can use the prototype method through $.
var _$ = window.$, _jQuery= window.jQuery;
noConflict:function(deep){
window.$ = _$;
if(deep) window.jQuery = _jQuery;
return jQuery;
}
2. Object extension :
Now that we have the namespace object, we need to extend the functionality. For example: I need to copy all the properties and methods of object A to object B. I don't have to write code in B objects one by one.
function mix(target, source){
var args = [].slice.call(arguments),i=1,
IScover = Typeof ARGS [ARGS.Length-] == "Boolean"? Args.pop (): True; // Do not write, the default is true, and the default is covered.
if(args.length == 1){ target = !this.window? this:{};
//If there is only one object parameter, expand this object. For example: I call mix(B) in the context of the A object, then this is A, so the properties and methods of B will be added to the A object. But if mix(B) is called in window, the properties and methods in the B object will be added to an empty object, and the empty object will be returned to prevent overwriting the properties and methods with the same name in the window object. (Only the window object has the window attribute)
i =0;
}
while((source = args[i ])){
for(key in source){
If (iSCOVER ||! (Key in target) // If it is covered, it will be directly assigned. If it does not cover, first determine whether the key is in the target object, if it exists, it will not assign a value
{
target[key] = source[key];
}
}
}
Return target;
}
3. Arrayization :
There are many array-like objects under the browser, such as arguments, document.forms, document.links, form.elements, document.getElementsByTagName, childNodes, etc. (HTMLCollection, NodeList).There is also a custom object written in a special way
var arrayLike = {
0:"a",
1:"b",
length:2
}
The way this object is written is the way jQuery objects are written.
We need to convert the above array-like object into an array object.
[].slice.call method can solve this problem. However, HTMLCollection and NodeList under the old version of IE are not subclasses of Object, and the [].slice.call method cannot be used.
So we can override a slice method.
A.slice = window.dispatchEvent ? function(nodes,start,end){ return [].slice.call(nodes,start,end); }
//If the window has dispatchEvent attribute, it proves that it supports the [].slice.call method and capability detection.
:function(nodes,start,end){
var ret = [],n=nodes.length;
Start = PARSEINT (Start, 10) || 0; // If the start does not exist or is not a number, it is assigned 0.
end = end == undefined ? if(start < 0) start = n;
if(end<0) end = n;
if(end>n) end = n;
for(var i = start;i
}
return ret;
}
The five simple data types of js are: null, undefined, boolean, number, and string.
There are also complex data types: Object, Function, RegExp, Date, custom objects, such as Person, etc.Typeof is generally used to determine boolean, number, string, and instanceof is generally used to determine object type. But they all have flaws. For example: the array instance in firame is not the Array instance of the parent window, and calling instanceof will return false. (This question was asked during the 360 school recruitment). typeof new Boolean(true) // "object" , packaging object. There are three packaging objects of boolean, number and string, which are discussed in js advanced programming.
Many people use typeof document.all to determine whether it is IE. In fact, this is very dangerous, because Google and Firefox also like this attribute, so this situation occurs under Google Chrome: typeof document.all // undefined However, document.all //HTMLAllCollection, judged by typeof to be undefined, but this attribute value can be read.
But now you can use the Object.prototype.toString.call method to determine the type. This method can directly output the [[Class]] inside the object. However, this method cannot be used for window objects in IE8 and below. You can use window == document // true document == window // false under IE6,7,8.
NodeType (1: Element 2: Attribute 3: Text Text 9: document)
Method used to determine type in jquery:
class2type ={}
jQuery.each("Boolean Number String Function Array Date RegExp Object".split(" "),function(i,name){
class2type [ "[object " name "]" ] = name.toLowerCase();
//class2type = {"[object Boolean]":boolean,"[object Number ]":number ,"[object String ]":string ,"[object Function ]":function ,"[object Array ]":array . .....}
});
jQuery.type = function(obj){ //If obj is null, undefined, etc., return the string "null", "undefined". If not, call the toString method, judge if it can be called, and return object (ActiveXobject objects such as window and Dom in lower versions of IE)
return obj == null ? String(obj) : class2type [ toString.call(obj) ] || "object";
}
5.domReady
When js operates dom nodes, the page must build a dom tree. Therefore, the window.onload method is usually used. But the onload method will not be executed until all resources are loaded. In order to make the page respond to user operations faster, we only need to use js operations after the DOM tree is constructed. There is no need to wait for all resources to be loaded (pictures, flash).
So the DOMContentLoaded event appears, which is triggered after the Dom tree is built. But the old version of IE does not support it, so there is a hack.
if(document.readyState === "complete"){ //In case the js file is loaded only after the Dom document is loaded. At this time, the fn method (the method you want to execute) is executed through this judgment. Because after the document is loaded, the value of document.readyState is complete
setTimeout(fn); setTimeout(fn); Here is the usage in jQuery, you don’t need to understand it.
}
else if(document.addEventListener){//Support DOMContentLoaded event
document.addEventListener("DOMContentLoaded",fn,false); // Bubble
window.addEventListener("load",fn,false); //In case the js file is loaded after the DOM tree is built. At this time, the DOMContentLoaded event will not be triggered (it has been triggered), only the load event
will be triggered. }
else{
document.attachEvent("onreadystatechange",function(){//For iframe security under IE, sometimes onload execution is given priority, sometimes not.
if(document.readyState ==="complete"){
fn();
}
});
window.attachEvent("onload",fn); //It will always work, in case other monitoring events are not obtained, so that at least the fn method can be triggered through the onload event.
var top = false;//Check if it is in iframe
Try{//window.frameElement is the iframe or frame object containing this page. If not, it is null.
top = window.frameElement == null && document.documentElement;
}catch(e){}
If(top && top.doScroll){ //If there is no iframe and it is IE
(function doScrollCheck(){
try{
top.doScroll("left");//Under IE, if the Dom tree is constructed, you can call the doScroll method of html
}catch(e){
Return settimeout (doscrollcheck, 50); // If it is not built, continue to listen to
}
fn();
})
}
}
The fn method must include removing all bound events.
Of course, IE can also use script defer hack. The principle is: the script with defer specified will not be executed until the DOM tree is built. But this requires adding additional js files, which is rarely used in a separate library.
Principle of use: Add a script tag to the document and use script.src = "xxx.js" to listen to the script's onreadystatechange event. When this.readyState == "complete", execute the fn method.
That is to say, after the DOM is built, xxx.js will be executed and its this.readyState will become complete.
The above are the reading notes for the first chapter of JavaScript framework design. The content is relatively concise, so that everyone can better understand the basic content of this chapter.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


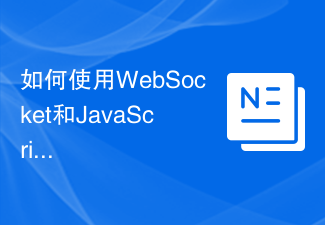
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
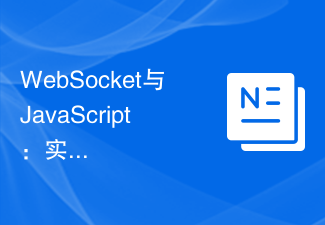
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
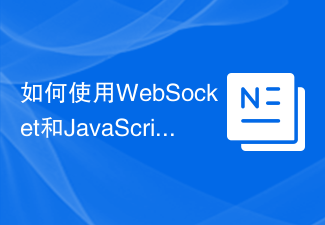
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
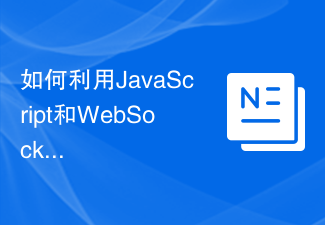
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
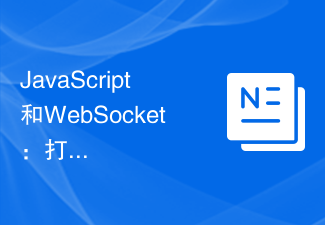
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
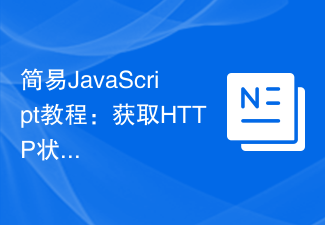
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
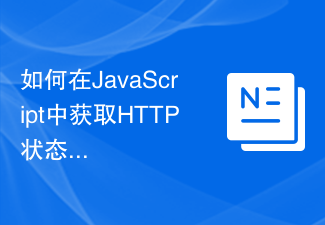
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
