jQuery event binding and delegate examples_jquery
The examples in this article describe jQuery event binding and delegation. Share it with everyone for your reference. The specific method is as follows:
JQuery event binding and delegation can be implemented using a variety of methods, on() , bind() , live() , delegate() , and one().
Sometimes we may bind an event like this:
alert("Trigger after click");
});
We can implement the above event binding in a variety of ways:
1. on()
$("p").on("click", function(){
alert( $(this).text() );
});
//There are data parameters
function myHandler(event) {
alert(event.data.foo);
}
$("p").on("click", {foo: "bar"}, myHandler)
Corresponding to on() is off(), which is used to remove event binding:
// code to handle some kind of event
};
// ... now foo will be called when paragraphs are clicked ...
$("body").on("click", "p", foo);
// ... foo will no longer be called.
$("body").off("click", "p", foo);
off(): remove the binding by on()
one(): only bind once.
2. bind()
Parameters:
(type,[data],function(eventObject))
type: A string containing one or more event types, with multiple events separated by spaces. For example, "click" or "submit", or a custom event name.
data: Additional data object passed to the event object as the event.data attribute value
fn: The handler function bound to the event of each matching element
(type,[data],false)
type: A string containing one or more event types, with multiple events separated by spaces. For example, "click" or "submit", or a custom event name.
data: Additional data object passed to the event object as the event.data attribute value
false: Setting the third parameter to false disables the default action.
Bind multiple event types at the same time:
$(this).toggleClass('entered');
});
Bind multiple event types/handlers simultaneously:
click:function(){$("p").slideToggle();},
mouseover:function(){$("body").css("background-color","red");},
mouseout:function(){$("body").css("background-color","#FFFFFF");}
});
You can pass some additional data before event handling.
alert(event.data.foo);
}
$("p").bind("click", {foo: "bar"}, handler)
Cancel the default behavior and prevent the event from bubbling by returning false.
Problems with bind
If there are 10 columns and 500 rows in the table to which click events are bound, then finding and traversing 5000 cells will cause the script execution speed to slow down significantly, and saving 5000 td elements and corresponding event handlers will also Takes up a lot of memory (similar to having everyone physically stand at the door waiting for a delivery).
Based on the previous example, if we want to implement a simple photo album application, each page only displays thumbnails of 50 photos (50 cells), and the user clicks "Page x" (or "Next Page") link can dynamically load another 50 photos from the server via Ajax. In this case, it seems that binding events for 50 cells using the .bind() method is acceptable again.
This is not the case. Using the .bind() method will only bind click events to 50 cells on the first page. Cells in subsequent pages that are dynamically loaded will not have this click event. In other words, .bind() can only bind events to elements that already exist when it is called, and cannot bind events to elements that will be added in the future (similar to how new employees cannot receive express delivery).
Event delegation can solve the above two problems. Specific to the code, just use the .live() method newly added in jQuery 1.3 instead of the .bind() method:
The .live() method here will bind the click event to the $(document) object (but this cannot be reflected from the code. This is also an important reason why the .live() method has been criticized. We will discuss it in detail later. ), and you only need to bind $(document) once (not 50 times, let alone 5000 times), and then you can handle the click event of the subsequent dynamically loaded photo cell. When receiving any event, the $(document) object will check the event type and event target. If it is a click event and the event target is td, then the handler delegated to it will be executed.
unbind(): Removes the binding performed by bind.
3. live()
So far, everything seems perfect. Unfortunately, this is not the case. Because the .live() method is not perfect, it has the following major shortcomings:
The $() function will find all td elements in the current page and create jQuery objects. However, this td element collection is not used when confirming the event target. Instead, a selector expression is used to compare with event.target or its ancestor elements, so Generating this jQuery object will cause unnecessary overhead;
By default, events are bound to the $(document) element. If the DOM nested structure is very deep, events bubbling through a large number of ancestor elements will cause performance losses;
It can only be placed after the directly selected element, and cannot be used after the consecutive DOM traversal method, that is, $("#info_table td").live... can be used, but $("#info_table").find("td" ).live...No;
Collect td elements and create jQuery objects, but the actual operation is the $(document) object, which is puzzling.
The solution
To avoid generating unnecessary jQuery objects, you can use a hack called "early delegation", which is to call .live() outside the $(document).ready() method:
$("#info_table td").live("click",function(){/*Show more information*/});
})(jQuery);
Here, (function($){...})(jQuery) is an "anonymous function that is executed immediately", forming a closure to prevent naming conflicts. Inside the anonymous function, the $parameter refers to the jQuery object. This anonymous function does not wait until the DOM is ready before executing. Note that when using this hack, the script must be linked and/or executed in the head element of the page. The reason for choosing this timing is that the document element is available at this time, and the entire DOM is far from being generated; if the script is placed in front of the closing body tag, it makes no sense, because the DOM is fully available at that time.
In order to avoid performance losses caused by event bubbling, jQuery supports the use of a context parameter when using the .live() method starting from 1.4:
In this way, the "trustee" changes from the default $(document) to $("#info_table")[0], saving the bubbling trip. However, the context parameter used with .live() must be a separate DOM element, so the context object is specified here using $("#info_table")[0], which is obtained using the array index operator. A DOM element.
4. delegate()
As mentioned earlier, in order to break through the limitations of the single .bind() method and implement event delegation, jQuery 1.3 introduced the .live() method. Later, in order to solve the problem of too long "event propagation chain", jQuery 1.4 supported specifying context objects for the .live() method. In order to solve the problem of unnecessary generation of element collections, jQuery 1.4.2 simply introduced a new method.delegate().
Using .delegate(), the previous example can be written like this:
Using .delegate() has the following advantages (or solves the following problems of the .live() method):
Directly bind the target element selector ("td"), event ("click") and handler to the "dragee" $("#info_table"), without additional collection of elements, shortened event propagation path, and clear semantics;
Supports calling after the consecutive DOM traversal method, that is, supports $("table").find("#info").delegate..., supporting precise control;
It can be seen that the .delegate() method is a relatively perfect solution. But when the DOM structure is simple, .live() can also be used.
Tip: When using event delegation, if other event handlers registered on the target element use .stopPropagation() to prevent event propagation, the event delegation will become invalid.
undelegate(): Remove the binding of delegate
I hope this article will be helpful to everyone’s jQuery programming.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


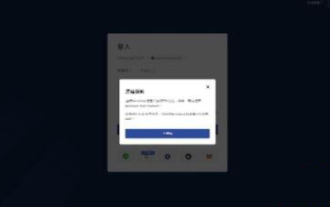
MetaMask (also called Little Fox Wallet in Chinese) is a free and well-received encryption wallet software. Currently, BTCC supports binding to the MetaMask wallet. After binding, you can use the MetaMask wallet to quickly log in, store value, buy coins, etc., and you can also get 20 USDT trial bonus for the first time binding. In the BTCCMetaMask wallet tutorial, we will introduce in detail how to register and use MetaMask, and how to bind and use the Little Fox wallet in BTCC. What is MetaMask wallet? With over 30 million users, MetaMask Little Fox Wallet is one of the most popular cryptocurrency wallets today. It is free to use and can be installed on the network as an extension
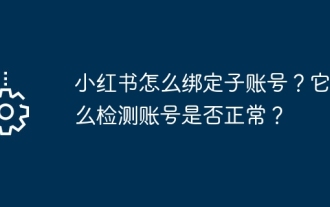
In today's era of information explosion, the construction of personal brand and corporate image has become increasingly important. As the leading fashion life sharing platform in China, Xiaohongshu has attracted a large number of user attention and participation. For those users who want to expand their influence and improve the efficiency of content dissemination, binding sub-accounts has become an effective means. So, how does Xiaohongshu bind a sub-account? How to check whether the account is normal? This article will answer these questions for you in detail. 1. How to bind a sub-account on Xiaohongshu? 1. Log in to your main account: First, you need to log in to your Xiaohongshu main account. 2. Open the settings menu: click "Me" in the upper right corner, and then select "Settings". 3. Enter account management: In the settings menu, find the "Account Management" or "Account Assistant" option and click
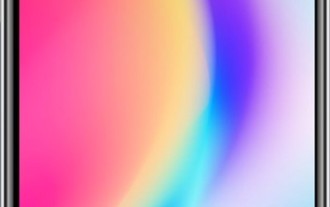
1. Open Toutiao. 2. Click My in the lower right corner. 3. Click [System Settings]. 4. Click [Account and Privacy Settings]. 5. Click the button on the right side of [Douyin] to bind Douyin.
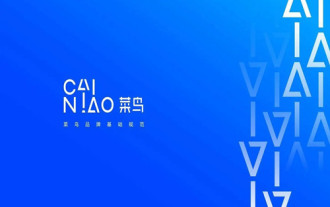
The Cainiao app is a platform that can provide you with various logistics information. The functions here are very powerful and easy to use. If you have any logistics-related problems, they can be solved here. Anyway, it can bring you a The one-stop service can solve everything in time. Checking the express delivery, picking up the express delivery, sending the express delivery, etc. are all without any problems. We have cooperated with various platforms and all the information can be queried. However, sometimes It will happen that the goods purchased on Pinduoduo cannot display the logistics information. In fact, you need to manually bind Pinduoduo to achieve this. The specific methods have been sorted out below, and everyone can take a look. . How to bind Cainiao to Pinduoduo account: 1. Open Cainiao APP and go to the main page
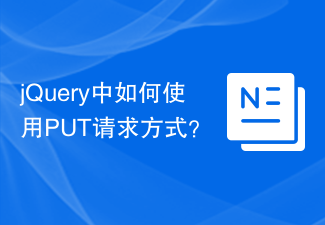
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
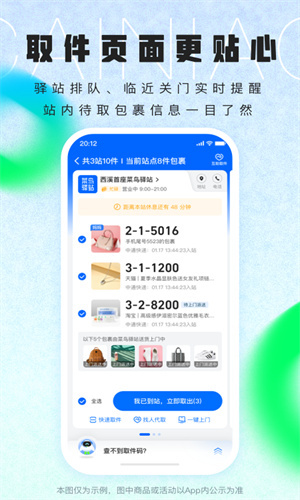
Do you know how to bind Pinduoduo when using Cainiao Wrap? The official version of Cainiao Wrap App does not automatically synchronize some Pinduoduo’s logistics information on this platform. All we need to do is You can copy the order number or check your mobile phone to see if there is any express delivery information. Of course, these all need to be completed manually. If you want to know more, come and take a look with the editor. How to bind the Cainiao APP to Pinduoduo 1. Open the Cainiao APP and click "Package Guide" in the upper left corner of the main page. 2. In the interface, there are many shopping websites, and accounts can be bound. 3. Click to import other e-commerce platforms. 4. User authorization: Click Pinduoduo to go to the interface
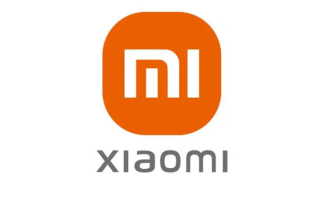
The latest Mi su7 model car launched by Xiaomi has dominated various hot search lists. Many users who happen to want to buy a car have chosen Xiaomi su7 model car for purchase. So how do you use your Xiaomi car app to bind the car after picking up the car? If you decide to use a home charging pile for charging, this tutorial guide will give you a detailed introduction, I hope it can help you. First, we open the Xiaomi mobile app, click the My button in the lower right corner, and then in the My interface, you can see the option of home charging pile. After entering the page to bind the charging pile, click the scan code button below and scan the QR code on the charging pile. The QR code can be used to bind the charging pile to the app.
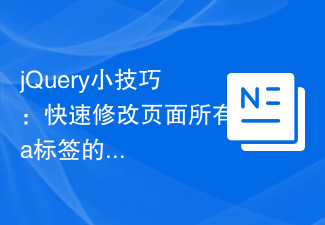
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
