JS Common Function Usage Guide_Basic Knowledge
1.document.write(""); Output statement
2. The comment in JS is //
3. The traditional order of HTML documents is: document->html->(head,body)
4. The DOM sequence in a browser window is: window->(navigator, screen, history, location, document)
5. Get the name and value of the element in the form: document.getElementById("ID number of the element in the form").name (or value)
6. A JS that converts lowercase to uppercase: document.getElementById("output").value = document.getElementById("input").value.toUpperCase();
7. Value types in JS: String, Number, Boolean, Null, Object, Function
8. Convert character type to numeric type in JS: parseInt(), parseFloat()
9. Convert numbers in JS to character type: ("" variable)
10. The length of string in JS is: (length)
11.Characters in JS are connected with characters using numbers.
12. The comparison operators in JS are: == equal to, != not equal to, >, >=, <.<=
13. Use: var to declare variables in JS
14. Judgment statement structure in JS: if(condition){}else{}
15. Loop structure in JS: for([initial expression];[condition];[upadte expression]) {inside loop}
16. The command to terminate the loop is: break
17. Function definition in JS: function functionName([parameter],...){statement[s]}
18. When multiple forms appear in the file, you can use document.forms[0], document.forms[1] instead.
19. Window: open window window.open(), close a window: window.close(), window itself: self
20. Status bar settings: window.status="character";
21. Pop-up prompt message: window.alert("Character");
22. Pop up confirmation box: window.confirm();
23. Pop up the input prompt box: window.prompt();
24. Specify the location of the currently displayed link: window.location.href="URL"
25. Get the number of all forms in the form: document.forms.length
26. Close the document’s output stream: document.close();
27. String append concatenation: =
28. Create a document element: document.createElement(), document.createTextNode()
29. Method to get elements: document.getElementById()
30. Set the value of all text members in the form to be empty:
var form = window.document.forms[0]
for (var i = 0; i
if (form.elements[i].type == "text"){
form.elements[i].value = "";
}
}
31. Determine whether the check button is checked in JS: document.forms[0].checkThis.checked (the checked attribute represents whether it is selected and returns TRUE or FALSE)
32. Radio button group (the names of the radio buttons must be the same): take the length of the radio button group document.forms[0].groupName.length
33. The radio button group also uses checked to determine whether it is selected.
34. The value of the drop-down list box: document.forms[0].selectName.options[n].value (n sometimes uses the name of the drop-down list box plus .selectedIndex to determine the selected value)
35. Definition of string: var myString = new String("This is lightsword");
36. Convert a string to uppercase: string.toUpperCase(); Convert a string to lowercase: string.toLowerCase();
37. Return the position where string 2 appears in string 1: String1.indexOf("String2")!=-1 means it was not found.
38. Get a character at the specified position in the string: StringA.charAt(9);
39. Take out the substring with specified starting point and end point in the string: stringA.substring(2,6);
40. Mathematical functions: Math.PI (returns pi), Math.SQRT2 (returns square root), Math.max(value1, value2) returns the greatest value of two numbers, Math.pow(value1,10 ) returns the tenth power of value1, Math.round(value1) rounding function, Math.floor(Math.random()*(n 1)) returns a random number
41. Define date variables: var today = new Date();
42. List of date functions: dateObj.getTime() gets the time, dateObj.getYear() gets the year, dateObj.getFullYear() gets the four-digit year, dateObj.getMonth() gets the month, dateObj.getDate() gets
Day, dateObj.getDay() gets the date, dateObj.getHours() gets the hours, dateObj.getMinutes() gets the minutes, dateObj.getSeconds() gets the seconds, dateObj.setTime(value) sets the time, dateObj.setYear (val) sets the year, dateObj.setMonth(val) sets the month, dateObj.setDate(val) sets the day, dateObj.setDay(val) sets the day of the week, dateObj.setHours sets the hours, dateObj.setMinutes(val) sets the minutes, dateObj .setSeconds(val) sets seconds [Note: This date and time starts from 0]
43.FRAME representation: [window.]frames[n].ObjFuncVarName,frames["frameName"].ObjFuncVarName,frameName.ObjFuncVarName
44.parent represents the parent object, and top represents the top object
45. The parent window that opens the child window is: opener
46. Indicates the current location: this
47. When calling a JS function in a hyperlink, use: (javascript:) to start with the function name
48. Do not execute this JS in old browsers:
49. Quote a file-style JS:
50. Specify HTML to be displayed in browsers that do not support scripts:
51. When there are both hyperlinks and ONCLICK events, the old version of the browser will redirect to a.html, otherwise it will redirect to b.html. Example: dfsadf
52. The built-in objects of JS are: Array, Boolean, Date, Error, EvalError, Function, Math, Number, Object, RangeError, ReferenceError, RegExp, String, SyntaxError, TypeError, URIError
53. Line breaks in JS:n
54. Window full screen size:<script>function fullScreen(){ this.moveTo(0,0);this.outerWidth=screen.availWidth;this.outerHeight=screen.availHeight;}</p> <p>window.maximize=fullScreen;</script>
55.all in JS represents all the elements below it
56.Focus order in JS: document.getElementByid("Form Element").tabIndex = 1
57. The value of innerHTML is the value of the form element: such as
"how are you"
, then the value of innerHTML is: how are you58.The value of innerTEXT is the same as above, except that tags like will not be displayed.
59.contentEditable can set whether the element can be modified, and isContentEditable returns whether the element can be modified.
60.isDisabled determines whether it is a prohibited state. disabled sets the prohibited state
61.length gets the length and returns an integer value
62.addBehavior() is an external function file called by JS. Its extension is .htc
63.window.focus() puts the current window in front of all windows.
64.blur() means losing focus. It is the opposite of FOCUS().
65.select() means that the element is selected.
66. Prevent users from entering text into the text box:
67. Get the number of occurrences of this element on the page: document.all.tags("div (or other HTML tags)").length
68.JS is divided into two types of form output: modal and non-modal.window.showModaldialog(), window.showModeless()
69. Status bar text setting: window.status='text', default status bar text setting: window.defaultStatus = 'text.';
70. Add to favorites: external.AddFavorite("http://www.xrss.cn","jaskdlf");
71. No action is taken when a script error is encountered in JS: window.onerror = doNothing; The syntax for specifying an error handle is: window.onerror = handleError;
72. Specify the parent window of the currently opened window in JS: window.opener, and support multiple continuations of opener.opener...
73.Self in JS refers to the current window
74. The content displayed in the status bar in JS: window.status="content"
75. The top in JS refers to the topmost frame in the frame set
76. Close the current window in JS: window.close();
77. Confirmation box in JS: if(confirm("Are you sure?")){alert("ok");}else{alert("Not Ok");}
78.Window redirection in JS: window.navigate("http://www.sina.com.cn");
79. Printing in JS: window.print()
Prompt input box in 80.JS: window.prompt("message","defaultReply");
Window scroll bar in 81.JS: window.scroll(x,y)
82.Scroll the window to the position in JS: window.scrollby
83. Set time interval in JS: setInterval("expr",msecDelay) or setInterval(funcRef,msecDelay) or setTimeout
84. The modal display in IE4 in JS does not work in NN: showModalDialog("URL"[,arguments][,features]);
85. The handle used before exiting in JS: function verifyClose(){event.returnValue="we really like you and hope you will stay longer.";}} window.onbeforeunload=verifyClose;
86. The file handle used when the form is called for the first time: onload()
87. File handle called when the form is closed: onunload()
Attributes of 88.window.location: protocol(http:),hostname(www.example.com),port(80),host(www.example.com:80),pathname("/a /a.html"), hash("#giantGizmo", refers to jumping to the corresponding anchor), href (all information)
89.window.location.reload() refreshes the current page.
89-1.parent.location.reload() refreshes the parent object (for frames)
89-2.opener.location.reload() refreshes the parent window object (for single-open window)
89-3.top.location.reload() refreshes the top object (for multiple windows)
90.window.history.back() returns to the previous page, window.history.forward() returns to the next page, window.history.go (return to which page, you can also use the visited URL)
91.document.write() output without line breaks, document.writeln() output with line breaks
92.document.body.noWrap=true; prevent link text from wrapping.
93. Variable name.charAt(number), get the character of the variable.
94."abc".charCodeAt(number), returns the ASCii code value of which character.
95. String concatenation: string.concat(string2), or use = to connect
96. Variable.indexOf("Character", starting position), returns the first appearing position (calculated from 0)
97.string.lastIndexOf(searchString[,startIndex]) The last occurrence position.
98.string.match(regExpression), determine whether the characters match.
99.string.replace(regExpression,replaceString) replaces the existing string.
100.string.split (separator) returns an array to store the value.
101.string.substr(start[,length]) takes the string from the digit to the specified length.
102.string.toLowerCase() makes the string all lowercase.
103.string.toUpperCase() makes all characters uppercase.
104.parseInt(string[,radix(representing base)]) is forced to convert to integer type.
105.parseFloat(string[,radix]) is forced to convert to floating point type.
106.isNaN (variable): Test whether it is a numeric type.
107. Keywords for defining constants: const, keywords for defining variables: var
In the end, I still didn’t get the one hundred and eight generals. I’m just a little sorry. Is it okay if my friends can help make up?

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


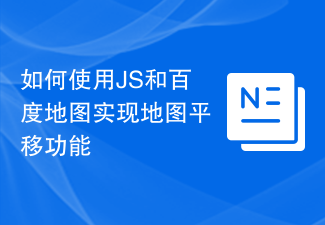
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
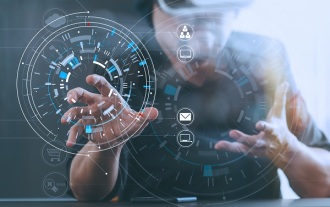
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
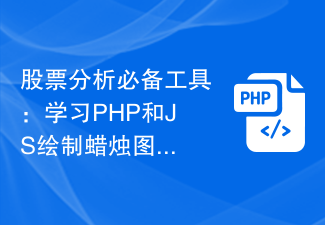
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
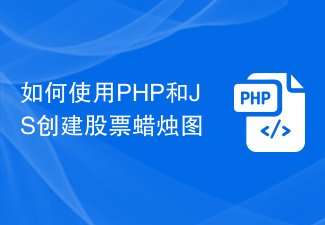
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
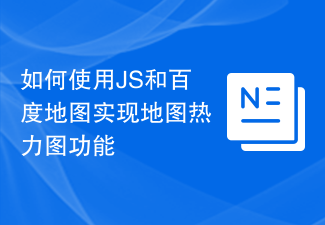
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
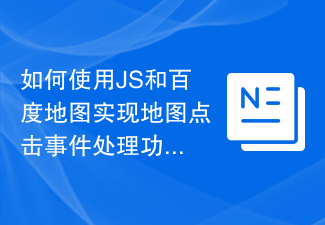
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
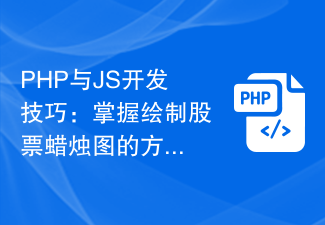
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
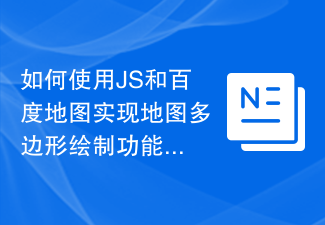
How to use JS and Baidu Maps to implement map polygon drawing function. In modern web development, map applications have become one of the common functions. Drawing polygons on the map can help us mark specific areas for users to view and analyze. This article will introduce how to use JS and Baidu Map API to implement map polygon drawing function, and provide specific code examples. First, we need to introduce Baidu Map API. You can use the following code to import the JavaScript of Baidu Map API in an HTML file
