


Use cluster to expand your Node server into a multi-threaded server_node.js
Friends who use nodejs all know that node is single-threaded, which means that it runs on an 8-core CPU and can only use the computing power of one core.
Single threading has always been a criticism of node, but with the introduction of cluster in version 0.6, this situation has changed. Developers can rely on cluster to easily expand their Node server into a multi-threaded server.
What is Cluster
Cluster is a multi-threading library provided by node. Users can use it to create multiple threads. The threads share a listening port. When there is an external request for this port, the cluster will forward the request to a random thread. Because each node thread will occupy dozens of megabytes of memory, you cannot create a thread for each request like PHP does. Generally speaking, the number of threads created will not exceed the number of cores of the CPU at most.
var cluster = require('cluster');
var http = require('http');
var numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
// Fork workers.
for (var i = 0; i < numCPUs; i ) {
cluster.fork();
}
cluster.on('exit', function(worker, code, signal) {
console.log('worker ' worker.process.pid ' died');
});
} else {
// Workers can share any TCP connection
// In this case its a HTTP server
http.createServer(function(req, res) {
res.writeHead(200);
res.end("hello worldn");
}).listen(8000);
}
As shown in the above code, cluster.isMaster will be set to true when the program is running. After calling cluster.fork(), the program will create a thread and run it again. At this time, cluster.isMaster will be set to false. . We mainly use this variable to determine whether the current thread is a child thread.
You can also notice that after each child thread is created, it will listen to port 8000 without causing conflicts. This is the function of cluster shared ports.
Communication between threads
When threads are created, they do not share memory or data with each other. All data exchanges can only be processed in the main thread through worker.send and worker.on('message', handler). Here is an example of a broadcast system.
var cluster = require('cluster');
var http = require('http');
var numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
var workers=[];
//Create new worker
function newWorker(){
var worker=cluster.fork();
//Listen to information, if the type is broadcast, it is determined to be broadcast
worker.on('message', function(msg) {
If(msg.type=='broadcast'){
var event=msg.event;
//Send this broadcast to all workers
workers.forEach(function(worker){
worker.send(event);
})
}
});
Return worker;
}
for (var i = 0; i < numCPUs; i ) {
Workers.push(newWorker());
}
cluster.on('online',function(worker){
console.log('worker %d is online',worker.id);
})
} else {
var worker=cluster.worker;
//Broadcast is to send a message of type broadcast, and event is the broadcast content
worker.broadcast=function(event){
worker.send({
Type:'broadcast',
event:event
});
}
//It seems that the returned information cannot be monitored using worker.on here
process.on('message',function(event){
console.log('worker: ' worker.id ' recived event from ' event.workerId);
})
//Send broadcast
worker.broadcast({
Message:'online',
workerId:worker.id
})
}
Issues that need attention
As mentioned above, data cannot be shared between threads, and all data exchange can only be exchanged through communication between threads. And the data exchanged is all serializable, so things like functions, file descriptors and HttpResponse cannot be passed.
If you use cluster, you need to consider the issue of data exchange during program design. My own approach is to store data like sessions in redis, and each thread does a good job of accessing it. None of the data is placed in node memory.
Last point, cluster is currently officially marked as Experimental by Node, and the API may change in the future.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
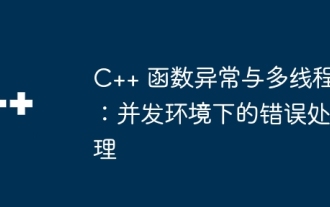
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
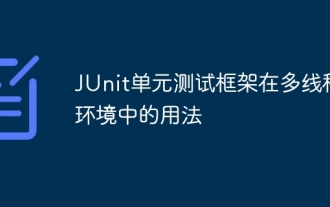
There are two common approaches when using JUnit in a multi-threaded environment: single-threaded testing and multi-threaded testing. Single-threaded tests run on the main thread to avoid concurrency issues, while multi-threaded tests run on worker threads and require a synchronized testing approach to ensure shared resources are not disturbed. Common use cases include testing multi-thread-safe methods, such as using ConcurrentHashMap to store key-value pairs, and concurrent threads to operate on the key-value pairs and verify their correctness, reflecting the application of JUnit in a multi-threaded environment.
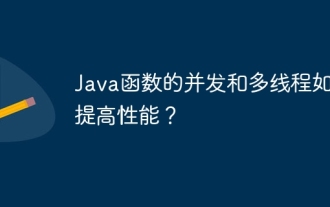
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
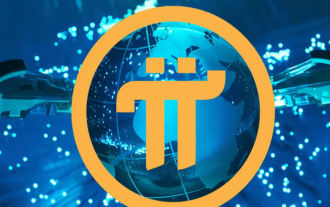
Detailed explanation and installation guide for PiNetwork nodes This article will introduce the PiNetwork ecosystem in detail - Pi nodes, a key role in the PiNetwork ecosystem, and provide complete steps for installation and configuration. After the launch of the PiNetwork blockchain test network, Pi nodes have become an important part of many pioneers actively participating in the testing, preparing for the upcoming main network release. If you don’t know PiNetwork yet, please refer to what is Picoin? What is the price for listing? Pi usage, mining and security analysis. What is PiNetwork? The PiNetwork project started in 2019 and owns its exclusive cryptocurrency Pi Coin. The project aims to create a one that everyone can participate
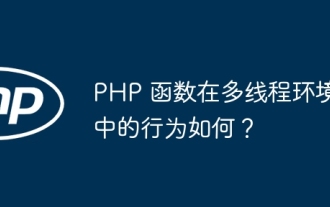
In a multi-threaded environment, the behavior of PHP functions depends on their type: Normal functions: thread-safe, can be executed concurrently. Functions that modify global variables: unsafe, need to use synchronization mechanism. File operation function: unsafe, need to use synchronization mechanism to coordinate access. Database operation function: Unsafe, database system mechanism needs to be used to prevent conflicts.
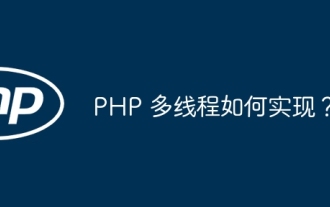
PHP multithreading refers to running multiple tasks simultaneously in one process, which is achieved by creating independently running threads. You can use the Pthreads extension in PHP to simulate multi-threading behavior. After installation, you can use the Thread class to create and start threads. For example, when processing a large amount of data, the data can be divided into multiple blocks and a corresponding number of threads can be created for simultaneous processing to improve efficiency.
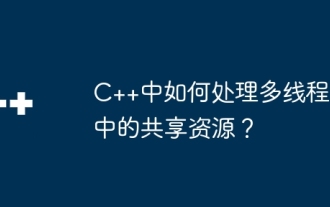
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
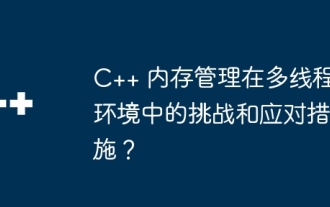
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
