


Specific implementation of js preventing event appending_javascript skills
May 16, 2016 pm 04:34 PMSometimes you can use e.stopPropagation(); e.preventDefault(); to prevent event bubbling and the execution of default events. But it cannot prevent the event from being added.
Under what circumstances should we prevent the addition of events?
For example:
Click "Checkout". When doing this, the checkout itself has its own event, but you must determine whether to log in before checking out.
We might write:
Js code
if(isLogin){ //Determine whether to log in
console.log("Not logged in")
}else{
//Checkout related code
}
If you click "My Homepage", there is also a login check
Login judgment code
if(isLogin){ //Determine whether to log in
console.log("Not logged in")
}else{
//Personal Center
}
If there are more logins to judge. Will there be more code like the above? Later I discovered the stopImmediatePropagation() method to prevent events from being appended. The above problem is no longer a problem.
Important: Make sure the login judgment event is the first bound event.
Demo code
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title>demo</title>
<script src="http://lib.sinaapp.com/js/jquery/1.7.2/jquery.min.js"></script>
</head>
<body>
<a href="#" class="bill isLogin">Checkout </a>
<ul>
<li class="a1 isLogin">Add to favorites</li>
<li class="a2 isLogin">Pay by others</li>
<li class="a3">Add to Cart</li>
<li class="a4 isLogin">My Homepage</li>
</ul>
<script>
//Bind first
$(".isLogin").on("click", function (e) {
if(true){ //Login judgment
alert("Not logged in");
e.stopImmediatePropagation();
}
return false;
});
$(".bill").on("click",function(){
alert("Checkout related content!");
});
$(".a1").on("click",function(){
alert("a1");
});
$(".a2").on("click",function(){
alert("a2");
});
$(".a3").on("click",function(){
alert("Added to cart");
});
$(".a4").on("click",function(){
alert("Login determination");
});
</script>
</body>
</html>
In fact, jquery provides us with the method $._data($('.isLogin').get(0)) to view events, open firebug and enter in the console.
Js code
$._data($('.isLogin').get(0))
You will see the following:
Js code
Object { events={...}, handle=function()}
Click to see the event array, making it easy to see what events are bound to the element.

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
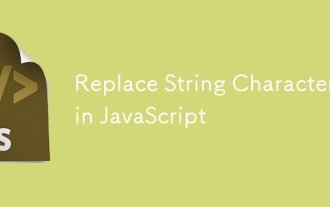
Replace String Characters in JavaScript
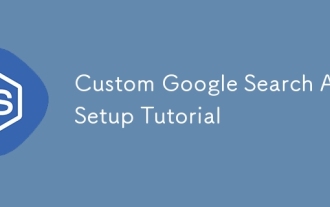
Custom Google Search API Setup Tutorial
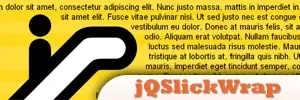
8 Stunning jQuery Page Layout Plugins
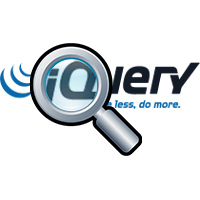
Improve Your jQuery Knowledge with the Source Viewer
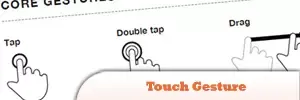
10 Mobile Cheat Sheets for Mobile Development
