A timer is needed in the project, the effect is as follows:
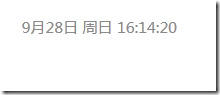
js code:
/*Get the current time*/
Function getCurrentDate()
{
var timeStr = '';
var curDate = new Date();
var curMonth = curDate.getMonth() 1; //Get the current month (0-11, 0 represents January)
var curDay = curDate.getDate(); //Get the current day (1-31)
var curWeekDay = curDate.getDay(); //Get the current week X (0-6, 0 represents Sunday)
var curHour = curDate.getHours(); var curHour = curDate.getHours(); var curHour = curDate.getHours(); var curHour = curDate.getHours();
var curMinute = curDate.getMinutes(); // Get the current minutes (0-59)
var curSec =curDate.getSeconds(); //Get the current number of seconds (0-59)
timeStr = curMonth 'month' curDay 'day week';
switch(curWeekDay)
{
case 0:timeStr = '日';break;
case 1:timeStr = '一';break;
case 2:timeStr = '二';break;
case 3:timeStr = '三';break;
case 4:timeStr = '四';break;
case 5:timeStr = '五';break;
case 6:timeStr = '六';break;
}
If(curHour < 10)
{
If(curMinute < 10)
{
if(curSec < 10)
{
timeStr = ' 0' curHour ':0' curMinute ':0' curSec;
}
else
{
timeStr = ' 0' curHour ':0' curMinute ':' curSec;
}
}
else
{
if(curSec < 10)
{
timeStr = ' 0' curHour ':' curMinute ':0' curSec;
}
else
{
timeStr = ' 0' curHour ':' curMinute ':' curSec;
}
}
}
else
{
If(curMinute < 10)
{
if(curSec < 10)
{
timeStr = ' ' curHour ':0' curMinute ':0' curSec;
}
else
{
timeStr = ' ' curHour ':0' curMinute ':' curSec;
}
}
else
{
if(curSec < 10)
{
timeStr = ' ' curHour ':' curMinute ':0' curSec;
}
else
{
timeStr = ' ' curHour ':' curMinute ':' curSec;
}
}
}
$("#time").text(timeStr);
}
Then just use this function.
Finally, a summary of some functions of Javascript date:
var myDate = new Date();
myDate.getYear(); //Get the current year (2 digits)
myDate.getFullYear(); //Get the complete year (4 digits, 1970-????)
myDate.getMonth(); //Get the current month (0-11, 0 represents January)
myDate.getDate(); //Get the current day (1-31)
myDate.getDay(); //Get the current week X (0-6, 0 represents Sunday)
myDate.getTime(); //Get the current time (number of milliseconds since 1970.1.1)
myDate.getHours(); //Get the current hours (0-23)
myDate.getMinutes(); //Get the current minutes (0-59)
myDate.getSeconds(); //Get the current seconds (0-59)
myDate.getMilliseconds(); //Get the current number of milliseconds (0-999)
myDate.toLocaleDateString(); //Get the current date
var mytime=myDate.toLocaleTimeString(); //Get the current time
myDate.toLocaleString( ); //Get date and time