


JavaScript method to determine whether a variable is an object or an array_javascript skills
typeof all returns object
All data types in JavaScript are objects in the strict sense, but in actual use we still have types. If you want to determine whether a variable is an array or an object, you can’t use typeof because it all returns object
var o = { 'name':'lee' };
var a = ['reg','blue'];
document.write( ' o typeof is ' typeof o);
document.write( '
');
document.write( ' a typeof is ' typeof a);
Execution:
o typeof is object
a typeof is object
Therefore, we can only give up this method. There are two ways to determine whether it is an array or an object
First, use typeof plus length attribute
Array has length attribute, object does not, and typeof array and object both return object, so we can judge this way
var o = { 'name':'lee' };
var a = ['reg','blue'];
var getDataType = function(o){
If(typeof o == 'object'){
If( typeof o.length == 'number' ){
return 'Array';
}else{
return 'Object'; }
}else{
return 'param is no object type';
}
};
alert( getDataType(o) ); // Object
alert( getDataType(a) ); // Array
alert( getDataType(1) ); // param is no object type
alert( getDataType(true) ); // param is no object type
alert( getDataType('a') ); // param is no object type
Second, use instanceof
Use instanceof to determine whether a variable is an array, such as:
var a = ['reg','blue'];
alert( a instanceof Array ); // true
alert( o instanceof Array ); // false
var a = ['reg','blue'];
alert( a instanceof Object ); // true
alert( o instanceof Object ); // true
var a = ['reg','blue'];
var getDataType = function(o){
If(o instanceof Array){
return 'Array'
}else if( o instanceof Object ){
return 'Object';
}else{
return 'param is no object type';
}
};
alert( getDataType(o) ); // Object
alert( getDataType(a) ); // Array
alert( getDataType(1) ); // param is no object type
alert( getDataType(true) ); // param is no object type
alert( getDataType('a') ); // param is no object type
If you don’t judge Array first, for example:
var o = { 'name':'lee' };
var a = ['reg','blue'];
var getDataType = function(o){
If(o instanceof Object){
return 'Object'
}else if( o instanceof Array ){
return 'Array';
}else{
return 'param is no object type';
}
};
alert( getDataType(o) ); // Object
alert( getDataType(a) ); // Object
alert( getDataType(1) ); // param is no object type
alert( getDataType(true) ); // param is no object type
alert( getDataType('a') ); // param is no object type
Then the array will also be judged as object.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
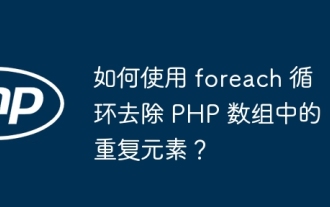
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.
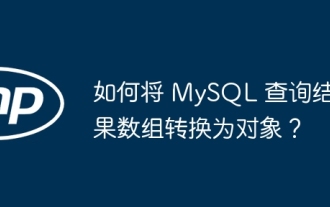
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
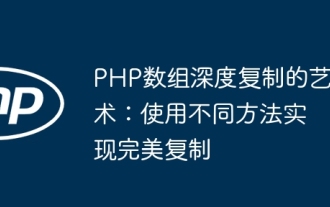
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.
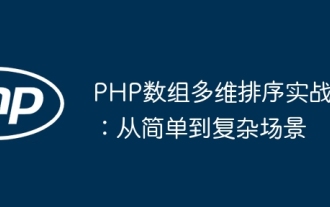
Multidimensional array sorting can be divided into single column sorting and nested sorting. Single column sorting can use the array_multisort() function to sort by columns; nested sorting requires a recursive function to traverse the array and sort it. Practical cases include sorting by product name and compound sorting by sales volume and price.
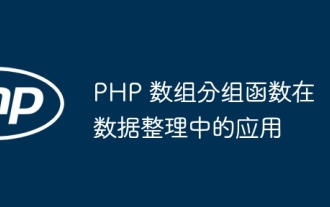
PHP's array_group_by function can group elements in an array based on keys or closure functions, returning an associative array where the key is the group name and the value is an array of elements belonging to the group.
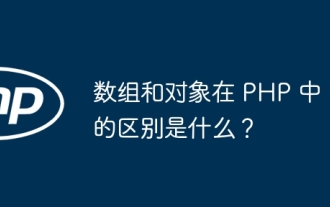
In PHP, an array is an ordered sequence, and elements are accessed by index; an object is an entity with properties and methods, created through the new keyword. Array access is via index, object access is via properties/methods. Array values are passed and object references are passed.
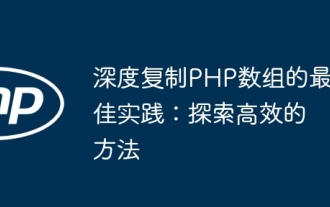
The best practice for performing an array deep copy in PHP is to use json_decode(json_encode($arr)) to convert the array to a JSON string and then convert it back to an array. Use unserialize(serialize($arr)) to serialize the array to a string and then deserialize it to a new array. Use the RecursiveIteratorIterator to recursively traverse multidimensional arrays.
