Example of use of getAttribute method in js_javascript skills
getAttribute() method
So far, we have introduced you to two methods of retrieving specific element nodes: one is to use the getElementById() method, and the other is to use the getElementsByTagName() method. After finding that element, we can use the getAttribute() method to query the values of its various attributes.
The getAttribute() method is a function. It has only one parameter - the name of the attribute you want to query:
object.getAttribute(attribute)
However, the getAttribute() method cannot be called through the document object, unlike the other methods we have introduced before. We can only call it through an element node object.
For example, you can combine it with the getElementsByTagName() method to query the title attribute of each
element, as follows:
var text=document.getElementsByTagName("p") for (var i=0;i<text.length;i++) { alert(text[i].getAttribute("title")); }
If you insert the above code at the end of the "Shopping List" example document given earlier, and reload the page in a web browser, a text message "a gentle reminder" will pop up on the screen. alter dialog box.
There is only one
element with a title attribute in the "Shopping List" document. If this document also has one or more
elements without a title attribute, the corresponding getAttribute("title") call will return null. null is the null value in the JavaScript language, which means "the thing you are talking about does not exist." If you want to verify this for yourself, first insert the following text into your Shopping List document after the existing text paragraph:
This is just test
Then reload the page. This time, you will see two alter dialog boxes, and the second dialog box will be blank or just display the word "null" - depending on how your web browser displays null values.
We can modify our script so that it only pops up a message when the title attribute exists. We will add an if statement to check if the return value of the getAttribute() method is null. Taking advantage of this opportunity, we also added a few variables to improve the readability of the script:
var ts=document.getElementsByTagName("li"); for (var i=0; i<ts.length;i++) {text=ts[i].getAttribute("title"); if(text!=null) { alert(text) } }
Now, if you reload this page, you will only see an alter dialog box with the message "a gentle reminder" as shown below.
We can even make this code shorter. When checking whether an item of data is null, we are actually checking whether it exists. This check can be simplified by directly using the data being checked as the condition of the if statement. if (something) is completely equivalent to if (something != null), but the former is obviously more concise. At this point, if something exists, the condition of the if statement will be true; if something does not exist, the condition of the if statement will be false.
Specific to this example, as long as we replace if (title_text != null) with if (title_text), we can get a more concise code. In addition, in order to further increase the readability of the code, we can also take this opportunity to write the alter statement and the if statement on the same line, which can make them closer to the English sentences in our daily life:
var ts=document.getElementsByTagName("li"); for (var i=0; i<ts.length;i++) {text=ts[i].getAttribute("title"); if(text) alert(text) }
3.4.2 setAttribute() method
All the methods we have introduced to you before can only be used to retrieve information. The setAttribute() method has one essential difference from them: it allows us to modify the value of the attribute node.
Similar to the getAttribute() method, the setAttribute() method is also a function that can only be called through the element node object, but the setAttribute() method requires us to pass it two parameters:
obiect.setAttribute(attribute,value)
In the following example, the first statement will retrieve the element whose id attribute value is purchase, and the second statement will set the title attribute value of this element to a list of goods:
var shopping=document.getElementById("purchases") shopping.setAttribute("title","a list of goods")
We can use the getAttribute() method to prove that the value of the title attribute of this element has indeed changed:
var shopping=document.getElementById("purchases"); alert(shopping.getAttribute("title")); shopping.setAttribute("title","a list of goods"); alert(shopping.getAttribute("title"));
The above statements will pop up two alert dialog boxes on the screen: the first alter dialog box appears before the setAttribute() method is called, it will be blank or display the word "null"; the second one appears After the title attribute value is set, it will display the "a list of goods" message.
In the above example, we set the title attribute of an existing node, but this attribute did not originally exist. This means that the setAttribute() call we issued actually completed two operations: first created the attribute, and then set its value. If we use the setAttribute() method on an existing attribute of an element node, the current value of this attribute will be overwritten.
In the "Shopping List" example document, the
element already has a title attribute whose value is a gentle reminder. We can use the setAttribute() method to change its current value:
<script type="text/javascript"> var ts=document.getElementsByTagName("li"); for (var i=0; i<ts.length;i++) { var text=ts[i].getAttribute("title"); alert(ts[i].getAttribute("title")) if(text) { ts[i].setAttribute("title","我会成功!") alert(ts[i].getAttribute("title")) } }
The above code will first retrieve all
elements with title attributes from the document, and then modify all their title attribute values to brand new title text. Specific to the "Shopping List" document, the attribute value a gentle reminder will be overridden.
There is a detail worthy of attention here: modifications to the document through the setAttribute() method will cause corresponding changes in the display effect and/or behavior of the document in the browser window, but when we use the browser's view When you view the source code of a document using the source option, you will still see the original attribute values - that is, changes made by the setAttribute() method will not be reflected in the source code of the document itself. This phenomenon of "inconsistency between appearance and inside" comes from the working mode of the DOM: first load the static content of the document, and then refresh them dynamically. Dynamic refresh does not affect the static content of the document. This is the true power and allure of the DOM: refreshing page content does not require the end user to perform a page refresh operation in their browser.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
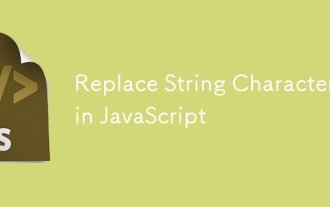
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
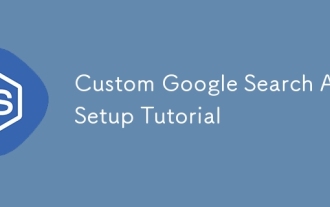
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
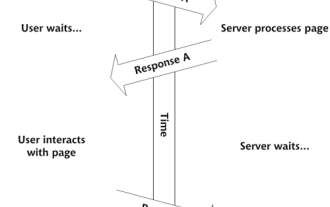
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
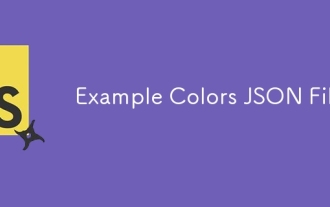
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
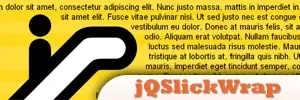
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
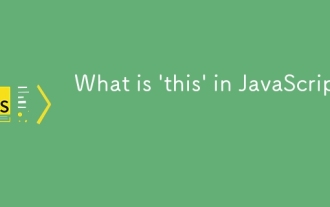
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
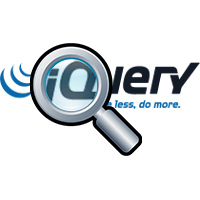
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
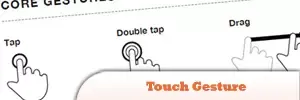
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
