


Javascript Study Notes - Objects (2): Prototype Object_Basic Knowledge
Javascript is the only language with widely used prototypal inheritance, so it takes time to understand the differences between the two inheritance methods.
The first major difference is that Javascript uses prototype chains for inheritance:
function Foo() { this.value = 42; } Foo.prototype = { method: function() {} }; function Bar() {}
Set Bar's prototype to an object instance of Foo:
Bar.prototype = new Foo(); Bar.prototype.foo = 'Hello World';
Make sure that the constructor of Bar is itself and create a new Bar object instance:
Bar.prototype.constructor = Bar; var test = new Bar();
Let’s take a look at the composition of the entire prototype chain:
test [instance of Bar] Bar.prototype [instance of Foo] { foo: 'Hello World' } Foo.prototype { method: ... } Object.prototype { toString: ... /* etc. */ }
In the above example, the object test will inherit both Bar.prototype and Foo.prototype. Therefore it has access to the function method defined in Foo. Of course, it can also access the property value. It should be mentioned that when new Bar() is called, it does not create a new Foo instance, but reuses the Foo instance that comes with its prototype object. Likewise, all Bar instances share the same value property. Let’s give an example:
test1 = new Bar(); test2 = new Bar(); Bar.prototype.value = 41; test1.value //41 test2.value//41
Prototype chain search mechanism
When accessing a property of an object, Javascript will traverse the entire prototype chain starting from the object itself until it finds the corresponding property. If the top of the prototype chain is reached at this time, which is Object.prototype in the above example, and the property to be found is still not found, then Javascript will return an undefined value.
Prototype object properties
Although the properties of the prototype object are used by Javascript to build the prototype chain, we can still assign values to it. But copying the original value to prototype is invalid, such as:
function Foo() {} Foo.prototype = 1; // no effect
Here is a digression from this article, introducing what is the original value:
In Javascript, variables can store two types of values, namely primitive values and reference values.
1.Primitive value:
Primitive values are fixed and simple values, which are simple data segments stored in the stack, that is, their values are stored directly at the locations where variables are accessed.
There are five primitive types: Undefined, Null, Boolean, Number, and String.
2. Reference value:
The reference value is a relatively large object, which is stored in the heap. In other words, the value stored in the variable is a pointer, pointing to the memory where the object is stored. All reference types are integrated from Object.
Prototype chain performance issues
If the property that needs to be looked up is located at the upper end of the prototype chain, then the lookup process will undoubtedly have a negative impact on performance. This will be an important factor to consider in scenarios where performance requirements are necessarily strict. Additionally, trying to find a property that doesn't exist will traverse the entire prototype chain.
Likewise, when traversing an object's properties, all properties on the prototype chain will be accessed.
Summary
Understanding prototypal inheritance is a prerequisite for writing more complex Javascript code. At the same time, pay attention to the height of the prototype chain in the code. Learn to split the prototype chain when facing performance bottlenecks. In addition, the prototype object prototype and the prototype __proto__ should be distinguished. The prototype object prototype is mainly discussed here and the issues about the prototype __proto__ will not be elaborated.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


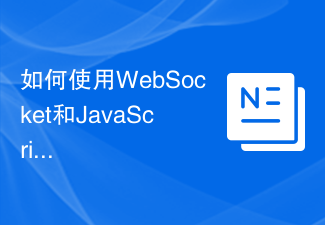
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
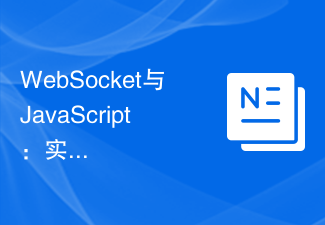
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
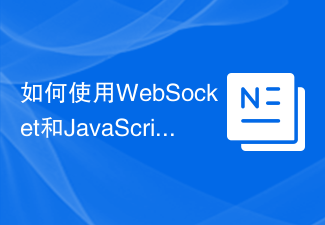
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
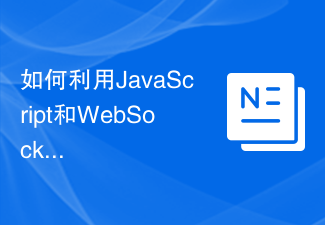
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
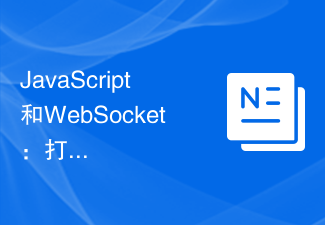
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
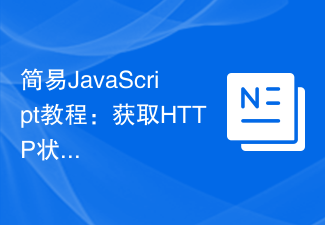
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
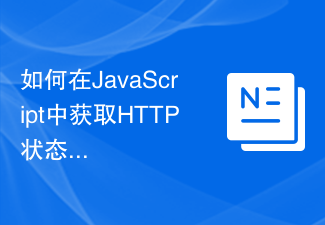
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
