


Examples of N ways to determine integer types in JavaScript_javascript skills
Integer type (Integer) often causes some strange problems in JavaScript. In the ECMAScript specification, they exist only as concepts:
All numbers are floating point numbers, and integers are just a set of numbers without decimals.
In this blog, I will explain how to check whether a value is an integer.
ECMAScript 5
There are many methods you can use in ES5. Sometimes, you may want to use your own method: a function isInteger(x), which returns true if it is an integer, otherwise it returns false.
Let’s look at some examples.
Pass remainder check
You can use the remainder operation (%) to calculate the remainder of a number by 1 to see if the remainder is 0.
function isInteger(x) { return x % 1 === 0; }
I like this method because it is very simple and effective.
> isInteger(17) true > isInteger(17.13) false
You have to be careful when operating remainder operations, because the result depends on the sign of the first number. If it is positive, the result is positive; otherwise, it is negative.
> 3.5 % 1 0.5 > -3.5 % 1 -0.5
Then, we can also check for 0, which is not really a problem. But the problem is: this method will also return true for non-numbers, because % will convert it to a number:
> isInteger('') true > isInteger('33') true > isInteger(false) true > isInteger(true) true
Can be solved with a very simple type check:
function isInteger(x) { return (typeof x === 'number') && (x % 1 === 0); }
Through Math.round(), if a number is the same as its previous value after rounding, then it is an integer. This can be checked via Math.round() in JavaScript:
function isInteger(x) { return Math.round(x) === x; }
This method is also good
> isInteger(17) true > isInteger(17.13) false
It can also determine non-numbers, because Math.round() always returns numbers, and === returns true only when the types are the same.
> isInteger('') false
If you want to make the code a little clearer, you can add type checking (which is what we did in previous versions). Additionally, Math.floor() and Math.ceil() work like Math.round(). Checking through bit operations Bit operators provide another way of "rounding":
function isInteger(x) { return (x | 0) === x; }
This solution (like other bitwise operations) has a flaw: it cannot handle numbers larger than 32 bits.
> isInteger(Math.pow(2, 32)) false
Check by parseInt() parseInt() also provides a method similar to Math.round() to convert numbers into integers. Let's see why this method is good.
function isInteger(x) { return parseInt(x, 10) === x; }
Like the Math.round() solution, it can also handle non-numeric cases, but it also does not handle all integer numbers correctly:
> isInteger(1000000000000000000000) false
Why? parseInt() forces the first argument to be parsed as a string before parsing an integer. Therefore using this method to convert numbers to integers is not a good choice.
> parseInt(1000000000000000000000, 10) 1 > String(1000000000000000000000) '1e+21'
Just like the above, parseInt() stopped processing at 1 when parsing '1e 21', so it will return 1. ECMAScript 6 In addition to Math.round(), ES6 provides another number Method to convert to integer: Math.trunc(). This function removes the decimal part of a number.
> Math.trunc(4.1) 4 > Math.trunc(4.9) 4 > Math.trunc(-4.1) -4 > Math.trunc(-4.9) -4
Additionally, ECMAScript 6 does not need to deal with the trivial task of checking integers because it comes with a built-in function Number.isInteger().

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


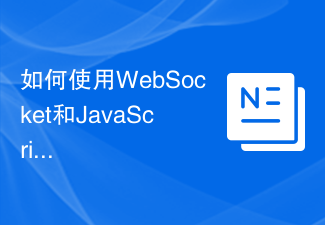
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
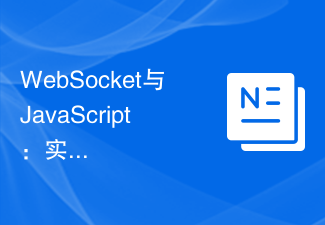
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
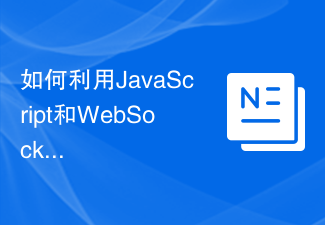
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
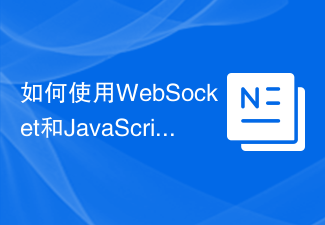
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
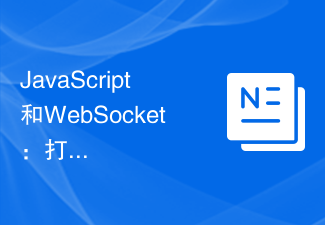
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
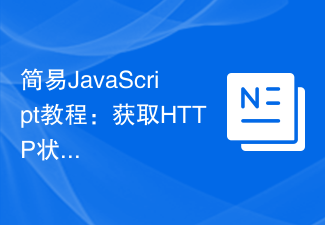
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
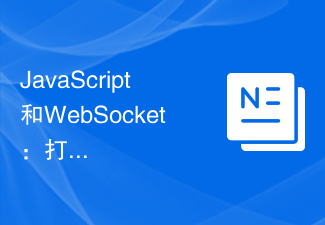
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
