Code to use jQuery to determine IE browser version_jquery
IE is really something that designers hate, and there are now five different versions of browsers: IE6, IE7, IE8, IE9, and IE10, and they all have slight differences. But there is no way, in order to make the web page display the same in every browser, it must be accommodated.
But now I am basically unwilling to be compatible with lower versions of IE. For example, IE6 and IE7 are simply ignored! IE8 is just fine. Fortunately, IE9 and IE10 have comparable support for network standards. It will be great when browsers IE9 and above become popular.
But if it is not compatible, it is not compatible. It still needs to be dealt with simply. Fortunately, jQuery provides the browser tag so that we can determine what browser and version the current visitor is using:
<script type="text/javascript" src="http://img.jb51.net/jslib/jquery/jquery.js"></script> <script type="text/javascript"> $(function() { var userAgent = window.navigator.userAgent.toLowerCase(); var version = $.browser.version; $(".info").html( "<h3>userAgent:</h3>" + userAgent + "<br />" + "<h3>version:</h3>" + version ); }); </script> <body> <div class="info"></div> </body>
I used a simple example to display the userAgent and jQuery.browser.version of the current browser, and then tested it in IE 6~8, but the results displayed were really shocking!
From the results, the judgment of IE 7 is wrong. When you look carefully at its userAgent, you will find that in addition to msie 7.0, it also contains msie 6.0, so the comparison of jQuery.browser.version is incorrect. Something is wrong. Now that we know the problem, we can solve it.
The first method is a more direct method. First determine if there is a higher version in userAgent, then it will be based on that version:
When you want to use it, you can use $.browser.msie6~10 for judgment processing. The other is to directly modify the comparison method of jQuery.browser.version:
<script type="text/javascript" src="http://img.jb51.net/jslib/jquery/jquery.js"></script> <script type="text/javascript"> $(function() { var userAgent = window.navigator.userAgent.toLowerCase(); $.browser.msie10 = $.browser.msie && /msie 10\.0/i.test(userAgent); $.browser.msie9 = $.browser.msie && /msie 9\.0/i.test(userAgent); $.browser.msie8 = $.browser.msie && /msie 8\.0/i.test(userAgent); $.browser.msie7 = $.browser.msie && /msie 7\.0/i.test(userAgent); $.browser.msie6 = !$.browser.msie8 && !$.browser.msie7 && $.browser.msie && /msie 6\.0/i.test(userAgent); $(".info").html( "<h3>userAgent:</h3>" + userAgent + "<br />" + "<h3>Is IE 10?</h3>" + $.browser.msie10 + "<h3>Is IE 9?</h3>" + $.browser.msie9 + "<h3>Is IE 8?</h3>" + $.browser.msie8 + "<h3>Is IE 7?</h3>" + $.browser.msie7 + "<h3>Is IE 6?</h3>" + $.browser.msie6 ); }); </script> <body> <div class="info"></div> </body>
After this correction, when we use jQuery.browser.version to judge, the version number of IE can be displayed correctly. Both methods have their convenience, it depends on which one you choose to use!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


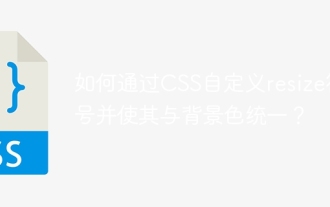
The method of customizing resize symbols in CSS is unified with background colors. In daily development, we often encounter situations where we need to customize user interface details, such as adjusting...
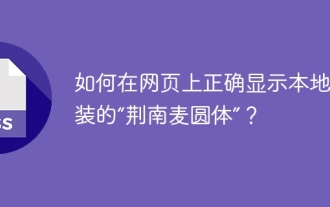
Using locally installed font files in web pages Recently, I downloaded a free font from the internet and successfully installed it into my system. Now...
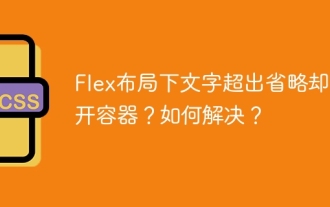
The problem of container opening due to excessive omission of text under Flex layout and solutions are used...
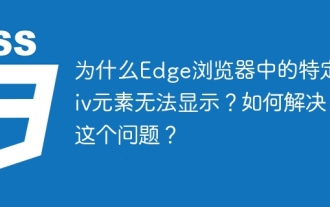
How to solve the display problem caused by user agent style sheets? When using the Edge browser, a div element in the project cannot be displayed. After checking, I posted...
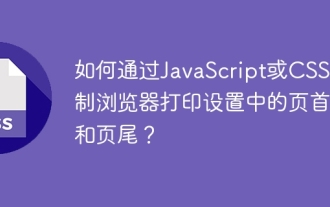
How to use JavaScript or CSS to control the top and end of the page in the browser's printing settings. In the browser's printing settings, there is an option to control whether the display is...
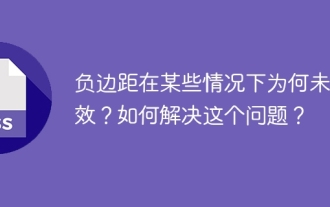
Why do negative margins not take effect in some cases? During programming, negative margins in CSS (negative...
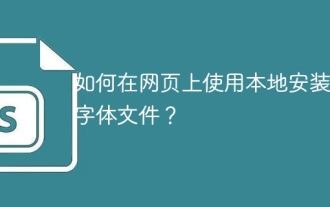
How to use locally installed font files on web pages Have you encountered this situation in web page development: you have installed a font on your computer...
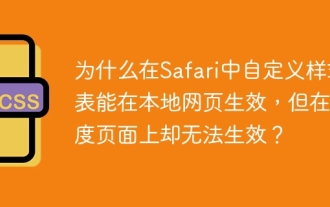
Discussion on using custom stylesheets in Safari Today we will discuss a custom stylesheet application problem for Safari browser. Front-end novice...
