nosql数据库STSDB的一般性使用
接着上一篇这里罗列下STSDB的一般性使用 以下内容基于stsdb4.dll(4.0.3.0版本)库(百度分享资源:http://pan.baidu.com/s/1jGxHE3k),截止本文发布,官方最新版本是4.0.5.0,官方地址:http://stsdb.com/ using System;using System.Collections.Generic;
接着上一篇这里罗列下STSDB的一般性使用
以下内容基于stsdb4.dll(4.0.3.0版本)库(百度分享资源:http://pan.baidu.com/s/1jGxHE3k),截止本文发布,官方最新版本是4.0.5.0,官方地址:http://stsdb.com/
using System; using System.Collections.Generic; namespace STSDB { [Serializable] public class TStudent { public TStudent() { } public string Name { get; set; } public int Age { get; set; } public int GroupNumber { get; set; } public List<TCourse> CourseList { get; set; } } }
using System; namespace STSDB { [Serializable] public class TCourse { public string CourseName { get; set; } public string Teacher { get; set; } public int Score { get; set; } } }
/* * 1. STSdb 4.0 是一个开源的NoSQL 数据库和虚拟文件系统,支持实时索引,完全用c#开发的。 * 引擎原理基于WaterfallTree(瀑布树)数据结构搭建 * * * 2.特性 * 支持几十亿级别的数据存取 * 支持TB级别文件大小 * 实时索引 * 内置压缩 * 内置序列化 * 支持稀疏分散的文件(byte[]) * 存储内存可控 * 支持多线程,且线程安全 * Storage engine instance is thread-safe. Creating (opening) XTable and XFile instances in one storage engine from different threads is thread-safe. XTable and XFile instances are also thread-safe. Manipulating different XTable/XFile instances from different threads is thread-safe. * * 3.缺点 * 不支持事务 * 同时处理所有打开的表 * * 支持多种情况下的数据引擎连接 IStorageEngine engine = STSdb.FromMemory(); //从内存中读取 IStorageEngine engine = STSdb.FromStream(stream); //从数据流中读取 IStorageEngine engine = STSdb.FromHeap(heap); //从堆栈中读取 IStorageEngine engine = STSdb.FromNetwork(host, port); //从远程地址读取 * * */ using System; using System.IO; using System.Linq; using System.Collections.Generic; namespace STSDB { using Newtonsoft.Json; using STSdb4.Data; using STSdb4.Database; using STSdb4.Storage; using STSdb4.WaterfallTree; using STSdb4.Remote.Heap; class Program { static void Main(string[] args) { ExecuteCode(WriteData); ExecuteCode(ReadData); //ExecuteCode(DatabaseSchemeInfo); //ExecuteCode(ReadItem); //ExecuteCode(DeleteItems); //ExecuteCode(ReadItem); //ExecuteCode(GetRecord); //ExecuteCode(PageRecord); //ExecuteCode(Others); //ExecuteCode(ReNameTable); //ExecuteCode(ExistsTable); //ExecuteCode(DeleteTable); //ExecuteCode(ExistsTable); #region test //bool quit = false; //while (!quit) //{ // Console.Write("get item data: "); // string demo = Console.ReadLine(); // switch (demo) // { // case "Y": // break; // case "Q": // quit = true; // break; // default: // Console.WriteLine("Choose a Word between Y and Q(to quit)"); // break; // } //} #endregion Console.ReadKey(); } /// <summary>执行方法</summary> static void ExecuteCode(Action act) { System.Diagnostics.Stopwatch stopwatch = new System.Diagnostics.Stopwatch(); stopwatch.Start(); act(); stopwatch.Stop(); TimeSpan timespan = stopwatch.Elapsed; Console.WriteLine("运行{0}秒", timespan.TotalSeconds); } /// <summary> /// 数据库名 /// </summary> /// <remarks>文件名和扩展名不限制</remarks> protected static string DataBase = "ClassDB.db"; /// <summary> /// 学生表名 /// </summary> protected static string TableName = "tb_student"; /// <summary> /// 【新】学生表名 /// </summary> protected static string NewTableName = "new_tb_student"; /// <summary> /// XFile /// </summary> protected static string XFileName = "tb_file"; #region 基本操作 /// <summary> /// 创建库,写入数据 /// </summary> static void WriteData() { /* * ①:没有数据库会自动创建的,默认目录和应用程序目录一致; * ②:打开表,Key支持组合结构 => OpenXTable<TKey, TRecord> */ using (IStorageEngine engine = STSdb.FromFile(DataBase)) //① { var table = engine.OpenXTable<int, TStudent>(TableName); //② //var table2 = engine.OpenXTable<TKey, TTick>("table2"); //支持key嵌套 for (int i = 0; i < 1000; i++) { table[i] = new TStudent { Name = "Jon_" + i.ToString(), Age = new Random().Next(25, 30), GroupNumber = i + (new Random().Next(310, 399)), CourseList = new List<TCourse>() { new TCourse{ CourseName="C#高级编程"+i.ToString(), Teacher="老陈"+i.ToString(), Score=80 }, new TCourse{ CourseName="C#函数式程序设计"+i.ToString(), Teacher="老李"+i.ToString(), Score=90 }, new TCourse{ CourseName="多线程实战应用"+i.ToString(), Teacher="老张"+i.ToString(), Score=95 }, } }; } engine.Commit(); } } /// <summary> /// 读取数据 /// </summary> static void ReadData() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { var table = engine.OpenXTable<int, TStudent>(TableName); //ITable:IEnumerable对象 foreach (var item in table) Console.WriteLine(JsonConvert.SerializeObject(item, Newtonsoft.Json.Formatting.Indented)); Console.WriteLine(table.Count()); //TableName表中有100行数据 } } /// <summary> /// /// </summary> static void DatabaseSchemeInfo() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) //① { IDescriptor descriptor = engine[TableName]; Console.WriteLine(descriptor.CreateTime.ToString("yyyy-MM-dd HH:mm:ss")); Console.WriteLine(descriptor.ModifiedTime.ToString("yyyy-MM-dd HH:mm:ss")); Console.WriteLine(descriptor.Name); //ID是表的唯一标识id,表一旦创建,它就创建了,后面只要表在就不会修改 //重建表它会从新分配 Console.WriteLine(descriptor.ID); //... } } /// <summary> /// 读取单条数据 /// </summary> static void ReadItem() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { var table = engine.OpenXTable<int, TStudent>(TableName); //ITable: IEnumerable对象 //var item = table.FirstOrDefault(x => x.Key <= 15 && x.Key >= 10); //key是5的记录 //table[10]; var item = table.FirstOrDefault(x => x.Key == 5); //key是5的记录 if (item.Value != null) Console.WriteLine(JsonConvert.SerializeObject(item, Newtonsoft.Json.Formatting.Indented)); else Console.WriteLine("key = 5 的记录不存在!"); //Console.WriteLine("10<= key <= 15 的记录不存在!"); } } static void AddItems() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { var table = engine.OpenXTable<int, TStudent>(TableName); //table[100] = new TStudent(){....}; table.InsertOrIgnore(2, new TStudent()); engine.Commit(); } } /// <summary> /// 删除表数据 /// </summary> static void DeleteItems() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { var table = engine.OpenXTable<int, TStudent>(TableName); //ITable:IEnumerable对象 if (table != null) { //table.Clear(); //清空表数据 table.Delete(5); //删掉key是5的记录 //table.Delete(10, 15); //删掉key从10到15的记录 engine.Commit(); //提交操作,不能少 } } } /// <summary> /// 按需获取数据 /// </summary> static void GetRecord() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { /* Forward向前读取, Backward向后读取, 它们都有2个重载,下面重点说明第二个重载 * Forward(TKey from, bool hasFrom, TKey to, bool hasTo); * Backward(TKey to, bool hasTo, TKey from, bool hasFrom); * 超出范围的都不会排除,另外,查询范围超出也不会有影响,但是要注意一点,formkey和endkey的大小关系 * * 0<----------[(S)]----------------[(E)]------------->N * */ var table = engine.OpenXTable<int, TStudent>(TableName); var fiterTB = table.Forward(2, true, 9, true); //索引从2到9 //var fiterTB = table.Forward(2, false, 9, true); //索引从0到9 //var fiterTB = table.Forward(2, false, 9, false); //索引从0到表结尾 //var fiterTB = table.Forward(2, true, 9, false); //索引从2到表结尾 //Backward刚好相反 foreach (var item in fiterTB) Console.WriteLine(JsonConvert.SerializeObject(item, Newtonsoft.Json.Formatting.Indented)); } } /// <summary> /// 数据分页 /// </summary> static void PageRecord() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { int pageIndex = 2; int pageSize = 10; var table = engine.OpenXTable<int, TStudent>(TableName); var fiterTB = table.Skip(pageSize * (pageIndex - 1)).Take(pageSize); foreach (var item in fiterTB) Console.WriteLine(JsonConvert.SerializeObject(item, Newtonsoft.Json.Formatting.Indented)); } } /// <summary> /// 文件数和记录数 /// </summary> static void Others() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { //表和虚拟文件的数量 Console.WriteLine("数据库 " + DataBase + " 中有 {0} 张表:{1}", engine.Count, TableName); //表记录数 var table = engine.OpenXTable<int, TStudent>(TableName); Console.WriteLine("表" + TableName + "中有" + table.Count() + "条记录"); } } /// <summary> /// 表是否存在 /// </summary> static void ExistsTable() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { //判断表存在与否 //bool exists = engine.Exists(NewTableName); //Console.WriteLine(NewTableName + " exist?=>{0}", exists.ToString()); bool exists = engine.Exists(TableName); Console.WriteLine(TableName + " exist?=>{0}", exists.ToString()); } } /// <summary> /// 重命名表名 /// </summary> static void ReNameTable() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { //判断表存在与否 bool exists = engine.Exists(TableName); Console.WriteLine(TableName + " exist? =>{0}", exists.ToString()); //表重命名 engine.Rename(TableName, NewTableName); Console.WriteLine("表" + TableName + "被重命名为:" + NewTableName); if (engine.Exists(TableName)) Console.WriteLine("old table name \"" + TableName + "\" exist"); if (engine.Exists(NewTableName)) Console.WriteLine("new table name \"" + NewTableName + "\" exist"); } } /// <summary> /// 删除表 /// </summary> static void DeleteTable() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { //删除表 engine.Delete(TableName); //engine.Delete(NewTableName); engine.Commit(); } } #endregion #region XFile static void TestXFile() { using (IStorageEngine engine = STSdb.FromFile(DataBase)) { XFile file = engine.OpenXFile(XFileName); Random random = new Random(); byte[] buffer = new byte[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; for (int i = 0; i < 100; i++) { long position = random.Next(); file.Seek(position, SeekOrigin.Begin); file.Write(buffer, 0, buffer.Length); } engine.Commit(); } } //XFile uses special XTable<long, byte[]> implementation to provide effective sparse file functionality. //One storage engine can have many files #endregion #region Client/Server static void ClientUpdateData() { using (IStorageEngine engine = STSdb.FromNetwork("localhost", 7182)) { ITable<int, string> table = engine.OpenXTable<int, string>("table"); for (int i = 0; i < 100000; i++) { table[i] = i.ToString(); } engine.Commit(); } } static void ServerHandleData() { string _dbname = "test.stsdb4"; using (IStorageEngine engine = STSdb.FromFile(_dbname)) { var server = STSdb.CreateServer(engine, 7182); server.Start(); //server is ready for connections //server.Stop(); } } //The created server instance will listen on the specified port //and receive/send data from/to the clients #endregion #region Memory Usage /* min/max children (branches) in each internal (non-leaf) node max operations in the root node min/max operations in each internal node min/max records in each leaf node number of cached nodes in the memory */ static void MemoryUsageHandle() { using (StorageEngine engine = (StorageEngine)STSdb.FromFile(DataBase)) { //下面的demo都是STS.DB的默认值设置 //min/max children (branches) in each internal node engine.INTERNAL_NODE_MIN_BRANCHES = 2; engine.INTERNAL_NODE_MAX_BRANCHES = 4; //max operations in the root node engine.INTERNAL_NODE_MAX_OPERATIONS_IN_ROOT = 8 * 1024; //min/max operations in each internal node engine.INTERNAL_NODE_MIN_OPERATIONS = 64 * 1024; engine.INTERNAL_NODE_MAX_OPERATIONS = 128 * 1024; //min/max records in each leaf node engine.LEAF_NODE_MIN_RECORDS = 16 * 1024; engine.LEAF_NODE_MAX_RECORDS = 128 * 1024; //at least 2 x MIN_RECORDS //number of cached nodes in memory engine.CacheSize = 32; } } #endregion #region Heap /*using => STSdb4.WaterfallTree; STSdb4.Storage; STSdb4.Remote.Heap; */ static void HeaperEngine() { //Server端 IHeap heap = new Heap(new FileStream("Heap.db", FileMode.OpenOrCreate)); HeapServer server = new HeapServer(heap, 7183); //监听堆服务器 server.Start(); //开始监听 //从远程堆中创建 IStorageEngine 引擎,并处理数据 //using (IStorageEngine engine = STSdb.FromHeap(new RemoteHeap("host", 7183))) //{ // ITable<int, string> table = engine.OpenXTable<int, string>("table"); // for (int i = 0; i < 100000; i++) // { // table[i] = i.ToString(); // } // engine.Commit(); //} } #endregion //... } }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
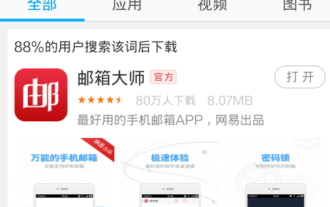
NetEase Mailbox, as an email address widely used by Chinese netizens, has always won the trust of users with its stable and efficient services. NetEase Mailbox Master is an email software specially created for mobile phone users. It greatly simplifies the process of sending and receiving emails and makes our email processing more convenient. So how to use NetEase Mailbox Master, and what specific functions it has. Below, the editor of this site will give you a detailed introduction, hoping to help you! First, you can search and download the NetEase Mailbox Master app in the mobile app store. Search for "NetEase Mailbox Master" in App Store or Baidu Mobile Assistant, and then follow the prompts to install it. After the download and installation is completed, we open the NetEase email account and log in. The login interface is as shown below
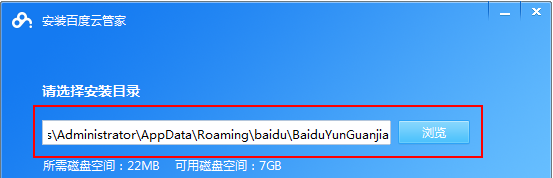
Cloud storage has become an indispensable part of our daily life and work nowadays. As one of the leading cloud storage services in China, Baidu Netdisk has won the favor of a large number of users with its powerful storage functions, efficient transmission speed and convenient operation experience. And whether you want to back up important files, share information, watch videos online, or listen to music, Baidu Cloud Disk can meet your needs. However, many users may not understand the specific use method of Baidu Netdisk app, so this tutorial will introduce in detail how to use Baidu Netdisk app. Users who are still confused can follow this article to learn more. ! How to use Baidu Cloud Network Disk: 1. Installation First, when downloading and installing Baidu Cloud software, please select the custom installation option.
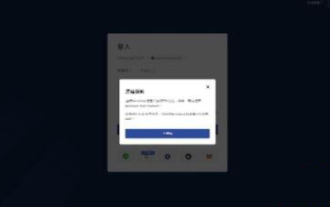
MetaMask (also called Little Fox Wallet in Chinese) is a free and well-received encryption wallet software. Currently, BTCC supports binding to the MetaMask wallet. After binding, you can use the MetaMask wallet to quickly log in, store value, buy coins, etc., and you can also get 20 USDT trial bonus for the first time binding. In the BTCCMetaMask wallet tutorial, we will introduce in detail how to register and use MetaMask, and how to bind and use the Little Fox wallet in BTCC. What is MetaMask wallet? With over 30 million users, MetaMask Little Fox Wallet is one of the most popular cryptocurrency wallets today. It is free to use and can be installed on the network as an extension
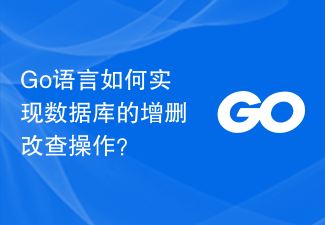
Go language is an efficient, concise and easy-to-learn programming language. It is favored by developers because of its advantages in concurrent programming and network programming. In actual development, database operations are an indispensable part. This article will introduce how to use Go language to implement database addition, deletion, modification and query operations. In Go language, we usually use third-party libraries to operate databases, such as commonly used sql packages, gorm, etc. Here we take the sql package as an example to introduce how to implement the addition, deletion, modification and query operations of the database. Assume we are using a MySQL database.
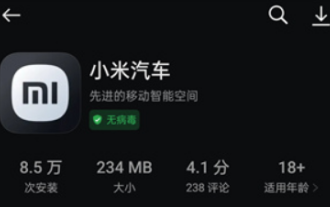
Xiaomi car software provides remote car control functions, allowing users to remotely control the vehicle through mobile phones or computers, such as opening and closing the vehicle's doors and windows, starting the engine, controlling the vehicle's air conditioner and audio, etc. The following is the use and content of this software, let's learn about it together . Comprehensive list of Xiaomi Auto app functions and usage methods 1. The Xiaomi Auto app was launched on the Apple AppStore on March 25, and can now be downloaded from the app store on Android phones; Car purchase: Learn about the core highlights and technical parameters of Xiaomi Auto, and make an appointment for a test drive. Configure and order your Xiaomi car, and support online processing of car pickup to-do items. 3. Community: Understand Xiaomi Auto brand information, exchange car experience, and share wonderful car life; 4. Car control: The mobile phone is the remote control, remote control, real-time security, easy
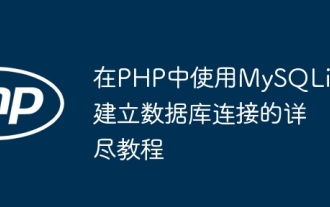
How to use MySQLi to establish a database connection in PHP: Include MySQLi extension (require_once) Create connection function (functionconnect_to_db) Call connection function ($conn=connect_to_db()) Execute query ($result=$conn->query()) Close connection ( $conn->close())
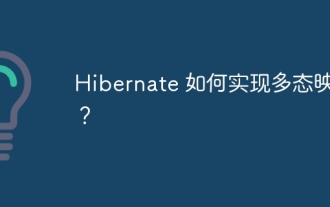
Hibernate polymorphic mapping can map inherited classes to the database and provides the following mapping types: joined-subclass: Create a separate table for the subclass, including all columns of the parent class. table-per-class: Create a separate table for subclasses, containing only subclass-specific columns. union-subclass: similar to joined-subclass, but the parent class table unions all subclass columns.
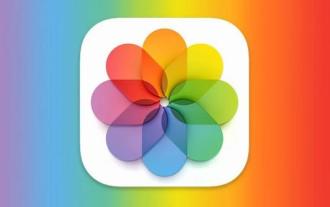
Apple's latest releases of iOS18, iPadOS18 and macOS Sequoia systems have added an important feature to the Photos application, designed to help users easily recover photos and videos lost or damaged due to various reasons. The new feature introduces an album called "Recovered" in the Tools section of the Photos app that will automatically appear when a user has pictures or videos on their device that are not part of their photo library. The emergence of the "Recovered" album provides a solution for photos and videos lost due to database corruption, the camera application not saving to the photo library correctly, or a third-party application managing the photo library. Users only need a few simple steps
